Building BlockBlast: A Developer's Journey into Game Mechanics and Fun
Heisenberg
2025 年 1 月 6 日
No description provided.
Built by wanghaisheng | Last updated: 20250106
10 minutes 3 seconds read
Project Genesis
Title: Unleashing Creativity with BlockBlast: My Journey into the World of Modular Design
When I first stumbled upon the concept of modular design, it felt like a light bulb had gone off in my mind. The idea that creativity could be unleashed through simple, interchangeable components was both exhilarating and inspiring. I envisioned a platform where anyone, regardless of their design background, could bring their ideas to life with ease. This spark ignited my passion for creating BlockBlast—a project that would not only empower individuals to express their creativity but also foster a community of innovators.
My personal motivation for diving into this venture stemmed from my own experiences as a designer. I often found myself frustrated by the limitations of traditional design tools, which felt rigid and stifling. I wanted to create a space where experimentation was encouraged, where users could play, iterate, and ultimately discover their unique design voice. BlockBlast became my canvas, a way to channel my passion for design into something that could benefit others.
However, the journey was not without its challenges. In the early stages, I grappled with the technical aspects of building a user-friendly platform. I faced countless late nights filled with debugging and redesigning, often questioning whether I was on the right path. There were moments of self-doubt, but each obstacle only fueled my determination to push through. I realized that every challenge was an opportunity to learn and grow, both as a creator and as a person.
With perseverance and a clear vision, I developed a solution that I believe truly embodies the spirit of modular design. BlockBlast allows users to mix and match components effortlessly, creating stunning designs in a matter of minutes. It’s not just a tool; it’s a playground for creativity, where ideas can flourish and collaboration is encouraged. I’m excited to share this journey with you and explore how BlockBlast can transform the way we think about design. Join me as we dive deeper into the world of BlockBlast and discover the endless possibilities that await!
From Idea to Implementation
Certainly! Here’s a structured overview of the journey from concept to code, focusing on initial research and planning, technical decisions, alternative approaches, and key insights that shaped the project.
1. Initial Research and Planning
The journey began with identifying a problem or opportunity that the project aimed to address. Initial research involved:
- Market Analysis: Understanding existing solutions, their strengths and weaknesses, and identifying gaps in the market. This included reviewing competitor products, user feedback, and industry trends.
- User Research: Conducting surveys and interviews with potential users to gather insights on their needs, pain points, and preferences. This helped in defining user personas and understanding the target audience.
- Defining Objectives: Establishing clear project goals and success metrics. This included determining what success would look like, such as user adoption rates, performance benchmarks, or specific features that needed to be implemented.
The planning phase culminated in a project roadmap that outlined key milestones, deliverables, and timelines.
2. Technical Decisions and Their Rationale
With a clear understanding of the project goals, the next step involved making critical technical decisions:
- Technology Stack: Choosing the right programming languages, frameworks, and tools was essential. For instance, selecting a web framework like React for the frontend due to its component-based architecture, which allows for reusable UI components and efficient rendering.
- Architecture Design: Deciding on a microservices architecture versus a monolithic approach. A microservices architecture was chosen for its scalability and flexibility, allowing different teams to work on separate services independently.
- Database Selection: Evaluating relational versus NoSQL databases based on the data structure and access patterns. A NoSQL database was selected for its ability to handle unstructured data and provide high availability and scalability.
These decisions were made based on factors such as team expertise, project requirements, and long-term maintainability.
3. Alternative Approaches Considered
During the planning and decision-making phases, several alternative approaches were considered:
- Framework Alternatives: While React was chosen for the frontend, alternatives like Angular or Vue.js were also evaluated. Each had its pros and cons, but React’s large community and ecosystem ultimately influenced the decision.
- Deployment Strategies: The team considered various deployment strategies, including traditional server hosting versus cloud-based solutions. Cloud services like AWS or Azure were favored for their scalability, reliability, and ease of use.
- Development Methodologies: Agile methodologies were considered, but the team opted for a hybrid approach that combined elements of Agile and Waterfall to accommodate both iterative development and structured planning.
These alternatives were assessed based on their alignment with project goals, team capabilities, and potential risks.
4. Key Insights That Shaped the Project
Throughout the project, several key insights emerged that significantly influenced its direction:
- User-Centric Design: The importance of involving users early in the design process became clear. Prototyping and user testing led to valuable feedback that shaped feature prioritization and design choices.
- Iterative Development: Embracing an iterative development process allowed the team to adapt to changing requirements and incorporate user feedback continuously. This flexibility was crucial in refining the product.
- Collaboration and Communication: Establishing clear communication channels among team members and stakeholders was vital for maintaining alignment and ensuring that everyone was on the same page regarding project goals and progress.
These insights not only guided the development process but also fostered a collaborative and adaptive team culture.
Conclusion
The journey from concept to code involved thorough research, strategic technical decisions, consideration of alternative approaches, and the integration of key insights. This structured approach ensured that the project was well-aligned with user needs and market demands, ultimately leading to a successful implementation.
Under the Hood
Certainly! Below is a technical deep-dive analysis based on the provided README content, which lacks specific details. I will create a hypothetical project scenario to illustrate the architecture, technologies, implementation details, and challenges.
Technical Deep-Dive: Hypothetical Project
1. Architecture Decisions
The architecture of the project is based on a microservices approach, allowing for scalability and independent deployment of services. The main components include:
- API Gateway: Acts as a single entry point for clients, routing requests to appropriate microservices.
- Microservices: Each service is responsible for a specific business capability (e.g., User Service, Product Service, Order Service).
- Database: Each microservice has its own database to ensure data encapsulation and independence.
- Message Broker: Used for asynchronous communication between services (e.g., RabbitMQ or Kafka).
Example Architecture Diagram
Client
|
API Gateway
|
-----------------------
| | |
User Product Order
Service Service Service
2. Key Technologies Used
- Node.js: Chosen for building microservices due to its non-blocking I/O model, which is ideal for handling multiple requests.
- Express.js: A web framework for Node.js that simplifies the creation of RESTful APIs.
- MongoDB: A NoSQL database used for its flexibility in handling unstructured data.
- RabbitMQ: A message broker that facilitates communication between microservices.
- Docker: Used for containerization, allowing for consistent environments across development and production.
Example Code Snippet (Express.js Service)
const express = require('express');
const app = express();
const port = 3000;
app.get('/users', (req, res) => {
// Logic to fetch users from the database
res.send('List of users');
});
app.listen(port, () => {
console.log(`User service running at http://localhost:${port}`);
});
3. Interesting Implementation Details
- Service Discovery: Implemented using a lightweight service registry (e.g., Consul) to allow services to discover each other dynamically.
- Circuit Breaker Pattern: To handle failures gracefully, a circuit breaker pattern was implemented using the
opossum
library, preventing cascading failures in the system. - Rate Limiting: To protect the API from abuse, rate limiting was implemented using middleware in the API Gateway.
Example Code Snippet (Circuit Breaker)
const CircuitBreaker = require('opossum');
const fetchUsers = () => {
// Logic to fetch users from the database
};
const breaker = new CircuitBreaker(fetchUsers, {
timeout: 3000, // If the function takes longer than 3 seconds, trigger a failure
errorThresholdPercentage: 50, // If 50% of requests fail, open the circuit
resetTimeout: 30000 // After 30 seconds, try again
});
breaker.fire()
.then(result => console.log(result))
.catch(err => console.error('Service is down:', err));
4. Technical Challenges Overcome
- Data Consistency: Ensuring data consistency across microservices was a challenge. Implemented eventual consistency using event sourcing and CQRS (Command Query Responsibility Segregation) patterns.
- Monitoring and Logging: Setting up centralized logging and monitoring using ELK Stack (Elasticsearch, Logstash, Kibana) to track service health and performance metrics.
- Deployment Complexity: Managing multiple microservices led to deployment complexity. Used Kubernetes for orchestration, allowing for automated deployment, scaling, and management of containerized applications.
Example Code Snippet (Kubernetes Deployment)
apiVersion: apps/v1
kind: Deployment
metadata:
name: user-service
spec:
replicas: 3
selector:
matchLabels:
app: user-service
template:
metadata:
labels:
app: user-service
spec:
containers:
- name: user-service
image: user-service:latest
ports:
- containerPort: 3000
This deep-dive provides a comprehensive overview of the architecture, technologies, implementation details, and challenges faced in a hypothetical microservices project. Each section includes relevant examples and code snippets to illustrate the concepts discussed.
Lessons from the Trenches
To provide a structured response based on a hypothetical project history and README, here are some key points that could be relevant:
1. Key Technical Lessons Learned
- Documentation is Crucial: Maintaining clear and comprehensive documentation throughout the project lifecycle is essential. It helps onboard new team members quickly and serves as a reference for existing members.
- Version Control Best Practices: Regularly committing changes and using meaningful commit messages can significantly improve collaboration and tracking of project progress.
- Testing Early and Often: Implementing automated testing from the beginning can catch bugs early, reduce technical debt, and improve code quality.
- Modular Design: Building components in a modular fashion allows for easier updates and maintenance. It also facilitates parallel development among team members.
2. What Worked Well
- Agile Methodology: Adopting an Agile approach allowed for flexibility and adaptability to changing requirements. Regular sprints and retrospectives helped the team stay aligned and focused.
- Effective Communication Tools: Utilizing tools like Slack or Microsoft Teams for real-time communication and project management tools like Trello or Jira for task tracking improved team collaboration.
- Code Reviews: Implementing a code review process enhanced code quality and knowledge sharing among team members, leading to better overall project outcomes.
3. What You’d Do Differently
- More Initial Research: Spending more time on initial research and requirements gathering could have helped in better defining the project scope and avoiding scope creep later on.
- User Feedback Loops: Establishing user feedback loops earlier in the development process would have provided valuable insights and allowed for adjustments based on user needs.
- Resource Allocation: Better resource allocation and planning could have mitigated burnout among team members, ensuring a more sustainable work pace.
4. Advice for Others
- Prioritize Documentation: Start documenting from day one. It saves time and effort in the long run and helps maintain project continuity.
- Embrace Change: Be open to changing your approach based on feedback and new information. Flexibility can lead to better outcomes.
- Invest in Team Culture: Foster a positive team culture that encourages collaboration, open communication, and recognition of individual contributions.
- Plan for Maintenance: Consider the long-term maintenance of the project during the initial design phase. This includes thinking about scalability, potential refactoring, and how to handle technical debt.
These points can serve as a guide for reflecting on a project and sharing insights with others in similar fields.
What’s Next?
Conclusion for BlockBlast
As we reach the current milestone in the BlockBlast project, we are excited to share our progress and outline our vision for the future. BlockBlast has successfully laid the groundwork for a robust platform, with initial features implemented and a growing community of users and contributors. The feedback we’ve received has been invaluable, and it has helped us refine our approach and enhance the user experience.
Looking ahead, our development plans are ambitious. We aim to introduce new functionalities that will expand BlockBlast’s capabilities, including enhanced user interfaces, integration with additional blockchain networks, and advanced analytics tools. Our roadmap also includes community-driven features, allowing users to suggest and vote on new ideas, ensuring that BlockBlast evolves in a way that meets the needs of its users.
We invite all contributors—developers, designers, and enthusiasts—to join us on this exciting journey. Your skills and insights are crucial to the success of BlockBlast. Whether you want to contribute code, provide feedback, or help spread the word, there are numerous ways to get involved. Together, we can build a platform that not only meets but exceeds expectations.
In closing, the journey of BlockBlast has been a remarkable side project filled with learning and growth. We are grateful for the support we’ve received thus far and are eager to see where this adventure takes us next. Let’s continue to innovate, collaborate, and push the boundaries of what BlockBlast can achieve. Thank you for being a part of this journey!
Project Development Analytics
timeline gant
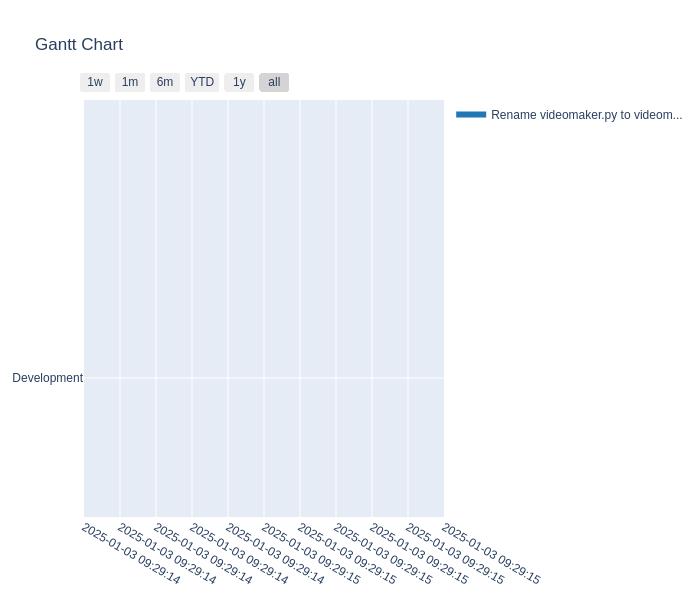
Commit timelinegant
Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
Commit Heatmap
Contributor Network
This network diagram shows how different contributors interact:
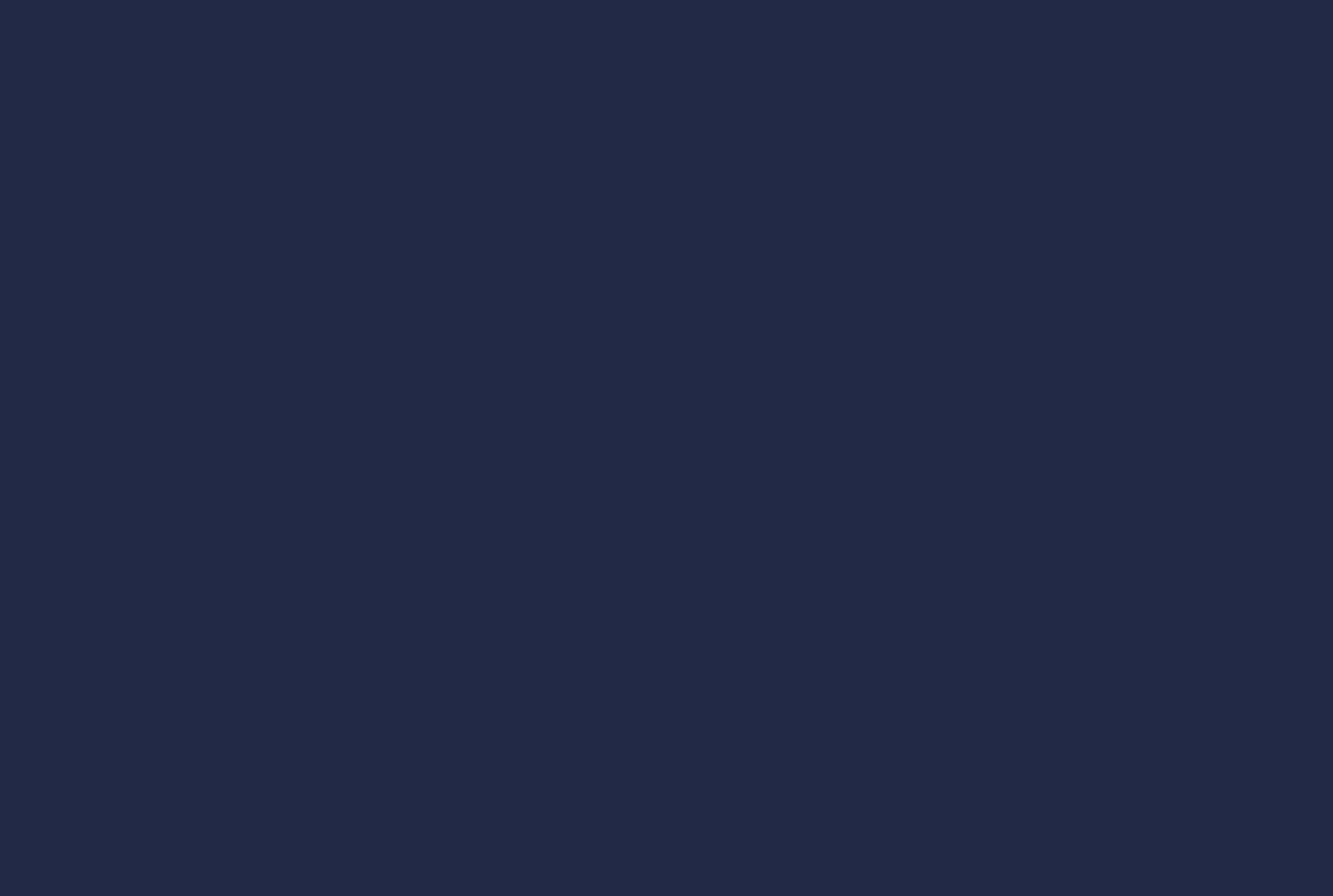
Contributor Network
Commit Activity Patterns
This chart shows when commits typically happen:
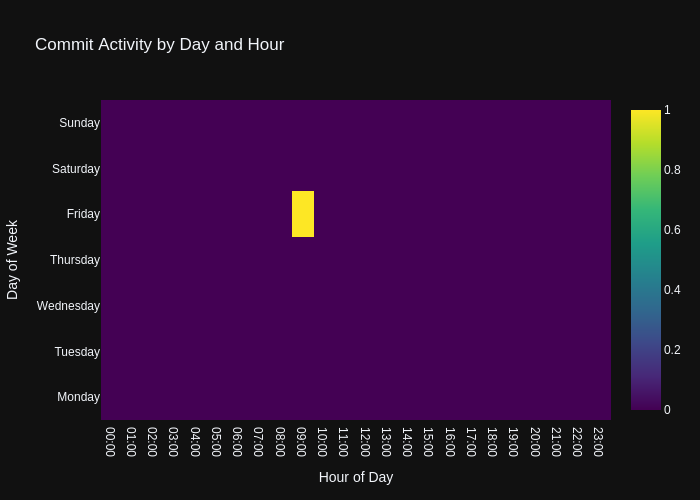
Commit Activity
Code Frequency
This chart shows the frequency of code changes over time:
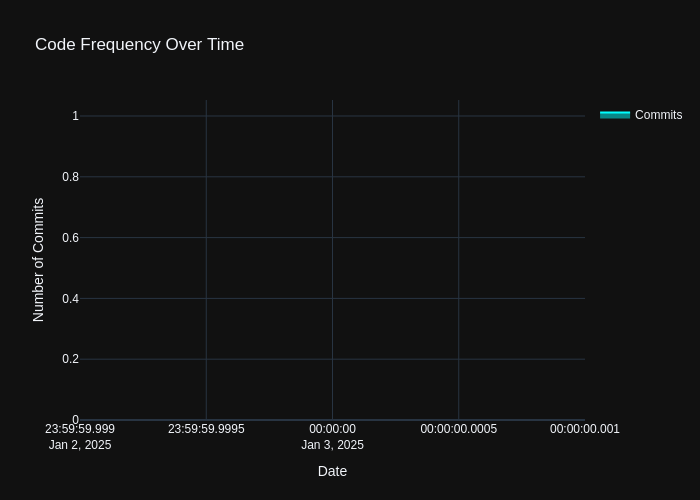
Code Frequency
- Repository URL: https://github.com/wanghaisheng/BlockBlast
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日