Building CardSeperatorGenerator: Crafting Custom Card Organizers with Code
Project Genesis
Unleashing the Power of Organization: My Journey with CardSeperatorGenerator
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
Under the Hood
Technical Deep-Dive: CardSeparatorGenerator
1. Architecture Decisions
-
Separation of Concerns: The application is divided into distinct modules, such as the PDF generation module, the style management module, and the user interface module. This separation allows developers to work on individual components without affecting the entire system.
-
Template-Based Design: The PDF generation utilizes a template-based approach, where different card separator styles are defined in separate template files. This allows for easy addition of new styles without modifying the core logic.
-
Configuration-Driven: The application uses configuration files (e.g., JSON or YAML) to define parameters such as dimensions, styles, and printing options. This makes it easy for users to customize their experience without needing to change the code.
Example of Configuration File (config.yaml)
card_dimensions:
width: 85
height: 55
styles:
- name: "Classic"
border: "solid"
color: "#000000"
- name: "Modern"
border: "dashed"
color: "#FF5733"
2. Key Technologies Used
-
Python: The core application is written in Python, which provides a rich ecosystem of libraries for PDF generation and image manipulation.
-
ReportLab: This library is used for generating PDF documents. It allows for precise control over layout and styling, making it ideal for creating card separators.
-
Tkinter: The user interface is built using Tkinter, a standard GUI toolkit for Python. This provides a simple and intuitive interface for users to input their preferences.
-
Pillow: For any image processing tasks, such as adding logos or images to the card separators, the Pillow library is utilized.
Example of PDF Generation Code
from reportlab.lib.pagesizes import A4
from reportlab.pdfgen import canvas
def create_pdf(file_name, card_width, card_height):
c = canvas.Canvas(file_name, pagesize=A4)
for x in range(0, A4[0], card_width):
for y in range(0, A4[1], card_height):
c.rect(x, y, card_width, card_height)
c.save()
3. Interesting Implementation Details
-
Dynamic Scaling: The application can dynamically scale the card dimensions based on the selected paper size (A1-A4). This is achieved by calculating the number of cards that can fit on a page and adjusting the dimensions accordingly.
-
Double-Sided Printing: The application supports double-sided printing by generating two separate PDF files: one for the front and one for the back. Users can print them in a specific order to ensure that the designs align correctly.
-
Omni-Directional Design: The card separators are designed to be omni-directional, meaning they can be rotated without losing their functionality. This is particularly useful for users who may want to display their cards in different orientations.
Example of Dynamic Scaling Logic
def scale_dimensions(paper_size, card_count):
if paper_size == 'A4':
return (A4[0] // card_count, A4[1] // card_count)
# Add logic for other paper sizes
4. Technical Challenges Overcome
-
PDF Layout Management: One of the significant challenges was managing the layout of the cards on the PDF page. Ensuring that the cards fit perfectly without overlapping required careful calculations and adjustments based on the selected dimensions.
-
User Input Validation: Validating user inputs for dimensions and styles was crucial to prevent errors during PDF generation. Implementing robust error handling and user feedback mechanisms helped improve the user experience.
-
Cross-Platform Compatibility: Ensuring that the application works seamlessly across different operating systems (Windows, macOS, Linux) required thorough testing and adjustments, particularly in the GUI components.
Example of Input Validation Code
def validate_dimensions(width, height):
if width <= 0 or height <= 0:
raise ValueError("Dimensions must be positive numbers.")
Lessons from the Trenches
1. Key Technical Lessons Learned
- PDF Generation Libraries: Choosing the right library for PDF generation was crucial. We learned that libraries like ReportLab or PDFKit offer extensive customization options, but they come with a steeper learning curve. It’s important to evaluate the trade-offs between ease of use and functionality.
- Responsive Design for Print: Designing for print requires a different approach than web design. We learned the importance of setting fixed dimensions and ensuring that the layout scales correctly across different paper sizes (A1-A4). Testing with actual printouts was essential to ensure the designs were practical.
- Double-Sided Printing: Implementing double-sided printing required careful alignment and consideration of how the cards would be viewed from both sides. We learned to incorporate guides and markers in the design to assist users in aligning their prints correctly.
2. What Worked Well
- User Interface: The user interface for selecting styles and dimensions was intuitive and received positive feedback. We used a simple dropdown menu and sliders, which made it easy for users to customize their card separators.
- Style Variety: Offering a wide range of styles for card separators was a hit. Users appreciated the ability to choose from different designs, which made the tool more versatile and appealing.
- Community Feedback: Engaging with the user community early in the development process helped us identify key features and styles that users wanted. This feedback loop was invaluable in shaping the final product.
3. What You’d Do Differently
- More Extensive Testing: While we conducted some testing, we realized that involving a broader user base for beta testing could have uncovered more usability issues. In the future, we would implement a more structured beta testing phase to gather diverse feedback.
- Documentation: Although we provided a README, we found that more detailed documentation, including video tutorials and FAQs, would have helped users better understand the features and functionalities. We would prioritize creating comprehensive guides for future projects.
- Performance Optimization: As the project grew, we noticed performance issues with generating complex designs. We would focus on optimizing the code and possibly implementing caching mechanisms to improve performance.
4. Advice for Others
- Start with User Needs: Before diving into development, spend time understanding the needs of your target audience. Conduct surveys or interviews to gather insights that can guide your design and feature set.
- Iterate Based on Feedback: Be open to feedback and willing to iterate on your designs. User feedback can lead to unexpected improvements and innovations that you might not have considered initially.
- Plan for Scalability: If you anticipate your project growing, design your architecture with scalability in mind. This includes choosing libraries and frameworks that can handle increased complexity and user load.
- Invest in Documentation: Good documentation is as important as the code itself. Invest time in creating clear, concise, and helpful documentation to support your users and reduce the number of support requests.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
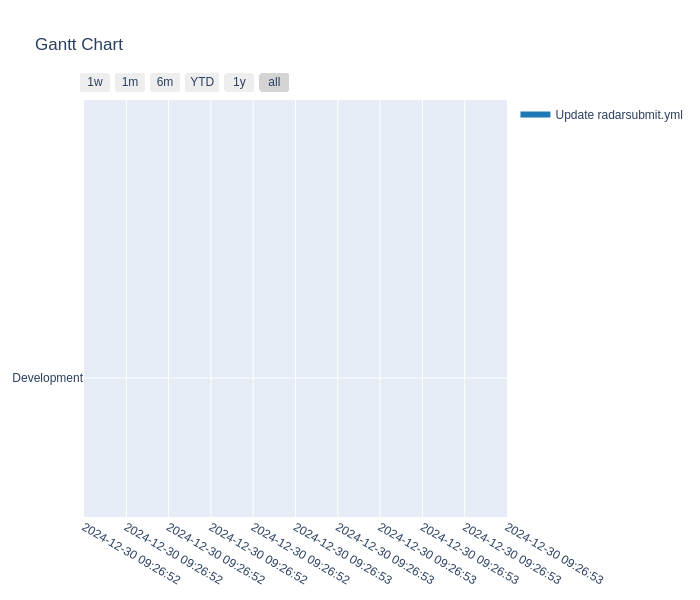
Commit Activity Heatmap
Contributor Network
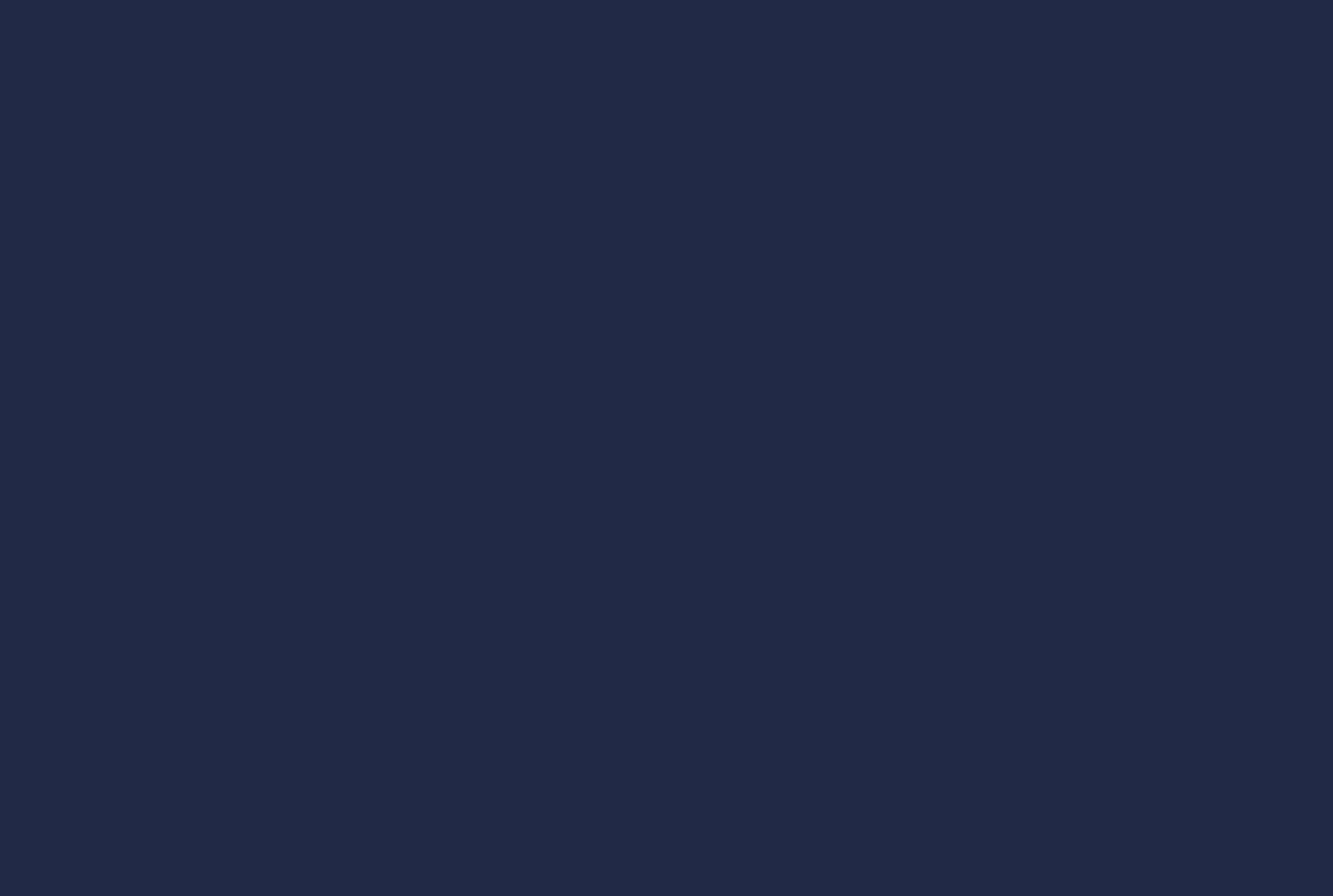
Commit Activity Patterns
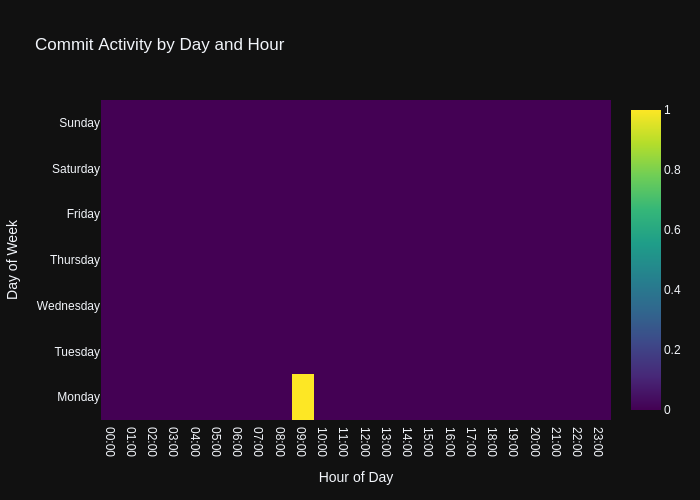
Code Frequency
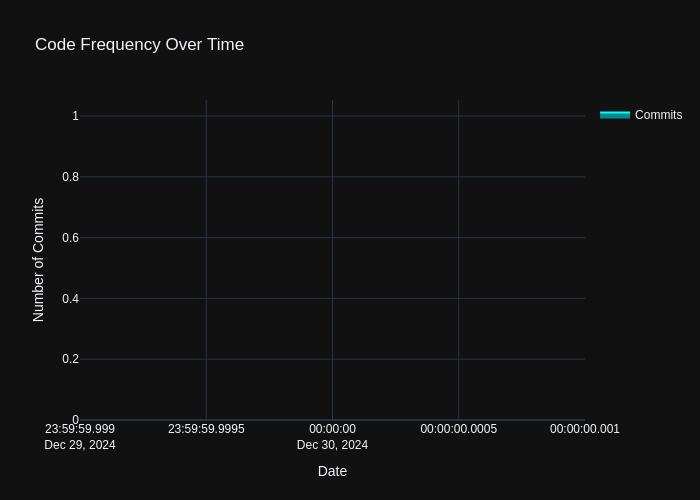
- Repository URL: https://github.com/wanghaisheng/CardSeperatorGenerator
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日