Building Reid Store: My Journey Creating a React E-Commerce Platform
Project Genesis
Welcome to the World of Reid: A Fashion E-commerce Journey
From Idea to Implementation
Reid - Fashion Ecommerce Website: Journey from Concept to Code
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
Under the Hood
Technical Deep-Dive: Reid - Fashion Ecommerce Website
1. Architecture Decisions
-
Client-Server Architecture: The application follows a client-server model where the frontend is built using React.js, and the backend is powered by Strapi, a headless CMS. This separation allows for a clear distinction between the user interface and data management.
-
API-First Approach: By utilizing GraphQL for data fetching, the application can request only the data it needs, reducing over-fetching and improving performance. This is particularly beneficial for ecommerce applications where data requirements can vary significantly between different views.
-
State Management: Redux Toolkit is employed for state management, providing a predictable state container that simplifies the management of application state across various components.
-
Responsive Design: The use of MUI (Material-UI) ensures that the application is responsive and adheres to modern design principles, enhancing user experience across devices.
2. Key Technologies Used
-
React.js: A JavaScript library for building user interfaces, allowing for the creation of reusable UI components.
-
TypeScript: A superset of JavaScript that adds static typing, improving code quality and maintainability.
-
Redux Toolkit: A library that simplifies the process of managing application state in React applications.
-
MUI: A popular React UI framework that provides pre-built components adhering to Material Design guidelines.
-
Framer Motion: A library for animations in React, enhancing the user experience with smooth transitions and animations.
-
Strapi: A headless CMS that provides a flexible API for managing content, making it easy to create and manage products, categories, and other data.
-
GraphQL: A query language for APIs that allows clients to request only the data they need.
-
Stripe: A payment processing platform that enables secure transactions for ecommerce applications.
3. Interesting Implementation Details
GraphQL Integration
query GetProduct($id: ID!) {
product(id: $id) {
id
name
price
description
images {
url
}
}
}
State Management with Redux Toolkit
import { createSlice } from '@reduxjs/toolkit';
const productSlice = createSlice({
name: 'products',
initialState: [],
reducers: {
setProducts(state, action) {
return action.payload;
},
addProduct(state, action) {
state.push(action.payload);
},
},
});
export const { setProducts, addProduct } = productSlice.actions;
export default productSlice.reducer;
Animations with Framer Motion
import { motion } from 'framer-motion';
const ProductCard = ({ product }) => (
<motion.div
initial={{ opacity: 0 }}
animate={{ opacity: 1 }}
transition={{ duration: 0.5 }}
>
<h2>{product.name}</h2>
<p>{product.price}</p>
</motion.div>
);
4. Technical Challenges Overcome
Handling Asynchronous Data Fetching
import { useQuery } from 'react-query';
const useProduct = (id) => {
return useQuery(['product', id], () => fetchProduct(id));
};
Payment Integration with Stripe
const createCheckoutSession = async (items) => {
const response = await fetch('/api/checkout_sessions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
## Lessons from the Trenches
Certainly! Here’s a structured response based on the project history and README for the Reid Fashion Ecommerce Website:
### Key Technical Lessons Learned
1. **State Management with Redux Toolkit**: Implementing Redux Toolkit for state management significantly simplified the process of managing global state across the application. I learned the importance of using slices and reducers effectively to keep the state predictable and maintainable.
2. **Type Safety with TypeScript**: Utilizing TypeScript helped catch errors during development, leading to more robust code. I learned to define interfaces for props and state, which improved code clarity and reduced runtime errors.
3. **GraphQL for Data Fetching**: Using GraphQL instead of REST APIs allowed for more efficient data fetching. I learned how to structure queries and mutations, which provided flexibility in retrieving only the data needed for each component.
4. **Integrating Payment Systems with Stripe**: Implementing Stripe for payment processing taught me about handling sensitive data securely and managing payment workflows. I learned the importance of testing payment flows thoroughly to ensure a smooth user experience.
### What Worked Well
1. **Responsive Design with MUI**: The Material-UI (MUI) library facilitated the creation of a responsive and visually appealing design. The pre-built components saved time and ensured consistency across the application.
2. **Smooth Animations with Framer Motion**: Integrating Framer Motion for animations enhanced the user experience. The animations were easy to implement and added a professional touch to the website.
3. **Content Management with Strapi**: Using Strapi as a headless CMS allowed for easy content management. It provided a user-friendly interface for non-technical users to update product information without needing to modify the codebase.
### What You'd Do Differently
1. **Improved Testing Coverage**: While the application is functional, I would focus on increasing the testing coverage, particularly for critical components and user flows. Implementing unit tests and integration tests would help catch issues earlier in the development process.
2. **Performance Optimization**: I would conduct a more thorough performance audit to identify and address any bottlenecks. This could include optimizing images, lazy loading components, and minimizing bundle sizes to improve load times.
3. **User Feedback Loop**: Establishing a more structured user feedback loop during the development phase would help identify usability issues earlier. Conducting user testing sessions could provide valuable insights into user behavior and preferences.
### Advice for Others
1. **Plan Your State Management Early**: Before diving into development, take the time to plan your state management strategy. This will save you from potential headaches later on as your application grows in complexity.
2. **Leverage Documentation and Community Resources**: Don’t hesitate to utilize the extensive documentation and community resources available for the technologies you are using. They can provide solutions to common problems and best practices that can save you time.
3. **Iterate Based on User Feedback**: Always be open to feedback from users. Iterating based on real user experiences can lead to a more successful product that meets the needs of your audience.
4. **Stay Updated with Technology Trends**: The tech landscape is constantly evolving. Stay informed about new tools, libraries, and best practices to keep your skills sharp and your projects modern.
By reflecting on these aspects, you can gain valuable insights that will enhance your future projects and development practices.
## What's Next?
## Conclusion
As we wrap up this phase of the Reid fashion eCommerce website project, we are excited to share our current status and future development plans. The website is live and operational, showcasing a sleek design and user-friendly interface built with cutting-edge technologies such as React, TypeScript, and GraphQL. Our team has successfully integrated essential features, including a seamless checkout process powered by Stripe, ensuring a smooth shopping experience for our users.
Looking ahead, we have ambitious plans for Reid. Our roadmap includes enhancing the user experience with personalized recommendations, expanding our product catalog, and implementing advanced analytics to better understand our customers' needs. We also aim to optimize our website's performance and accessibility, ensuring that Reid remains a top choice for fashion enthusiasts. Additionally, we are exploring partnerships with local designers to feature exclusive collections, further enriching our offerings.
We invite all contributors—developers, designers, and fashion aficionados—to join us on this exciting journey. Your skills and insights can help shape Reid into a leading fashion destination. Whether you want to contribute code, share design ideas, or provide feedback on user experience, your involvement is invaluable. Together, we can create a platform that not only meets but exceeds the expectations of our customers.
In closing, the journey of building Reid has been both challenging and rewarding. Each step has taught us valuable lessons about collaboration, creativity, and the ever-evolving landscape of eCommerce. We are grateful for the support we've received so far and look forward to what lies ahead. Let’s continue to innovate and inspire in the world of fashion together!
## Project Development Analytics
### timeline gant
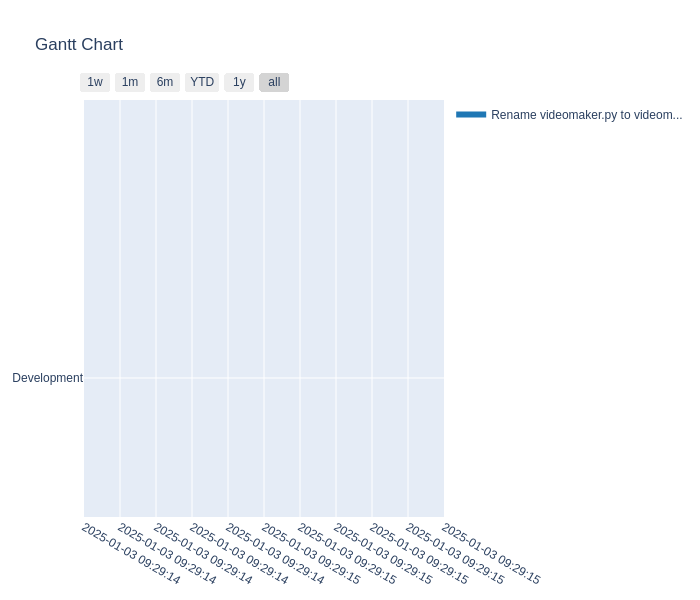
### Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
![Commit Heatmap]()
### Contributor Network
This network diagram shows how different contributors interact:
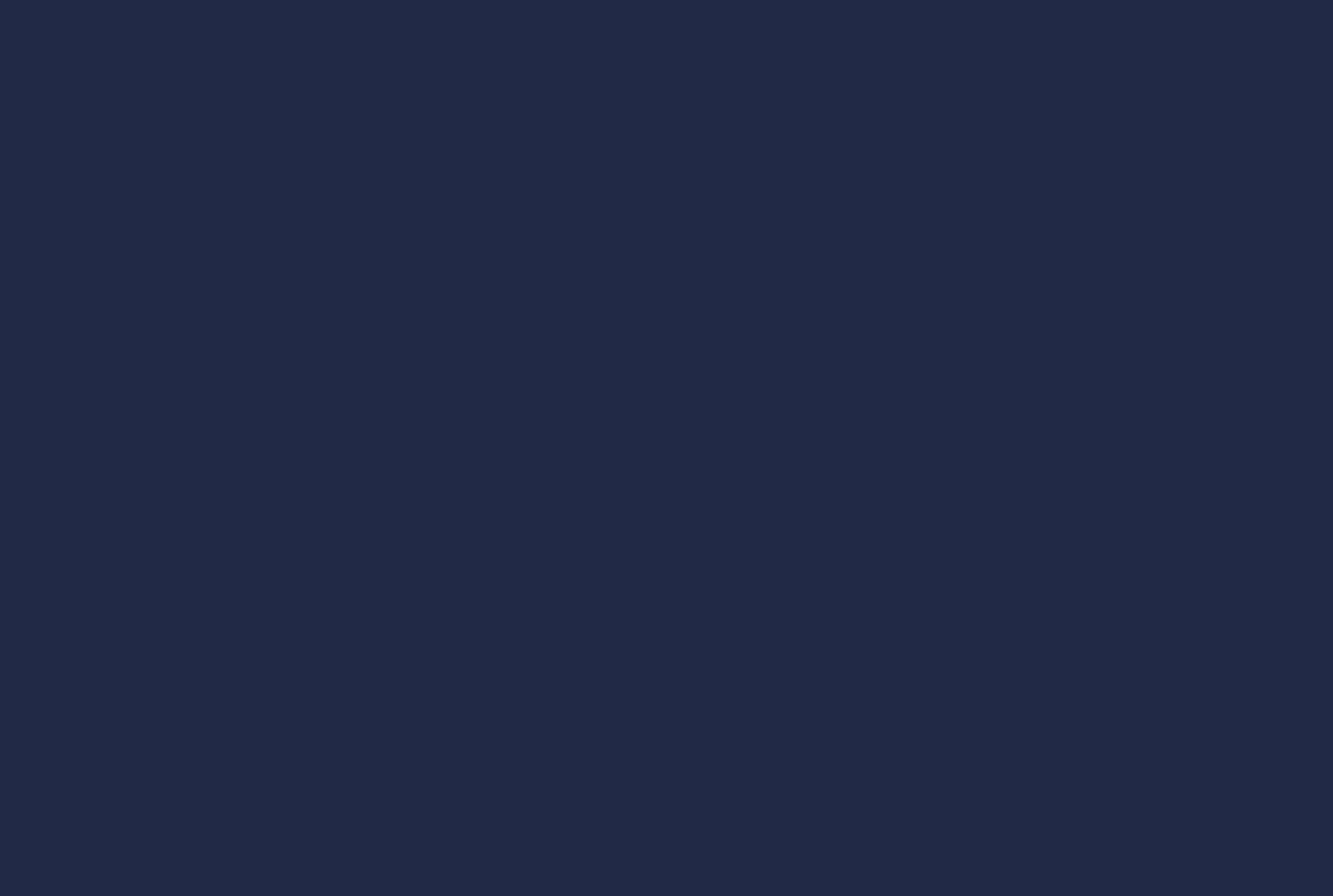
### Commit Activity Patterns
This chart shows when commits typically happen:
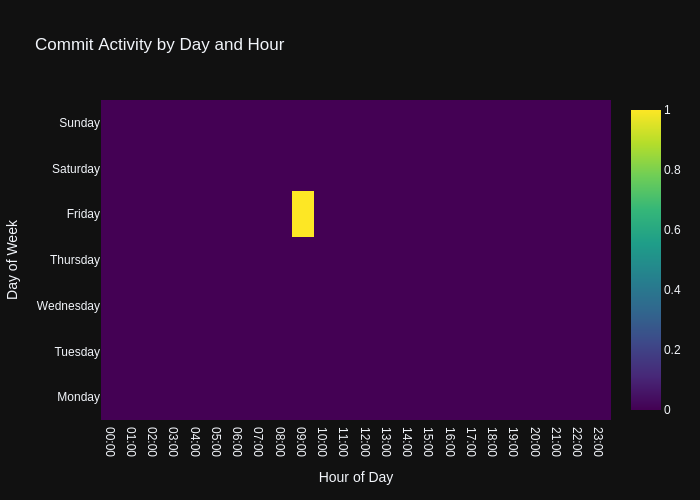
### Code Frequency
This chart shows the frequency of code changes over time:
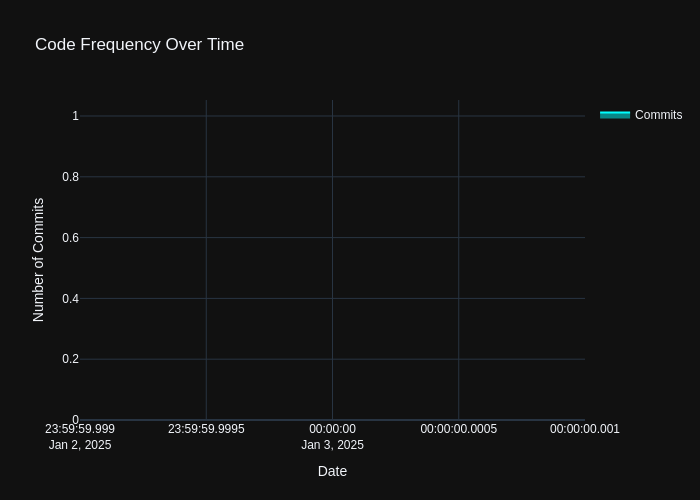
* Repository URL: [https://github.com/wanghaisheng/a-Reid-store](https://github.com/wanghaisheng/a-Reid-store)
* Stars: **0**
* Forks: **0**
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日