From Idea to Reality: Building a Cloudflare Native Web Starter Kit
Project Genesis
Unleashing Creativity with the Cloudflare Native Web Starter Kit
From Idea to Implementation
Journey from Concept to Code: Building the Cloudflare Native and Web Starter Kit
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Cloudflare Workers: We opted for Cloudflare Workers as the backbone of our application due to their serverless nature and ability to run code at the edge. This choice ensures that our AI processing is fast and efficient, providing a seamless user experience.
-
tRPC for API Communication: The decision to use tRPC was driven by the need for type-safe API communication. This choice enhances developer productivity by reducing runtime errors and improving the overall development experience.
-
D1 Database and R2 Storage: We selected D1 for its edge SQLite capabilities, which allow for quick data access and manipulation. R2 was chosen for asset storage due to its scalability and integration with Cloudflare’s ecosystem, making it easy to manage images and other assets generated by the application.
-
Clerk for Authentication: Security was a top priority, and Clerk was chosen for user management due to its robust authentication features and ease of integration. This decision ensures that user data is handled securely while providing a smooth onboarding experience.
3. Alternative Approaches Considered
-
Other Serverless Platforms: We evaluated other serverless platforms like AWS Lambda and Google Cloud Functions. However, the edge computing capabilities of Cloudflare Workers stood out, particularly for applications requiring low-latency responses.
-
Different Database Solutions: Initially, we considered using traditional SQL databases or NoSQL solutions. However, the lightweight nature of D1 and its integration with Cloudflare Workers made it a more appealing choice for our needs.
-
Frameworks for Mobile Development: We looked into various mobile frameworks, including React Native and Flutter. Ultimately, we chose Expo for its ease of use and ability to streamline the development process for cross-platform applications.
4. Key Insights That Shaped the Project
-
User-Centric Design: Understanding the end-user experience was crucial. We focused on creating a seamless interaction between the mobile app and the web landing page, ensuring that users could easily navigate and engage with the AI-generated content.
-
Scalability and Performance: The importance of scalability became evident as we designed the architecture. By leveraging Cloudflare’s edge network, we ensured that the application could handle varying loads without compromising performance.
-
Developer Experience: We recognized that a great developer experience is essential for adoption. By providing clear documentation, a well-structured project layout, and easy setup instructions, we aimed to lower the barrier to entry for developers looking to build on our starter kit.
-
Iterative Development: Emphasizing an iterative approach allowed us to refine features based on feedback and testing. This flexibility enabled us to adapt to challenges and improve the overall quality of the application.
Conclusion
Under the Hood
Technical Deep-Dive: Cloudflare Native and Web Starter Kit
1. Architecture Decisions
-
Microservices Approach: The application is structured into multiple microservices, each responsible for a specific functionality. This includes separate services for the API, mobile app, landing page, and workflows. This separation allows for independent development, testing, and deployment of each component.
-
Edge Computing: By utilizing Cloudflare Workers, the application processes requests at the edge, closer to the user. This reduces latency and improves performance, especially for AI tasks that require quick responses.
-
Type Safety with tRPC: The use of tRPC for API communication ensures type safety across the application. This minimizes runtime errors and enhances developer experience by providing autocompletion and type checking.
-
Durable Workflows: The application employs Cloudflare’s Durable Workflows for managing long-running tasks, such as AI processing. This allows for better handling of asynchronous operations and state management.
2. Key Technologies Used
-
Cloudflare Workers: A serverless platform that allows developers to run JavaScript code at the edge, enabling low-latency responses and efficient resource usage.
-
Wrangler CLI: A command-line tool for managing Cloudflare Workers projects, facilitating deployment, and configuration.
-
Expo: A framework for building cross-platform mobile applications using React Native, allowing for rapid development and deployment.
-
Astro: A static site generator that enables the creation of fast, modern web applications with a focus on performance.
-
Clerk: A user management solution that provides secure authentication and user management features.
-
tRPC: A TypeScript-first RPC framework that allows for type-safe API communication between the client and server.
-
R2 Storage: Cloudflare’s object storage solution for storing images and assets.
-
D1 Database: A serverless SQLite database that provides a lightweight and efficient data storage solution.
3. Interesting Implementation Details
API Service with tRPC
import { createRouter } from '@trpc/server';
import { z } from 'zod';
const appRouter = createRouter()
.query('getStory', {
input: z.string(),
resolve: async ({ input }) => {
// Fetch AI-generated story based on user input
const story = await generateStory(input);
return story;
},
});
export type AppRouter = typeof appRouter;
getStory
that takes a string input and returns an AI-generated story. The use of zod
for input validation ensures that the input adheres to the expected format.Durable Workflows for AI Processing
import { durable } from '@cloudflare/workers-types';
export const processAI = durable(async (input) => {
const story = await generateStory(input);
const image = await generateImage(story);
return { story, image };
});
4. Technical Challenges Overcome
Managing State in Durable Workflows
Authentication and User Management
const isAuthenticated = async ({ ctx }) => {
if (!ctx.user) {
throw new Error('Unauthorized');
}
};
// Usage in a protected route
const protectedRouter = createRouter()
.middleware(isAuthenticated)
.query('getUserData', {
resolve: async ({ ctx }) => {
return ctx.user.data;
},
});
getUserData
query.Deployment and Configuration Management
.env
files to store environment-specific variables and ensuring that the `Lessons from the Trenches
1. Key Technical Lessons Learned
-
Understanding Cloudflare Workers: The project provided a deep dive into serverless architecture using Cloudflare Workers. It was crucial to grasp how to deploy and manage serverless functions effectively, especially in terms of performance and scalability.
-
Type Safety with tRPC: Implementing a type-safe API using tRPC was a significant learning experience. It reinforced the importance of type safety in API communication, reducing runtime errors and improving developer experience.
-
Database Management with D1: Working with the D1 database and Drizzle ORM highlighted the importance of understanding edge databases. The nuances of managing data at the edge, including latency and data consistency, were critical to the app’s performance.
-
Authentication with Clerk: Integrating Clerk for user management emphasized the importance of secure authentication practices. It was a reminder of the complexities involved in user management and the need for robust security measures.
2. What Worked Well
-
Modular Project Structure: The clear separation of concerns in the project structure (apps, packages, tooling) made it easy to navigate and maintain. Each component had a specific purpose, which facilitated collaboration and code reuse.
-
Fast Development Cycle: Using
pnpm
for package management significantly sped up the installation and dependency resolution process. The ability to run the app locally withpnpm dev
allowed for rapid iteration and testing. -
Comprehensive Documentation: The README provided clear and detailed instructions for setup and deployment. This was invaluable for onboarding new developers and ensuring consistency in the development process.
-
Integration of Multiple Technologies: The combination of Expo for mobile, Astro for the landing page, and Cloudflare Workers for backend processing showcased a modern tech stack that is both powerful and flexible.
3. What You’d Do Differently
-
Enhanced Error Handling: While the project had a solid foundation, implementing more robust error handling and logging mechanisms would improve the debugging process. This could include centralized logging for Cloudflare Workers to track issues in production.
-
Automated Testing: Incorporating automated testing (unit and integration tests) from the beginning would have been beneficial. This would ensure that changes do not introduce new bugs and maintain the integrity of the application.
-
Environment Configuration Management: Instead of manually creating
.env
files, using a configuration management tool or library could streamline the process of managing environment variables, especially in larger teams. -
Performance Monitoring: Setting up performance monitoring tools early in the development process would help identify bottlenecks and optimize the application before deployment.
4. Advice for Others
-
Start Small: If you’re new to serverless architecture or any of the technologies used, start with a small feature or component. This allows you to learn and experiment without being overwhelmed.
-
Leverage Community Resources: Utilize community forums, documentation, and tutorials for Cloudflare Workers, tRPC, and other technologies. The community can provide valuable insights and solutions to common problems.
-
Focus on Security: Always prioritize security, especially when dealing with user authentication and data management. Regularly review security practices and stay updated on best practices.
-
Iterate Based on Feedback: After deploying the initial version, gather user feedback and iterate on the application. Continuous improvement based on real user experiences is key to building a successful application.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
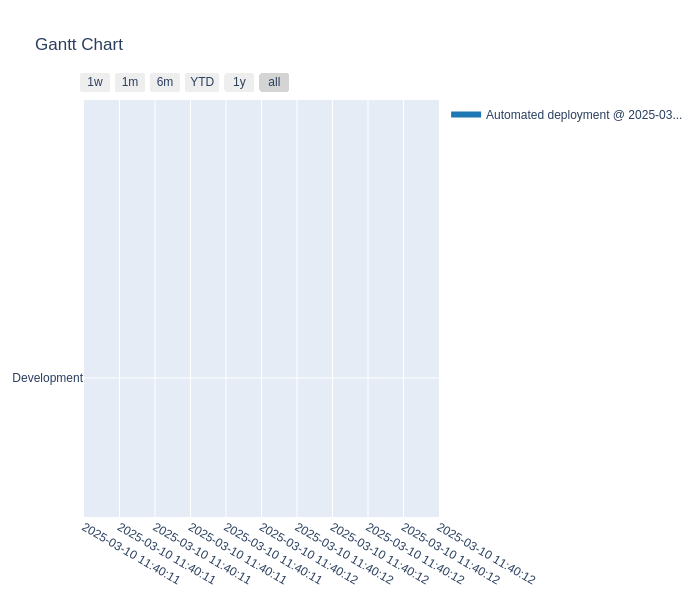
Commit Activity Heatmap
Contributor Network
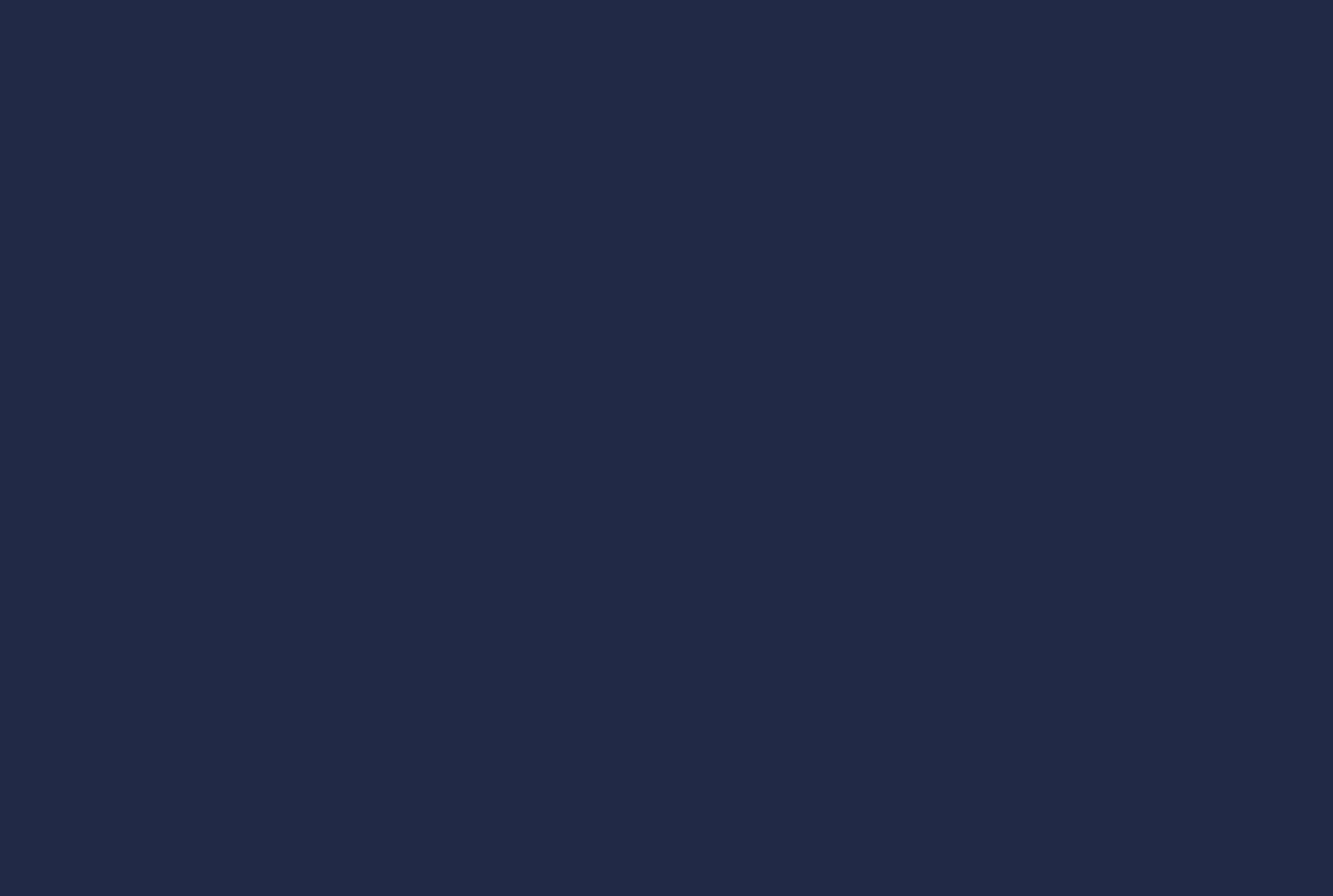
Commit Activity Patterns
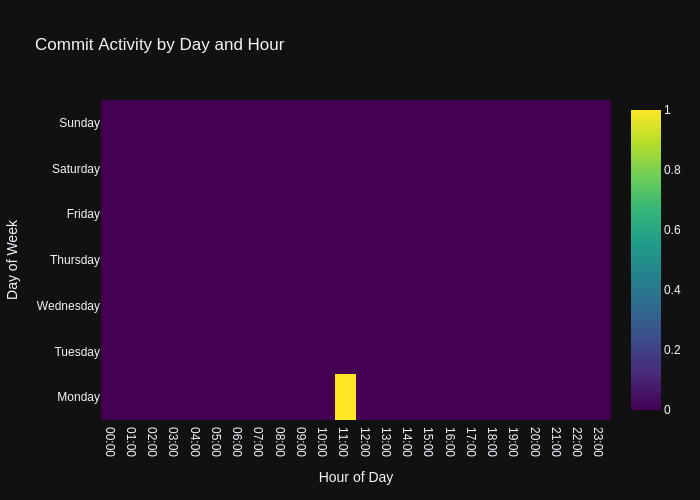
Code Frequency
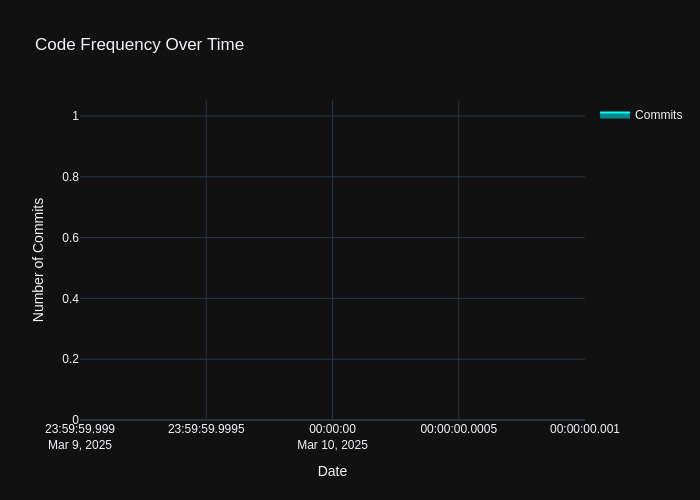
- Repository URL: https://github.com/wanghaisheng/a-cloudflare-native-web-starter-kit
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 3 月 17 日