Building AigoTools: Crafting a Dynamic Website Directory with SEO & Auto-Crawling
Project Genesis
Unleashing the Power of AigoTools: My Journey into Website Management
From Idea to Implementation
Initial Research and Planning
Technical Decisions and Their Rationale
docker-compose
, allowing for seamless management of multiple services like MongoDB and Redis.Alternative Approaches Considered
Key Insights That Shaped the Project
-
User-Centric Design: The importance of a user-friendly interface became evident early on. The team prioritized creating an intuitive design that would allow users to easily navigate the tool and manage their sites without a steep learning curve.
-
Flexibility and Customization: Users expressed a desire for customizable features that could cater to different needs. This feedback led to the inclusion of dark/light theme toggles and multiple image storage solutions, allowing users to tailor the tool to their preferences.
-
Community Engagement: The decision to open-source the design drafts and encourage community contributions was driven by the belief that collaboration could enhance the project. This approach not only fosters a sense of community but also allows for continuous improvement through user feedback and contributions.
-
Focus on Performance: The need for a fast and responsive application was a recurring theme in user feedback. This insight guided the team to implement performance optimization strategies, such as using caching and efficient data retrieval methods.
Under the Hood
Technical Deep-Dive: AigoTools
1. Architecture Decisions
- Main Navigation Site (
packages/aigotools
): This is the core of the application, responsible for rendering the user interface and managing site content. - Inclusion Service (
packages/crawler
): This service handles the automatic collection of site information using various APIs, such as OpenAI and Jina.
Deployment Strategy
2. Key Technologies Used
- Docker: For containerization, allowing easy deployment and management of dependencies.
- MongoDB: A NoSQL database used for storing site data and user information.
- Redis: An in-memory data structure store used for caching and session management.
- OpenAI API: Utilized for generating site content and enhancing user experience.
- Jina: A neural search framework that aids in site information collection.
- Clerk: A user management service that simplifies authentication and user management.
- pnpm: A fast, disk space-efficient package manager for JavaScript.
3. Interesting Implementation Details
Automatic Site Information Collection
const { OpenAI } = require('openai');
const openai = new OpenAI({ apiKey: process.env.OPENAI_API_KEY });
async function generateContent(prompt) {
const response = await openai.chat.completions.create({
model: 'gpt-3.5-turbo',
messages: [{ role: 'user', content: prompt }],
});
return response.choices[0].message.content;
}
User Management with Clerk
import { ClerkProvider, RedirectToSignIn } from '@clerk/clerk-react';
function App() {
return (
<ClerkProvider frontendApi={process.env.CLERK_FRONTEND_API}>
<RedirectToSignIn />
{/* Other components */}
</ClerkProvider>
);
}
Dark/Light Theme Toggle
:root {
--background-color: white;
--text-color: black;
}
[data-theme='dark'] {
--background-color: black;
--text-color: white;
}
function toggleTheme() {
const currentTheme = document.documentElement.getAttribute('data-theme');
document.documentElement.setAttribute('data-theme', currentTheme === 'dark' ? 'light' : 'dark');
}
4. Technical Challenges Overcome
Managing Multiple Image Storage Solutions
function getImageStorage() {
switch (process.env.IMAGE_STORAGE) {
case 'minio':
return new MinioClient();
case 'aws':
return new S3Client();
case 'tencent':
return new TencentClient();
default:
throw new Error('Invalid image storage option');
}
}
Environment Configuration Management
.env
files to manage these configurations, and the deployment scripts ensure that the correct environment variables are loaded based on the deployment context.# Copy environment variables for production
cp packages/aigotools/.env packages/aigotools/.env.prod
cp packages/crawler/.env packages/crawler/.env.prod
Ensuring SEO Optimization
function generateMetaTags(title, description) {
return `
<meta name="title" content="${title}">
<meta name="description" content="${description}">
## Lessons from the Trenches
Here are some key technical lessons learned, what worked well, what could be done differently, and advice for others based on the project history and README of AigoTools:
### Key Technical Lessons Learned
1. **Importance of Clear Documentation**: Comprehensive documentation is crucial for onboarding new users and contributors. The README provides a clear structure, making it easy to navigate through features, deployment instructions, and contribution guidelines.
2. **Environment Configuration Management**: Managing environment variables through `.env` files is essential for maintaining different configurations for development and production. This practice helps in avoiding hardcoding sensitive information and makes the application more secure.
3. **Containerization with Docker**: Using Docker for deployment simplifies the setup process and ensures consistency across different environments. It allows developers to run the application without worrying about system dependencies.
4. **Utilizing Third-Party Services**: Integrating services like Clerk for user management and OpenAI for site inclusion demonstrates the power of leveraging existing solutions to enhance functionality without reinventing the wheel.
### What Worked Well
1. **Feature-Rich Application**: The inclusion of features like internationalization, SEO optimization, and multiple image storage solutions adds significant value to the application, making it versatile for various user needs.
2. **User-Friendly Deployment Options**: Providing both local and hosting service deployment options caters to different user preferences and technical expertise, making it accessible to a wider audience.
3. **Community Engagement**: Encouraging contributions through clear guidelines and maintaining an open-source approach fosters community involvement, which can lead to improvements and new features.
4. **Design Resources**: Sharing Figma design drafts allows other developers to utilize the UI components, promoting collaboration and innovation within the community.
### What You'd Do Differently
1. **Enhanced Error Handling**: Implementing more robust error handling and logging mechanisms could improve the debugging process and user experience, especially during deployment.
2. **Automated Testing**: Incorporating automated testing (unit tests, integration tests) would help ensure code quality and reliability, making it easier to catch issues early in the development process.
3. **Performance Optimization**: Conducting performance profiling and optimization could enhance the application's responsiveness, especially when dealing with large datasets or high traffic.
4. **User Feedback Mechanism**: Establishing a more structured way to gather user feedback could provide valuable insights for future improvements and feature prioritization.
### Advice for Others
1. **Prioritize Documentation**: Invest time in creating and maintaining clear documentation. It pays off in the long run by reducing the onboarding time for new contributors and users.
2. **Embrace Community Contributions**: Be open to feedback and contributions from the community. This can lead to unexpected improvements and foster a sense of ownership among users.
3. **Focus on Security**: Always prioritize security best practices, especially when handling user data and integrating third-party services. Regularly review and update dependencies to mitigate vulnerabilities.
4. **Iterate Based on User Needs**: Continuously gather and analyze user feedback to guide development priorities. Building features that address real user pain points can significantly enhance the application's value.
By reflecting on these aspects, future projects can benefit from the experiences gained through AigoTools, leading to more successful and user-friendly applications.
## What's Next?
## Conclusion
As we wrap up this phase of the AigoTools project, we are excited to share our current status and future aspirations. AigoTools has successfully established itself as a robust platform for creating and managing website directories, complete with features like site management, automatic information collection, internationalization, and SEO optimization. Our deployment options, whether through local setups or hosting services like Zeabur, have made it accessible to a wide range of users.
Looking ahead, we have ambitious plans for further development. We aim to enhance our user management capabilities, expand our internationalization features, and integrate more advanced SEO tools. Additionally, we are exploring partnerships with other platforms to broaden our image storage solutions and improve overall performance. Your feedback and ideas are invaluable as we shape the future of AigoTools.
We invite all contributors—developers, designers, and users alike—to join us on this journey. Whether you want to report an issue, suggest a feature, or contribute code, your involvement can make a significant impact. Check out our [GitHub repository](https://github.com/someu/aigotools.git) to get started, and don’t hesitate to reach out with your thoughts or questions.
In closing, the journey of AigoTools has been a rewarding side project filled with learning and growth. We are grateful for the support from our community and excited about the possibilities that lie ahead. Together, we can continue to innovate and create a tool that empowers users to build their own navigation sites with ease. Thank you for being a part of this adventure!
## Project Development Analytics
### timeline gant
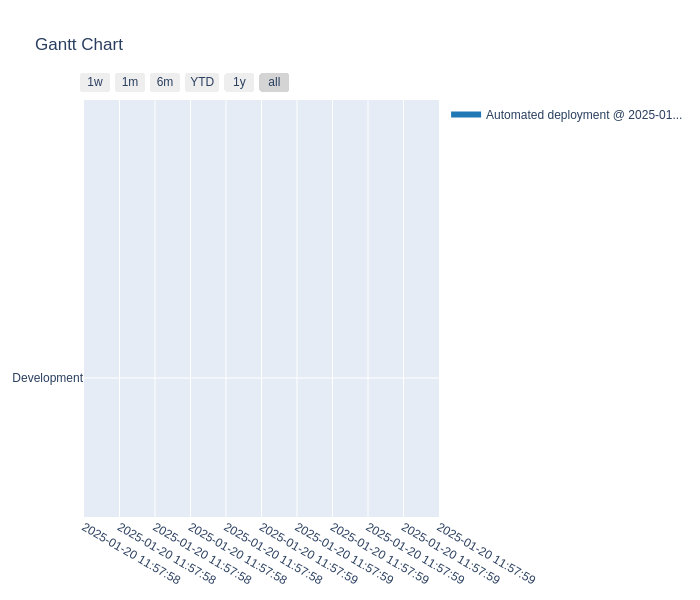
### Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
![Commit Heatmap]()
### Contributor Network
This network diagram shows how different contributors interact:
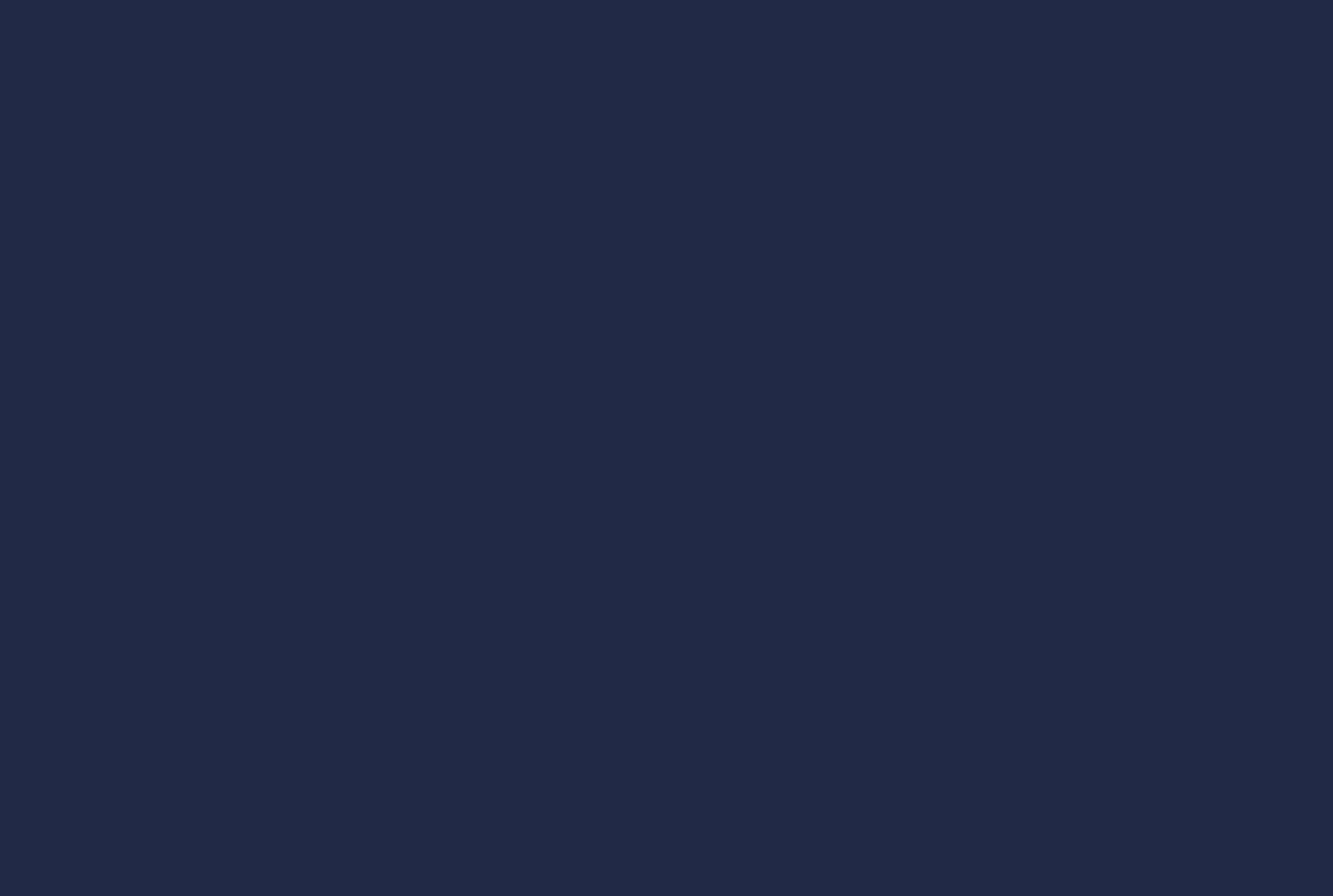
### Commit Activity Patterns
This chart shows when commits typically happen:
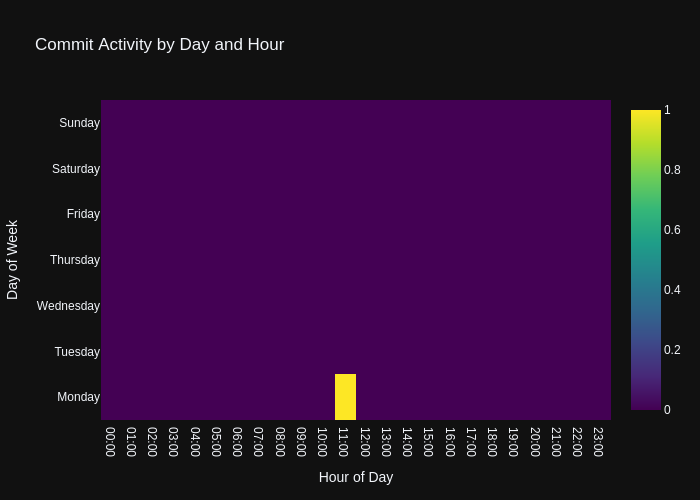
### Code Frequency
This chart shows the frequency of code changes over time:
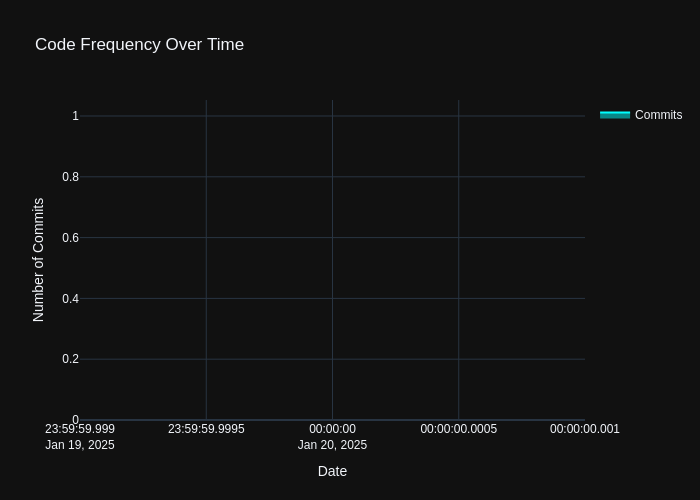
* Repository URL: [https://github.com/wanghaisheng/a-d-aigotools](https://github.com/wanghaisheng/a-d-aigotools)
* Stars: **0**
* Forks: **0**
编辑整理: Heisenberg 更新日期:2025 年 1 月 27 日