From Idea to Reality: Crafting the a-hono-astro-remix-template
Project Genesis
Unleashing the Power of Web Development: My Journey with the A-Hono-Astro-Remix-Template
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
Performance Matters: The importance of performance in web applications became evident early on. The decision to use SSR with Remix and static generation with Astro was driven by the need for fast load times and a better user experience.
-
Scalability is Key: As the project aimed to incorporate AI-driven features, the need for a scalable architecture became paramount. The choice of BullMQ for task management and Redis for caching highlighted the importance of building a system that could handle increased loads without compromising performance.
-
Developer Experience: The team recognized that a smooth developer experience is crucial for long-term project success. The modern features of Hono, along with the integration of TypeScript and BiomeJS for linting and formatting, contributed to a more enjoyable and efficient development process.
-
Flexibility and Modularity: The decision to use a modular approach with separate technologies for different aspects of the application (e.g., Astro for static content, Hono for the API) allowed for greater flexibility and easier maintenance. This insight reinforced the value of choosing the right tool for each specific task rather than relying on a single framework for everything.
Under the Hood
Technical Deep-Dive: Remix + Astro + Hono Boilerplate
1. Architecture Decisions
-
Separation of Concerns: The architecture separates the server-side and client-side technologies, allowing for a clear distinction between backend logic and frontend rendering. This separation facilitates easier maintenance and scalability.
-
Server-Side Rendering (SSR): Using Remix for SSR enhances performance and SEO capabilities. SSR allows for faster initial page loads and better indexing by search engines, which is crucial for web applications.
-
Static Site Generation (SSG): Astro is employed for static content, such as landing pages and documentation. This approach reduces server load and improves performance by serving pre-rendered HTML.
-
Task Queue with BullMQ: The decision to use BullMQ for background processing allows the application to handle long-running tasks efficiently. This is particularly important for applications that require processing large datasets or performing complex computations.
2. Key Technologies Used
-
Hono: A lightweight web framework for building APIs and web applications. It provides modern features like middleware support and OpenAPI documentation generation.
-
TypeScript: A superset of JavaScript that adds static typing, enhancing code quality and maintainability.
-
Postgres: A powerful relational database used for data storage, providing robust querying capabilities.
-
DrizzleORM: An ORM for TypeScript that simplifies database interactions with Postgres.
-
Redis: An in-memory data structure store used for caching and managing state, particularly for session management and task queues.
-
BullMQ: A task queue library that integrates with Redis, allowing for background job processing.
-
Astro: A static site generator that allows for building fast, content-focused websites.
-
TailwindCSS: A utility-first CSS framework that enables rapid UI development.
3. Interesting Implementation Details
Hono Middleware Example
import { Hono } from 'hono';
import { z } from 'zod';
const app = new Hono();
const userSchema = z.object({
name: z.string(),
email: z.string().email(),
});
app.post('/users', async (c) => {
const result = userSchema.safeParse(c.req.json());
if (!result.success) {
return c.json({ error: result.error.errors }, 400);
}
// Proceed with user creation
});
BullMQ Job Processing
import { Queue } from 'bullmq';
const queue = new Queue('emailQueue');
queue.add('sendEmail', { to: '[email protected]', subject: 'Welcome!' });
queue.process('sendEmail', async (job) => {
const { to, subject } = job.data;
// Logic to send email
});
Astro Static Content
---
title: "Welcome to Our App"
---
<Layout>
<Hero title="Your App Title" />
<Features />
<Pricing />
</Layout>
4. Technical Challenges Overcome
Integrating Multiple Frameworks
Managing State Across SSR and SSG
Background Job Management
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- Integration Complexity: Combining multiple frameworks (Remix, Astro, Hono) can lead to integration challenges. Each framework has its own conventions and lifecycle, which can complicate routing and state management. Understanding how they interact is crucial.
- State Management: Managing state across server-side rendered (SSR) and static content can be tricky. It’s important to establish a clear strategy for data fetching and state synchronization between the server and client.
- Task Queue Management: Implementing BullMQ for background tasks highlighted the importance of managing task queues effectively. Understanding how to configure job priorities, retries, and error handling is essential for building a robust application.
- Database Operations: Using DrizzleORM with Postgres required a solid understanding of both the ORM and the underlying database. Optimizing queries and understanding how to handle migrations effectively is key to maintaining performance.
- Email Handling: Integrating email services (like Resend or Nodemailer) requires careful consideration of user experience, especially for features like Magic Links and OTPs. Ensuring deliverability and managing user expectations around email timing is critical.
2. What Worked Well
- Hono as a Server Framework: Hono’s modern features, such as middleware support and OpenAPI documentation generation, made it easier to build a scalable backend. The runtime-agnostic nature allowed for flexibility in deployment.
- Astro for Static Content: Using Astro for static content management worked seamlessly. The ability to create a fast-loading landing page, blog, and documentation site without heavy lifting on the server side was a significant advantage.
- TailwindCSS and shadcn/ui: The combination of TailwindCSS and shadcn/ui provided a rapid development experience for building responsive and visually appealing UI components.
- Vite for Build System: Vite’s fast build times and hot module replacement significantly improved the development experience, allowing for quick iterations and testing.
3. What You’d Do Differently
- Documentation and Onboarding: Invest more time in creating comprehensive documentation for the project. Clear onboarding guides for new developers can help reduce the learning curve associated with the various technologies used.
- SEO Features: Prioritize the implementation of SEO features earlier in the project. This would ensure that the application is optimized for search engines from the start, rather than as an afterthought.
- Testing Strategy: Establish a more robust testing strategy, including unit tests and integration tests, especially for critical components like authentication and background job processing. This would help catch issues early in the development cycle.
- Error Handling: Implement a more comprehensive error handling strategy across the application. This includes both server-side and client-side error management to improve user experience and debugging.
4. Advice for Others
- Start Small: If you’re new to combining multiple frameworks, start with a smaller project to understand how they interact before scaling up to a full application. This will help you identify potential pitfalls early.
- Leverage Community Resources: Utilize community resources, such as forums, documentation, and example projects, to learn best practices and common patterns for the frameworks you are using.
- Focus on Performance: Always keep performance in mind, especially when dealing with SSR and static content. Optimize your database queries, minimize bundle sizes, and leverage caching strategies where possible.
- Iterate on Feedback: Regularly gather feedback from users and team members to identify pain points and areas for improvement. Iterative development based on real-world usage can lead to a more refined product.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
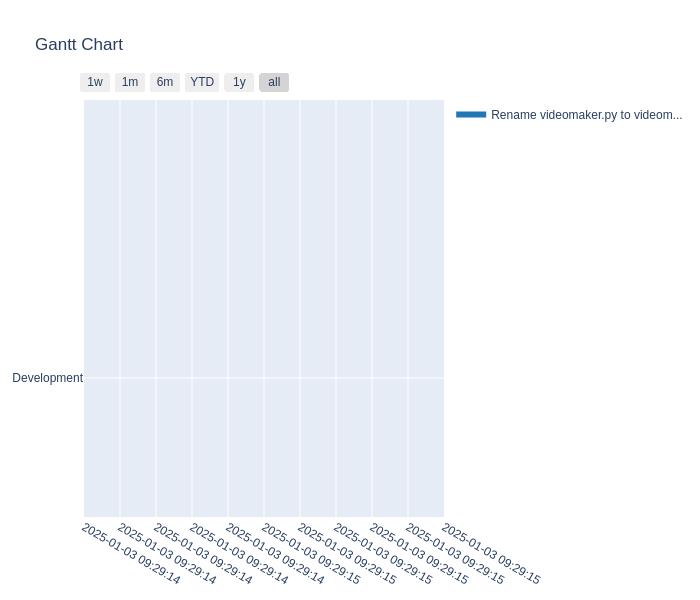
Commit Activity Heatmap
Contributor Network
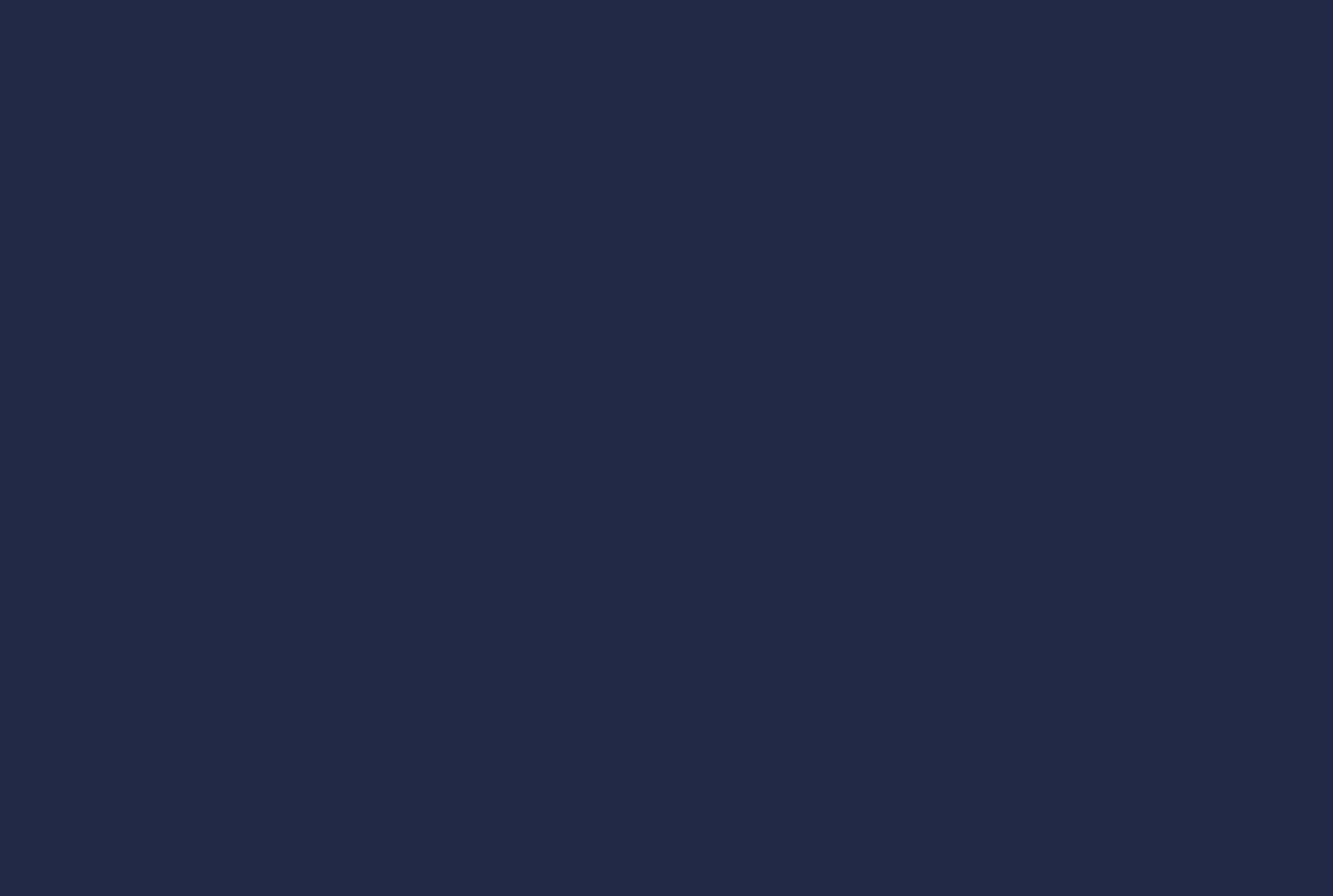
Commit Activity Patterns
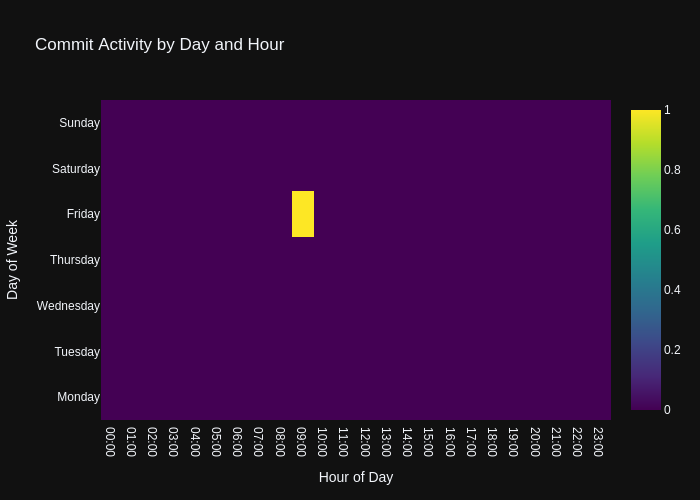
Code Frequency
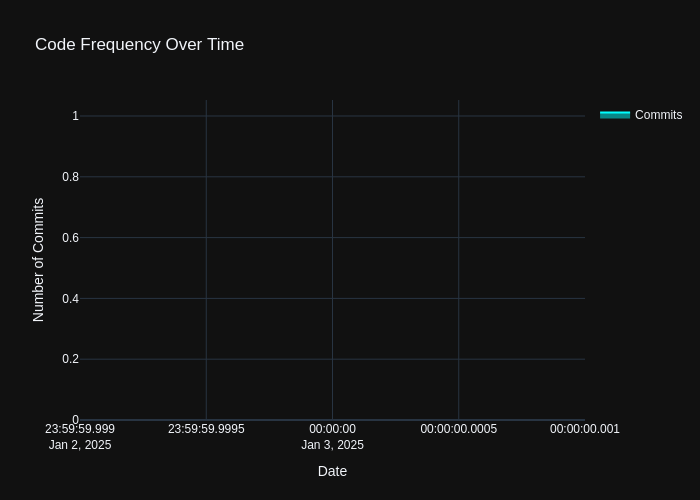
- Repository URL: https://github.com/wanghaisheng/a-hono-astro-remix-template
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日