From Idea to Reality: Building a-hub-movie-5star with Remix and NextUI
Project Genesis
Discovering the Magic of Movies: My Journey with A-Hub-Movie-5Star
From Idea to Implementation
1. Initial Research and Planning
- User Experience (UX): Understanding how users navigate similar applications and what features they find most valuable.
- Content Variety: Recognizing the demand for a diverse range of media, including movies, TV shows, and anime.
- Performance and Responsiveness: Ensuring that the app would perform well across devices, particularly mobile, given the increasing trend of mobile media consumption.
2. Technical Decisions and Their Rationale
-
Remix with TypeScript: Remix was selected for its ability to create fast, dynamic web applications with a focus on user experience. TypeScript was chosen to enhance code quality and maintainability through static typing.
-
NextUI: This library provided pre-made components that accelerated development while ensuring a consistent design language throughout the app.
-
TailwindCSS and Stitches: These tools were chosen for styling due to their flexibility and ease of use, allowing for rapid prototyping and customization.
-
Supabase for Authentication and Database: Supabase was selected for its simplicity and powerful features, enabling quick setup of authentication and real-time database capabilities.
-
Framer Motion for Animation: This library was integrated to enhance user engagement through smooth animations, making the app feel more dynamic and responsive.
-
Zustand for State Management: Zustand was chosen for its minimalistic approach to state management, which suited the app’s needs without adding unnecessary complexity.
3. Alternative Approaches Considered
-
Framework Alternatives: While Remix was ultimately chosen, other frameworks like Next.js and Vue.js were evaluated. Remix’s focus on user experience and data loading patterns aligned better with the project goals.
-
Styling Solutions: Other CSS frameworks like Bootstrap and Material-UI were considered. However, TailwindCSS’s utility-first approach offered greater flexibility for custom designs.
-
Database Options: Firebase was initially considered for backend services, but Supabase’s open-source nature and SQL-based database made it a more appealing choice for developers familiar with relational databases.
4. Key Insights That Shaped the Project
-
User-Centric Design: Continuous user feedback highlighted the importance of intuitive navigation and a clean interface. This reinforced the decision to prioritize UX in every aspect of the design.
-
Performance Matters: Early testing revealed that users are sensitive to load times and responsiveness. This insight drove the choice of technologies that optimize performance, such as Remix and LRU Cache for efficient data handling.
-
Community and Collaboration: Engaging with developer communities and forums provided valuable insights and best practices, which helped refine technical decisions and avoid common pitfalls.
-
Iterative Development: Emphasizing an iterative approach allowed for flexibility in adapting features based on user feedback and testing results, ensuring that the final product met user expectations.
Under the Hood
Technical Deep-Dive: SORA Web App
1. Architecture Decisions
Key Architectural Choices:
- Server-Side Rendering (SSR): By leveraging Remix, the app benefits from SSR, which improves SEO and initial load performance. This is particularly important for a media application where discoverability is key.
- Component-Based Architecture: Utilizing NextUI and Radix UI for UI components allows for a consistent design language and rapid development. This modular approach enables developers to create reusable components that can be easily maintained and updated.
- State Management: The use of Zustand for state management simplifies the handling of global state across the application, making it easier to manage user authentication states, media playback states, and user preferences.
2. Key Technologies Used
- Remix: A framework for building web applications that focuses on performance and user experience.
- NextUI: A React UI library that provides pre-made components, allowing for rapid UI development.
- TailwindCSS: A utility-first CSS framework that enables custom styling without leaving the HTML.
- Supabase: An open-source Firebase alternative that provides authentication and database services.
- Framer Motion: A library for animations that enhances the user interface with smooth transitions and effects.
- Artplayer: A custom media player that allows for a tailored video playback experience.
Example of Component Usage:
import { Button } from 'nextui';
const PlayButton = () => {
return (
<Button color="primary" onClick={handlePlay}>
Play
</Button>
);
};
3. Interesting Implementation Details
Internationalization (i18n)
Custom Media Player
Example of Media Player Integration:
import Artplayer from 'artplayer';
const MediaPlayer = () => {
const player = new Artplayer({
container: '#player',
url: 'video-url.mp4',
// Additional configurations
});
return <div id="player"></div>;
};
4. Technical Challenges Overcome
Performance Optimization
Authentication Flow
Example of Authentication Handling:
import { createClient } from '@supabase/supabase-js';
const supabase = createClient('your-supabase-url', 'your-anon-key');
const signIn = async (email, password) => {
const { user, error } = await supabase.auth.signIn({ email, password });
if (error) console.error('Error signing in:', error);
return user;
};
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- Framework Synergy: Using both Remix and NextUI allowed for a seamless integration of server-side rendering and pre-made UI components. This combination enhanced the performance and user experience of the app.
- State Management: Implementing Zustand for state management proved to be efficient and lightweight, making it easier to manage global state without the boilerplate often associated with other state management libraries.
- Internationalization: Integrating i18n for internationalization was crucial for reaching a broader audience. It highlighted the importance of planning for localization from the start of the project.
- Custom Media Player: Building a custom media player with Artplayer provided flexibility in design and functionality, but it also required a deeper understanding of media handling in web applications.
2. What Worked Well
- Component Libraries: Utilizing NextUI and Radix UI for pre-made components significantly sped up the development process. The availability of customizable components allowed for a consistent design language throughout the app.
- Responsive Design: TailwindCSS facilitated rapid styling and responsiveness, making it easier to create a mobile-friendly interface.
- Development Workflow: The use of
pnpm
for dependency management improved installation speed and reduced disk space usage, which streamlined the development workflow.
3. What You’d Do Differently
- Documentation: While the README provides a good overview, more detailed documentation on setting up the environment and using specific features would be beneficial for new contributors or users.
- Testing: Implementing a more robust testing strategy from the beginning, including unit tests and end-to-end tests, would help catch bugs earlier and improve code quality.
- Performance Optimization: Although the app is performant, future iterations could benefit from profiling and optimizing specific components, especially those involving animations and media playback.
4. Advice for Others
- Start with a Clear Plan: Before diving into development, outline the core features and tech stack. This helps in making informed decisions and reduces scope creep.
- Iterate and Gather Feedback: Regularly seek feedback from users and stakeholders during development. This can guide feature prioritization and improve user satisfaction.
- Embrace Modular Design: Build components in a modular way to promote reusability and maintainability. This approach can save time in the long run and make it easier to implement changes.
- Stay Updated: The tech landscape evolves rapidly. Keep an eye on updates to the libraries and frameworks you use, as they often introduce performance improvements and new features that can enhance your project.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
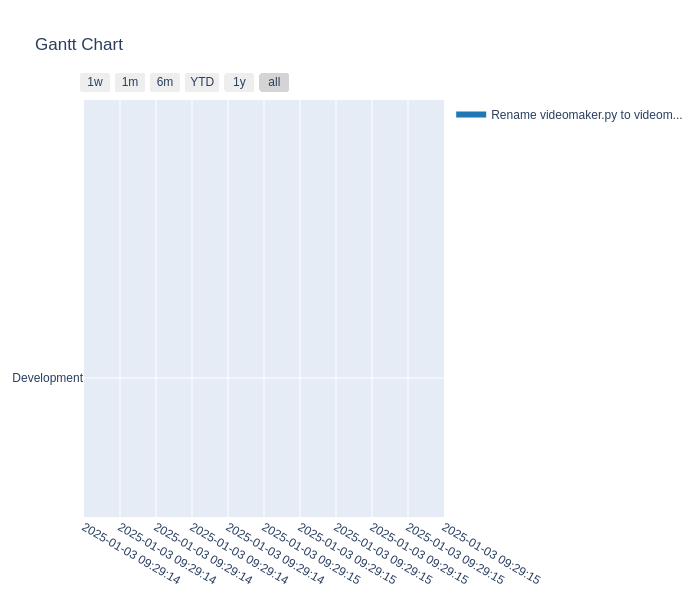
Commit Activity Heatmap
Contributor Network
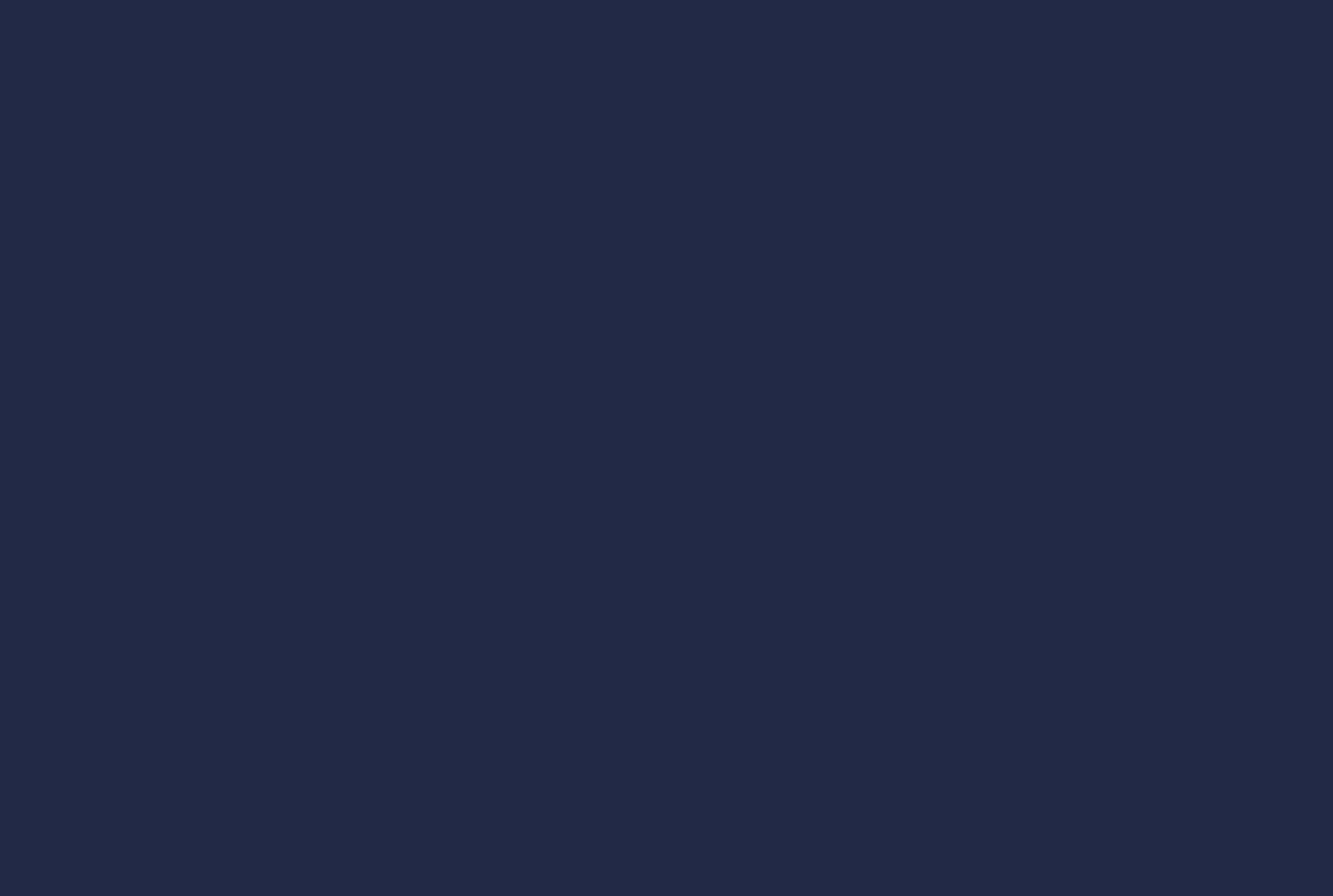
Commit Activity Patterns
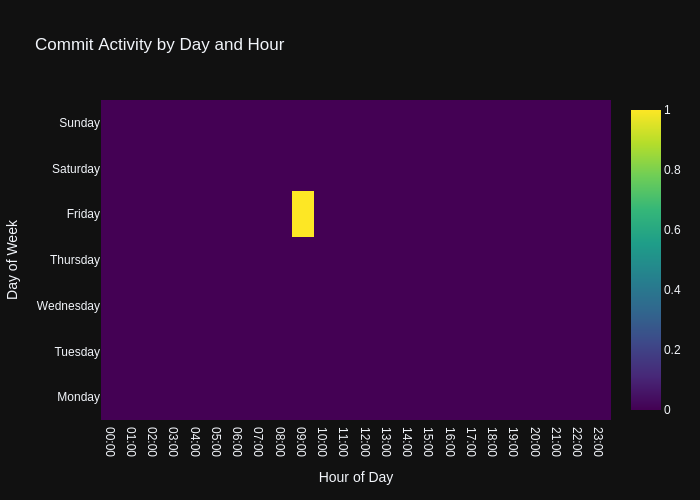
Code Frequency
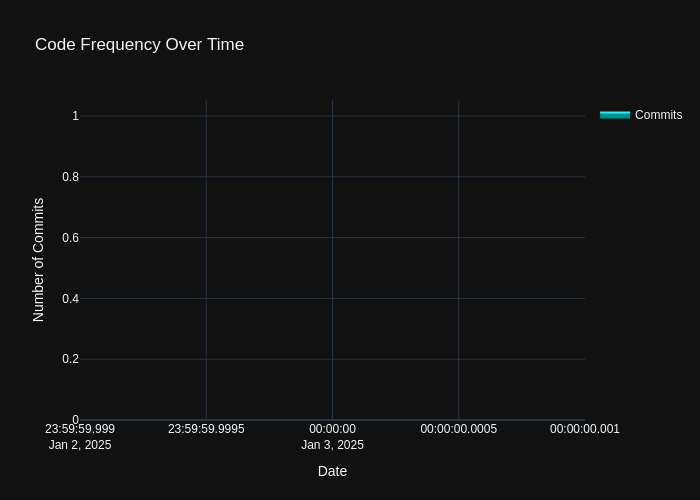
- Repository URL: https://github.com/wanghaisheng/a-hub-movie-5star
- Stars: 1
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日