Building a-next-flux-saas: Crafting a Seamless SaaS Experience with Next.js
Project Genesis
Introduction
From Idea to Implementation
Journey from Concept to Code: Next Money Stripe Starter
1. Initial Research and Planning
- Market Demand: There was a growing need for SaaS solutions that could be quickly deployed and easily customized. This was particularly evident in the rise of startups and small businesses looking to leverage technology without extensive resources.
- Technology Stack: The selection of technologies was crucial. Next.js was chosen for its performance and developer experience, while Prisma and Supabase were selected for their robust database management capabilities. Clerk Auth was included for user management, and Stripe was integrated for payment processing, which is essential for any SaaS application.
2. Technical Decisions and Their Rationale
-
Next.js: Chosen for its server-side rendering capabilities and static site generation, which enhance performance and SEO. Its component-based architecture aligns well with React, making it a natural choice for building interactive UIs.
-
Prisma: Selected as the ORM for its TypeScript-first approach, which ensures type safety and improves developer productivity. It simplifies database interactions and migrations, making it easier to manage data models.
-
Supabase: This platform was integrated for its serverless Postgres database, which offers scalability and a generous free tier. It allows developers to focus on building features rather than managing infrastructure.
-
Clerk Auth: Implemented for user authentication, providing a comprehensive solution for managing user identities and sessions without the need to build a custom solution from scratch.
-
Stripe: Integrated for payment processing, as it is widely recognized and trusted in the industry, providing a seamless experience for handling subscriptions and transactions.
3. Alternative Approaches Considered
-
Using a Monolithic Framework: Initially, there was consideration of using a monolithic framework like Ruby on Rails or Django. However, the decision was made to go with a microservices architecture using Next.js to allow for greater flexibility and scalability.
-
Different Authentication Solutions: Other authentication solutions were evaluated, such as Firebase Authentication and Auth0. Ultimately, Clerk Auth was chosen for its ease of integration and comprehensive feature set tailored for SaaS applications.
-
Email Handling: Various email services were considered for handling notifications and user communications. Resend was selected for its powerful capabilities and seamless integration with React Email, which simplifies email development.
4. Key Insights That Shaped the Project
-
Developer Experience Matters: A focus on providing a smooth developer experience was paramount. This included clear documentation, easy setup, and a well-structured codebase. The use of TypeScript, Prettier, and ESLint helped maintain code quality and consistency.
-
Community and Collaboration: The importance of community-driven development became evident. Drawing inspiration from existing projects like Shadcn’s Taxonomy and Steven Tey’s Precedent highlighted the value of collaboration and learning from others in the open-source community.
-
Iterative Development: Emphasizing an iterative approach allowed for continuous improvement based on user feedback and testing. This flexibility enabled the team to adapt to changing requirements and incorporate new features as needed.
-
Focus on Performance: Performance optimization was a recurring theme, leading to the integration of tools like Vercel Analytics for tracking user engagement and ensuring that the application remains responsive and efficient.
Under the Hood
Technical Deep-Dive: Next Money Stripe Starter
1. Architecture Decisions
Key Architectural Choices:
- Microservices Approach: By leveraging services like Supabase for database management and Clerk for authentication, the architecture adheres to a microservices approach, allowing each component to be developed, deployed, and scaled independently.
- Separation of Concerns: The use of different libraries for UI (Tailwind CSS, Shadcn/ui) and email handling (React Email, Resend) ensures that each aspect of the application is handled by the best-suited tool, promoting a clean separation of concerns.
2. Key Technologies Used
- Next.js: A React framework that enables SSR and SSG, enhancing performance and user experience.
- Prisma: A TypeScript-first ORM that simplifies database interactions and provides type safety.
- Supabase: A backend-as-a-service platform that offers a Postgres database, authentication, and real-time capabilities.
- Clerk Auth: A user management platform that simplifies authentication and user management.
- Resend: A service for sending emails, integrated with React Email for seamless email handling.
- Tailwind CSS: A utility-first CSS framework that allows for rapid UI development with a focus on responsiveness and customization.
Example of Integration:
import { ClerkProvider, RedirectToSignIn } from '@clerk/nextjs';
function MyApp({ Component, pageProps }) {
return (
<ClerkProvider>
<Component {...pageProps} />
</ClerkProvider>
);
}
3. Interesting Implementation Details
Environment Configuration
.env.local
file to manage environment variables, which is crucial for keeping sensitive information secure. The setup process includes copying a template file and updating it with specific configurations.Email Handling
.react-email
folder to avoid common errors, showcasing the importance of keeping dependencies up to date.Custom Hooks
- useIntersectionObserver: This hook can be used to trigger animations or load content when elements enter the viewport.
- useLocalStorage: A utility for persisting data in the browser’s local storage, which can be useful for maintaining user preferences.
Example of a Custom Hook:
import { useEffect, useState } from 'react';
function useLocalStorage(key, initialValue) {
const [storedValue, setStoredValue] = useState(() => {
try {
const item = window.localStorage.getItem(key);
return item ? JSON.parse(item) : initialValue;
} catch (error) {
console.error(error);
return initialValue;
}
});
useEffect(() => {
try {
window.localStorage.setItem(key, JSON.stringify(storedValue));
} catch (error) {
console.error(error);
}
}, [key, storedValue]);
return [storedValue, setStoredValue];
}
4. Technical Challenges Overcome
Dependency Management
pnpm
for package management. The project includes a note on using npm-check-updates
to keep dependencies current, which is crucial for security and performance.Email Rendering Issues
renderToReadableStream not found
). This proactive approach helps users troubleshoot problems effectively.Performance Optimization
next/font
for font optimization and ImageResponse
for generating dynamic Open Graph images demonstrates a focus on performance. These optimizations are essential for improving load times and enhancing the user experience.Example of Image Optimization:
import Image from 'next/image';
function MyComponent() {
return (
<Image
src="/path/to/image.jpg"
alt="Description"
width={500}
height={300}
priority
/>
);
}
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Integration of Multiple Technologies: The project successfully integrates various technologies such as Next.js, Prisma, Supabase, and Clerk Auth. Understanding how to effectively combine these tools was crucial for building a robust SaaS application. Each technology has its strengths, and leveraging them together can significantly enhance the development process.
-
Environment Configuration: Managing environment variables through
.env.local
is essential for maintaining different configurations for development and production. This practice helps in keeping sensitive information secure and allows for easy updates without altering the codebase. -
Email Handling: Implementing email functionality using React Email and Resend highlighted the importance of having a reliable email service in SaaS applications. Understanding how to set up and troubleshoot email services is vital for user communication.
-
State Management and Hooks: Utilizing custom hooks like
useLocalStorage
anduseScroll
improved the application’s performance and user experience. Learning how to create and manage custom hooks can lead to cleaner and more maintainable code.
What Worked Well
-
Rapid Development with Next.js: The use of Next.js allowed for quick setup and development of the application. Its built-in features like server-side rendering and static site generation contributed to better performance and SEO.
-
UI Development with Tailwind CSS: Tailwind CSS facilitated rapid UI development with its utility-first approach. This made it easy to create responsive designs without writing extensive custom CSS.
-
Code Quality Tools: Implementing TypeScript, Prettier, and ESLint helped maintain high code quality and consistency throughout the project. These tools caught errors early and enforced coding standards, making collaboration easier.
-
Documentation and Community Resources: The availability of comprehensive documentation for each technology used, along with community support, made it easier to troubleshoot issues and implement features.
What You’d Do Differently
-
More Comprehensive Testing: While the project focused on development speed, incorporating more automated testing (unit and integration tests) from the beginning would have improved reliability and reduced bugs in production.
-
User Feedback Loop: Establishing a feedback mechanism early in the development process could have provided valuable insights into user experience and feature requests, leading to a more user-centered product.
-
Performance Optimization: Although the project performed well, dedicating more time to performance optimization techniques (like code splitting and lazy loading) could enhance the user experience, especially as the application scales.
Advice for Others
-
Start Small and Iterate: Begin with a minimal viable product (MVP) and gradually add features based on user feedback. This approach helps in managing scope and ensures that the core functionalities are solid before expanding.
-
Leverage Existing Solutions: Don’t reinvent the wheel. Use existing libraries and frameworks that solve common problems. This can save time and effort, allowing you to focus on unique aspects of your application.
-
Stay Updated: The tech landscape is constantly evolving. Keep an eye on updates and new features in the tools you use, as they can significantly improve your development process and application performance.
-
Engage with the Community: Participate in forums, GitHub discussions, and social media groups related to the technologies you are using. Engaging with the community can provide support, inspiration, and collaboration opportunities.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
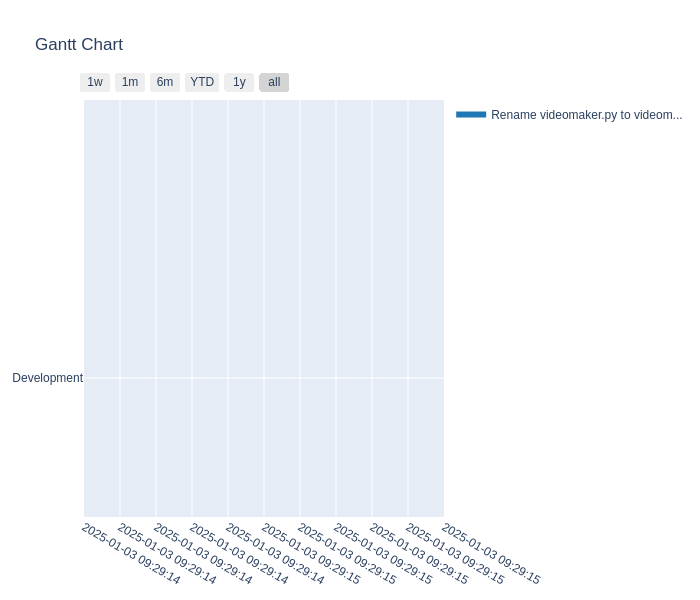
Commit Activity Heatmap
Contributor Network
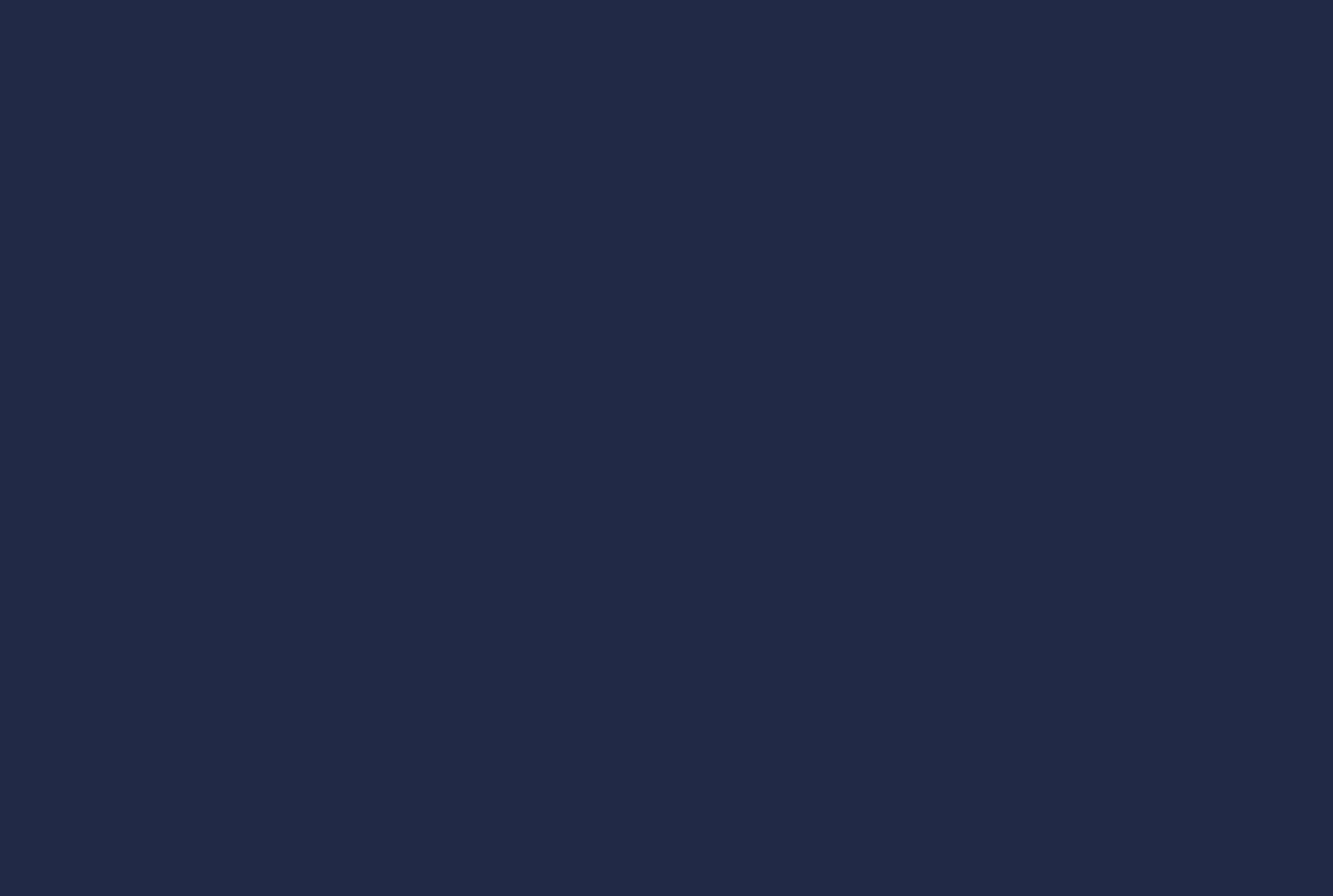
Commit Activity Patterns
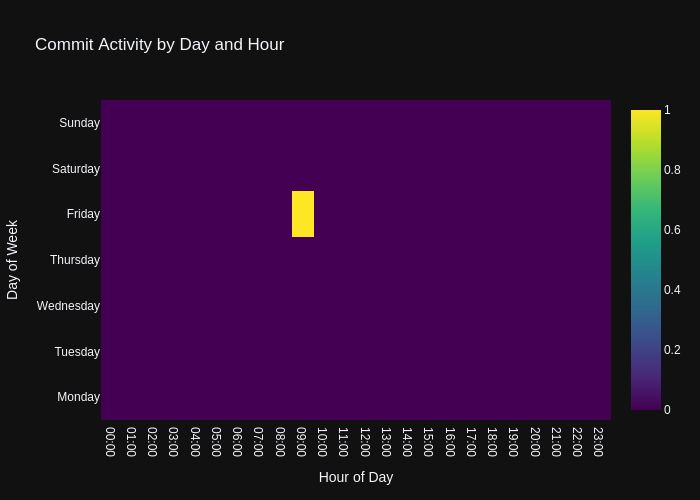
Code Frequency
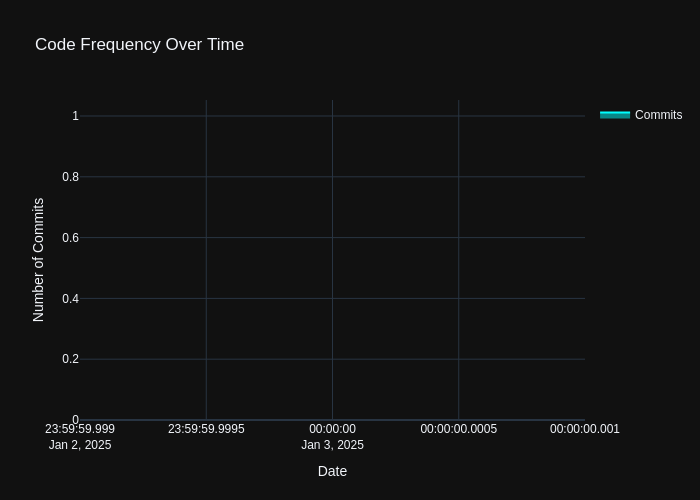
- Repository URL: https://github.com/wanghaisheng/a-next-flux-saas
- Stars: 1
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日