Building PopShop: Crafting an eCommerce Experience with ReactTS & Supabase
Project Genesis
🌟 PopShop 🌟
From Idea to Implementation
Under the Hood
Technical Deep-Dive: PopShop eCommerce Website
1. Architecture Decisions
-
Frontend Framework: React with TypeScript was chosen for the frontend to provide a robust and type-safe development experience. This allows for better maintainability and fewer runtime errors.
-
Styling: Daisy UI, a component library built on Tailwind CSS, was selected for its utility-first approach, enabling rapid UI development while maintaining a consistent design language.
-
Backend and Database: Supabase serves as the backend and database solution. It provides a real-time database, authentication, and storage, which simplifies the development process by offering a complete backend-as-a-service (BaaS) solution.
-
Deployment: The application is deployed using Vercel, which offers seamless integration with GitHub for continuous deployment, ensuring that the latest changes are automatically reflected in the production environment.
Example Architecture Diagram
+-------------------+ +-------------------+
| React Frontend | <--> | Supabase Backend |
| (TypeScript, Daisy UI) | | (Auth, Database) |
+-------------------+ +-------------------+
|
v
+-------------------+
| Vercel Hosting |
+-------------------+
2. Key Technologies Used
-
React: A JavaScript library for building user interfaces, allowing for the creation of reusable UI components.
-
TypeScript: A superset of JavaScript that adds static types, enhancing code quality and developer experience.
-
Daisy UI: A component library that extends Tailwind CSS, providing pre-designed components that can be easily customized.
-
Supabase: An open-source Firebase alternative that provides a real-time database, authentication, and storage solutions.
-
Tailwind CSS: A utility-first CSS framework that allows for rapid UI development with a focus on responsiveness and customization.
Example Code Snippet
import React from 'react';
interface ButtonProps {
label: string;
onClick: () => void;
}
const Button: React.FC<ButtonProps> = ({ label, onClick }) => {
return (
<button
className="btn btn-primary"
onClick={onClick}
>
{label}
</button>
);
};
export default Button;
3. Interesting Implementation Details
-
User Authentication: Supabase provides built-in authentication features, allowing users to sign up and log in easily. The integration is straightforward, using Supabase’s client library to handle authentication flows.
-
Real-time Features: Leveraging Supabase’s real-time capabilities, the application can update the UI in response to database changes without requiring a page refresh. This enhances the user experience, especially in a shopping context where inventory levels may change frequently.
-
Responsive Design: The use of Tailwind CSS and Daisy UI ensures that the application is fully responsive, providing a seamless experience across devices. The utility classes allow for quick adjustments to layout and styling.
Example of Real-time Data Fetching
import { useEffect, useState } from 'react';
import { supabase } from './supabaseClient';
const ProductsList = () => {
const [products, setProducts] = useState([]);
useEffect(() => {
const fetchProducts = async () => {
const { data } = await supabase.from('products').select('*');
setProducts(data);
};
fetchProducts();
const subscription = supabase
.from('products')
.on('INSERT', (payload) => {
setProducts((prev) => [...prev, payload.new]);
})
.subscribe();
return () => {
supabase.removeSubscription(subscription);
};
}, []);
return (
<div>
{products.map((product) => (
<div key={product.id}>{product.name}</div>
))}
</div>
);
};
4. Technical Challenges Overcome
-
State Management: Managing the state across various components was initially challenging. The team decided to use React’s Context API to provide a global state for user authentication and cart management, simplifying data flow and reducing prop drilling.
-
Deployment Issues: During the initial deployment phase, there were issues with environment variables not being set correctly in Vercel. The team resolved this by ensuring that all necessary environment variables were configured in the Vercel dashboard.
-
Cross-Origin Resource Sharing (CORS): When integrating Supabase, CORS issues arose when making API calls from the frontend. This was resolved by configuring the Supabase project settings to allow requests from the frontend domain.
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Integration of Technologies: Successfully integrating React with TypeScript and Supabase taught me the importance of choosing the right tech stack for scalability and maintainability. TypeScript’s type safety helped catch errors early in the development process, while Supabase provided a robust backend solution with real-time capabilities.
-
State Management: Managing state effectively in a React application is crucial. I learned to utilize React’s Context API and hooks to manage global state, which simplified data flow and improved component reusability.
-
Responsive Design: Implementing a responsive design using Daisy UI and TailwindCSS was a valuable lesson in creating user-friendly interfaces. I learned how utility-first CSS frameworks can speed up the styling process and ensure consistency across the application.
What Worked Well
-
Community Engagement: The open-source nature of PopShop fostered a collaborative environment. Contributors were eager to share ideas and improvements, which enhanced the overall quality of the project.
-
Documentation: Providing clear and comprehensive documentation (README, CONTRIBUTING.md, CODE_OF_CONDUCT.md) helped onboard new contributors quickly and ensured everyone was aligned with the project’s goals and standards.
-
Deployment Process: Utilizing Vercel for deployment streamlined the process, allowing for quick iterations and easy access to both production and development environments. This facilitated rapid testing and feedback.
What I’d Do Differently
-
Enhanced Testing: While the project included some basic testing, I would prioritize implementing a more comprehensive testing strategy, including unit tests and end-to-end tests, to ensure the reliability of features as the codebase grows.
-
User Feedback Loop: Establishing a more structured feedback loop with users could provide valuable insights into usability and feature requests. This could involve surveys or user testing sessions to gather direct input.
-
Performance Optimization: I would focus more on performance optimization from the start, such as code splitting and lazy loading components, to improve the application’s load time and overall user experience.
Advice for Others
-
Start Small: If you’re new to a tech stack, start with a small project to familiarize yourself with the tools and libraries. This will build your confidence and understanding before tackling larger projects.
-
Embrace Open Source: Contributing to open-source projects is a great way to learn and grow as a developer. Engage with the community, ask questions, and don’t hesitate to share your ideas.
-
Prioritize Documentation: Good documentation is essential for any project. It not only helps others understand your work but also serves as a reference for yourself in the future. Make it a habit to document as you go.
-
Stay Updated: The tech landscape is constantly evolving. Keep learning and stay updated with the latest trends and best practices in web development to enhance your skills and project quality.
What’s Next?
Conclusion: Looking Ahead for PopShop 🌟
Project Development Analytics
timeline gant
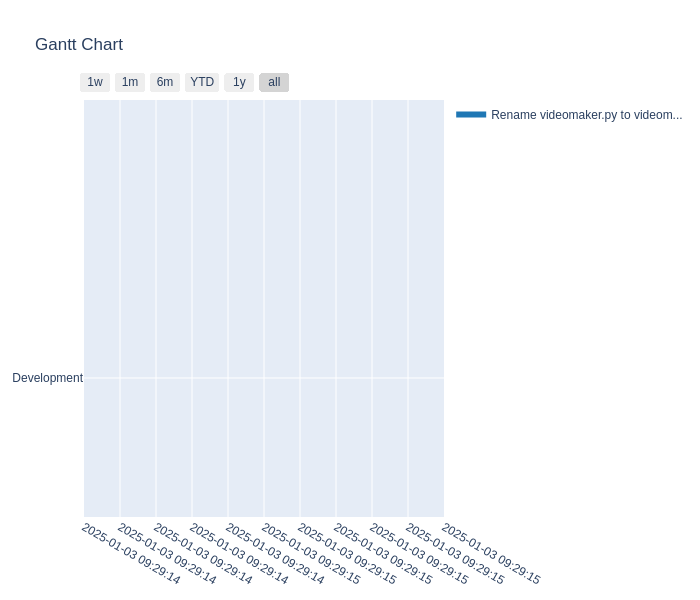
Commit Activity Heatmap
Contributor Network
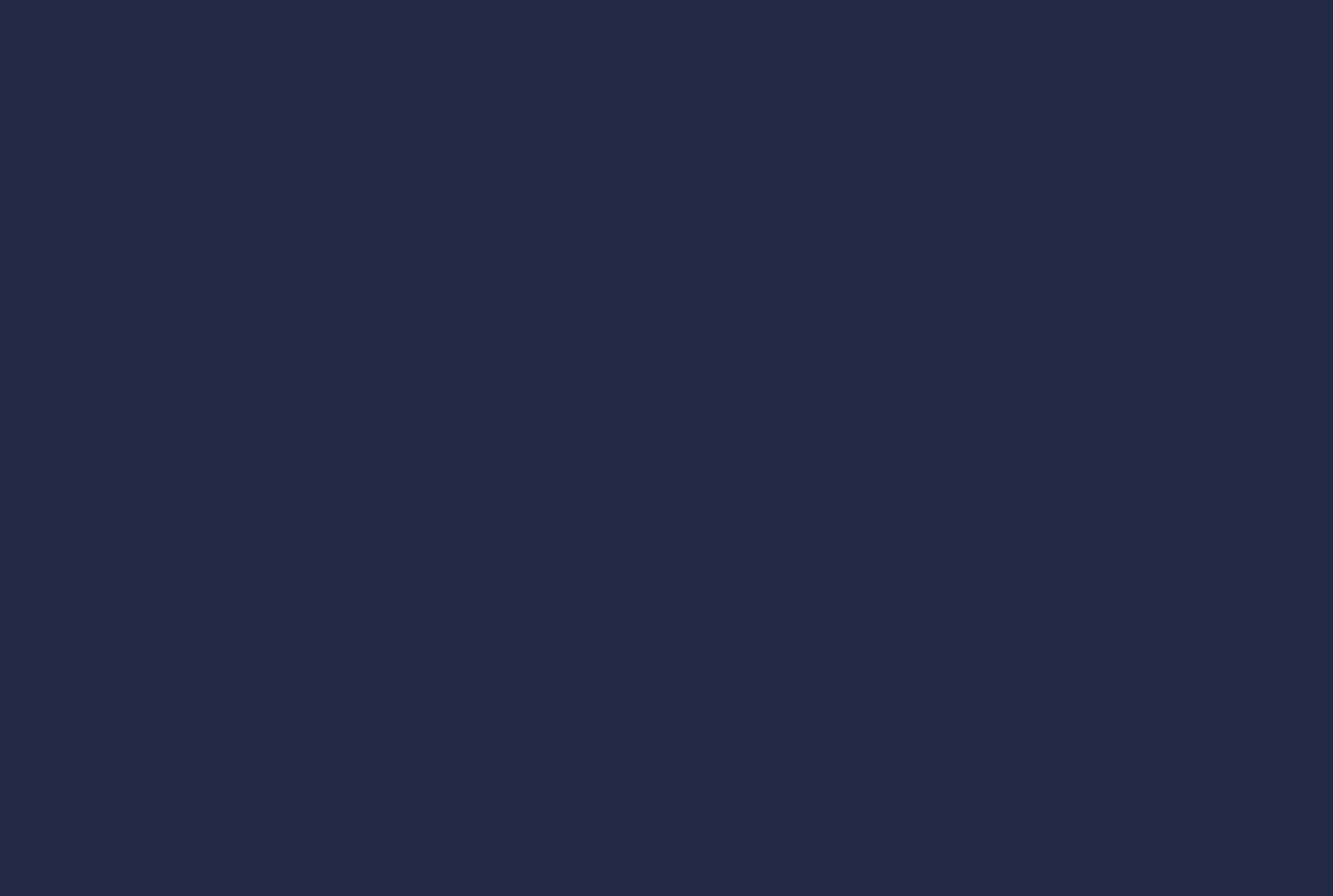
Commit Activity Patterns
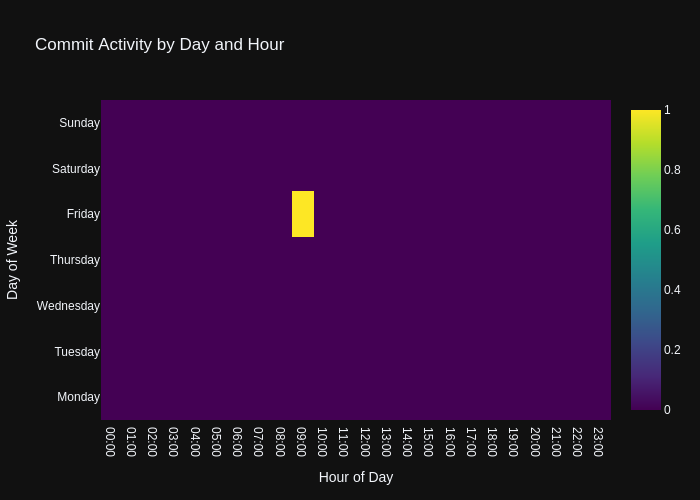
Code Frequency
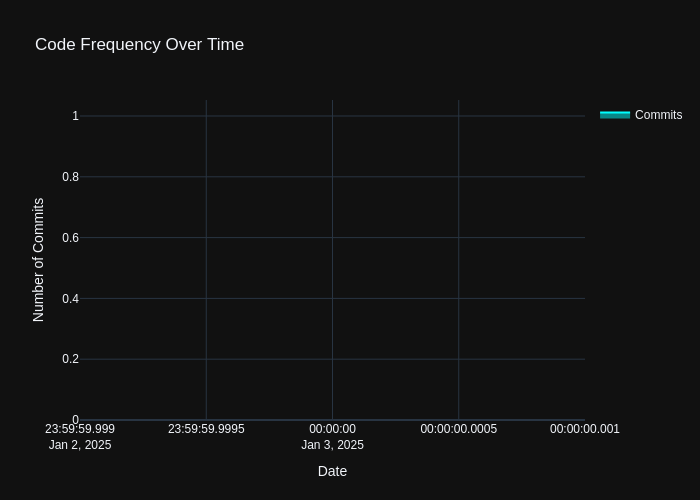
- Repository URL: https://github.com/wanghaisheng/a-pop-style-Shop
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日