From Idea to Reality: a-turn-paper-daily-track-to-blog-template
Project Genesis
Turning Research into Readable: My Journey from Paper to Blog
From Idea to Implementation
From Concept to Code: Building a Blog Generator for Research Papers
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
MkDocs
- Rationale: MkDocs is a static site generator that is easy to use and allows for quick deployment of documentation. Its Markdown support made it an ideal choice for converting research paper content into a structured format suitable for blogs.
Astro
- Rationale: Astro is a modern static site generator that allows for the creation of fast, optimized websites. Its component-based architecture enables us to build customizable themes, which is essential for users who want to personalize their blogs. Additionally, Astro’s ability to integrate with various front-end frameworks provided flexibility in design and functionality.
3. Alternative Approaches Considered
-
Custom CMS Development: Building a custom content management system from scratch was initially appealing due to its flexibility. However, the time and resources required for development and maintenance were significant drawbacks.
-
Existing Blogging Platforms: We explored existing platforms like WordPress and Medium. While they offer robust features, they lack the specific automation and customization we aimed to provide. Additionally, these platforms often come with limitations in terms of design and user control.
-
Natural Language Processing (NLP) Tools: We considered using NLP tools to summarize and generate content from research papers. While this could enhance automation, the complexity and potential inaccuracies in content generation led us to prioritize a more manual approach for the initial version.
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of user feedback became evident early on. Engaging with potential users helped us refine our features and prioritize functionality that would enhance their blogging experience.
-
Simplicity and Usability: We learned that while advanced features are appealing, simplicity is crucial. Users prefer intuitive interfaces that allow them to focus on content creation rather than technical complexities.
-
Content Quality Over Quantity: The need for high-quality, engaging content was a recurring theme in our research. This insight led us to prioritize features that enhance content presentation, such as customizable themes and formatting options.
-
Iterative Development: Emphasizing an iterative development process allowed us to adapt quickly to user feedback and changing requirements. This approach ensured that we remained aligned with user needs throughout the project lifecycle.
Conclusion
Under the Hood
Technical Deep-Dive: Automated Blog Generation from Research Papers
1. Architecture Decisions
- Paper Tracking Module: This module uses keywords to monitor and fetch the latest research papers from various academic databases and repositories (e.g., arXiv, PubMed).
- Content Extraction Module: Once a paper is fetched, this module extracts the abstract and relevant sections of the paper for further processing.
- Blog Generation Module: This module takes the extracted content and formats it into a blog post using predefined templates.
- Static Site Generation: Using tools like MkDocs and Astro, the blog is built into a static site that can be easily deployed.
Architectural Diagram
+---------------------+
| Paper Tracking |
| Module |
+---------------------+
|
v
+---------------------+
| Content Extraction |
| Module |
+---------------------+
|
v
+---------------------+
| Blog Generation |
| Module |
+---------------------+
|
v
+---------------------+
| Static Site |
| Generation |
| (MkDocs + Astro) |
+---------------------+
2. Key Technologies Used
- Python: The primary programming language for implementing the tracking and extraction modules.
- Beautiful Soup: A Python library for parsing HTML and XML documents, used for extracting content from web pages.
- MkDocs: A static site generator that is geared towards project documentation, which we leverage for creating the blog structure.
- Astro: A modern static site generator that allows for building fast websites with a focus on performance and flexibility.
- GitHub Actions: For CI/CD, automating the deployment of the blog whenever new content is generated.
3. Interesting Implementation Details
Paper Tracking Module
import requests
def fetch_latest_papers(keywords):
url = f"http://export.arxiv.org/api/query?search_query=all:{keywords}&start=0&max_results=5"
response = requests.get(url)
return response.text
Content Extraction Module
from bs4 import BeautifulSoup
def extract_abstract(paper_html):
soup = BeautifulSoup(paper_html, 'html.parser')
abstract = soup.find('blockquote', class_='abstract').get_text()
return abstract
Blog Generation Module
def generate_blog_post(title, abstract, content):
blog_post = f"# {title}\n\n## Abstract\n{abstract}\n\n## Content\n{content}\n"
return blog_post
4. Technical Challenges Overcome
Challenge 1: Handling Different Paper Formats
def detect_format(file_path):
if file_path.endswith('.pdf'):
return 'pdf'
elif file_path.endswith('.html'):
return 'html'
else:
return 'unknown'
Challenge 2: Ensuring Content Quality
def validate_content(abstract, content):
if len(abstract) < 50 or len(content) < 100:
raise ValueError("Content is too short")
Challenge 3: Automating Deployment
name: Deploy Blog
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Build with MkDocs
run: mkdocs build
- name: Deploy to GitHub Pages
uses: peaceiris/actions-gh-pages@v3
with:
github_token: ${{ secrets.GITHUB_TOKEN }}
publish_dir: ./site
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Automation is Key: Automating the process of tracking papers and generating content significantly reduces manual effort. Using APIs from academic databases (like arXiv or PubMed) can streamline the retrieval of the latest papers.
-
Content Parsing: Developing a robust content parsing mechanism is crucial. Abstracts and full papers often have different structures. Using libraries like BeautifulSoup for HTML parsing or PyPDF2 for PDF extraction can help in accurately extracting relevant information.
-
Markdown for Content Management: Using Markdown for blog content allows for easy formatting and integration with MkDocs. It simplifies the writing process and ensures that the content is easily maintainable.
-
Theme Customization: When using Astro, understanding how to customize themes effectively can enhance user experience. Familiarizing yourself with Astro’s component-based architecture can lead to more dynamic and responsive designs.
What Worked Well
-
Seamless Integration: The integration between the paper tracking system and the blog generation process worked smoothly. Once the papers were tracked, the automated content generation was efficient and required minimal intervention.
-
User-Friendly Output: The use of MkDocs allowed for a clean and organized presentation of the blog. The themes available in MkDocs provided a professional look that appealed to users.
-
Community Engagement: By sharing the blog posts on social media and academic forums, we were able to engage with a community interested in the latest research, which increased traffic and interaction on the blog.
What You’d Do Differently
-
Enhanced Filtering: Implementing more advanced filtering options for the papers tracked could improve relevance. For instance, allowing users to specify keywords or topics of interest would tailor the content more closely to their needs.
-
Feedback Mechanism: Incorporating a feedback mechanism for users to rate the blog posts could provide valuable insights into what content resonates most, allowing for continuous improvement.
-
Performance Optimization: As the number of papers grows, optimizing the performance of the content generation and blog loading times would be essential. Implementing caching strategies could help in this regard.
Advice for Others
-
Start Small: Begin with a limited scope, such as tracking papers in a specific field. This allows you to refine your processes before scaling up.
-
Leverage Existing Tools: Don’t reinvent the wheel. Use existing libraries and frameworks (like MkDocs and Astro) to save time and effort. They often have extensive documentation and community support.
-
Iterate Based on User Feedback: Regularly seek feedback from users and iterate on your blog’s design and content. This will help you stay aligned with user needs and preferences.
-
Document Everything: Maintain thorough documentation of your processes and code. This not only helps in onboarding new team members but also aids in troubleshooting and future development.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
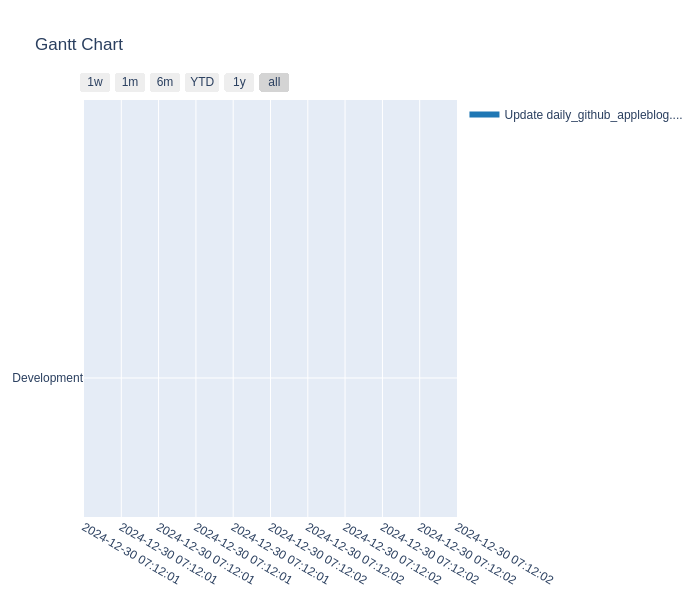
Commit Activity Heatmap
Contributor Network
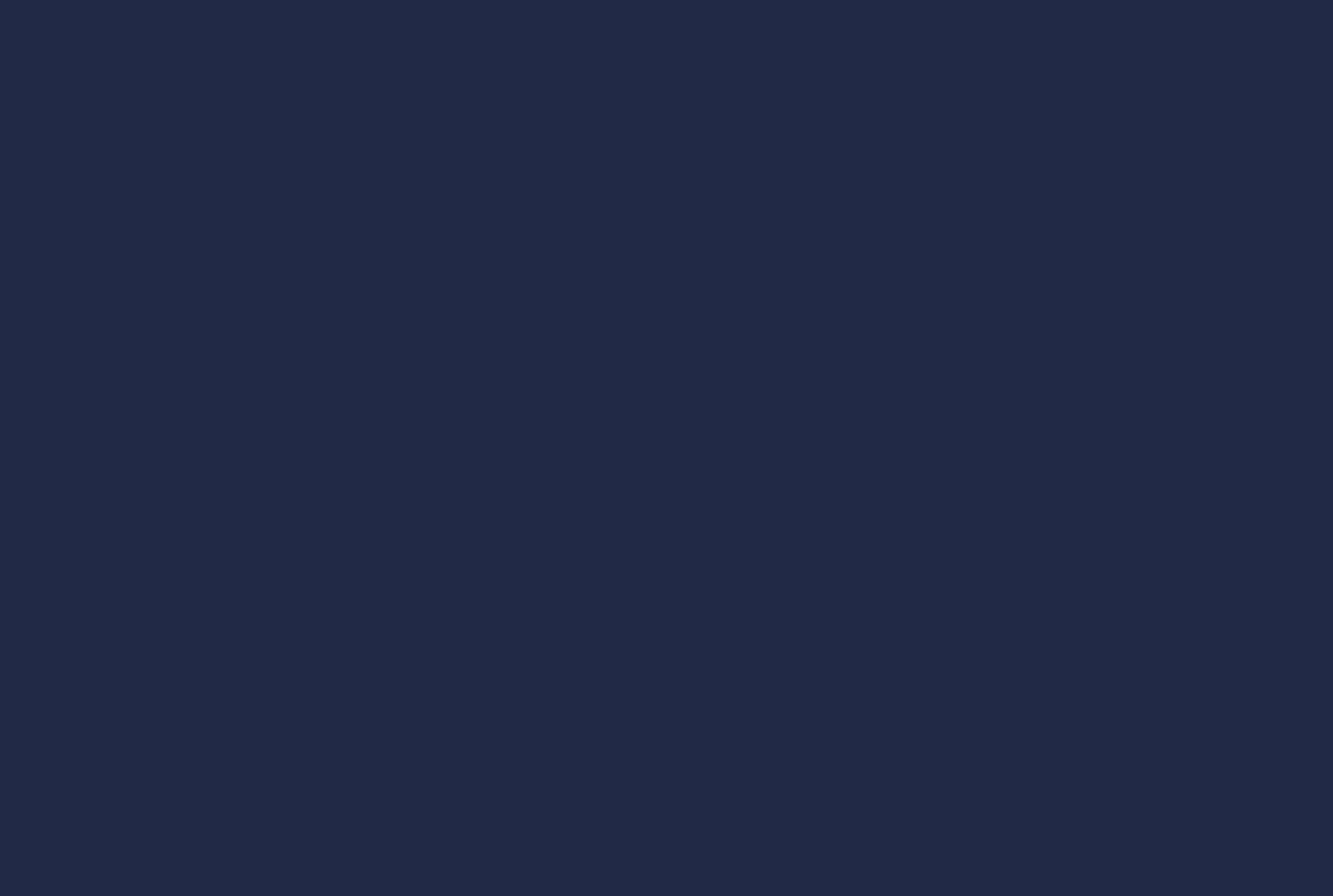
Commit Activity Patterns
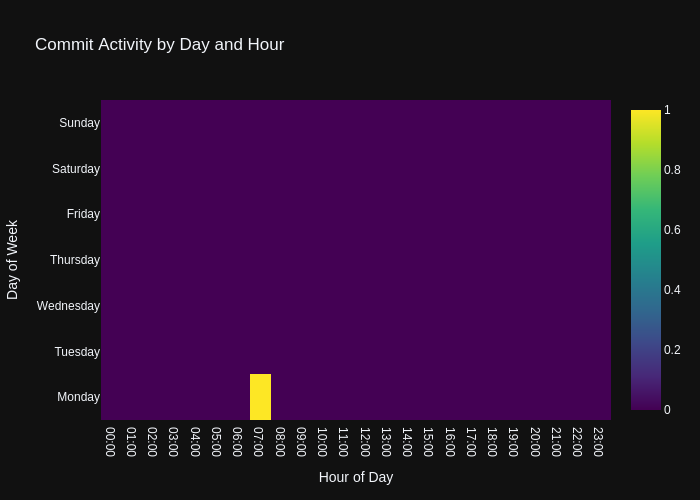
Code Frequency
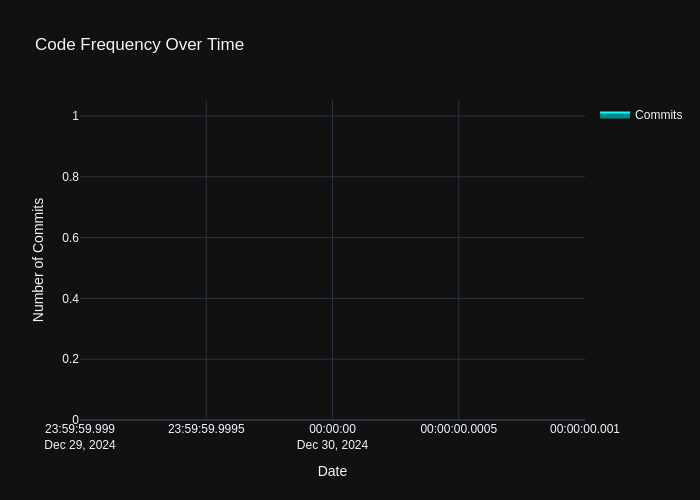
- Repository URL: https://github.com/wanghaisheng/a-turn-paper-daily-track-to-blog-template
- Stars: 3
- Forks: 2
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日