From Idea to Reality: Building a Fullstack Django-React Starter with Docker
Heisenberg
2024 年 12 月 30 日
Django-React starter with Docker support for fast and easy web development.
Built by wanghaisheng | Last updated: 20241230
11 minutes 28 seconds read
Project Genesis
Unleashing the Power of Full-Stack Development: My Journey with the Django-React Starter
As a developer, I’ve always been fascinated by the seamless interaction between front-end and back-end technologies. The spark for creating the Django-React Starter ignited during a late-night coding session, where I found myself juggling multiple frameworks and libraries, trying to piece together a cohesive web application. Frustrated yet inspired, I realized there had to be a better way to streamline the development process.
My personal motivation for this project stemmed from a desire to empower fellow developers—whether they’re seasoned pros or just starting out. I wanted to create a starter kit that not only simplifies the setup but also provides a robust foundation for building dynamic web applications. After all, the joy of coding should come from creating and innovating, not from wrestling with configuration files and dependencies.
Of course, the journey wasn’t without its challenges. I faced hurdles like integrating Docker for containerization and ensuring smooth communication between Django and React. Each obstacle taught me valuable lessons, pushing me to refine my approach and ultimately leading to a more polished product.
In this blog post, I’ll take you through the key features of the Django-React Starter, share insights from my development process, and provide a quick overview of how you can get started on your own full-stack journey. Whether you’re looking to build a personal project or a scalable application, I hope this starter kit inspires you to dive into the world of full-stack development with confidence!
From Idea to Implementation
Journey from Concept to Code: Django React Starter
1. Initial Research and Planning
The journey of creating the Django React Starter began with a thorough analysis of the current web development landscape. The goal was to create a starter template that would streamline the development process for full-stack applications using Django for the backend and React for the frontend.
During the initial research phase, several key factors were considered:
- Market Demand: There was a growing demand for modern web applications that leverage the strengths of both Django and React. Django’s robust backend capabilities combined with React’s dynamic user interface offered a powerful solution for developers.
- Community Feedback: Engaging with developer communities revealed common pain points, such as the complexity of setting up a full-stack environment and the need for efficient deployment strategies.
- Existing Solutions: A review of existing starter templates highlighted gaps in documentation, testing, and deployment processes. This provided an opportunity to create a more comprehensive solution.
The planning phase culminated in defining the core features and architecture of the project, ensuring that it would meet the needs of developers looking for a reliable starting point for their applications.
2. Technical Decisions and Their Rationale
Several technical decisions were made during the development of the Django React Starter, each with a clear rationale:
- Framework Selection: Django was chosen for the backend due to its maturity, security features, and built-in admin interface. React was selected for the frontend because of its component-based architecture, which promotes reusability and maintainability.
- Docker Integration: The decision to include Docker support was driven by the need for a consistent development environment. Docker allows developers to run applications in isolated containers, reducing the “it works on my machine” problem.
- Celery and RabbitMQ: For handling asynchronous tasks, Celery was integrated with RabbitMQ as the message broker. This combination is widely used in the industry and provides a robust solution for background processing.
- Postgres Database: Postgres was chosen for its advanced features, such as support for JSONB and full-text search, which are beneficial for modern web applications.
These decisions were made with the intent of providing a solid foundation that would be easy to extend and maintain.
3. Alternative Approaches Considered
Throughout the development process, several alternative approaches were considered:
- Monolithic vs. Microservices: Initially, there was a debate about whether to adopt a monolithic architecture or a microservices approach. Ultimately, a monolithic structure was chosen for simplicity and ease of deployment, especially for a starter template aimed at new developers.
- Frontend Frameworks: While React was the final choice, other frontend frameworks like Vue.js and Angular were considered. React’s popularity and extensive ecosystem made it the preferred option.
- Database Options: Alternatives like MySQL and SQLite were evaluated, but Postgres was favored for its scalability and feature set.
These considerations helped refine the project scope and ensure that the final product would be both practical and user-friendly.
4. Key Insights That Shaped the Project
Several key insights emerged during the development of the Django React Starter:
- Documentation is Crucial: The importance of clear and comprehensive documentation became evident early on. A well-documented project not only aids in onboarding new developers but also enhances the overall user experience.
- Community Engagement: Actively engaging with the developer community provided valuable feedback that shaped the project’s features and usability. This reinforced the idea that a project should evolve based on user needs.
- Testing and Quality Assurance: The integration of testing tools and CI/CD pipelines was recognized as essential for maintaining code quality. This insight led to the inclusion of automated testing and quality checks as core components of the project.
These insights not only guided the development of the Django React Starter but also established a framework for future projects, emphasizing the importance of user-centric design and quality assurance.
In conclusion, the journey from concept to code for the Django React Starter was marked by careful research, thoughtful technical decisions, and a commitment to quality. The result is a robust starter template that empowers developers to build modern web applications efficiently.
Under the Hood
Technical Deep-Dive: Django React Starter
1. Architecture Decisions
The architecture of the Django React Starter is designed to facilitate rapid web development while ensuring scalability and maintainability. The choice of a decoupled architecture, where the frontend and backend are separated, allows for independent development and deployment cycles. This separation is achieved through the use of Django as the backend framework and React as the frontend library.
Key Architectural Choices:
- Microservices Approach: The integration of Celery for asynchronous task management and RabbitMQ for messaging allows for a microservices-like architecture, where different components can communicate efficiently.
- Containerization: The use of Docker ensures that the application can run consistently across different environments, simplifying the development and deployment processes.
- Database Choice: PostgreSQL is chosen for its robustness and support for advanced features like JSONB, which can be beneficial for handling complex data structures.
2. Key Technologies Used
The project leverages a variety of technologies that work together to create a powerful web application:
- Django: A high-level Python web framework that encourages rapid development and clean, pragmatic design.
- Django REST Framework (DRF): A powerful toolkit for building Web APIs, allowing for easy integration with the React frontend.
- Celery: An asynchronous task queue/job queue based on distributed message passing, used for handling background tasks.
- RabbitMQ: A message broker that facilitates communication between different services, particularly between Django and Celery.
- React: A JavaScript library for building user interfaces, providing a dynamic and responsive user experience.
- Vite: A build tool that provides a fast development environment for modern web projects, enhancing the React development experience.
- PostgreSQL: A powerful, open-source relational database system known for its reliability and feature set.
- Meilisearch: A powerful search engine that provides fast and relevant search results, integrated with the application for enhanced search capabilities.
- Docker: A platform for developing, shipping, and running applications in containers, ensuring consistency across environments.
- Fly.io: A platform for deploying applications globally, simplifying the deployment process.
3. Interesting Implementation Details
Docker Integration
The project includes a
Dockerfile
and docker-compose.yml
file, which allows developers to run the entire application stack locally with a single command. The docker-compose
file defines services for the backend, frontend, database, and message broker, making it easy to manage dependencies.Example
docker-compose.yml
snippet:version: '3.8'
services:
web:
build: .
command: python manage.py runserver 0.0.0.0:8000
volumes:
- .:/code
ports:
- "8000:8000"
db:
image: postgres
environment:
POSTGRES_DB: mydatabase
POSTGRES_USER: user
POSTGRES_PASSWORD: password
Celery and RabbitMQ Integration
Celery is configured to work with RabbitMQ as the message broker. This allows for background tasks to be processed asynchronously, improving the responsiveness of the application.
Example Celery task:
from celery import shared_task
@shared_task
def send_email_task(email_address):
# Logic to send email
print(f"Sending email to {email_address}")
4. Technical Challenges Overcome
Asynchronous Task Management
One of the challenges faced was managing asynchronous tasks effectively. Integrating Celery with Django required careful configuration of the message broker (RabbitMQ) and ensuring that tasks were retried in case of failure.
Cross-Origin Resource Sharing (CORS)
When developing the frontend and backend separately, CORS issues often arise. Configuring Django to allow requests from the React frontend was necessary to ensure smooth communication between the two.
Example CORS configuration in Django:
# settings.py
CORS_ALLOWED_ORIGINS = [
"http://localhost:3000",
]
Deployment
Deploying the application to Fly.io required creating a
fly.toml
configuration file that specifies the deployment settings. This included defining the services, environment variables, and build settings.Example
fly.toml
snippet:app = "my-django-react-app"
[build]
builder = "heroku/buildpacks:20"
[env]
DATABASE_URL = "postgres://user:password@db:5432/mydatabase"
Conclusion
The Django React Starter project exemplifies modern web development practices by combining powerful technologies and architectural decisions that promote scalability and maintainability. The integration of Docker, Celery, and RabbitMQ, along with a decoupled frontend and backend, provides a robust foundation for building complex web applications. The challenges faced during development, such as asynchronous task management and CORS configuration, were effectively addressed, resulting in a well-structured and functional application.
Lessons from the Trenches
Here are some key technical lessons learned, what worked well, what could be done differently, and advice for others based on the project history and README of the Django React Starter:
1. Key Technical Lessons Learned
- Integration of Technologies: Successfully integrating Django with React using Docker has shown the importance of containerization in modern web development. It simplifies the setup process and ensures consistency across different environments.
- Celery and RabbitMQ: Implementing Celery for background tasks and RabbitMQ for messaging demonstrated the effectiveness of asynchronous processing in improving application performance and user experience.
- Database Management: Using PostgreSQL as the database highlighted the importance of choosing a robust database system that can handle complex queries and large datasets efficiently.
- Search Functionality: Integrating Meilisearch provided insights into how to implement fast and relevant search capabilities, which is crucial for user engagement.
2. What Worked Well
- Documentation: The clear and structured README file made it easy for new developers to get started quickly. The use of templates for
README.md
andCHANGELOG.md
helped maintain consistency and clarity. - Docker Support: The inclusion of Docker and
docker-compose
allowed for easy local development and testing, which is a significant advantage for teams working in different environments. - Quality Assurance Tools: The integration of tests, linters, and formatters ensured code quality and maintainability, which is essential for long-term project success.
- CI/CD Pipeline: Setting up continuous integration and deployment processes streamlined the workflow, allowing for quicker iterations and deployments.
3. What You’d Do Differently
- More Modular Structure: While the project is well-structured, further modularizing components (e.g., separating API and frontend services) could enhance scalability and maintainability.
- Enhanced Error Handling: Implementing more robust error handling and logging mechanisms would improve debugging and monitoring in production environments.
- User Authentication: Exploring more advanced user authentication methods (e.g., OAuth, JWT) could enhance security and user experience.
- Performance Optimization: Conducting performance testing and optimization earlier in the development process could help identify bottlenecks and improve application responsiveness.
4. Advice for Others
- Start with Clear Documentation: Invest time in creating comprehensive documentation from the beginning. It pays off by making onboarding easier for new contributors and reducing the learning curve.
- Embrace Containerization: Use Docker for all projects, as it simplifies dependencies and environment management, making it easier to collaborate with others.
- Focus on Testing: Prioritize writing tests for both backend and frontend components. This not only ensures code quality but also builds confidence when making changes or adding new features.
- Iterate and Refine: Be open to feedback and continuously iterate on your project. Regularly review and refine your codebase, documentation, and processes to adapt to new challenges and technologies.
By following these lessons and advice, developers can enhance their projects and create more robust, maintainable, and user-friendly applications.
What’s Next?
Conclusion
As we reach the current milestone of the Django React Starter project, we are excited to share that the foundation is robust and ready for further enhancements. The integration of Django, React, and various supporting technologies like Docker, Celery, and RabbitMQ has resulted in a fully-tested application that is not only functional but also scalable. With features such as user management, health checks, and a seamless deployment process, we believe this starter kit provides an excellent base for developers looking to kickstart their web applications.
Looking ahead, our development plans include expanding the feature set to include more advanced functionalities, such as enhanced user authentication methods, improved search capabilities with Meilisearch, and additional integrations with third-party services. We also aim to refine the documentation further, making it even easier for new users to get started and contribute to the project. Continuous improvements in testing and quality assurance will ensure that the project remains reliable and up-to-date with the latest technologies.
We invite all developers, whether you’re a seasoned pro or just starting your coding journey, to contribute to the Django React Starter. Your insights, code contributions, and feedback are invaluable in shaping the future of this project. Whether it’s fixing bugs, adding new features, or enhancing documentation, every contribution helps us create a more robust and user-friendly starter kit.
In closing, the journey of this side project has been both challenging and rewarding. It has provided us with an opportunity to learn, collaborate, and innovate. We hope that this starter kit serves as a springboard for your own projects and inspires you to explore the endless possibilities of web development. Together, let’s build something amazing!
Project Development Analytics
timeline gant
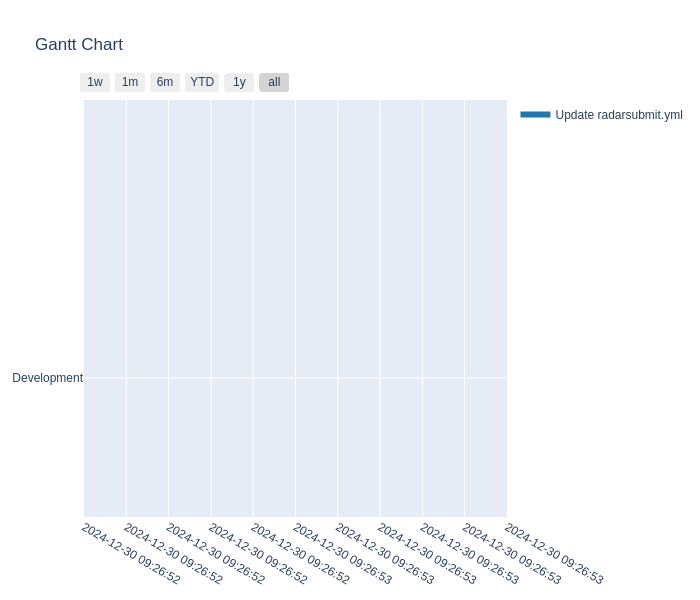
Commit timelinegant
Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
Commit Heatmap
Contributor Network
This network diagram shows how different contributors interact:
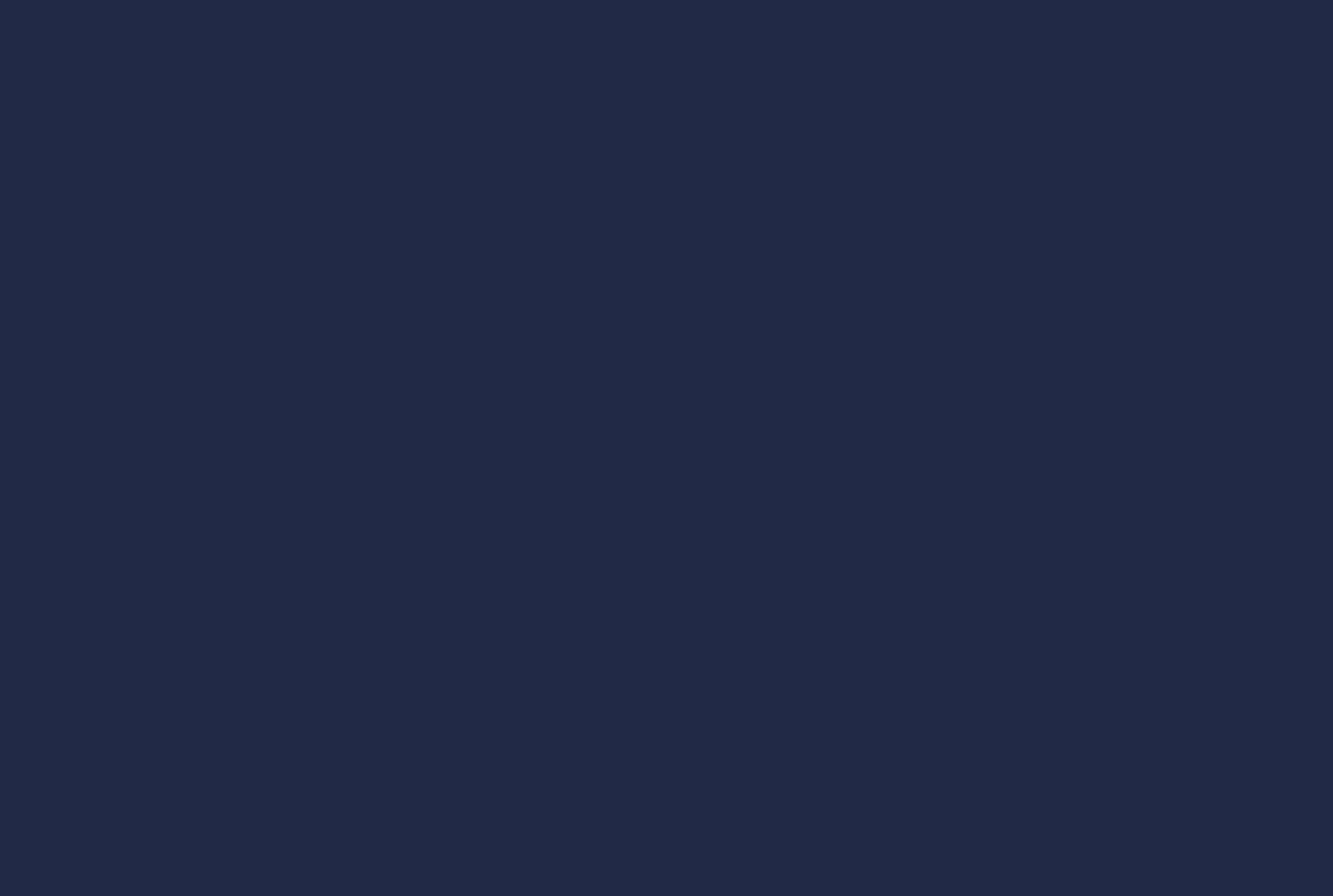
Contributor Network
Commit Activity Patterns
This chart shows when commits typically happen:
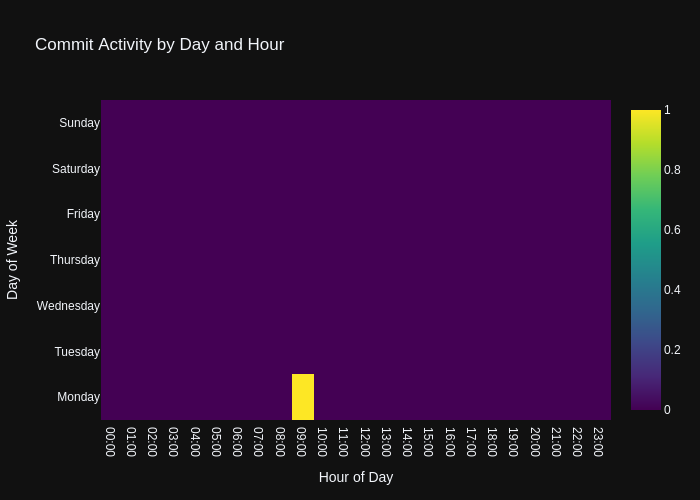
Commit Activity
Code Frequency
This chart shows the frequency of code changes over time:
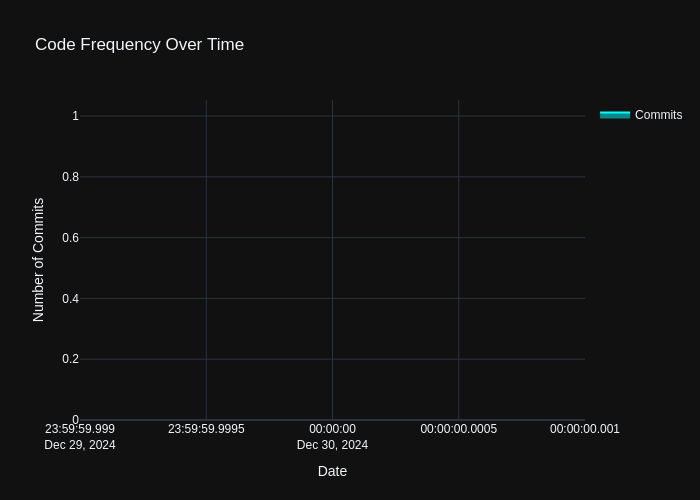
Code Frequency
- Repository URL: https://github.com/wanghaisheng/a_fullstack_django-react_-starter
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日