From Idea to Reality: Crafting Comics with AI Comic Factory
Project Genesis
Unleashing Creativity with AI Comic Factory 👩🎨
From Idea to Implementation
Journey from Concept to Code: AI Comic Factory
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Choice of LLM Engine: After evaluating several LLM options, I decided to use Hugging Face’s
zephyr-7b-beta
model for its balance of performance and accessibility. This model was chosen for its ability to generate coherent and contextually relevant text, which is essential for storytelling. -
Rendering Engine Selection: The rendering of comic panels required a robust image generation solution. I opted for a combination of Hugging Face’s SD-XL and Replicate APIs, as they provided high-quality image outputs and were relatively easy to integrate. This decision was driven by the need for a seamless user experience, where text prompts could be transformed into visually appealing comic panels.
-
Containerization with Docker: To ensure that the application could be easily deployed across different environments, I chose to use Docker. This decision facilitated the management of dependencies and configurations, making it easier for users to run the application locally or in the cloud.
-
Authentication and API Management: Given the reliance on third-party APIs, I implemented a secure authentication mechanism using OAuth tokens. This approach not only enhanced security but also allowed for easy integration with various LLM and rendering services.
3. Alternative Approaches Considered
-
Monolithic vs. Microservices Architecture: Initially, I contemplated building a monolithic application that would handle all functionalities in a single codebase. However, this approach was quickly dismissed in favor of a microservices architecture, which offered greater scalability and maintainability.
-
Different LLM Providers: While Hugging Face’s models were the primary choice, I also explored options from OpenAI and Anthropic. Ultimately, the decision to use Hugging Face was based on the community support, ease of access, and the specific capabilities of the
zephyr-7b-beta
model. -
Custom Rendering Solutions: I considered developing a custom rendering engine to have complete control over the image generation process. However, this would have significantly increased development time and complexity. Instead, leveraging existing APIs allowed for rapid prototyping and deployment.
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of a user-friendly interface became evident early on. Users wanted a tool that was intuitive and required minimal technical knowledge. This insight drove the design of the frontend, ensuring that the comic creation process was straightforward and enjoyable.
-
Community Engagement: Engaging with the community throughout the development process provided valuable feedback and ideas. This interaction not only helped refine features but also fostered a sense of ownership among users, which is crucial for the success of any open-source project.
-
Iterative Development: Embracing an iterative development approach allowed for continuous improvement based on user feedback. Regular updates and feature enhancements were implemented, ensuring that the project remained aligned with user needs and technological advancements.
-
Flexibility and Modularity: The decision to build a modular application architecture proved beneficial. It allowed for easy integration of new features and components, such as additional LLMs or rendering engines, without disrupting the existing functionality.
Under the Hood
Technical Deep-Dive: AI Comic Factory
1. Architecture Decisions
Key Components:
- Frontend: The user interface where users input prompts and view generated comics.
- Backend: Handles API requests, manages authentication, and orchestrates communication between the frontend and various AI services.
- LLM (Large Language Model): Responsible for generating comic scripts based on user prompts.
- Rendering Engine: Generates images for comic panels using various APIs.
Configuration Management:
.env
files) allows for easy configuration of different components without hardcoding sensitive information. This approach enhances security and flexibility, enabling users to switch between different LLMs and rendering engines seamlessly.2. Key Technologies Used
- Docker: The project is containerized using Docker, which simplifies deployment and ensures consistency across different environments.
- Hugging Face Inference API: Utilized for accessing pre-trained models, such as
zephyr-7b-beta
, which is optimized for generating JSON responses suitable for comic scripts. - OpenAI API: Provides access to powerful language models like
gpt-4-turbo
, allowing users to leverage state-of-the-art AI capabilities. - Stable Diffusion: Used for generating images, with options to integrate various rendering engines like Replicate and VideoChain.
Example of Docker Configuration:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "start"]
3. Interesting Implementation Details
Dynamic Configuration:
.env.local
file, allowing for a tailored experience.Example of Environment Variable Configuration:
# LLM Configuration
LLM_ENGINE="OPENAI"
AUTH_OPENAI_API_KEY="YourOpenAIKey"
# Rendering Configuration
RENDERING_ENGINE="REPLICATE"
AUTH_REPLICATE_API_TOKEN="YourReplicateToken"
Community Sharing Features:
4. Technical Challenges Overcome
Integration of Multiple APIs:
Example of API Call Handling:
async function fetchComicScript(prompt) {
const response = await fetch(`${LLM_OPENAI_API_BASE_URL}/completions`, {
method: 'POST',
headers: {
'Authorization': `Bearer ${AUTH_OPENAI_API_KEY}`,
'Content-Type': 'application/json',
},
body: JSON.stringify({
model: LLM_OPENAI_API_MODEL,
prompt: prompt,
max_tokens: 150,
}),
});
if (!response.ok) {
throw new Error('Failed to fetch comic script');
}
return await response.json();
}
Handling Model Variability:
Future Enhancements:
Lessons from the Trenches
Key Technical Lessons Learned
-
Complexity of Integration: Integrating multiple APIs (LLM and rendering engines) requires careful management of environment variables and configurations. Each API has its own authentication and endpoint requirements, which can lead to confusion if not documented clearly.
-
Open-Source Collaboration: Leveraging open-source components can significantly speed up development, but it also requires understanding the dependencies and potential issues that may arise from using third-party libraries or services.
-
Scalability Considerations: When designing the architecture, it’s crucial to consider how the application will scale. Using cloud-based solutions for LLMs and rendering can help manage load, but it also introduces latency and potential costs that need to be monitored.
-
User Experience: The importance of a seamless user experience cannot be overstated. Ensuring that the application is intuitive and that error messages are clear can greatly enhance user satisfaction.
What Worked Well
-
Modular Design: The separation of concerns between the LLM and rendering engines allowed for flexibility in choosing different providers. This modularity makes it easier to swap out components without affecting the entire system.
-
Community Engagement: Encouraging community contributions and sharing through platforms like Hugging Face has fostered a collaborative environment. This has led to valuable feedback and improvements from users.
-
Documentation: Providing clear and comprehensive documentation, including examples for setting up the environment, has been beneficial for users trying to deploy the project on their own.
-
Use of Docker: Utilizing Docker for containerization simplified the deployment process, allowing users to run the application in a consistent environment without worrying about local dependencies.
What You’d Do Differently
-
Improved Documentation for APIs: While the documentation is comprehensive, it could benefit from more detailed examples and use cases for each API option. This would help users understand the practical implications of their choices.
-
Error Handling and Logging: Implementing more robust error handling and logging mechanisms would help in diagnosing issues during deployment and usage. This would also improve the overall reliability of the application.
-
Performance Optimization: Conducting performance testing and optimization earlier in the development process could help identify bottlenecks and improve the responsiveness of the application.
-
User Feedback Loop: Establishing a more formalized feedback loop with users could provide insights into their experiences and needs, leading to more targeted improvements in future releases.
Advice for Others
-
Start with a Clear Architecture: Before diving into coding, spend time designing the architecture of your application. Clearly define how different components will interact and what technologies you will use.
-
Prioritize Documentation: Invest time in creating thorough documentation from the start. This will save you and your users time in the long run and reduce the number of support requests.
-
Embrace Open Source: Don’t hesitate to use open-source libraries and APIs, but be mindful of their licensing and support. Contributing back to these projects can also enhance your own learning and the community.
-
Iterate Based on User Feedback: Regularly seek feedback from users and be willing to iterate on your design and features. This will help ensure that your project remains relevant and useful.
-
Test Early and Often: Implement testing at every stage of development. This includes unit tests, integration tests, and user acceptance testing to catch issues before they reach production.
What’s Next?
Conclusion: The Future of AI Comic Factory
Project Development Analytics
timeline gant
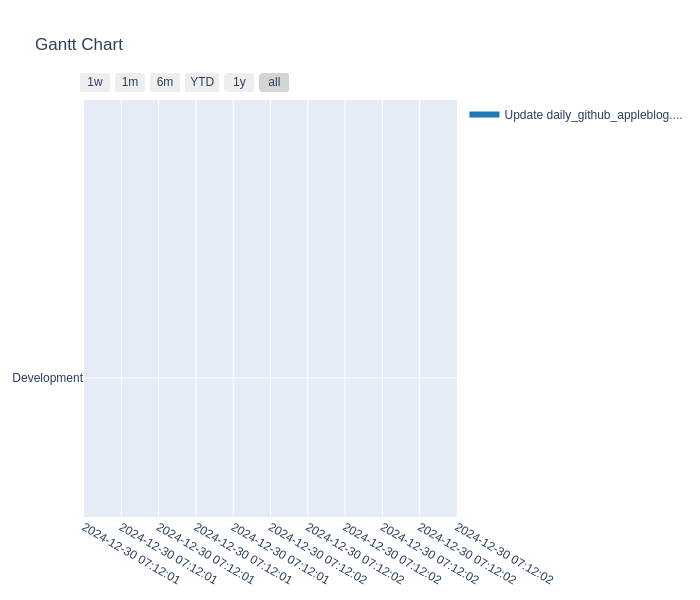
Commit Activity Heatmap
Contributor Network
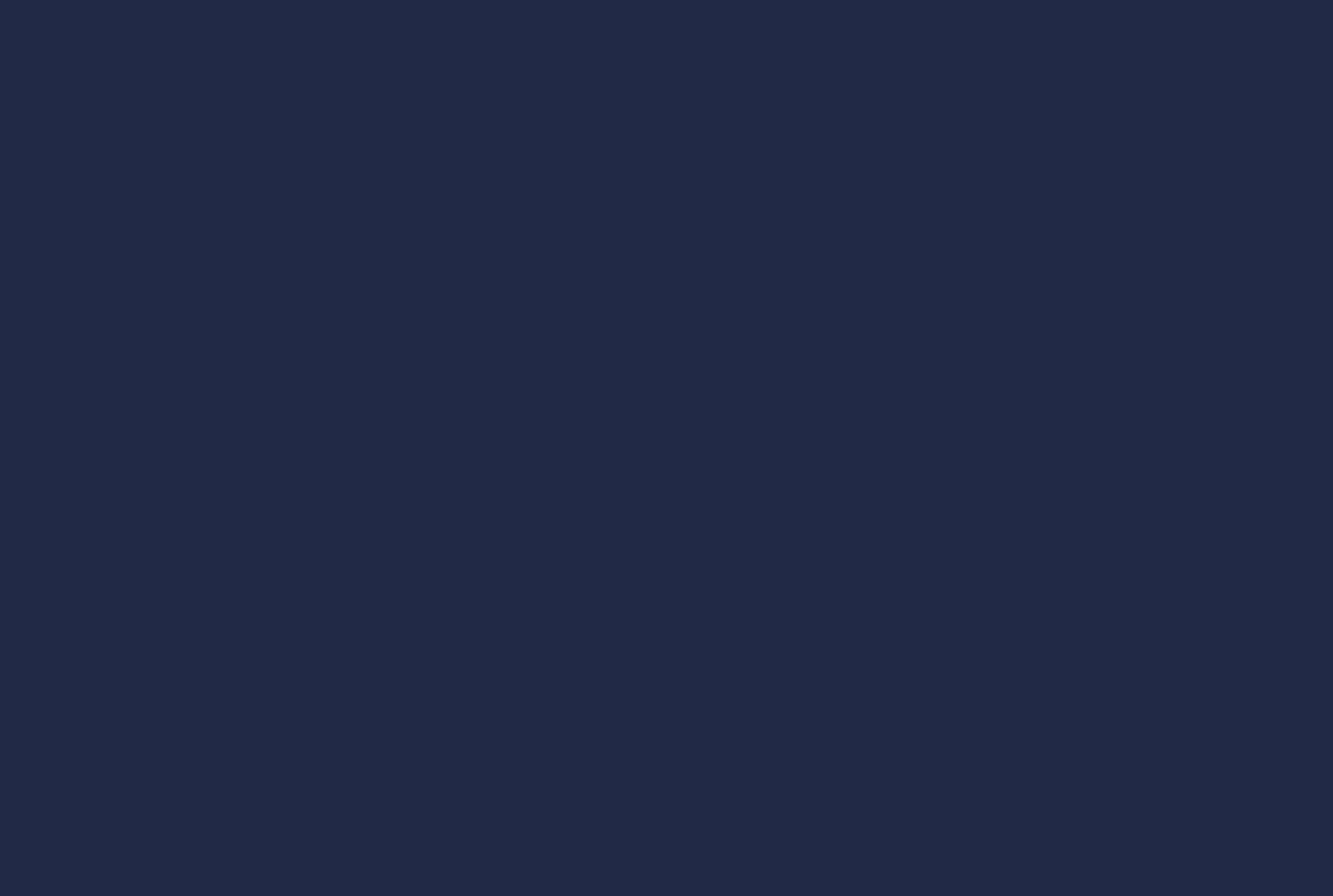
Commit Activity Patterns
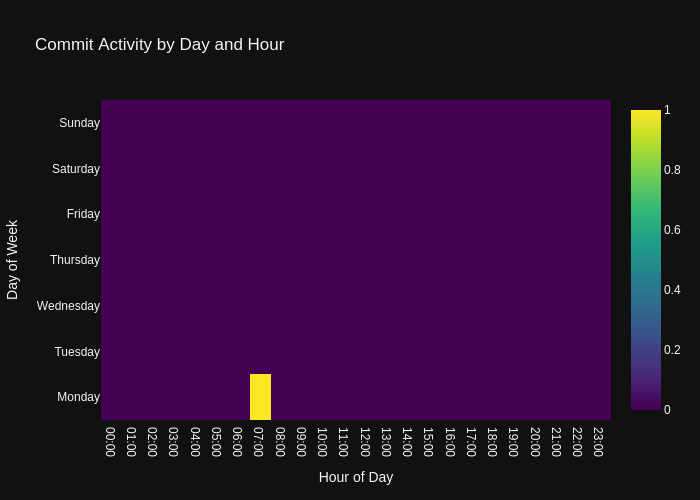
Code Frequency
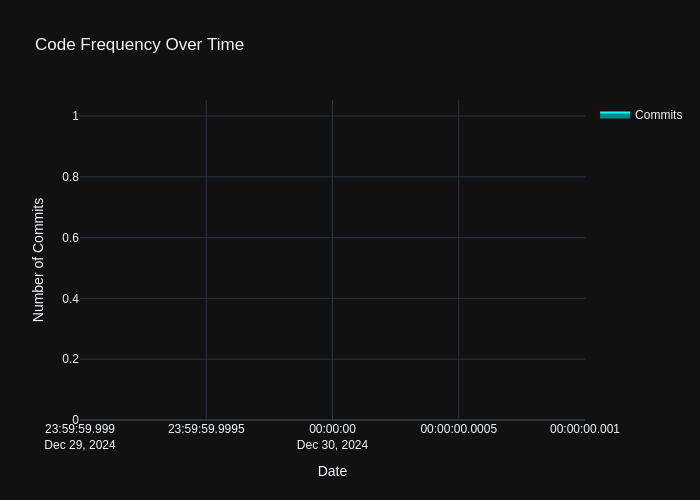
- Repository URL: https://github.com/wanghaisheng/ai-comic-factory
- Stars: 1
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日