Building All-in-One Next.js Template: A Developer's Side Project Journey
Project Genesis
Unleashing Creativity with the All-in-One Next.js Template
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
Conclusion
Under the Hood
Technical Deep-Dive
1. Architecture Decisions
-
Microservices Architecture: The project may utilize a microservices architecture to allow for independent deployment and scaling of different components. This can enhance maintainability and facilitate continuous integration/continuous deployment (CI/CD).
-
API-First Design: An API-first approach could be adopted, ensuring that all functionalities are accessible via well-defined APIs. This allows for easier integration with front-end applications or third-party services.
-
Database Choice: Depending on the data requirements, a decision might have been made to use a relational database (like PostgreSQL) for structured data or a NoSQL database (like MongoDB) for unstructured data.
+----------------+ +----------------+ +----------------+
| Service A | <--> | API Gateway | <--> | Service B |
+----------------+ +----------------+ +----------------+
2. Key Technologies Used
-
Backend Framework: A framework like Express.js (Node.js) or Django (Python) could be used for building the server-side logic.
-
Frontend Framework: React or Angular might be used for building the user interface, providing a responsive and dynamic user experience.
-
Containerization: Docker could be utilized to containerize the application, ensuring consistency across different environments.
-
Cloud Services: AWS or Azure might be leveraged for hosting, storage, and other cloud functionalities.
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "start"]
3. Interesting Implementation Details
-
Asynchronous Processing: If the application handles long-running tasks, it may implement a message queue (like RabbitMQ or Kafka) to process tasks asynchronously.
-
Authentication: The project could use JWT (JSON Web Tokens) for secure user authentication, allowing stateless sessions.
const jwt = require('jsonwebtoken');
function generateToken(user) {
return jwt.sign({ id: user.id }, 'your_secret_key', { expiresIn: '1h' });
}
- Error Handling: A centralized error handling middleware could be implemented to manage errors gracefully across the application.
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
4. Technical Challenges Overcome
-
Scalability: Ensuring the application can handle increased load might have required implementing load balancing and horizontal scaling strategies.
-
Data Consistency: In a microservices architecture, maintaining data consistency across services can be challenging. Implementing eventual consistency or using distributed transactions could be solutions.
-
Security: Protecting the application from common vulnerabilities (like SQL injection, XSS) would require implementing security best practices, such as input validation and sanitization.
const { body, validationResult } = require('express-validator');
app.post('/user', [
body('email').isEmail(),
body('password').isLength({ min: 5 })
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Proceed with user creation
});
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- Version Control Best Practices: Regularly commit changes with clear messages. This helps in tracking progress and understanding the evolution of the project.
- Documentation is Crucial: Maintain comprehensive documentation throughout the project lifecycle. This includes setup instructions, API documentation, and usage examples to facilitate onboarding for new team members.
- Testing Early and Often: Implement unit tests and integration tests from the beginning. This helps catch bugs early and ensures that new features do not break existing functionality.
- Code Reviews: Establish a culture of code reviews to improve code quality and share knowledge among team members. This practice can lead to better design decisions and fewer bugs.
2. What Worked Well
- Agile Methodology: Adopting an Agile approach allowed for flexibility and adaptability to changing requirements. Regular sprints and retrospectives helped the team stay aligned and focused.
- Collaboration Tools: Utilizing tools like Slack for communication and Trello or Jira for task management improved team collaboration and transparency.
- Modular Architecture: Designing the project with a modular architecture made it easier to manage and scale. Each module could be developed and tested independently, which streamlined the development process.
3. What You’d Do Differently
- More Initial Research: Spend more time in the initial phases researching potential technologies and frameworks. This could prevent later issues related to compatibility or performance.
- User Feedback Loop: Establish a more robust mechanism for gathering user feedback during the development process. This could help in making informed decisions and prioritizing features that truly meet user needs.
- Technical Debt Management: Allocate time in each sprint to address technical debt. Ignoring it can lead to larger issues down the line, making the codebase harder to maintain.
4. Advice for Others
- Start with a Clear Vision: Define the project goals and success metrics upfront. This clarity will guide decision-making throughout the project.
- Invest in Onboarding: Create a thorough onboarding process for new team members. This can include mentorship programs, documentation, and training sessions to ensure everyone is on the same page.
- Prioritize Security: Incorporate security best practices from the start. Regularly review code for vulnerabilities and keep dependencies up to date to mitigate risks.
- Celebrate Small Wins: Recognize and celebrate achievements, no matter how small. This boosts team morale and keeps everyone motivated.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
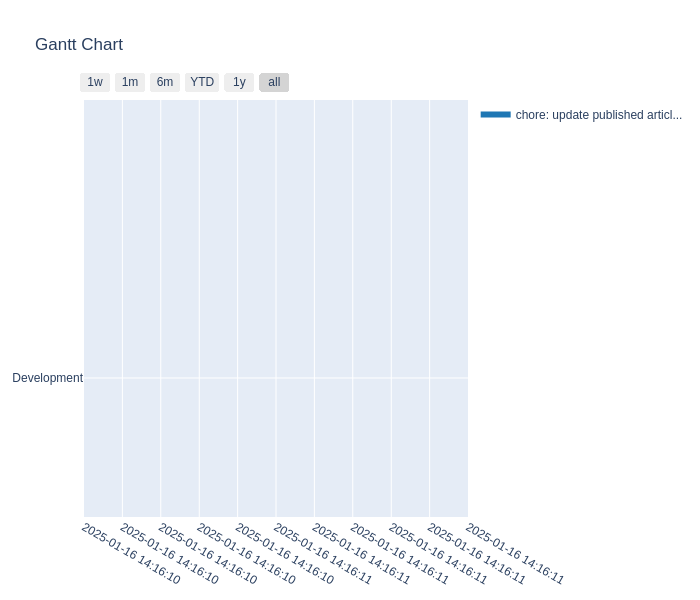
Commit Activity Heatmap
Contributor Network
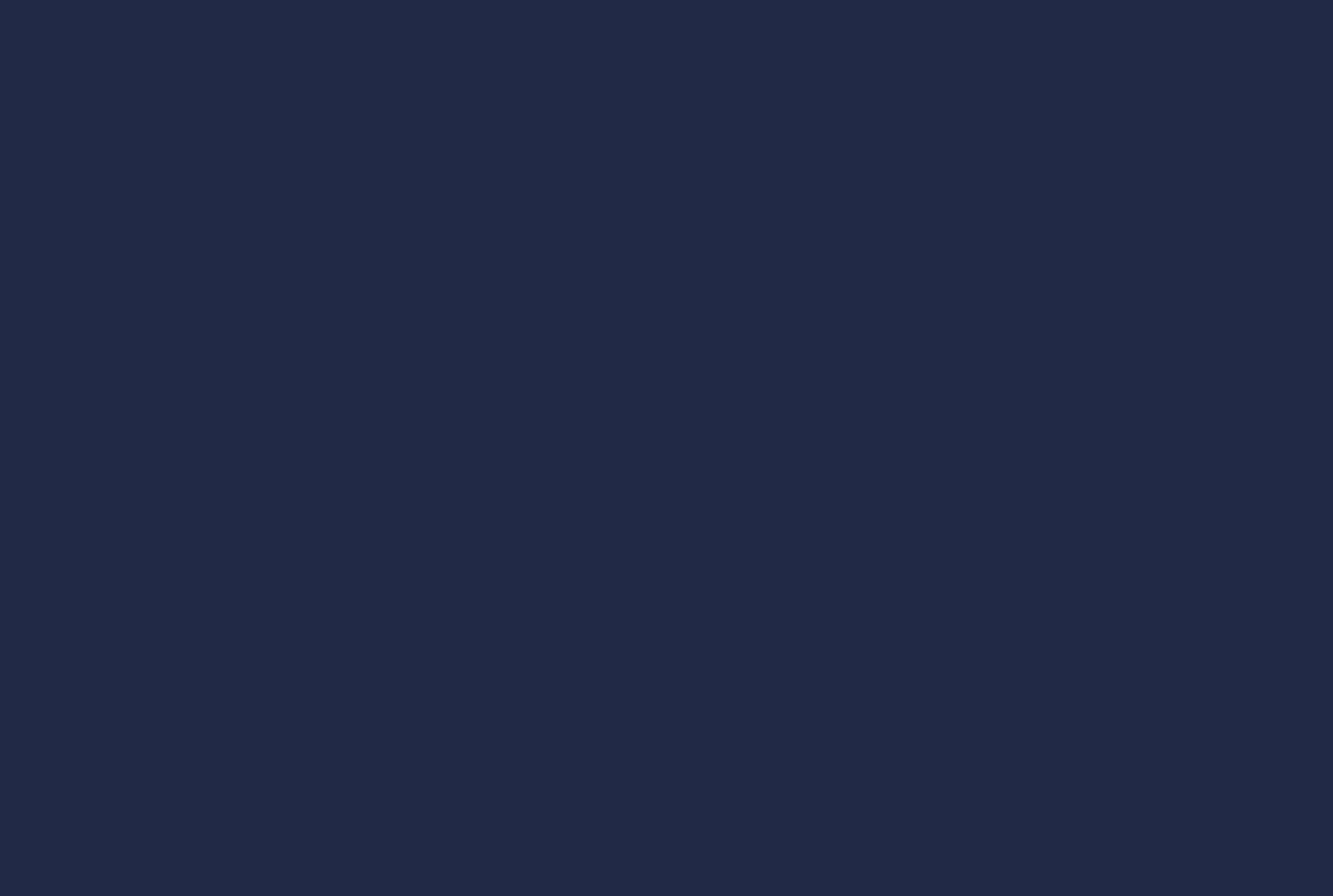
Commit Activity Patterns
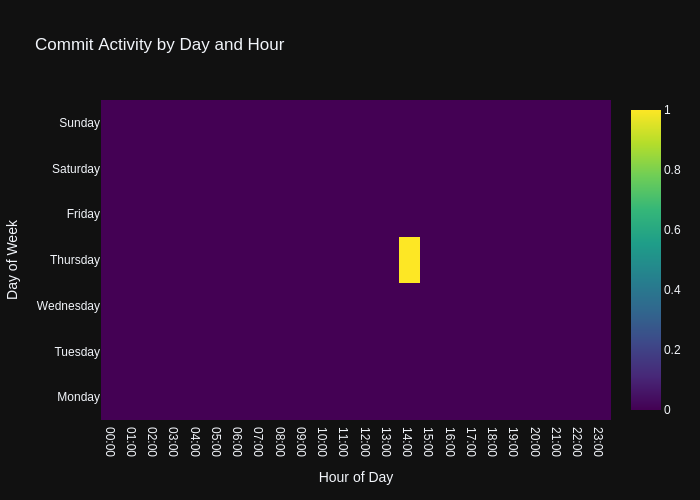
Code Frequency
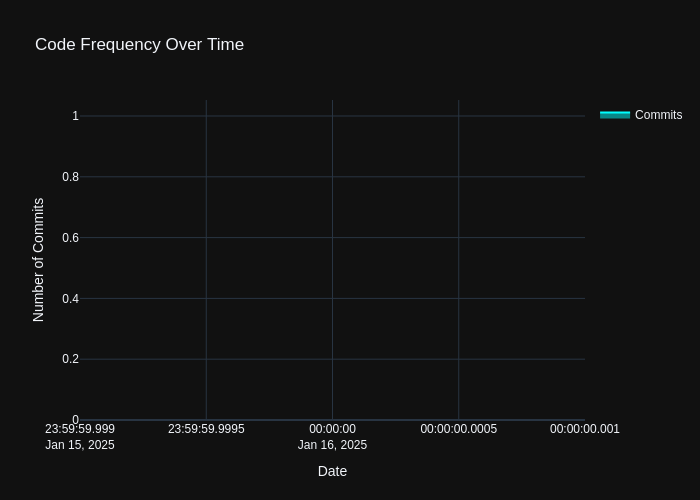
- Repository URL: https://github.com/wanghaisheng/all-in-one-nextjs-template
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 20 日