Building animunet: Crafting an Anime Haven with Next.js and Animetize API
Project Genesis
Unleashing Creativity with Animunet: A Journey of Passion and Innovation
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
Under the Hood
Technical Deep-Dive: Animunet
1. Architecture Decisions
-
Single Page Application (SPA): Utilizing Next.js allows Animunet to function as a SPA, which enhances user experience by loading content dynamically without full page reloads. This is crucial for a media-heavy application where users expect quick access to content.
-
Server-Side Rendering (SSR): Next.js supports SSR, which is beneficial for SEO and initial load performance. By rendering pages on the server, Animunet can deliver fully populated HTML to the client, improving the time to first paint (TTFP).
-
Component-Based Architecture: The use of React components promotes reusability and maintainability. Each UI element, such as the navbar, footer, and content cards, is encapsulated in its own component, making it easier to manage and update.
-
API Integration: The application likely interacts with external APIs to fetch anime data, which is essential for providing users with up-to-date content. This separation of concerns allows for a clean architecture where the frontend is decoupled from the backend.
2. Key Technologies Used
-
Next.js: A React framework that enables server-side rendering and static site generation, providing a robust foundation for building the application.
-
TypeScript: By using TypeScript, the development team benefits from static typing, which helps catch errors early in the development process and improves code quality.
-
NextUI: A React UI library that provides pre-built components, allowing for rapid development of a visually appealing user interface.
-
Vercel: The deployment platform that hosts the application, offering features like automatic scaling and serverless functions, which are ideal for handling varying traffic loads.
Example of a Component in Next.js
import React from 'react';
const Navbar = () => {
return (
<nav>
<h1>Animunet</h1>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
</ul>
</nav>
);
};
export default Navbar;
3. Interesting Implementation Details
-
Dynamic Routing: Next.js allows for dynamic routing, which is utilized to create pages for individual anime titles. For example, a URL like
/watch/[animeId]
can dynamically render the watch page for any anime based on its ID. -
Image Optimization: The application uses Next.js’s built-in image optimization features, which automatically serve images in the most efficient format and size, improving load times and user experience.
-
Progress Tracker: The README includes a progress tracker that visually represents the development status of various features. This is a great way to keep contributors and users informed about the project’s progress.
Example of Dynamic Routing
// pages/watch/[animeId].js
import { useRouter } from 'next/router';
const WatchPage = () => {
const router = useRouter();
const { animeId } = router.query;
return <div>Now watching: {animeId}</div>;
};
export default WatchPage;
4. Technical Challenges Overcome
-
Performance Optimization: One of the challenges was ensuring that the application loads quickly, especially given the media-heavy nature of anime streaming. By implementing SSR and optimizing images, the team was able to significantly reduce load times.
-
Handling API Rate Limits: When integrating with external APIs, the team had to manage rate limits effectively. This was achieved by implementing caching strategies to store frequently accessed data, reducing the number of API calls.
-
Responsive Design: Ensuring that the application is usable on various devices was a challenge. The team utilized CSS media queries and NextUI’s responsive components to create a seamless experience across desktops, tablets, and mobile devices.
Example of Responsive Design with CSS
@media (max-width: 768px) {
nav {
flex-direction: column;
}
}
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Framework Utilization: Using Next.js for server-side rendering and static site generation significantly improved the performance and SEO of the application. Understanding the nuances of these features helped in optimizing the user experience.
-
Component Libraries: Integrating NextUI provided a consistent and modern UI design with minimal effort. Learning how to effectively use component libraries can save time and enhance the visual appeal of the application.
-
Version Control: Utilizing Git for version control was crucial for collaboration and tracking changes. It reinforced the importance of committing changes regularly and using branches for feature development.
-
Deployment: Deploying the application on Vercel was straightforward and allowed for easy scaling. Understanding the deployment process and environment variables was essential for a smooth launch.
What Worked Well
-
User Experience: The focus on a simple UI with fast performance resonated well with users. Feedback indicated that the minimalistic design made navigation intuitive.
-
Community Engagement: Encouraging contributions and maintaining an active issues section fostered a sense of community. This led to valuable feedback and improvements from users and developers alike.
-
Documentation: Providing clear installation instructions and a progress tracker helped new contributors understand the project quickly. Good documentation is key to attracting and retaining contributors.
-
Responsive Design: Improvements made for mobile users were well-received, highlighting the importance of responsive design in modern web applications.
What You’d Do Differently
-
Feature Planning: More thorough planning of additional features could have been beneficial. Prioritizing features based on user feedback and usage analytics might have led to a more focused development process.
-
Testing: Implementing a more robust testing strategy (unit tests, integration tests) from the beginning could have helped catch bugs earlier in the development cycle.
-
Performance Monitoring: Setting up performance monitoring tools earlier would have provided insights into user interactions and potential bottlenecks, allowing for proactive optimizations.
-
Code Reviews: Establishing a formal code review process could improve code quality and knowledge sharing among contributors.
Advice for Others
-
Start Simple: Focus on building a minimum viable product (MVP) first. This allows you to gather user feedback early and iterate based on real-world usage.
-
Engage with Your Community: Actively seek feedback and encourage contributions. A strong community can provide support, ideas, and help with development.
-
Prioritize Documentation: Invest time in writing clear and comprehensive documentation. This will make it easier for new contributors to get involved and for users to understand how to use your application.
-
Iterate Based on Feedback: Be open to changing your plans based on user feedback. Regularly review what features are most requested and prioritize those in your development roadmap.
-
Stay Updated: Keep up with the latest trends and updates in the technologies you are using. This can help you leverage new features and improvements that can enhance your project.
What’s Next?
Conclusion: The Future of Animunet
Project Development Analytics
timeline gant
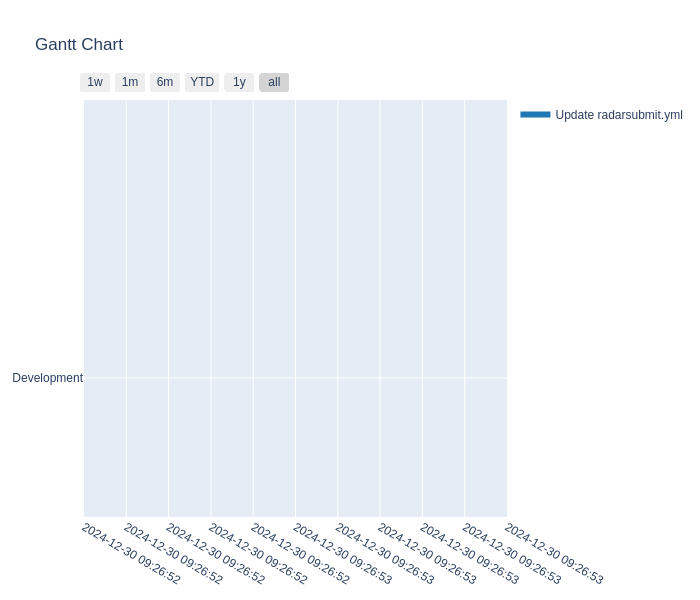
Commit Activity Heatmap
Contributor Network
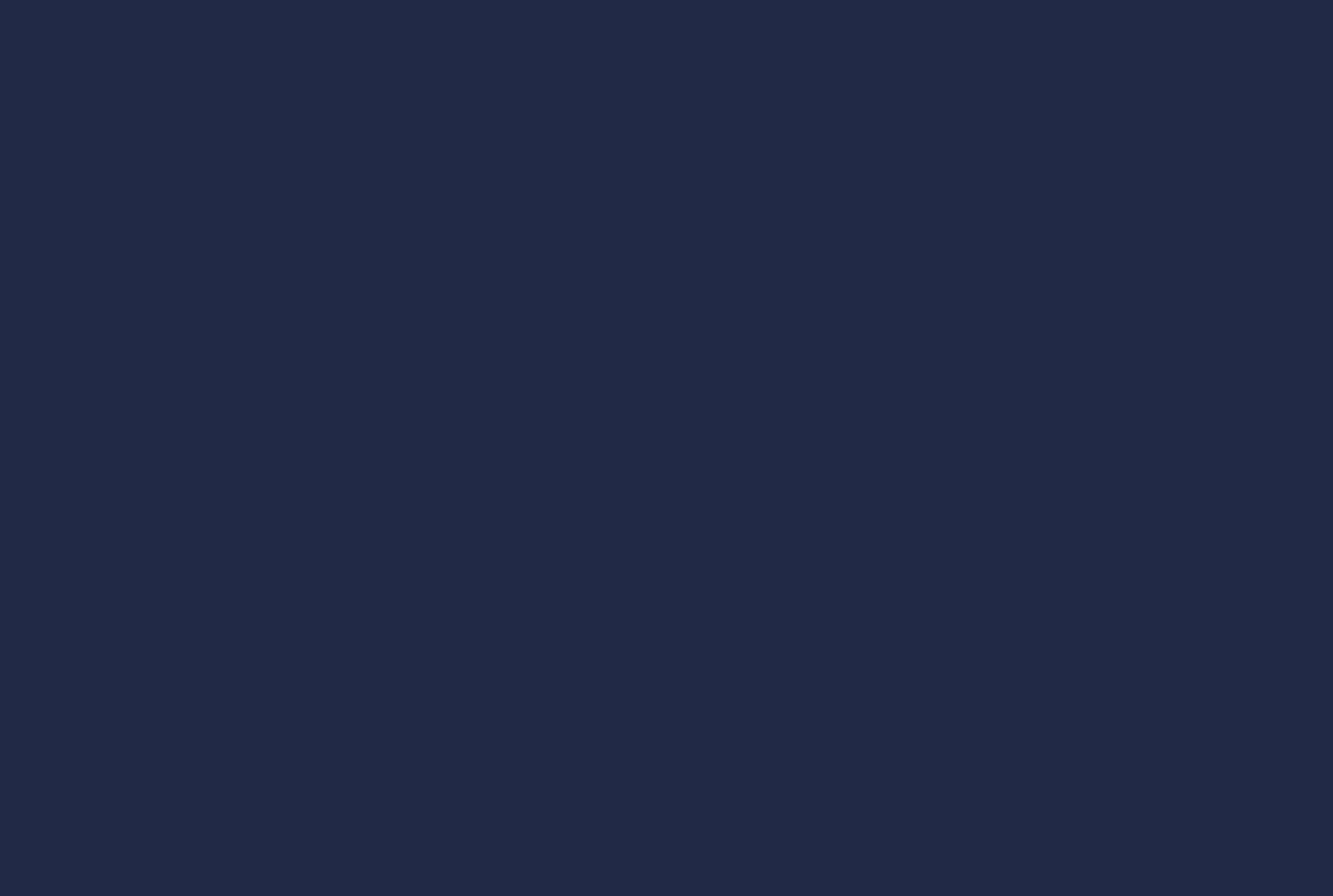
Commit Activity Patterns
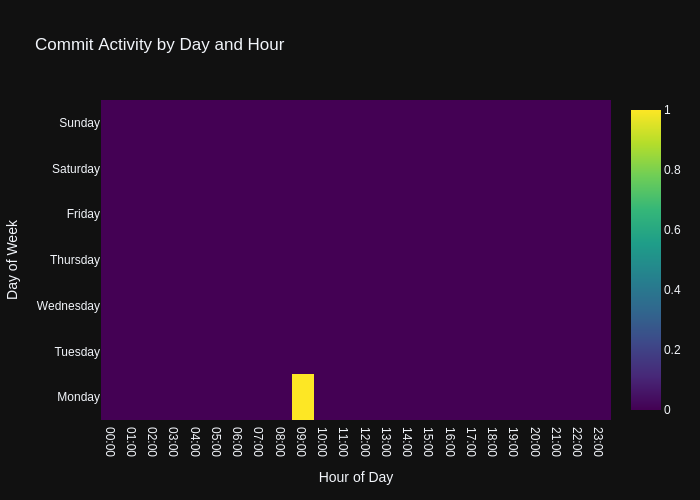
Code Frequency
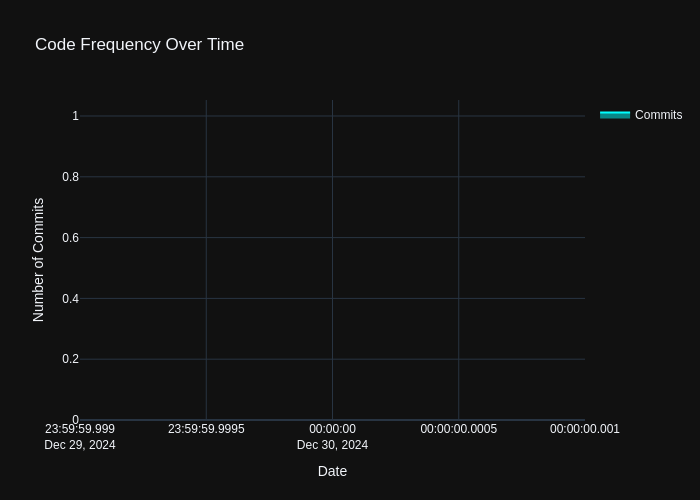
- Repository URL: https://github.com/wanghaisheng/animunet
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日