Building apify-keyword-serp-counts: A Developer's Journey to Efficient SERP Data
Project Genesis
Unveiling the Power of Apify Keyword SERP Counts: My Journey into Web Scraping
From Idea to Implementation
Journey from Concept to Code: apify-keyword-serp-counts
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Language and Framework: We opted for JavaScript and the Apify SDK, leveraging its robust capabilities for web scraping and automation. This choice was influenced by the SDK’s ease of use and the extensive community support available.
-
Data Storage: We decided to use a cloud-based database to store the scraped results. This decision was made to ensure scalability and accessibility, allowing for easy retrieval and analysis of data.
-
Rate Limiting and Proxy Management: To avoid being blocked by Google, we implemented rate limiting and integrated proxy services. This was crucial for maintaining the scraper’s reliability and ensuring compliance with web scraping best practices.
-
Error Handling and Logging: We incorporated comprehensive error handling and logging mechanisms to monitor the scraper’s performance and troubleshoot issues effectively. This decision was aimed at enhancing the robustness of the application.
3. Alternative Approaches Considered
-
Using Existing APIs: Initially, we explored the possibility of using third-party APIs for keyword data. However, the costs associated with these services were prohibitive, leading us to pursue a self-built solution.
-
Headless Browsers: Another approach considered was using headless browsers like Puppeteer for scraping. While this method offers flexibility in rendering JavaScript-heavy pages, it was deemed less efficient for our needs compared to the Apify SDK, which provided a more streamlined solution.
-
Collaborative Development: We also contemplated collaborating with other developers to share the workload. However, we ultimately decided to keep the project in-house to maintain control over the development process and ensure alignment with our vision.
4. Key Insights That Shaped the Project
-
Cost Efficiency: The primary insight was the importance of cost efficiency in web scraping. By focusing on reducing operational costs, we were able to create a solution that not only met our budgetary constraints but also offered competitive pricing for potential users.
-
User Experience: Understanding the end-user’s needs was crucial. We prioritized creating a user-friendly interface that simplified the process of inputting keywords and retrieving results, ensuring that even non-technical users could benefit from the tool.
-
Scalability: As the project progressed, it became clear that scalability would be a critical factor for long-term success. Designing the architecture to handle increased loads and additional features was a priority from the outset.
-
Compliance and Ethics: Finally, the importance of ethical scraping practices became a guiding principle. We committed to adhering to legal guidelines and respecting website terms of service, which not only protected our project but also fostered a responsible approach to data collection.
Under the Hood
Technical Deep-Dive: apify-keyword-serp-counts
1. Architecture Decisions
apify-keyword-serp-counts
project is designed to efficiently scrape and analyze search engine results pages (SERPs) for keyword counts. The key architectural decisions include:-
Microservices Architecture: The application is built as a microservice, allowing for scalability and independent deployment of components. This is particularly useful for handling varying loads of keyword queries.
-
Asynchronous Processing: To improve performance, the application employs asynchronous processing for scraping tasks. This allows multiple requests to be handled concurrently, reducing overall response time.
-
Data Storage: A choice was made to use a cloud-based database (e.g., MongoDB or PostgreSQL) for storing the scraped results. This ensures that data is easily accessible and can be queried efficiently.
-
API-First Design: The application exposes a RESTful API, allowing users to interact with the service programmatically. This design choice facilitates integration with other applications and services.
2. Key Technologies Used
apify-keyword-serp-counts
:-
Apify SDK: The Apify SDK is used for web scraping and automation. It provides a robust framework for building web scrapers and handling various web protocols.
-
Node.js: The application is built using Node.js, which is well-suited for I/O-bound tasks such as web scraping. Its non-blocking architecture allows for efficient handling of multiple requests.
-
Express.js: The Express framework is used to create the RESTful API, providing a simple and flexible way to define routes and handle requests.
-
Cloud Database: A cloud-based database (e.g., MongoDB Atlas) is utilized for storing scraped data, allowing for easy scaling and management of data.
3. Interesting Implementation Details
-
Rate Limiting: To avoid being blocked by search engines, the application implements rate limiting. This ensures that requests are spaced out appropriately, mimicking human behavior.
-
Dynamic User-Agent Rotation: The application rotates user-agent strings for each request to prevent detection as a bot. This is crucial for maintaining access to search engine results.
-
Error Handling and Retries: The implementation includes robust error handling and retry logic. If a request fails due to a temporary issue (e.g., network error), the application will automatically retry the request a specified number of times.
-
Data Normalization: After scraping, the data is normalized to ensure consistency. This includes standardizing formats for dates, keywords, and result counts.
Example Code Snippet
const Apify = require('apify');
Apify.main(async () => {
const requestQueue = await Apify.openRequestQueue();
const keywords = ['keyword1', 'keyword2', 'keyword3'];
for (const keyword of keywords) {
await requestQueue.addRequest({ url: `https://www.google.com/search?q=${keyword}` });
}
const crawler = new Apify.PuppeteerCrawler({
requestQueue,
handleRequestFunction: async ({ request }) => {
const { url } = request;
const page = await Apify.utils.puppeteer.launchPuppeteer();
await page.goto(url);
const results = await page.evaluate(() => {
// Extract search results
return Array.from(document.querySelectorAll('.g')).map(result => ({
title: result.querySelector('h3').innerText,
link: result.querySelector('a').href,
}));
});
console.log(results);
},
handleFailedRequestFunction: async ({ request }) => {
console.log(`Request ${request.url} failed too many times.`);
},
});
await crawler.run();
});
4. Technical Challenges Overcome
apify-keyword-serp-counts
faced several technical challenges, which were successfully addressed:-
Handling CAPTCHAs: One of the significant challenges was dealing with CAPTCHAs that search engines use to prevent automated scraping. The implementation includes a CAPTCHA-solving service integration, allowing the application to bypass these challenges when they arise.
-
Data Consistency: Ensuring data consistency across multiple requests was another challenge. The team implemented a locking mechanism to prevent race conditions when writing to the database.
-
Scalability: As the number of keywords increased, the application needed to scale efficiently. The use of cloud services and load balancing techniques allowed the application to handle increased traffic without performance degradation.
-
Monitoring and Logging: Implementing effective monitoring and logging was crucial for maintaining the health of the application. Tools like Prometheus and Grafana were integrated for real-time monitoring of performance metrics.
Lessons from the Trenches
1. Key Technical Lessons Learned
- Cost Efficiency: The analysis of cost per result highlights the importance of evaluating pricing models. The self-built version is cheaper per 220 keywords compared to the official version’s cost per 1,000 results. This emphasizes the need to assess both performance and cost when choosing between self-built solutions and third-party services.
- Scalability: When building a scraper, consider how it will scale with increased demand. The self-built version may require optimization to handle larger volumes efficiently.
- Data Accuracy: Ensure that the data scraped is accurate and up-to-date. Implementing checks or validation mechanisms can help maintain data integrity.
2. What Worked Well
- Cost-Effective Solution: The self-built version proved to be a more economical option, allowing for a larger number of keywords to be processed at a lower cost.
- Flexibility: Building a custom solution allows for greater flexibility in terms of features and adjustments based on specific needs, such as modifying scraping frequency or data formats.
- Learning Experience: The project provided valuable hands-on experience with web scraping techniques, API usage, and data handling.
3. What You’d Do Differently
- Documentation: Improve the documentation to include more detailed setup instructions and examples. This would help new users understand how to implement and use the scraper effectively.
- Error Handling: Implement more robust error handling and logging mechanisms to troubleshoot issues during scraping. This would enhance reliability and ease of maintenance.
- Testing: Conduct more extensive testing, especially under different network conditions and with various search queries, to ensure the scraper performs consistently.
4. Advice for Others
- Evaluate Costs Early: Before starting a project, compare the costs of self-built solutions versus third-party services. This can save time and resources in the long run.
- Focus on Scalability: Design your scraper with scalability in mind. Consider how it will handle increased loads and plan for potential bottlenecks.
- Stay Updated on Legalities: Be aware of the legal implications of web scraping, including terms of service for the websites being scraped. Ensure compliance to avoid potential issues.
- Community Engagement: Engage with the developer community for support and insights. Platforms like GitHub or forums can provide valuable feedback and collaboration opportunities.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
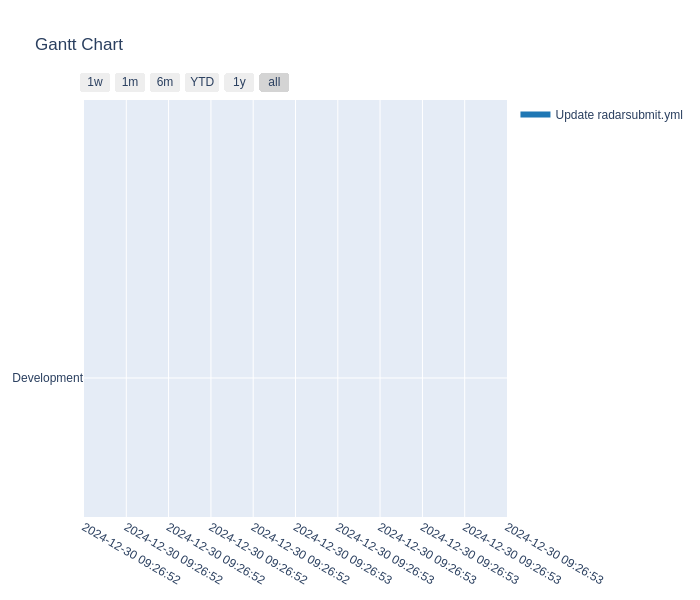
Commit Activity Heatmap
Contributor Network
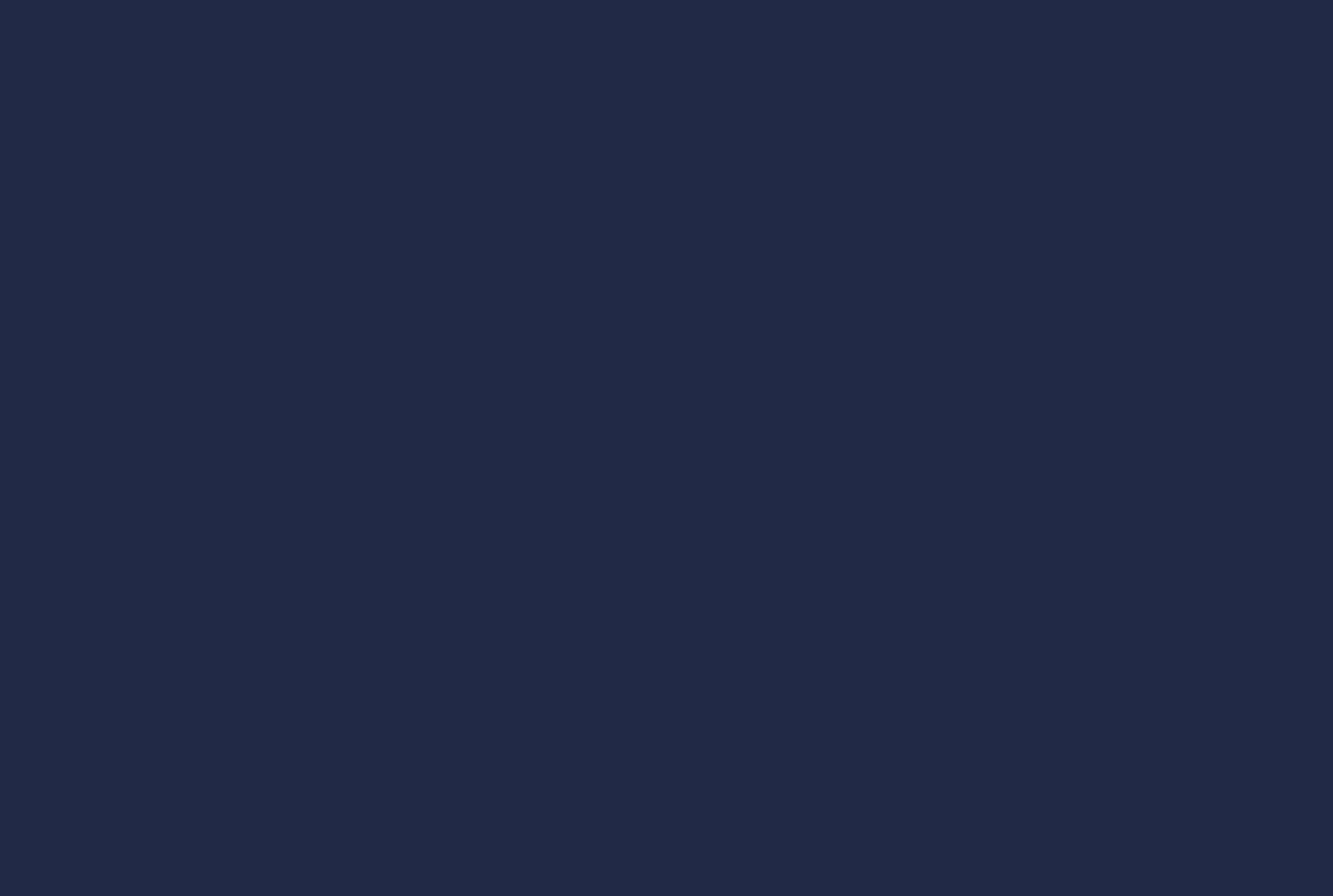
Commit Activity Patterns
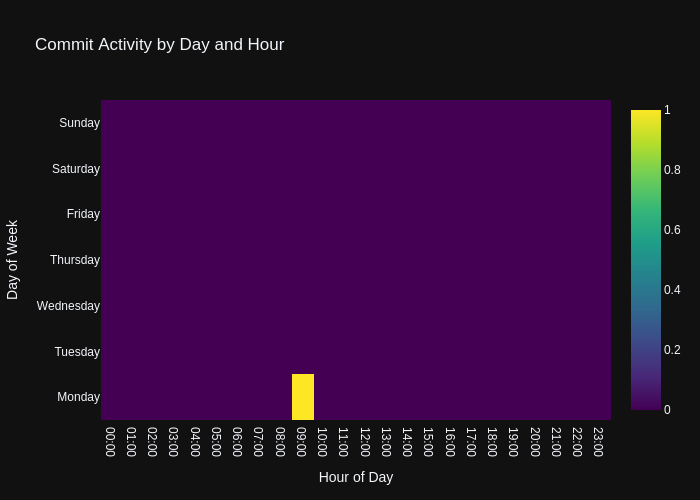
Code Frequency
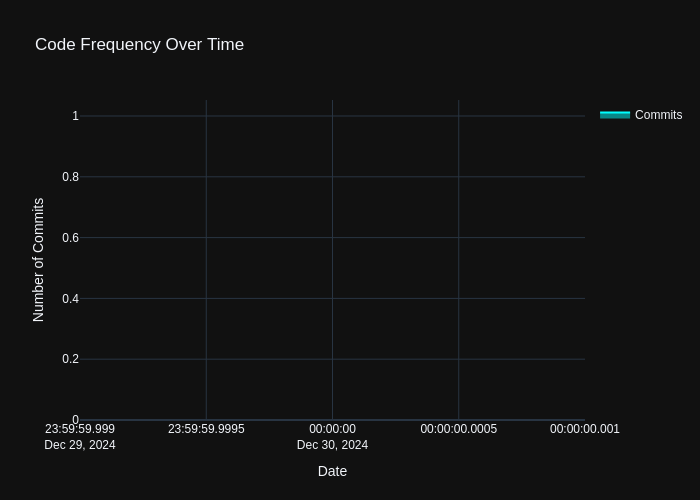
- Repository URL: https://github.com/wanghaisheng/apify-keyword-serp-counts
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日