From Idea to Reality: Building appFeedbackAnalyzer for Insightful App Reviews
Project Genesis
Unleashing the Power of User Feedback: My Journey with AppFeedbackAnalyzer
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
BeautifulSoup
for scraping, Pandas
for data manipulation, and Matplotlib
for visualization were chosen for their robustness and ease of use.3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
src/main.py
file.Conclusion
Under the Hood
Technical Deep-Dive: Feedback Analyzer
1. Architecture Decisions
- Data Collection Layer: This layer is responsible for scraping data from external sources. It utilizes APIs and web scraping techniques to gather user feedback.
- Data Processing Layer: Once the data is collected, it is processed to extract meaningful insights. This includes generating histograms and summarizing feedback.
- Data Storage Layer: The processed data is stored in Excel files for easy access and further analysis.
- User Interface Layer: While the current implementation does not have a graphical user interface, the command-line interface allows users to interact with the application and customize parameters.
2. Key Technologies Used
- Python: The primary programming language used for scripting and data analysis.
- Pandas: A powerful data manipulation library that is used for handling and analyzing data in tabular form.
- Matplotlib: A plotting library used to generate histograms for visualizing feedback data.
- Requests: A library for making HTTP requests to interact with APIs and scrape web content.
- PRAW (Python Reddit API Wrapper): A library that simplifies the process of accessing the Reddit API for collecting posts and comments.
Example of Data Collection with PRAW
import praw
# Initialize Reddit API client
reddit = praw.Reddit(
client_id='your_client_id',
client_secret='your_client_secret',
user_agent='your_user_agent'
)
# Collect recent posts from a subreddit
subreddit = reddit.subreddit('example_subreddit')
for submission in subreddit.new(limit=10):
print(submission.title)
3. Interesting Implementation Details
Example of Setting Up Environment Variables
# .env file content
REDDIT_CLIENT_ID=your_client_id
REDDIT_CLIENT_SECRET=your_client_secret
REDDIT_USER_AGENT=your_user_agent
python-dotenv
package to load these variables at runtime, ensuring that sensitive information is kept secure.4. Technical Challenges Overcome
- API Rate Limiting: When scraping data from Reddit, the application had to handle rate limits imposed by the Reddit API. To overcome this, the implementation includes error handling and retry logic to manage API request failures gracefully.
Example of Handling Rate Limits
import time
def collect_data():
try:
# Attempt to collect data
# ...
except praw.exceptions.APIException as e:
if e.error_type == 'RATELIMIT':
print("Rate limit exceeded. Sleeping for a while...")
time.sleep(60) # Sleep for 60 seconds before retrying
collect_data() # Retry data collection
- Data Cleaning: The collected data often contained noise, such as irrelevant comments or spam. Implementing a data cleaning process was essential to ensure the quality of the insights generated. This involved filtering out non-relevant posts and normalizing text data.
Example of Data Cleaning
def clean_data(data):
# Remove non-relevant posts
cleaned_data = [post for post in data if 'keyword' in post.title.lower()]
return cleaned_data
Lessons from the Trenches
Key Technical Lessons Learned
-
API Integration: Successfully integrating with the Reddit API and scraping the App Store reviews highlighted the importance of understanding API documentation and handling authentication securely. This experience reinforced the need for robust error handling when dealing with external APIs.
-
Data Analysis: Utilizing libraries like Pandas for data manipulation and Matplotlib/Seaborn for visualization proved essential in transforming raw data into meaningful insights. This emphasized the value of data visualization in identifying trends and pain points.
-
Environment Management: Setting up a
.env
file for sensitive information (like API keys) is crucial for maintaining security and flexibility in different environments. This practice prevents hardcoding sensitive data in the codebase.
What Worked Well
-
Rapid Prototyping: The ability to quickly collect and analyze feedback from multiple sources (Reddit and App Store) allowed for rapid iteration and insight generation. The end-to-end process took less than two hours, demonstrating the efficiency of the setup.
-
Visualization: The generation of histograms to visualize pain points was particularly effective. It provided a clear, immediate understanding of user sentiment and areas needing improvement, making it easier to communicate findings to stakeholders.
-
Modularity: The project’s structure, with separate scripts for data collection and analysis, facilitated easier debugging and enhancements. This modularity allowed for straightforward updates and maintenance.
What You’d Do Differently
-
Expand Data Sources: While the initial focus was on Reddit and the App Store, incorporating additional platforms like G2, Trustpilot, and social media could provide a more comprehensive view of customer sentiment. Future iterations should prioritize this expansion.
-
Automated Scheduling: Implementing a scheduling mechanism (e.g., using cron jobs) to automate the data collection process could ensure that insights are continuously updated without manual intervention.
-
User Interface: Developing a simple user interface for non-technical users to input parameters and view results could enhance accessibility and usability, allowing more team members to leverage the tool.
Advice for Others
-
Start Small: If you’re new to data collection and analysis, begin with a single source and gradually expand. This approach allows you to refine your process and understand the nuances of data collection before scaling.
-
Focus on Data Quality: Ensure that the data collected is clean and relevant. Implementing data validation checks during the scraping process can help maintain high-quality datasets.
-
Engage with the Community: Utilize platforms like GitHub to share your project and seek feedback. Engaging with the developer community can provide valuable insights and potential collaborators.
-
Document Everything: Maintain thorough documentation throughout the project. This practice not only aids in onboarding new contributors but also serves as a reference for future enhancements or troubleshooting.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
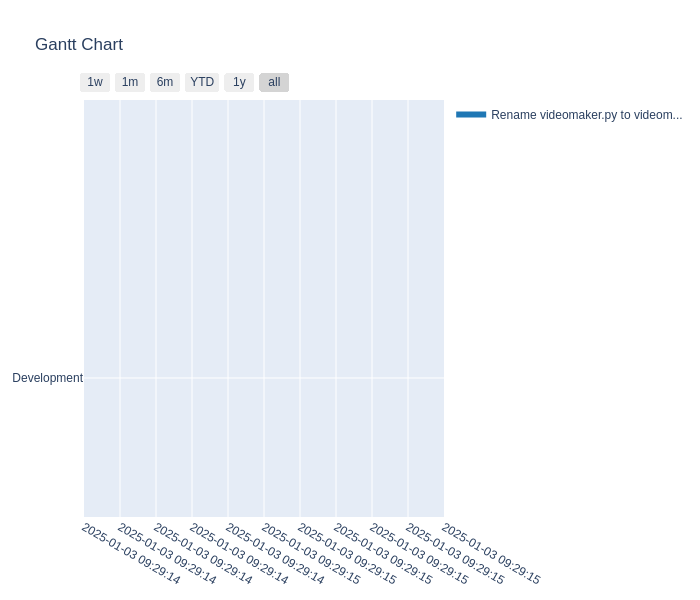
Commit Activity Heatmap
Contributor Network
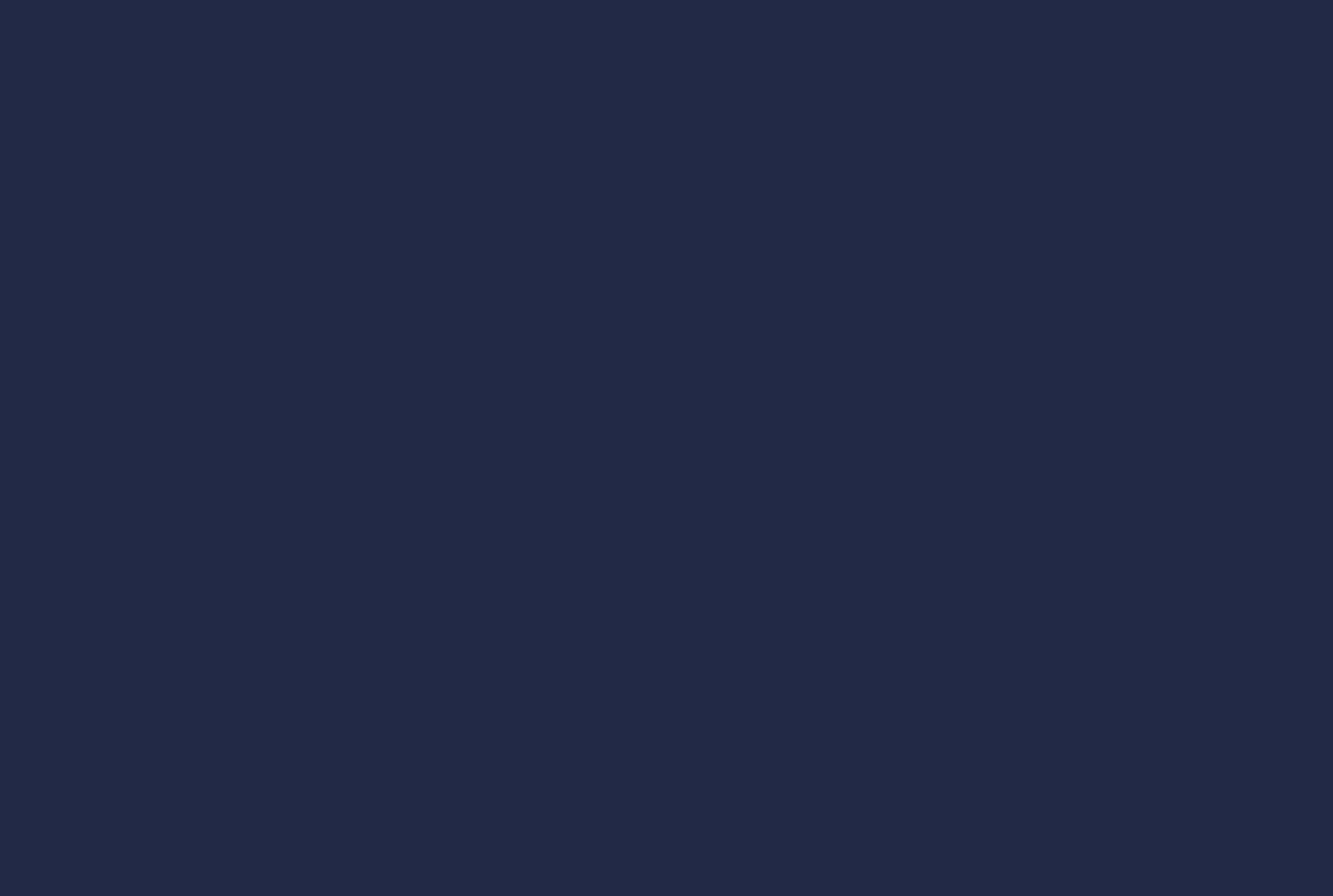
Commit Activity Patterns
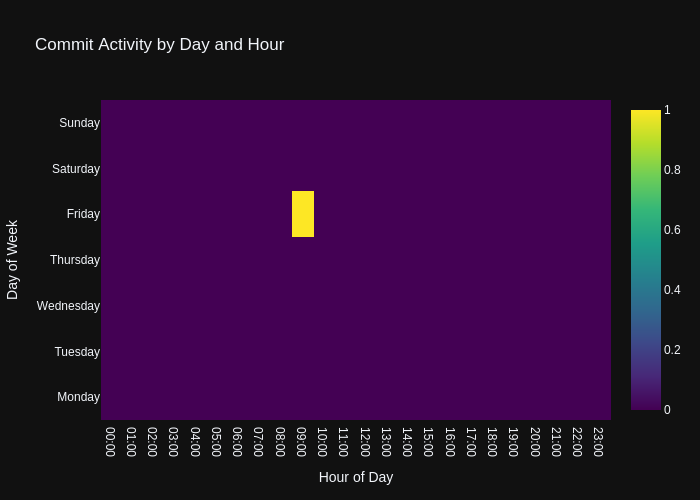
Code Frequency
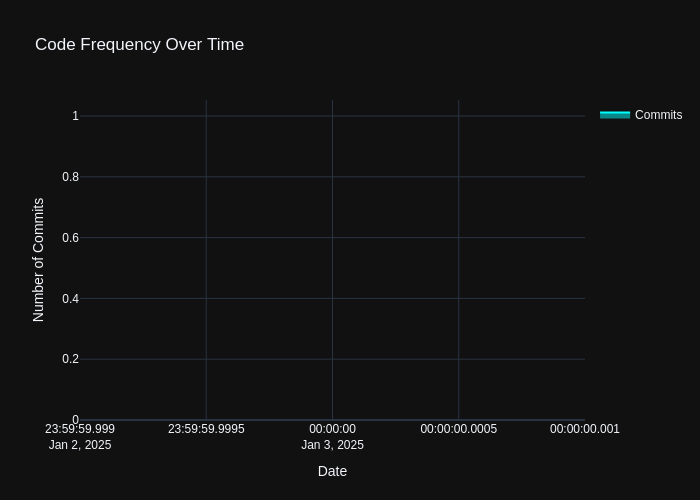
- Repository URL: https://github.com/wanghaisheng/appFeedbackAnalyzer
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日