Weekend Hack: How I Built an Apple Site Clone with Next.js
Project Genesis
Crafting My Own Apple: The Journey of Building an Apple-Site-Clone
app/page.tsx
, each step brought me closer to my vision.From Idea to Implementation
Journey from Concept to Code: A Next.js Project
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
next/font
for font optimization was made to ensure that our application would load quickly and provide a polished aesthetic. By automatically optimizing and loading the Inter font, we could enhance the overall user experience without compromising performance.3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
User-Centric Design: Prioritizing user experience from the outset led to a more intuitive interface. Regular user testing and feedback loops helped refine the design and functionality, ensuring that the application met user needs effectively.
-
Performance Matters: The importance of performance became evident early on. Implementing best practices for optimization, such as code splitting and image optimization, was crucial in delivering a fast and responsive application.
-
Collaboration and Communication: Maintaining open lines of communication among team members facilitated collaboration and allowed for the rapid resolution of issues. Regular stand-ups and code reviews ensured that everyone was aligned and contributed to a cohesive development process.
-
Continuous Learning: The project underscored the value of staying updated with the latest technologies and best practices. Engaging with the Next.js community and exploring its documentation provided valuable insights that informed our development approach.
Under the Hood
Technical Deep-Dive: Next.js Project
1. Architecture Decisions
- Hybrid Rendering: The ability to choose between SSR and SSG on a per-page basis, optimizing load times and user experience.
- File-based Routing: Automatic routing based on the file structure in the
app
directory, simplifying navigation and organization. - API Routes: Built-in support for creating API endpoints, allowing for seamless integration of backend functionality without needing a separate server.
Example of File-based Routing
app/
├── page.tsx
├── about/
│ └── page.tsx
└── api/
└── hello.ts
app/page.tsx
serves as the homepage, while app/about/page.tsx
serves the about page, and app/api/hello.ts
defines an API route.2. Key Technologies Used
- Next.js: The core framework for building the application, providing features like SSR, SSG, and API routes.
- React: The underlying library for building user interfaces, allowing for component-based architecture.
- TypeScript: Used for type safety and better development experience, enhancing code quality and maintainability.
- Vercel: The deployment platform that integrates seamlessly with Next.js, providing features like automatic scaling and serverless functions.
Example of TypeScript Usage
app/page.tsx
, you might see TypeScript interfaces for props:interface Props {
title: string;
}
const HomePage: React.FC<Props> = ({ title }) => {
return <h1>{title}</h1>;
};
export default HomePage;
3. Interesting Implementation Details
next/font
for font optimization. This allows for automatic loading and optimization of fonts, which can significantly improve performance.Example of Font Optimization
app/layout.tsx
, you might see:import { Inter } from 'next/font/google';
const inter = Inter({ subsets: ['latin'] });
export default function Layout({ children }) {
return (
<div className={inter.className}>
{children}
</div>
);
}
4. Technical Challenges Overcome
Challenge: Managing State Across Components
Example of State Management with Context
import React, { createContext, useContext, useState } from 'react';
const AppContext = createContext(null);
export const AppProvider = ({ children }) => {
const [state, setState] = useState({ user: null });
return (
<AppContext.Provider value={{ state, setState }}>
{children}
</AppContext.Provider>
);
};
export const useAppContext = () => useContext(AppContext);
Challenge: SEO Optimization
Example of SEO with Head Component
import Head from 'next/head';
const HomePage = () => {
return (
<>
<Head>
<title>My Next.js App</title>
<meta name="description" content="This is a Next.js application." />
</Head>
<h1>Welcome to My Next.js App</h1>
</>
);
};
Head
component, you can set the title and meta tags for better SEO.Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- File Structure and Routing: Next.js uses a file-based routing system, which simplifies the creation of routes. Understanding how to structure the
app
directory and utilize dynamic routes effectively was crucial. - API Routes: Leveraging Next.js API routes for backend functionality within the same project helped streamline development and reduce the need for a separate backend service for simple tasks.
- Static vs. Server-Side Rendering: Learning when to use static generation (
getStaticProps
) versus server-side rendering (getServerSideProps
) was essential for optimizing performance and SEO. - Font Optimization: Using
next/font
for automatic font optimization improved loading times and user experience, highlighting the importance of performance in web applications.
2. What Worked Well
- Development Experience: The hot-reloading feature in Next.js made the development process smooth and efficient, allowing for immediate feedback on changes.
- Documentation and Community: The extensive documentation and active community support made it easier to troubleshoot issues and learn best practices.
- Deployment with Vercel: Deploying the application on Vercel was seamless, with automatic integration for continuous deployment and easy scaling options.
3. What You’d Do Differently
- State Management: Initially, the project relied on local component state. In hindsight, implementing a state management solution (like Redux or Context API) from the start would have made managing global state more efficient as the application grew.
- Testing: Incorporating testing (unit and integration tests) earlier in the development process would have helped catch bugs sooner and improved code quality.
- Performance Monitoring: Setting up performance monitoring tools (like Google Lighthouse or Sentry) from the beginning would have provided insights into performance bottlenecks and user experience issues.
4. Advice for Others
- Start Small: If you’re new to Next.js, start with a small project to familiarize yourself with its features before scaling up to more complex applications.
- Utilize Built-in Features: Take advantage of Next.js’s built-in features like image optimization, API routes, and static site generation to enhance performance and reduce development time.
- Stay Updated: Next.js is actively developed, so keep an eye on updates and new features that can improve your application.
- Engage with the Community: Participate in forums, GitHub discussions, and local meetups to learn from others and share your experiences.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
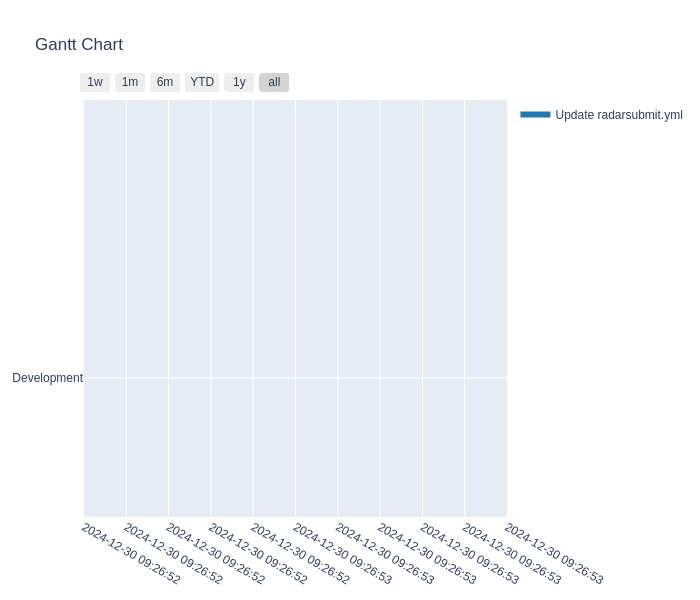
Commit Activity Heatmap
Contributor Network
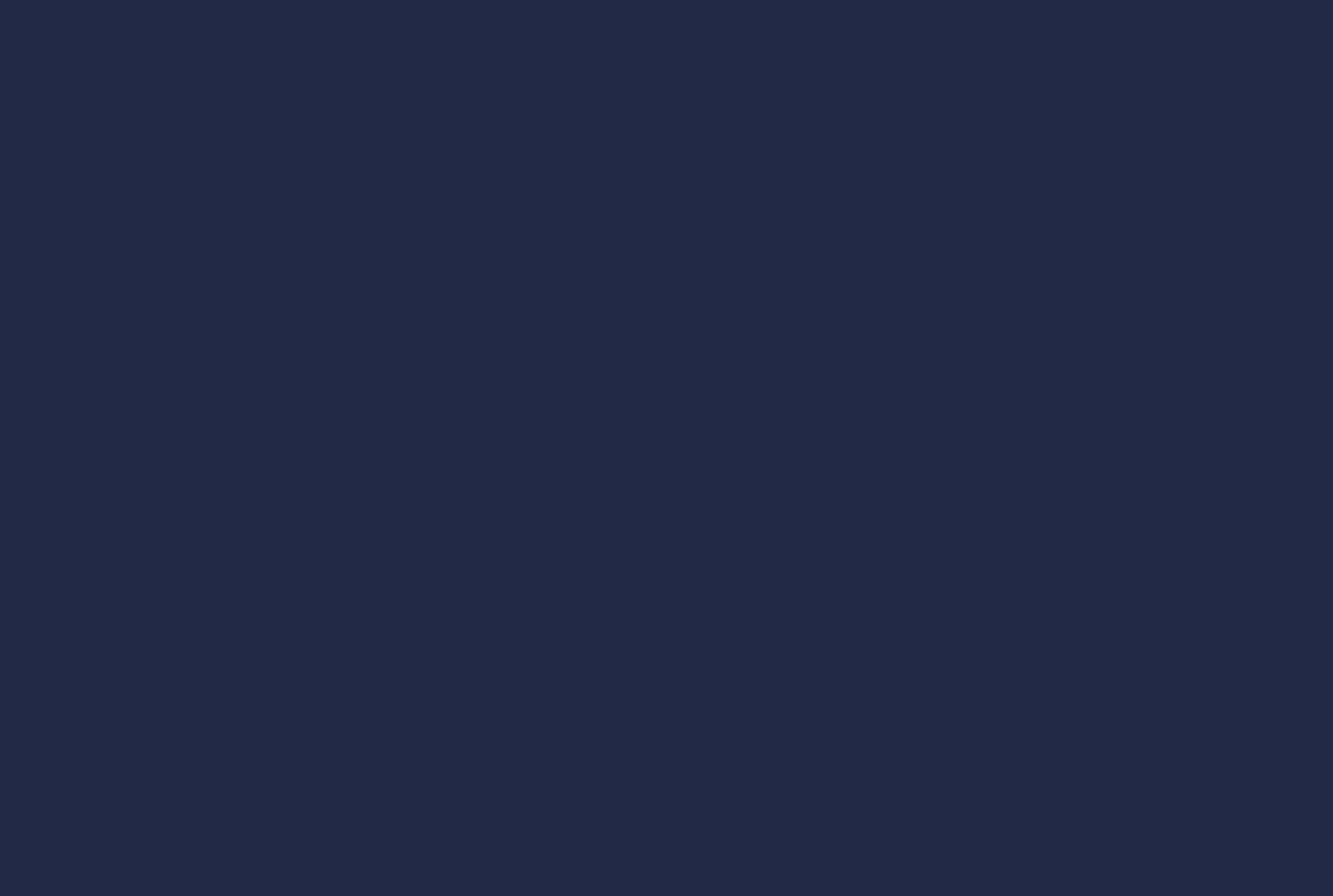
Commit Activity Patterns
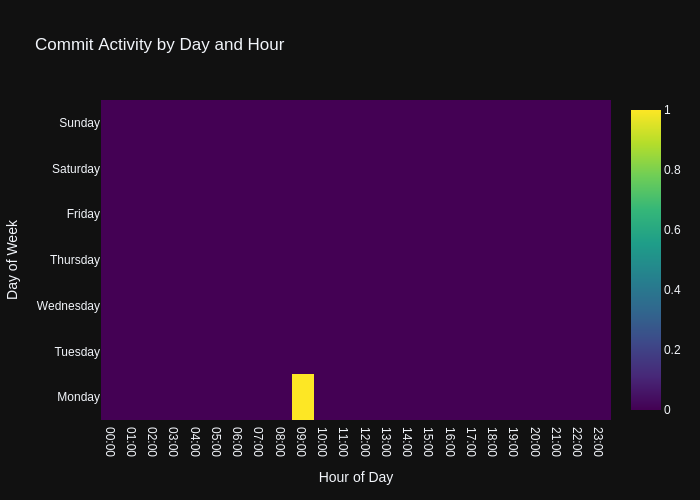
Code Frequency
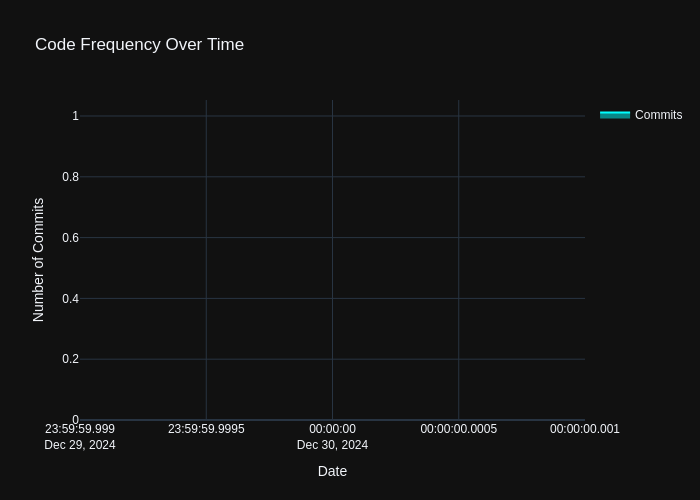
- Repository URL: https://github.com/wanghaisheng/apple-site-clone
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日