Building Astro-HTTP with Turso DB: A Developer's Side Project Journey
Project Genesis
Unleashing the Power of Astro with Turso DB: My Journey into Modern Web Development
From Idea to Implementation
Journey from Concept to Code: Building the Astro Starter Kit: Blog
1. Initial Research and Planning
- User Experience: Ensuring that the blog was easy to navigate and aesthetically pleasing.
- Performance: Prioritizing fast load times and optimal performance metrics, as indicated by tools like Google Lighthouse.
- SEO Optimization: Implementing features that enhance search engine visibility, such as canonical URLs and OpenGraph data.
- Content Management: Facilitating easy content creation and management through Markdown and MDX support.
2. Technical Decisions and Their Rationale
-
Framework Choice: Astro was selected for its ability to deliver high performance and its support for multiple front-end frameworks (React, Vue, Svelte, etc.). This flexibility allows developers to choose their preferred tools while benefiting from Astro’s optimization features.
-
File Structure: The decision to organize the project with a clear directory structure (e.g.,
src/components/
,src/content/
,src/pages/
) was made to enhance maintainability and scalability. This structure allows developers to easily locate and manage different parts of the application. -
Content Collections: Implementing content collections using Astro’s built-in features allowed for efficient management of blog posts. This decision was driven by the need for a robust content management system that could handle Markdown and MDX documents seamlessly.
-
Performance Optimization: The focus on achieving a 100/100 Lighthouse score led to the implementation of best practices in coding and asset management, ensuring that the blog loads quickly and efficiently.
3. Alternative Approaches Considered
-
Using a Full-Stack Framework: Initially, there was consideration of using a full-stack framework like Next.js or Nuxt.js. However, the decision to use Astro was made due to its static site generation capabilities and superior performance for content-focused sites.
-
Custom CMS Development: Another option was to build a custom content management system. However, this was deemed unnecessary given Astro’s existing content collection features, which provided a more efficient solution without the overhead of developing and maintaining a custom CMS.
-
Different Styling Approaches: Various styling frameworks (e.g., Tailwind CSS, Bootstrap) were evaluated. Ultimately, a minimal styling approach was chosen to allow users the freedom to customize the look and feel of their blog without being constrained by predefined styles.
4. Key Insights That Shaped the Project
-
Simplicity is Key: The importance of simplicity in design and functionality became evident. Users appreciate a clean interface that allows them to focus on content creation without unnecessary distractions.
-
Community Feedback: Engaging with the developer community through platforms like Discord provided valuable feedback and insights. This interaction helped refine features and prioritize user needs.
-
Documentation Matters: The realization that comprehensive documentation is crucial for user adoption led to the inclusion of detailed README files and links to further resources. This ensures that users can easily understand and utilize the template.
-
Iterative Development: Emphasizing an iterative development process allowed for continuous improvement based on user feedback and performance metrics. This approach fostered a culture of adaptability and responsiveness to user needs.
Conclusion
Under the Hood
Technical Deep-Dive: Astro Starter Kit - Blog
1. Architecture Decisions
public/
: This directory is for static assets like images, which can be directly served without processing.src/
: Contains the core application code, including components, content, layouts, and pages.astro.config.mjs
: Configuration file for the Astro framework, allowing customization of the build process and project settings.README.md
: Documentation for the project, providing guidance on setup and usage.package.json
: Manages project dependencies and scripts.tsconfig.json
: TypeScript configuration file, enabling type-checking for the project.
.astro
or .md
file in the src/pages/
directory corresponds to a route in the application. This design choice simplifies the routing process and enhances developer experience.2. Key Technologies Used
- Astro: A modern static site generator that allows developers to build fast websites using components from various frameworks (React, Vue, Svelte, etc.).
- Markdown & MDX: For content management, allowing developers to write blog posts in Markdown format while also enabling the use of React components within Markdown files through MDX.
- Lighthouse: A tool for auditing web performance, ensuring that the site achieves optimal performance metrics (100/100 in Lighthouse performance).
- OpenGraph and Canonical URLs: For SEO optimization, ensuring that the site is discoverable and properly indexed by search engines.
3. Interesting Implementation Details
Component Structure
src/components/
directory is where reusable components are stored. For example, a simple button component might look like this:// src/components/Button.astro
---
const { label, onClick } = Astro.props;
---
<button onClick={onClick} class="btn">
{label}
</button>
Content Collections
getCollection()
function:// src/pages/blog.astro
---
import { getCollection } from 'astro:content';
const posts = await getCollection('blog');
---
<ul>
{posts.map(post => (
<li>
<a href={`/blog/${post.slug}`}>{post.title}</a>
</li>
))}
</ul>
src/content/blog/
directory.4. Technical Challenges Overcome
Performance Optimization
<img>
tag with the loading="lazy"
attribute can help defer loading offscreen images:<img src="/path/to/image.jpg" alt="Description" loading="lazy" />
SEO Considerations
---
title: "My Blog Post"
description: "A brief description of my blog post."
canonical: "https://myblog.com/my-blog-post"
---
Markdown and MDX Integration
Lessons from the Trenches
1. Key Technical Lessons Learned
- Astro’s Component System: Understanding how Astro handles components (Astro, React, Vue, etc.) is crucial. The flexibility to use multiple frameworks within the same project allows for a tailored approach to building UI components.
- Content Collections: Utilizing
getCollection()
for managing Markdown and MDX documents is a powerful feature. It simplifies the process of retrieving and displaying blog posts, especially when combined with type-checking for frontmatter. - SEO Optimization: The built-in support for SEO features like canonical URLs and OpenGraph data is a significant advantage. It emphasizes the importance of SEO in modern web development and how frameworks can facilitate this.
2. What Worked Well
- Performance: Achieving a 100/100 Lighthouse performance score is a testament to Astro’s efficiency. This is particularly beneficial for user experience and search engine ranking.
- Minimal Styling: The minimal styling approach allows developers to customize the look and feel of the blog easily. This flexibility is appealing for those who want to create a unique brand identity.
- Documentation and Community Support: The availability of comprehensive documentation and an active Discord community makes it easier for developers to troubleshoot issues and learn best practices.
3. What You’d Do Differently
- Initial Setup: While the setup process is straightforward, providing a more guided tutorial or example project could help beginners get started faster. A step-by-step guide on customizing the blog could enhance the onboarding experience.
- Testing and Quality Assurance: Implementing a more robust testing strategy from the beginning could help catch issues early. Integrating tools for unit testing and end-to-end testing would be beneficial for maintaining code quality.
- Accessibility Considerations: While performance is prioritized, ensuring that the blog is also accessible to users with disabilities should be a focus. Incorporating accessibility checks into the development process would be a valuable addition.
4. Advice for Others
- Leverage the Community: Don’t hesitate to reach out to the Astro community for support. Engaging with others can provide insights and solutions that you might not have considered.
- Iterate on Design: Start with a minimal design and iterate based on user feedback. This approach allows for a more user-centered design process and can lead to a more engaging final product.
- Stay Updated: The web development landscape is constantly evolving. Keep an eye on updates to Astro and related technologies to take advantage of new features and improvements.
- Focus on Content: Ultimately, the success of a blog hinges on its content. Prioritize creating high-quality, engaging posts that resonate with your audience, and use the technical features of Astro to enhance that content.
What’s Next?
Conclusion: Looking Ahead for Astro HTTP Turso DB
Project Development Analytics
timeline gant
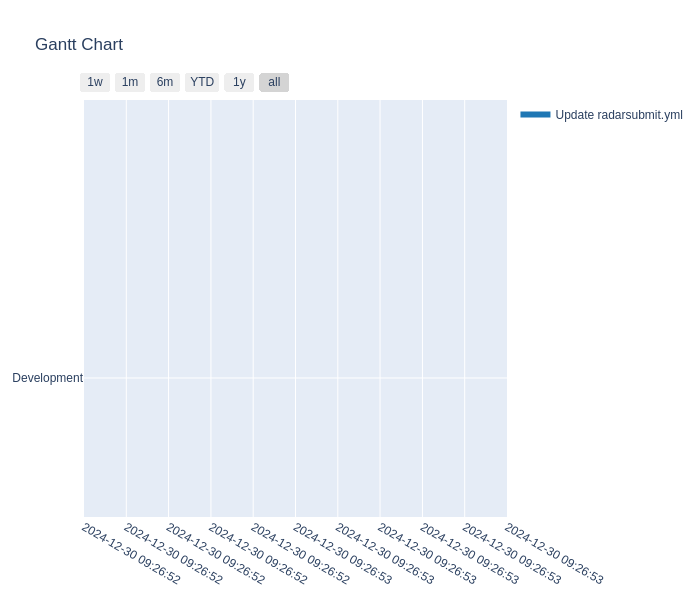
Commit Activity Heatmap
Contributor Network
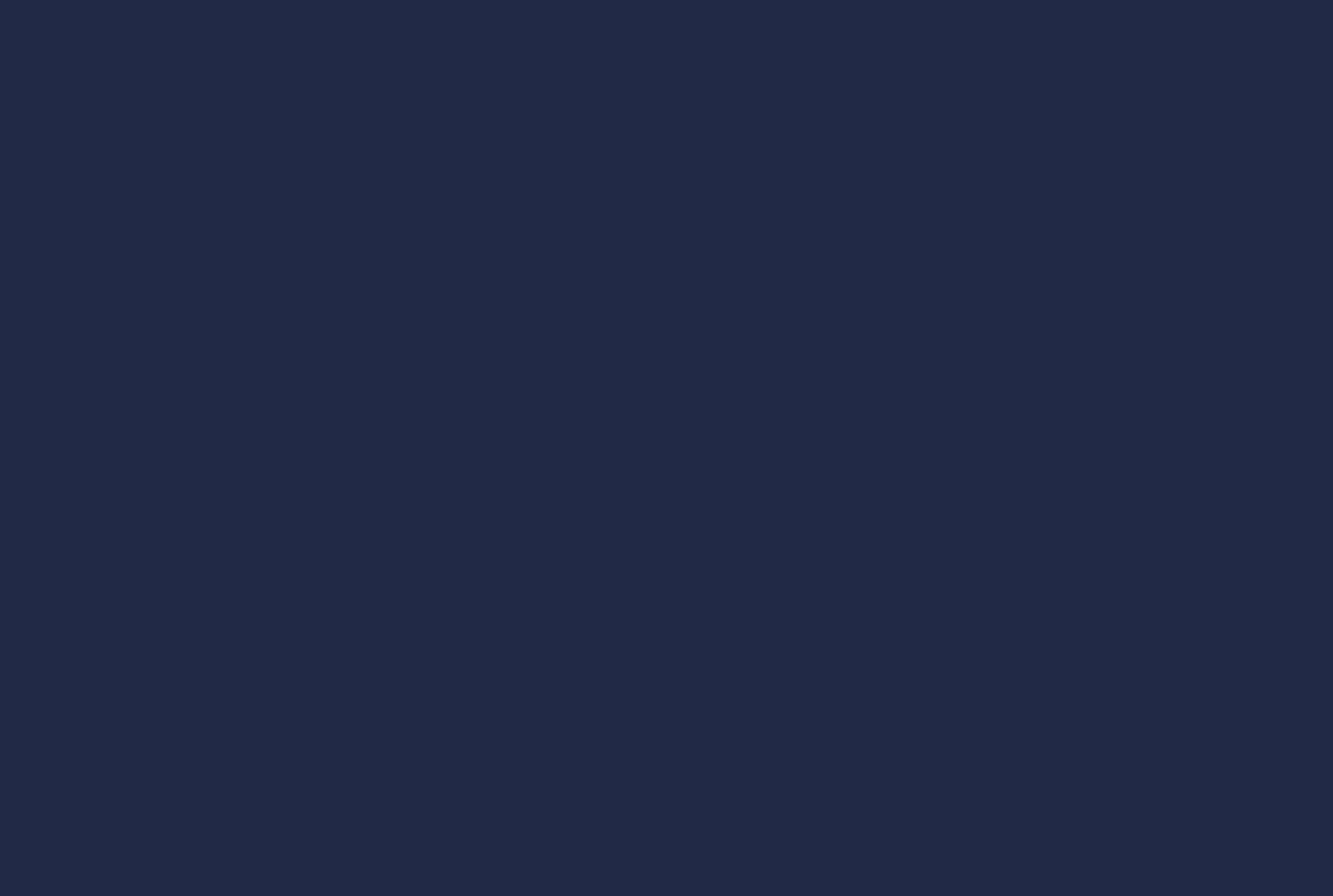
Commit Activity Patterns
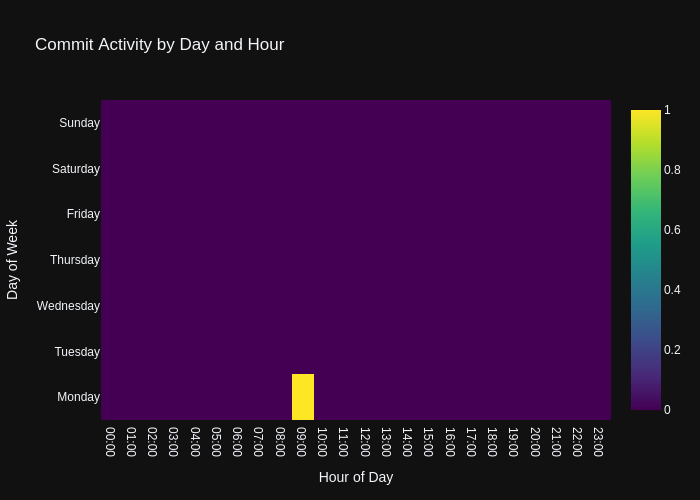
Code Frequency
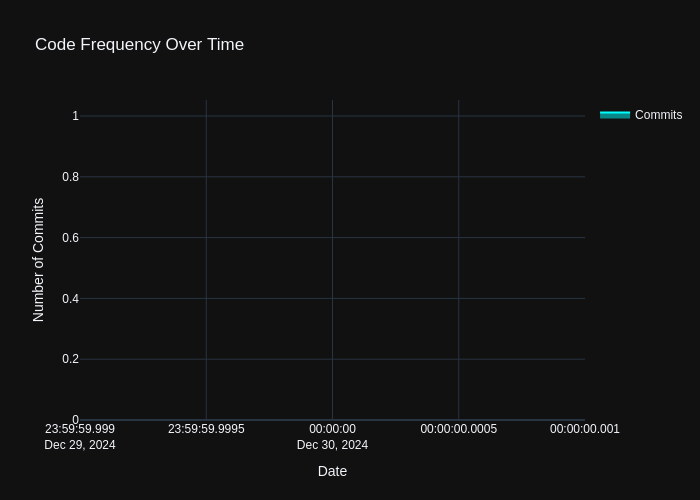
- Repository URL: https://github.com/wanghaisheng/astro-http-turso-db
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日