Building B2B Success: My Journey with Medusa and Next.js
Project Genesis
Medusa B2B Commerce Starter: A Journey into Customizable E-commerce
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of understanding user needs became evident early on. Features like company management and spending limits were directly inspired by user feedback, ensuring that the platform addresses real-world challenges faced by B2B customers.
-
Scalability and Flexibility: The decision to use a headless architecture was reinforced by the realization that B2B businesses often evolve rapidly. The ability to adapt and scale the platform without major overhauls was a critical factor in the design.
-
Community and Support: Engaging with the Medusa and Next.js communities provided valuable resources and support. The availability of documentation, tutorials, and community forums helped the team overcome challenges and implement best practices.
-
Iterative Development: The project adopted an agile methodology, allowing for iterative development and continuous feedback. This approach enabled the team to refine features and make adjustments based on testing and user interactions.
Under the Hood
Technical Deep-Dive: Medusa B2B Commerce Starter
1. Architecture Decisions
Key Architectural Choices:
- Separation of Concerns: The backend (Medusa) and frontend (Next.js) are decoupled, allowing independent development and deployment. This separation enables teams to work on different parts of the application without interfering with each other.
- RESTful API: Medusa exposes a RESTful API that the Next.js storefront consumes. This design choice facilitates easy integration with other services and allows for a more flexible frontend.
- Database Management: PostgreSQL is used as the database, providing robust data management capabilities and support for complex queries.
2. Key Technologies Used
- Medusa 2.0: An open-source headless commerce engine that provides essential e-commerce features such as product management, order processing, and user authentication.
- Next.js 15: A React-based framework that enables server-side rendering, static site generation, and API routes, enhancing performance and SEO.
- PostgreSQL 15: A powerful relational database that stores application data, ensuring data integrity and supporting complex transactions.
- Yarn: A package manager that simplifies dependency management and project setup.
3. Interesting Implementation Details
Company Management Feature
// In Medusa's backend
medusaRouter.post("/companies/:company_id/invite", async (req, res) => {
const { email } = req.body;
const companyId = req.params.company_id;
// Logic to send an invitation email
await sendInvitationEmail(email, companyId);
res.status(200).send({ message: "Invitation sent!" });
});
Bulk Add-to-Cart Feature
const handleBulkAddToCart = async (variants) => {
const response = await fetch('/api/cart/bulk-add', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ variants }),
});
if (response.ok) {
// Update cart state
const updatedCart = await response.json();
setCart(updatedCart);
}
};
4. Technical Challenges Overcome
Database Migration and Seeding
yarn medusa db:migrate
Handling User Authentication
const jwt = require('jsonwebtoken');
const token = jwt.sign({ userId: user.id }, process.env.JWT_SECRET, {
expiresIn: '1h',
});
Performance Optimization
export const getServerSideProps = async () => {
const res = await fetch('https://api.example.com/products');
const products = await res.json();
// Cache the response
cache.set('products', products);
return { props: { products } };
};
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Integration of Technologies: Successfully integrating Medusa 2.0 with Next.js 15 showcased the importance of understanding how different technologies can work together to create a seamless eCommerce experience. The use of server components and static pre-rendering in Next.js significantly improved performance.
-
Database Management: The setup process for the database using Medusa’s built-in commands (like
medusa db:create
andmedusa db:migrate
) highlighted the importance of having a robust database management strategy. It’s crucial to ensure that migrations are handled properly to avoid data inconsistencies. -
Environment Configuration: The use of environment variables for sensitive information (like API keys) is a best practice that enhances security. This project reinforced the need for a clear and organized way to manage these configurations.
-
User Role Management: Implementing features like company management and spending limits required a solid understanding of user roles and permissions. This is essential for B2B applications where different users have varying levels of access.
What Worked Well
-
Feature-Rich Functionality: The inclusion of features such as quote management, bulk add-to-cart, and promotions provided a comprehensive B2B eCommerce solution. These features were well-received and added significant value to the platform.
-
User Experience: The design and layout of the storefront, including the cart summary and product pages, were intuitive and user-friendly. This contributed to a positive user experience, which is critical in eCommerce.
-
Community Engagement: The project’s integration with Discord for community support and the encouragement of contributions through PRs fostered a collaborative environment. This helped in gathering feedback and improving the project continuously.
What You’d Do Differently
-
Documentation: While the README is informative, more detailed documentation on advanced features and troubleshooting could be beneficial. Including a FAQ section or common issues encountered during setup could help new users.
-
Testing: Implementing a more robust testing strategy, including unit and integration tests, would ensure that new features do not break existing functionality. This is especially important in a B2B context where reliability is paramount.
-
Performance Optimization: While the project performs well, continuous monitoring and optimization of performance, especially as the user base grows, should be a priority. This could include optimizing database queries and improving caching strategies.
Advice for Others
-
Start Small: If you’re new to building eCommerce platforms, start with a smaller project to understand the core concepts before diving into a full-fledged B2B solution. This will help you grasp the complexities involved.
-
Leverage Community Resources: Engage with the community through forums, Discord, or GitHub discussions. Learning from others’ experiences can save you time and help you avoid common pitfalls.
-
Focus on Security: Always prioritize security, especially when handling sensitive user data. Implement best practices for authentication, authorization, and data protection.
-
Iterate Based on Feedback: Regularly seek feedback from users and stakeholders. Use this feedback to iterate on your product, ensuring it meets the needs of your target audience.
-
Stay Updated: Keep an eye on updates to the technologies you are using (like Medusa and Next.js). New features and improvements can significantly enhance your project and provide better performance and security.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
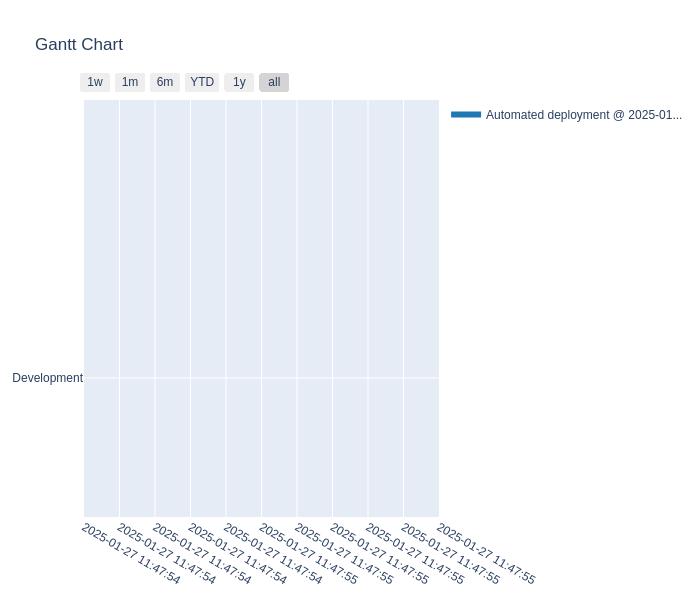
Commit Activity Heatmap
Contributor Network
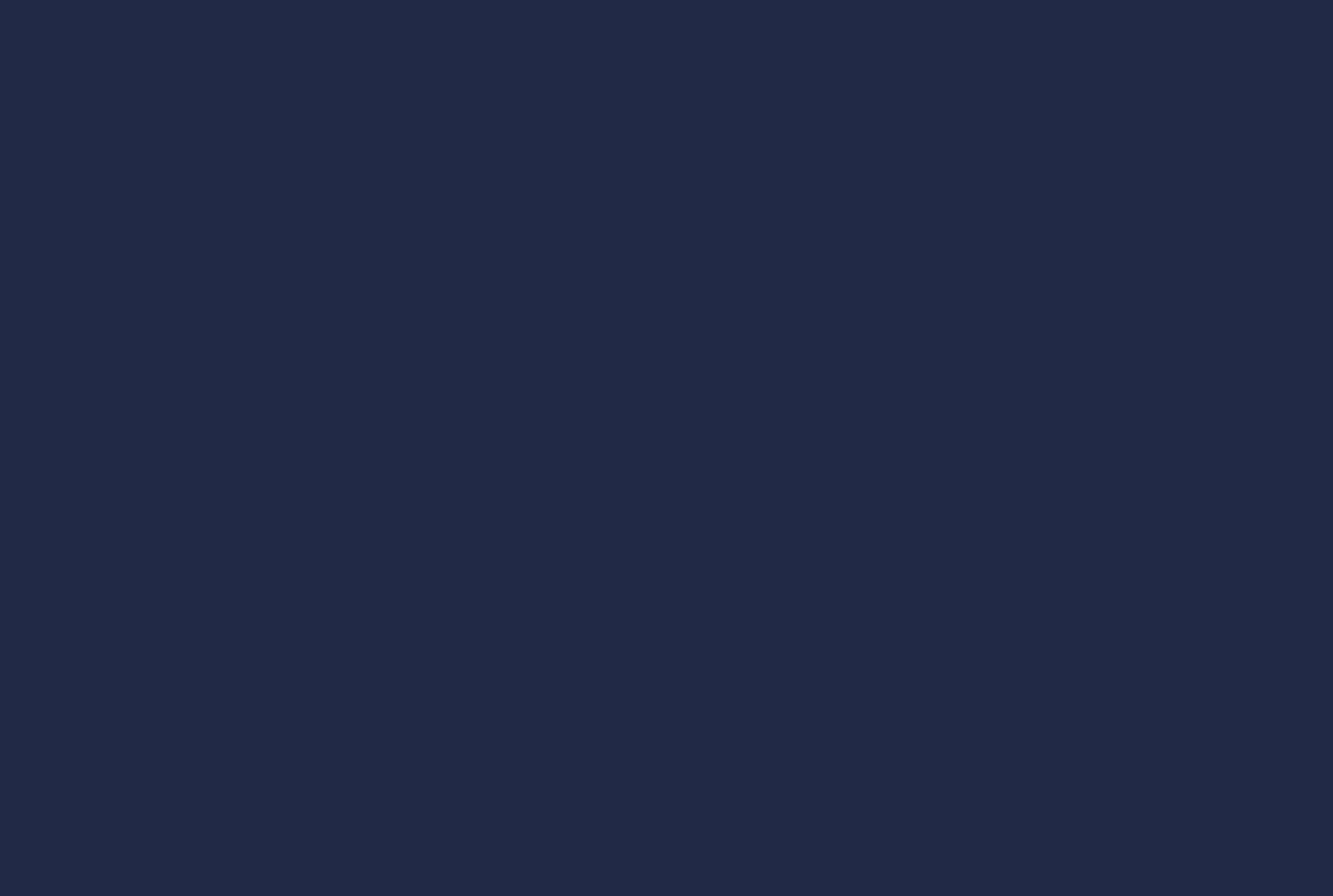
Commit Activity Patterns
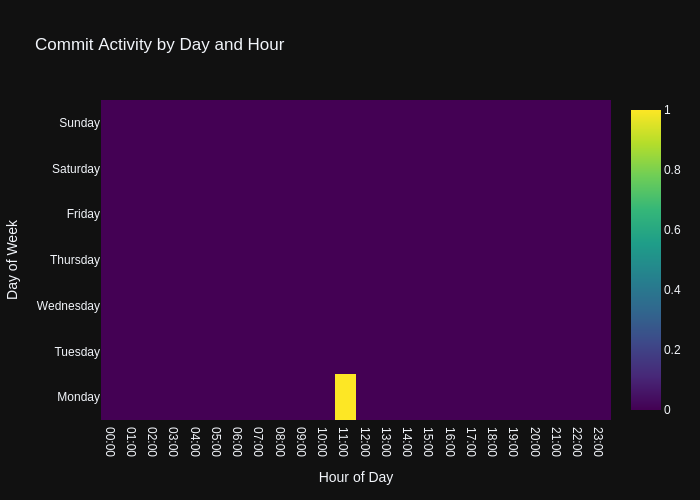
Code Frequency
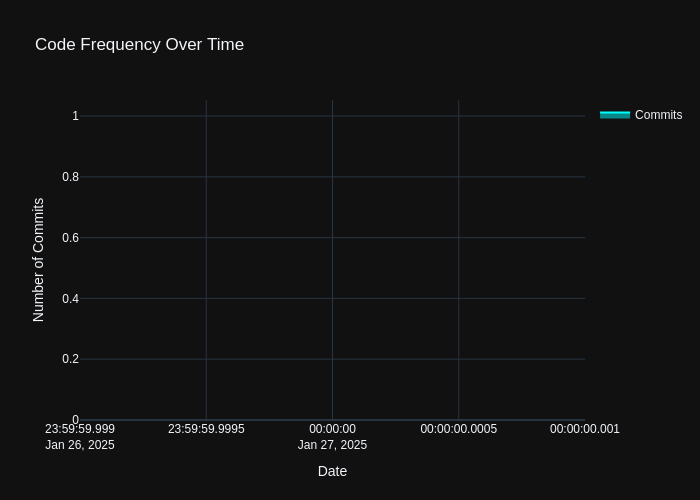
- Repository URL: https://github.com/wanghaisheng/b2b-starter-medusa
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 2 月 3 日