Weekend Hack: How I Built best-GifConverter for Effortless GIF Creation
Project Genesis
From Idea to Implementation
Journey from Concept to Code: MF-GifConverter
1. Initial Research and Planning
- User-Friendly Interface: A clean and intuitive design that allows users to easily navigate the application.
- Multiple Input Formats: Support for various file types, including images (JPEG, PNG), videos (MP4, AVI), and screen recordings.
- Customization Options: Features that allow users to adjust the GIF output, such as frame rate, resolution, and looping options.
- Performance Optimization: Ensuring that the conversion process is efficient and quick, even for larger files.
2. Technical Decisions and Their Rationale
-
Framework Selection: We chose to use a combination of HTML, CSS, and JavaScript for the front-end, leveraging libraries like React for building a responsive user interface. This decision was based on React’s component-based architecture, which allows for reusable UI components and easier state management.
-
Backend Services: For the conversion process, we opted for a serverless architecture using cloud functions. This approach minimizes server maintenance and scales automatically based on user demand. We integrated third-party APIs for video processing and GIF generation, which allowed us to focus on the front-end experience without getting bogged down in complex backend logic.
-
Caching Mechanism: To enhance performance, we implemented a caching strategy that stores frequently accessed data and user settings. This decision was crucial in reducing load times and improving the overall user experience.
3. Alternative Approaches Considered
-
Desktop Application: Initially, we contemplated creating a desktop application instead of a web-based tool. However, we recognized that a web application would provide greater accessibility, allowing users to convert files from any device without the need for installation.
-
Open Source Libraries: We explored using open-source libraries for GIF conversion. While this could have reduced development time, we ultimately decided to use established APIs to ensure reliability and support for a wider range of file formats.
-
Single Page Application (SPA): While we initially considered a multi-page application for different functionalities, we ultimately decided on a single-page application (SPA) approach. This decision was made to provide a smoother user experience, allowing for quick transitions between different features without full page reloads.
4. Key Insights That Shaped the Project
-
User Feedback is Crucial: Early user testing revealed that users valued customization options more than we initially anticipated. This feedback led to the addition of features like adjustable frame rates and resolution settings, which enhanced user satisfaction.
-
Performance Matters: As we tested the application, we realized that performance optimization was critical. Users expect quick results, especially when dealing with media files. This insight drove our decision to implement caching and optimize the conversion process.
-
Aesthetic Appeal: The importance of a visually appealing interface became evident during the design phase. Users are more likely to engage with an application that is not only functional but also aesthetically pleasing. This realization prompted us to invest time in creating a polished and modern design, including the addition of theme-switching animations in version 3.0.0.
Under the Hood
Technical Deep-Dive: MF-GifConverter
1. Architecture Decisions
Key Architectural Components:
- Frontend: Built using HTML, CSS, and JavaScript, the frontend is responsible for user interactions, file uploads, and displaying the converted GIFs.
- Backend: The backend processes the uploaded media files and performs the conversion to GIF format. This could be implemented using a server-side language like Node.js or Python, utilizing libraries for media processing.
- Caching: The application implements caching mechanisms to improve performance and reduce load times for frequently accessed resources.
2. Key Technologies Used
- HTML/CSS/JavaScript: The core technologies for building the user interface. JavaScript frameworks like React or Vue.js could be used to enhance interactivity.
- FFmpeg: A powerful multimedia framework that can decode, encode, transcode, mux, demux, stream, filter, and play almost anything that humans and machines have created. It is likely used in the backend for converting media files to GIF.
- Web APIs: The application may utilize various web APIs for file handling, such as the File API for reading files and the Fetch API for making network requests.
- Local Storage: For caching purposes, the application may use the browser’s local storage to save user preferences and recently converted files.
3. Interesting Implementation Details
File Upload and Conversion Process
<input type="file">
element to allow users to select files. Upon selection, JavaScript can read the file and send it to the server for processing.const fileInput = document.getElementById('fileInput');
fileInput.addEventListener('change', (event) => {
const file = event.target.files[0];
const formData = new FormData();
formData.append('file', file);
fetch('/upload', {
method: 'POST',
body: formData,
})
.then(response => response.json())
.then(data => {
console.log('Success:', data);
})
.catch((error) => {
console.error('Error:', error);
});
});
Theme Switching Animation
body {
transition: background-color 0.5s ease;
}
.dark-theme {
background-color: #333;
color: #fff;
}
4. Technical Challenges Overcome
Media Processing Performance
const worker = new Worker('converterWorker.js');
worker.postMessage(file);
worker.onmessage = function(event) {
const gifUrl = event.data;
// Display the converted GIF
};
Cross-Browser Compatibility
Error Handling
fetch('/upload', {
method: 'POST',
body: formData,
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.catch((error) => {
alert('There was a problem with your upload: ' + error.message);
});
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- Code Refactoring: The importance of refactoring code to improve maintainability and readability cannot be overstated. It allows for easier updates and debugging in the future.
- User Experience (UX) Design: Implementing theme switching and optimizing interface interactions significantly enhances user satisfaction. Understanding user needs and preferences is crucial for any application.
- Performance Optimization: Adding page caching can greatly improve load times and overall performance, especially for applications that handle media processing.
- Cross-Platform Compatibility: Ensuring that the application works seamlessly across different devices and browsers is essential, particularly for web-based tools.
2. What Worked Well
- Feature Implementation: The addition of new features like theme switching and camera recording optimization was well-received, indicating that user feedback was effectively integrated into the development process.
- Visual Design: The inclusion of screenshots in the README provides a clear visual representation of the application, making it easier for potential users to understand its functionality.
- Documentation: The README is concise and informative, which helps users quickly grasp the purpose and capabilities of the tool.
3. What You’d Do Differently
- User Testing: Conducting more extensive user testing before major releases could provide valuable insights into usability issues that may not be apparent during development.
- Versioning and Changelog: While the changelog is a good start, adopting a more structured versioning system (like Semantic Versioning) could help users understand the impact of updates more clearly.
- Community Engagement: Building a community around the project (e.g., through forums or social media) could foster user engagement and provide a platform for feedback and feature requests.
4. Advice for Others
- Prioritize User Feedback: Always seek and incorporate user feedback into your development process. It can guide feature development and help identify pain points.
- Focus on Performance: Optimize your application for performance from the start. Users are more likely to adopt tools that are fast and responsive.
- Maintain Clear Documentation: Keep your documentation up to date and clear. This not only helps users but also aids in onboarding new contributors to the project.
- Iterate and Improve: Embrace an iterative development approach. Regularly update your application based on user needs and technological advancements.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
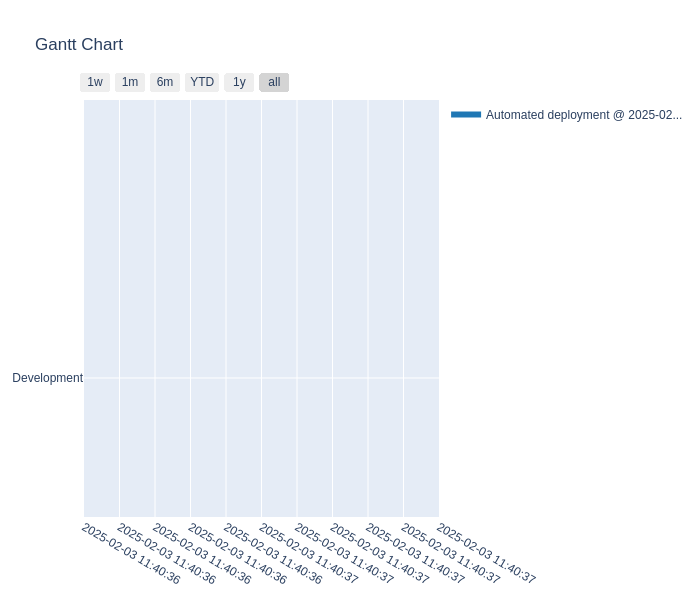
Commit Activity Heatmap
Contributor Network
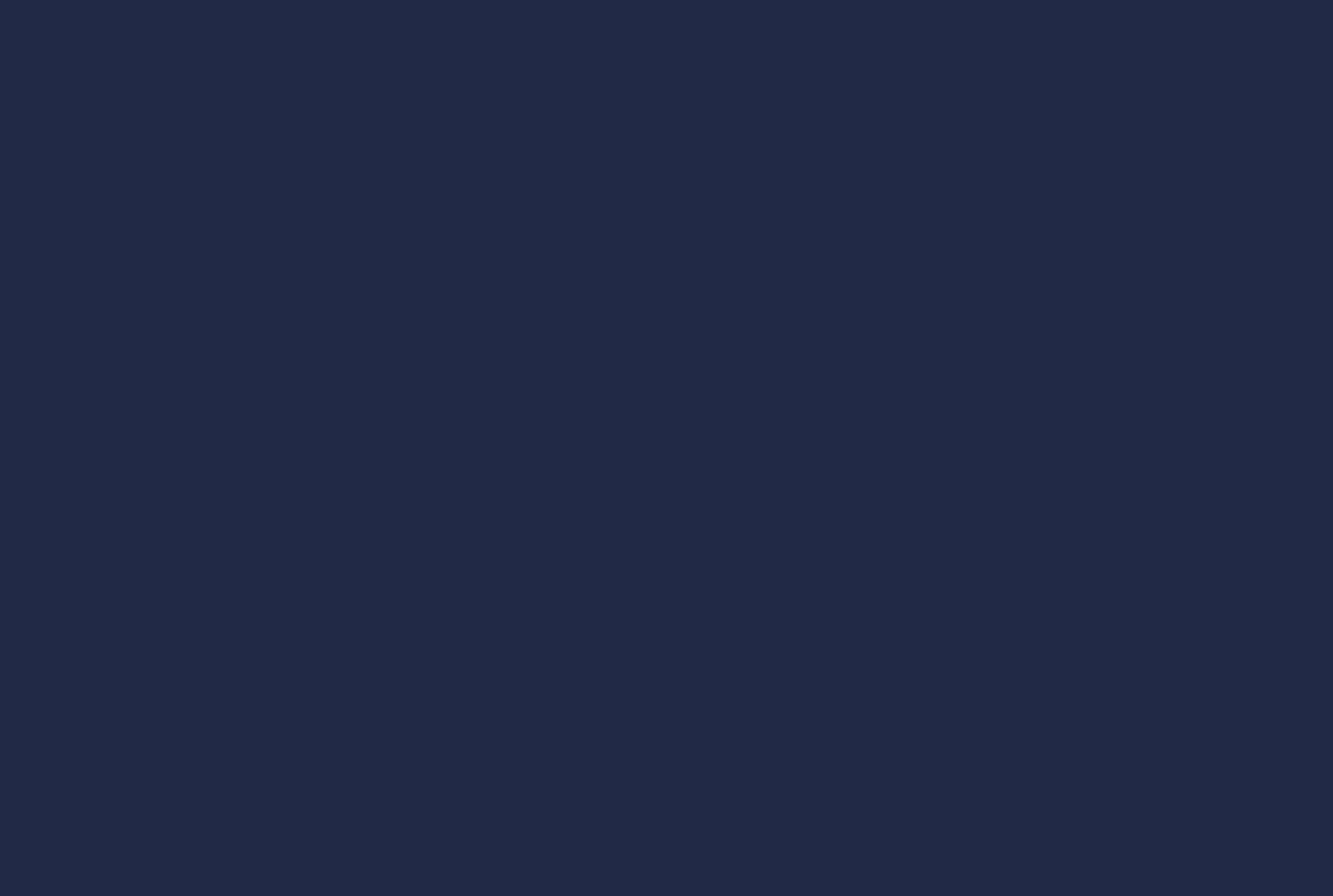
Commit Activity Patterns
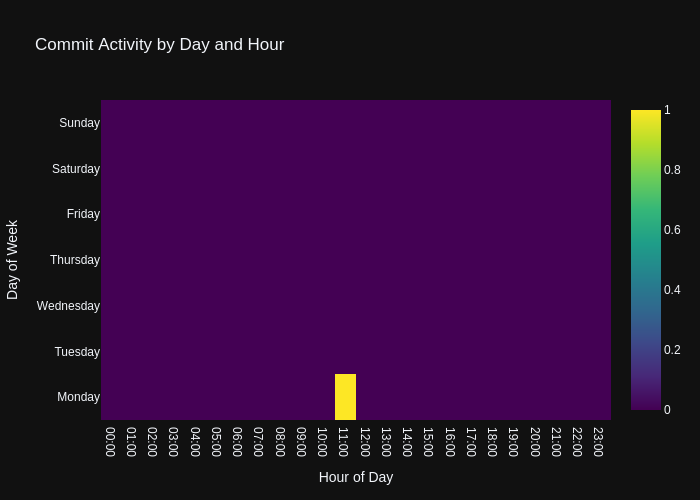
Code Frequency
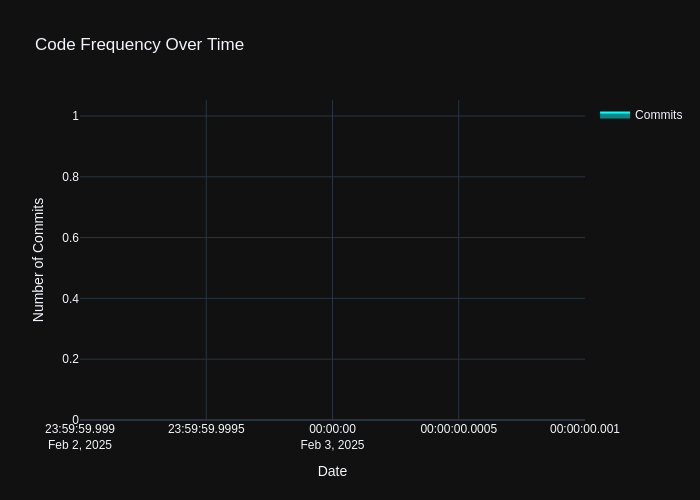
- Repository URL: https://github.com/wanghaisheng/best-GifConverter
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 2 月 17 日