From Idea to Reality: Building bestfreeai - Your AI Tools Directory
Project Genesis
Discovering the Power of AI: My Journey with Tap4 AI Web UI
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of a user-friendly interface became evident early on. Continuous user feedback led to iterative design changes that improved navigation and accessibility.
-
Community Engagement: The decision to make the project open-source was reinforced by the realization that community contributions could enhance the platform’s capabilities and foster a sense of ownership among users.
-
Scalability and Flexibility: The need for a scalable solution became apparent as the project evolved. The choice of a serverless architecture and modular design allowed the team to adapt quickly to changing requirements and user needs.
-
SEO and Discoverability: The significance of SEO in ensuring the platform’s visibility was a recurring theme. Implementing SEO-friendly practices from the outset helped position the project for success in a competitive landscape.
Under the Hood
Technical Deep-Dive: Open Source Tap4 AI Web UI
1. Architecture Decisions
-
Microservices Approach: The application is structured to separate concerns, with the front-end UI and back-end services (like the Supabase database and the Tap4 AI crawler) operating independently. This allows for easier updates and scalability.
-
Server-Side Rendering (SSR): Utilizing Next.js, the application benefits from server-side rendering, which improves SEO and performance by pre-rendering pages on the server before sending them to the client.
-
Internationalization (i18n): The architecture supports multiple languages, making it accessible to a broader audience. This is achieved through Next.js’s built-in internationalization features.
-
Dynamic Content Generation: The integration with Supabase allows for dynamic content generation based on user interactions and database queries, enabling a responsive user experience.
2. Key Technologies Used
-
Next.js: A React framework that enables server-side rendering and static site generation. The project uses Next.js 14, which includes features like app routing and React server components.
-
Supabase: An open-source Firebase alternative that provides a serverless database solution. It is used to store AI tool data and manage user submissions.
-
Tailwind CSS: A utility-first CSS framework that allows for rapid UI development with a focus on responsiveness and customization.
-
Vercel: The deployment platform that supports serverless functions and scheduled tasks, allowing for automated data fetching and submission.
Example of Next.js Page Component
import { useEffect, useState } from 'react';
import { supabase } from '../utils/supabaseClient';
const ToolList = () => {
const [tools, setTools] = useState([]);
useEffect(() => {
const fetchTools = async () => {
const { data } = await supabase.from('tools').select('*');
setTools(data);
};
fetchTools();
}, []);
return (
<div>
<h1>AI Tools Directory</h1>
<ul>
{tools.map(tool => (
<li key={tool.id}>{tool.name}</li>
))}
</ul>
</div>
);
};
export default ToolList;
3. Interesting Implementation Details
-
Dynamic Sitemap Generation: The application generates a dynamic
sitemap.xml
that updates based on the content in the Supabase database. This is crucial for SEO, as it helps search engines index the site effectively. -
Environment Variable Management: The project uses environment variables to manage sensitive information and configuration settings. This includes API keys and database URLs, which are essential for connecting to Supabase and the crawler API.
Example of Environment Variable Configuration
# .env.local
NEXT_PUBLIC_SITE_URL="https://tap4.ai"
NEXT_PUBLIC_SUPABASE_URL="https://xxxyyyzzz.supabase.co"
NEXT_PUBLIC_SUPABASE_ANON_KEY="XXX.YYY.ZZZ"
CRAWLER_API="https://{crawler_domain}/site/crawl_async"
- Automated Data Submission: The integration with Vercel’s scheduled tasks allows the application to automatically fetch and submit new AI tools to the database. This is done through a cron job that triggers the API endpoint at specified intervals.
4. Technical Challenges Overcome
-
Database Compatibility: Transitioning from version 1.0.0 to 2.0.0 involved ensuring compatibility with the Supabase database. This required careful planning of the database schema and migration scripts to avoid data loss.
-
Crawler Reliability: The web crawler faced challenges with various anti-crawling mechanisms employed by websites. To mitigate this, the team implemented fallback mechanisms that allow for manual data entry when the crawler fails.
Example of Manual Data Entry SQL Script
-- Insert new AI tool manually
INSERT INTO web_navigation (name, description, url) VALUES ('New AI Tool', 'Description of the new AI tool', 'https://newaitool.com');
- Performance Optimization: As the number of AI tools grew, performance became a concern. The team optimized database queries and implemented caching strategies to ensure fast load times for users.
Lessons from the Trenches
Key Technical Lessons Learned
-
Database Integration: Implementing Supabase as a serverless database was a significant learning experience. Understanding how to set up the database, execute SQL scripts, and manage data effectively was crucial. The importance of database structure and relationships became evident, especially when dealing with dynamic content.
-
Next.js Features: Utilizing Next.js 14 and its app routing capabilities allowed for a better understanding of React server components. Learning how to leverage features like internationalization (i18n) and dynamic routing was essential for creating a user-friendly interface.
-
SEO Optimization: Implementing SEO-friendly practices, including dynamic sitemap generation and proper metadata usage, highlighted the importance of search engine visibility for web applications. Understanding how to optimize content for search engines was a valuable takeaway.
-
Environment Variables Management: Managing environment variables for deployment on platforms like Vercel was a critical aspect. Learning how to securely handle sensitive information and configure the application for different environments (development vs. production) was essential.
What Worked Well
-
User-Friendly Interface: The design and implementation of a clean, intuitive user interface made it easy for users to navigate and find AI tools. The categorization and search functionalities were particularly well-received.
-
Community Engagement: Encouraging users to fork and star the project on GitHub fostered a sense of community and collaboration. The support for multiple languages also helped reach a broader audience.
-
Automated Crawler Integration: The integration with the Tap4 AI crawler project for automatic submission and collection of AI tools streamlined the process of keeping the directory updated. This automation reduced manual workload and improved efficiency.
-
Documentation: Providing comprehensive documentation, including deployment instructions and troubleshooting tips, helped users set up the project with minimal friction. Clear instructions on modifying the database and handling crawler issues were particularly useful.
What You’d Do Differently
-
Enhanced Error Handling: Implementing more robust error handling and logging mechanisms would improve the debugging process. This would help identify issues with the crawler or database interactions more quickly.
-
User Feedback Mechanism: Adding a feature for users to provide feedback directly through the platform could help gather insights on user experience and areas for improvement. This could lead to more user-driven enhancements.
-
Performance Optimization: Conducting performance testing and optimization earlier in the development process would ensure that the application scales effectively as the number of AI tools increases. This includes optimizing database queries and front-end performance.
-
Testing Framework: Establishing a testing framework for both unit and integration tests would enhance code reliability. This would help catch bugs early in the development cycle and ensure that new features do not break existing functionality.
Advice for Others
-
Start with a Clear Plan: Before diving into development, outline a clear plan that includes project goals, technical stack, and user requirements. This will help maintain focus and direction throughout the project.
-
Leverage Open Source Resources: Don’t hesitate to use existing open-source libraries and tools. They can save time and effort, allowing you to focus on unique features of your project.
-
Prioritize Documentation: Invest time in creating thorough documentation from the start. This will not only help you but also assist others who may want to contribute or use your project in the future.
-
Engage with the Community: Actively engage with users and contributors. Their feedback can provide valuable insights and help shape the direction of the project. Consider creating a dedicated channel for discussions and suggestions.
-
Iterate and Improve: Be open to iterating on your project based on user feedback and changing requirements. Continuous improvement is key to maintaining relevance and usability in the fast-evolving AI landscape.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
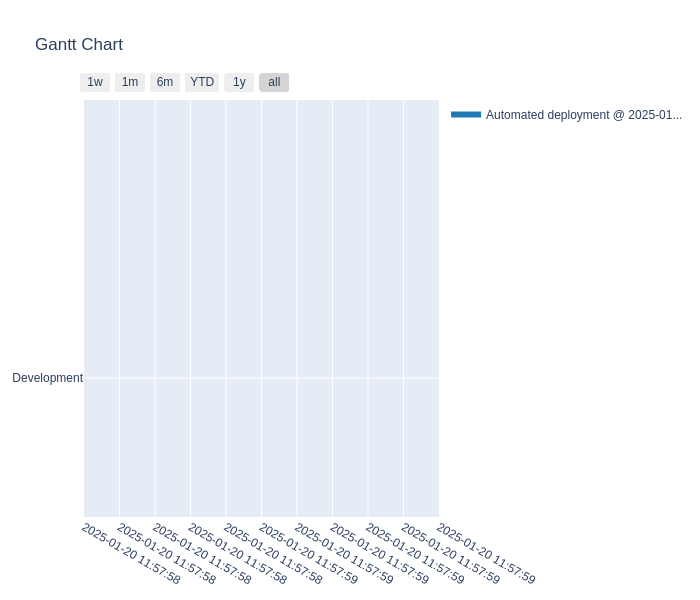
Commit Activity Heatmap
Contributor Network
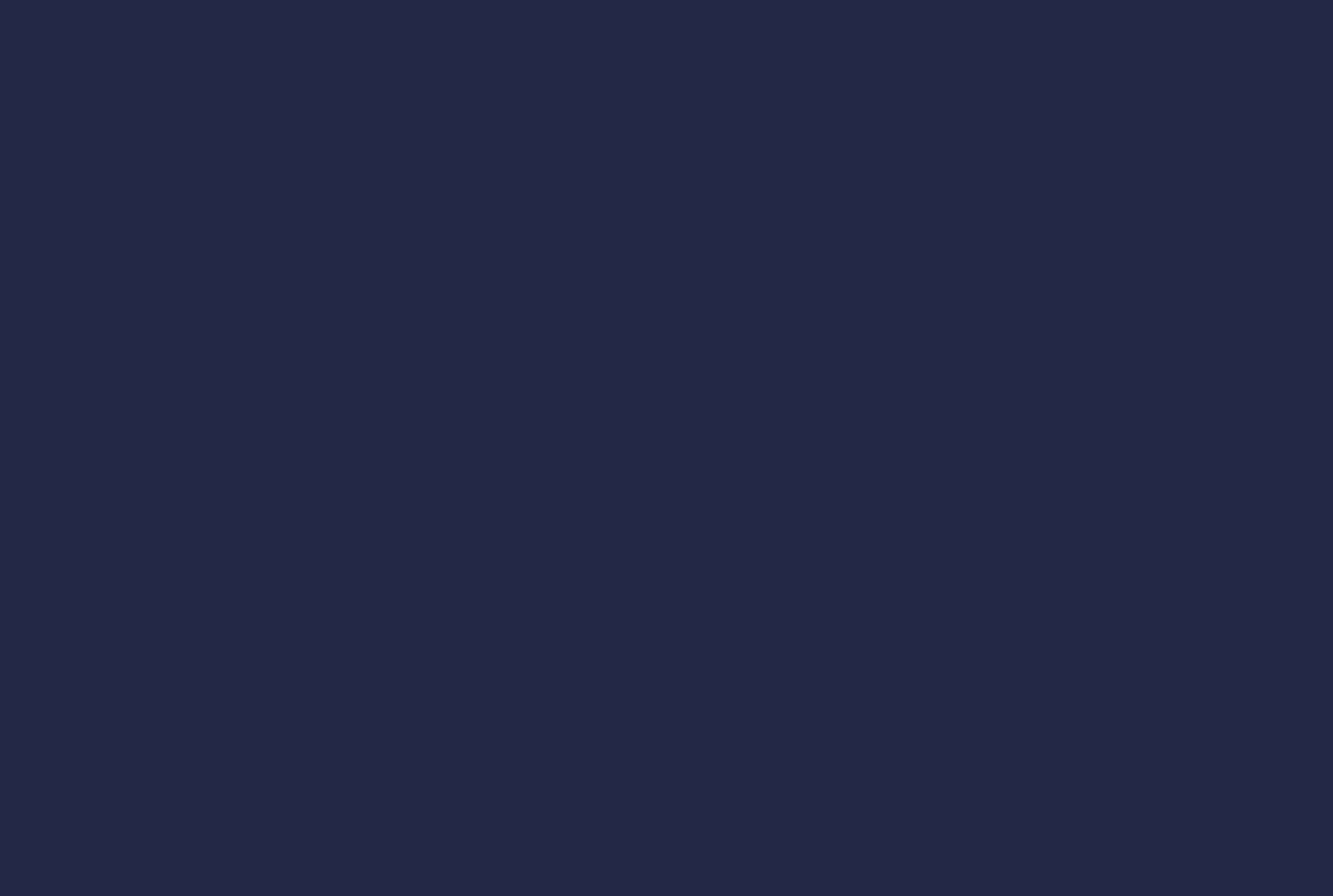
Commit Activity Patterns
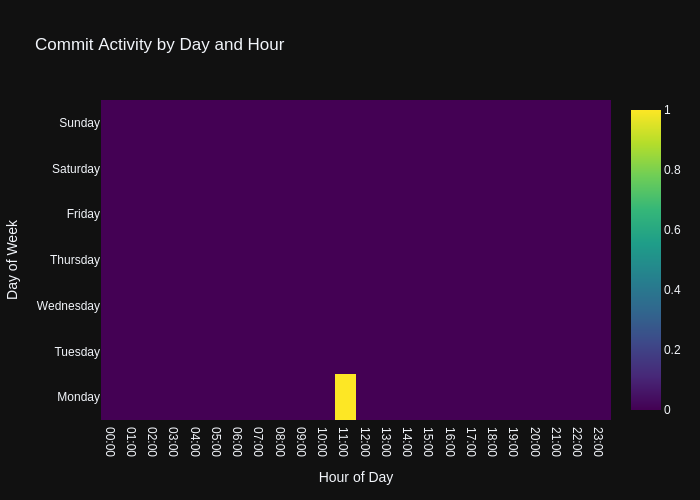
Code Frequency
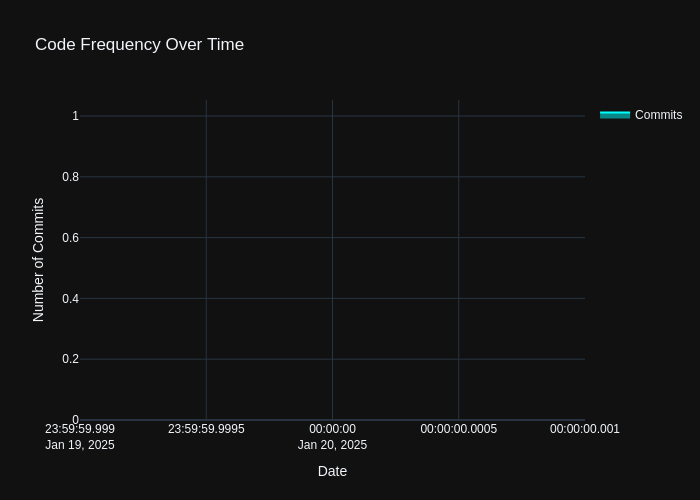
- Repository URL: https://github.com/wanghaisheng/bestfreeai
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 27 日