Building blackjack-realtime: A Web3 Live Blackjack Experience with Friends
Project Genesis
Unveiling Blackjack-Realtime: My Journey into High-Performance Gaming
From Idea to Implementation
JStack: From Concept to Code
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of prioritizing user experience became clear early on. We focused on creating a seamless onboarding process and intuitive user interface, ensuring that even those with limited technical expertise could deploy their applications easily.
-
Performance Matters: Our research highlighted that performance is a critical factor for user retention. We implemented best practices for optimizing Next.js applications, such as code splitting, image optimization, and caching strategies, to ensure that JStack applications load quickly and efficiently.
-
Cost Efficiency: Many developers and startups operate on tight budgets. By utilizing serverless architecture and cloud services, we were able to keep operational costs low while providing a robust platform for application deployment.
-
Community Feedback: Engaging with the developer community throughout the project was invaluable. Their feedback helped us refine our features and prioritize functionalities that would have the most significant impact on user satisfaction.
Under the Hood
Technical Deep-Dive on JStack
1. Architecture Decisions
Key Architectural Components:
- Frontend: Built with Next.js, providing a seamless user experience with fast page loads and optimized performance.
- Backend: Utilizes a RESTful API architecture, allowing the frontend to communicate with various microservices.
- Database: A NoSQL database (e.g., MongoDB) is used for flexibility in data modeling and scalability.
- Caching Layer: Implemented using Redis to enhance performance by caching frequently accessed data.
Example of API Route in Next.js:
// pages/api/users.js
import db from '../../lib/db';
export default async function handler(req, res) {
const users = await db.collection('users').find().toArray();
res.status(200).json(users);
}
2. Key Technologies Used
- Next.js: For server-side rendering and static site generation, improving SEO and load times.
- React: The core library for building user interfaces, enabling component-based architecture.
- Node.js: For the backend, allowing for asynchronous operations and handling multiple requests efficiently.
- MongoDB: A NoSQL database that provides flexibility in data storage and retrieval.
- Redis: For caching, which reduces database load and speeds up response times.
Example of a Next.js Component:
// components/UserList.js
import React from 'react';
const UserList = ({ users }) => (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
export default UserList;
3. Interesting Implementation Details
Example of ISR in Next.js:
// pages/users.js
import UserList from '../components/UserList';
export async function getStaticProps() {
const res = await fetch('https://api.example.com/users');
const users = await res.json();
return {
props: {
users,
},
revalidate: 10, // Re-generate the page every 10 seconds
};
}
const UsersPage = ({ users }) => <UserList users={users} />;
export default UsersPage;
4. Technical Challenges Overcome
Challenge 1: Performance Optimization
Challenge 2: Managing State
Example of a Custom Hook for State Management:
// hooks/useUser.js
import { useState, useEffect } from 'react';
const useUser = () => {
const [user, setUser] = useState(null);
useEffect(() => {
const fetchUser = async () => {
const res = await fetch('/api/user');
const data = await res.json();
setUser(data);
};
fetchUser();
}, []);
return user;
};
export default useUser;
Challenge 3: Deployment and CI/CD
Example of a GitHub Actions Workflow:
name: CI/CD Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
- name: Deploy to Vercel
run: npx vercel --prod
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- Performance Optimization: Leveraging Next.js’s built-in features like static site generation (SSG) and server-side rendering (SSR) can significantly enhance performance. Understanding when to use each method is crucial for optimizing load times and user experience.
- API Routes: Utilizing Next.js API routes for backend functionality can simplify the architecture by keeping everything within a single framework, reducing the need for separate backend services.
- Image Optimization: Implementing Next.js’s Image component helped in automatically optimizing images, which improved loading times and reduced bandwidth usage.
- Code Splitting: Next.js automatically splits code, but being mindful of how components are structured can further enhance performance. Lazy loading components that are not immediately necessary can lead to faster initial loads.
- Deployment Strategies: Using platforms like Vercel or Netlify for deployment can streamline the process and provide built-in optimizations for Next.js applications.
2. What Worked Well
- Rapid Development: The combination of Next.js and React allowed for rapid prototyping and development, enabling quick iterations based on user feedback.
- Community and Ecosystem: The strong community support and rich ecosystem of plugins and libraries for Next.js facilitated the integration of various features without reinventing the wheel.
- SEO Benefits: The SSR capabilities of Next.js improved SEO performance, leading to better visibility and traffic for the applications built with JStack.
- Cost Efficiency: By utilizing serverless functions and static hosting, the overall hosting costs were kept low, making it feasible to ship high-performance apps at a fraction of the cost.
3. What You’d Do Differently
- More Comprehensive Testing: Implementing a more robust testing strategy, including unit tests and end-to-end tests, could have caught issues earlier in the development process.
- Documentation: While the README provided a good starting point, more detailed documentation on setup, deployment, and troubleshooting would have been beneficial for new developers joining the project.
- User Feedback Loop: Establishing a more structured feedback loop with users could have led to quicker identification of pain points and feature requests, improving the overall product.
- Performance Monitoring: Integrating performance monitoring tools from the beginning would have provided insights into real-world performance and user experience, allowing for proactive optimizations.
4. Advice for Others
- Start Small: When building with Next.js, start with a small, focused feature set. This allows for quicker iterations and helps in understanding the framework’s capabilities without getting overwhelmed.
- Leverage Built-in Features: Take full advantage of Next.js’s built-in features like API routes, image optimization, and automatic code splitting to enhance performance and reduce development time.
- Stay Updated: The Next.js ecosystem evolves rapidly. Regularly check for updates and new features that can improve your application’s performance and developer experience.
- Engage with the Community: Participate in forums, GitHub discussions, and community events. Engaging with other developers can provide valuable insights and help solve challenges you may face.
- Plan for Scalability: Even if starting small, consider how your application will scale. Design your architecture with scalability in mind to avoid major refactoring later on.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
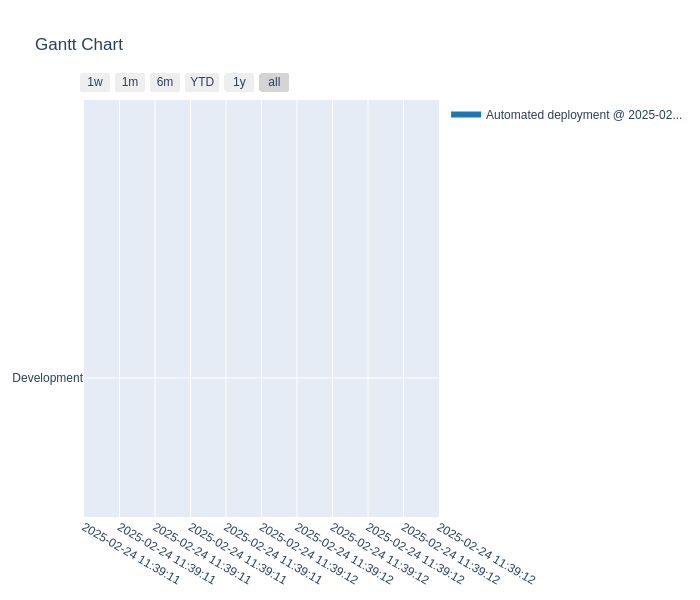
Commit Activity Heatmap
Contributor Network
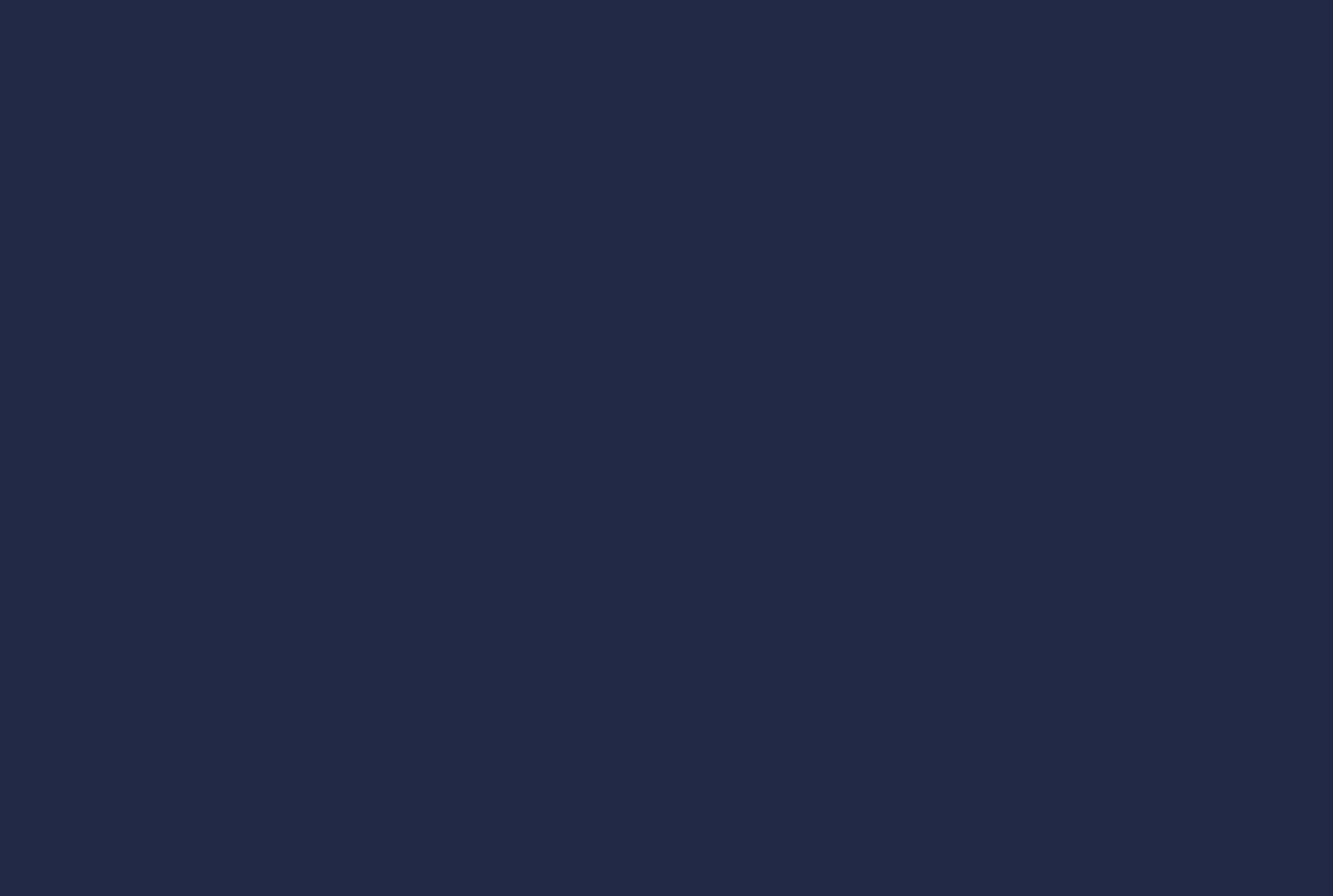
Commit Activity Patterns
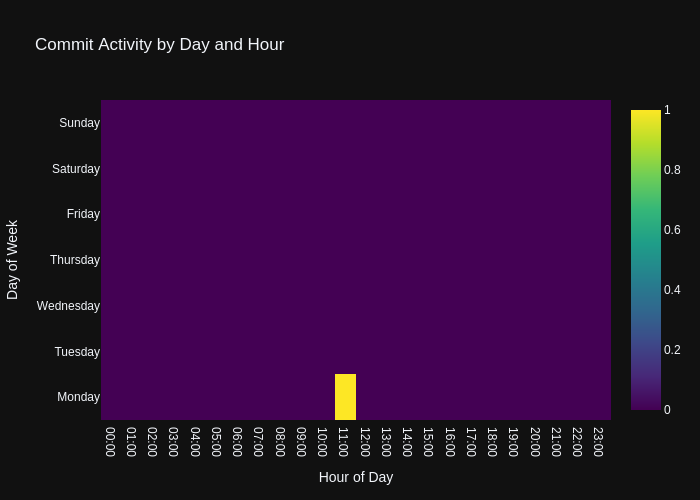
Code Frequency
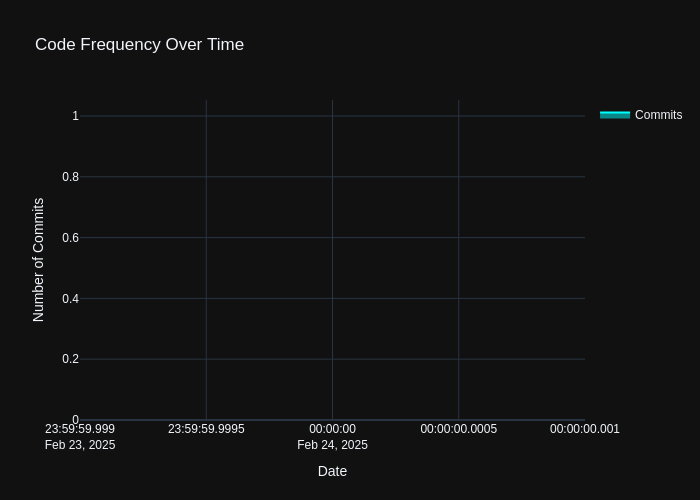
- Repository URL: https://github.com/wanghaisheng/blackjack-realtime
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 3 月 3 日