Weekend Hack: How I Built BulQR-Generator with React and TypeScript
Project Genesis
Unleashing the Power of Bulk QR Code Generation: My Journey with BulQR-Generator
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
Conclusion
Under the Hood
Technical Deep-Dive: shopconnan BulQR Code Generator
1. Architecture Decisions
Key Architectural Choices:
- Client-Side Rendering (CSR): The application is built as a single-page application (SPA) using React, which allows for a dynamic user experience without full page reloads.
- Progressive Web App (PWA): By integrating Vite-plugin-pwa, the application can function offline and be installed on devices, enhancing user engagement.
- Type Safety: TypeScript is used to enforce type safety, reducing runtime errors and improving code quality.
2. Key Technologies Used
-
Vite: A build tool that provides a fast development environment and optimized production builds. It supports hot module replacement (HMR), which speeds up the development process.
-
React: A JavaScript library for building user interfaces, allowing for the creation of reusable UI components.
-
TypeScript: A superset of JavaScript that adds static types, enabling better tooling and reducing bugs.
-
Chakra UI: A component library that provides accessible and customizable UI components, speeding up the design process.
-
QRcode: A library for generating QR codes, which is the core functionality of the application.
-
Client-zip: A utility for creating zip files on the client side, allowing users to download multiple QR codes in a single file.
-
React-hook-form: A library for managing form state and validation, simplifying the process of handling user input.
-
React-Colorful: A color picker component that allows users to customize the appearance of their QR codes.
3. Interesting Implementation Details
QR Code Generation
QRcode
library is utilized to create QR codes based on user input. Here’s a simple example of how QR codes are generated:import QRCode from 'qrcode';
const generateQRCode = async (text) => {
try {
const qrCodeDataUrl = await QRCode.toDataURL(text);
return qrCodeDataUrl;
} catch (error) {
console.error('Error generating QR code:', error);
}
};
Form Handling with React Hook Form
react-hook-form
to manage form submissions efficiently. This library minimizes re-renders and provides a simple API for form validation. Here’s an example of how to set up a form:import { useForm } from 'react-hook-form';
const QRCodeForm = () => {
const { register, handleSubmit } = useForm();
const onSubmit = (data) => {
console.log(data);
// Generate QR code logic here
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register('text')} placeholder="Enter text for QR code" />
<button type="submit">Generate QR Code</button>
</form>
);
};
Color Customization
react-colorful
, users can customize the color of their QR codes. This feature enhances user experience by allowing personalization. Here’s how color selection is integrated:import { ColorPicker } from 'react-colorful';
const QRCodeCustomizer = ({ onColorChange }) => {
return (
<ColorPicker onChange={onColorChange} />
);
};
4. Technical Challenges Overcome
Performance Optimization
Handling Large Data Sets
useMemo
and useCallback
hooks to prevent unnecessary re-renders and optimize performance.const memoizedQRCode = useMemo(() => generateQRCode(inputText), [inputText]);
File Download Management
client-zip
library was integrated to handle this functionality seamlessly. The implementation allows users to download all generated QR codes in a single action:import JSZip from 'client-zip';
const downloadZip = async (qrCodes) => {
const zip = new JSZip();
qrCodes.forEach((code, index) => {
zip.file(`qr_code_${index + 1}.png`, code);
});
const content = await zip.generateAsync({ type: 'blob
## Lessons from the Trenches
Certainly! Here’s a breakdown based on the project history and README for the `shopconnan` BulQR Code generator:
### 1. Key Technical Lessons Learned
- **State Management**: Managing the state for multiple QR codes can become complex. Using React's context or a state management library like Redux could simplify the handling of shared state across components.
- **Performance Optimization**: Generating up to 200 QR codes can be resource-intensive. Implementing lazy loading or pagination for the display of QR codes can enhance performance and user experience.
- **Error Handling**: Ensuring robust error handling for user inputs (e.g., invalid URLs) is crucial. Implementing validation with `React-hook-form` helped streamline this process.
- **PWA Features**: Integrating PWA features using `vite-plugin-pwa` was straightforward, but understanding the nuances of service workers and caching strategies was essential for offline functionality.
### 2. What Worked Well
- **Component Library**: Using Chakra UI allowed for rapid UI development with a consistent design system. The pre-built components helped maintain a clean and responsive layout.
- **TypeScript Integration**: TypeScript provided type safety, which reduced runtime errors and improved code maintainability. It was particularly beneficial in defining the structure of QR code data.
- **QR Code Generation**: The `qrcode` package was easy to integrate and provided high-quality QR codes. The ability to customize QR code styles with `React-Colorful` added a nice touch for user personalization.
- **User Experience**: The overall user experience was enhanced by the intuitive form handling with `React-hook-form`, making it easy for users to input data and generate codes quickly.
### 3. What You'd Do Differently
- **Testing**: Implementing more comprehensive testing (unit and integration tests) from the start would have helped catch bugs earlier. Using tools like Jest and React Testing Library could improve code reliability.
- **Accessibility**: While the UI was functional, focusing more on accessibility (a11y) from the beginning would ensure a wider audience can use the application. This includes proper ARIA roles and keyboard navigation support.
- **Deployment Strategy**: Exploring different deployment options (e.g., Vercel, Netlify) earlier in the project could have streamlined the process. Understanding CI/CD pipelines would also help automate deployments.
### 4. Advice for Others
- **Plan for Scalability**: When building applications that may handle large datasets (like 200 QR codes), plan your architecture to accommodate scalability from the beginning.
- **Documentation**: Maintain clear documentation throughout the development process. This will help onboard new team members and serve as a reference for future updates.
- **User Feedback**: Engage with users early and often. Gathering feedback on usability can guide feature development and improve the overall product.
- **Stay Updated**: The web development ecosystem evolves rapidly. Regularly check for updates on libraries and tools you use to leverage new features and improvements.
By reflecting on these aspects, future projects can benefit from the experiences gained during the development of the `shopconnan` BulQR Code generator.
## What's Next?
## Conclusion
As we wrap up this phase of the BulQR Code Generator project, we are excited to share our current status and future development plans. The project is currently in a robust state, allowing users to generate up to 200 QR codes seamlessly on the client side. Built with modern technologies like React, TypeScript, and Chakra UI, we have created a user-friendly interface that simplifies the QR code generation process. The integration of tools such as Vite and PWA support ensures that our application is not only fast but also accessible across various devices.
Looking ahead, we have ambitious plans for the future. We aim to enhance the functionality of BulQR by introducing features such as customizable QR code designs, improved export options, and enhanced user analytics to track code performance. Additionally, we are exploring the possibility of integrating a collaborative feature that allows teams to work together on QR code projects in real-time. These developments will not only improve user experience but also expand the utility of BulQR in various applications.
We invite contributors to join us on this exciting journey! Whether you are a developer, designer, or simply someone passionate about QR technology, your input and expertise can help shape the future of BulQR. We encourage you to check out our GitHub repository, share your ideas, report issues, or even submit pull requests. Together, we can make BulQR an indispensable tool for anyone looking to leverage the power of QR codes.
In closing, the journey of developing BulQR has been both challenging and rewarding. It has been a testament to the power of collaboration and innovation in the tech community. We are grateful for the support we have received so far and look forward to what lies ahead. Let’s continue to build, innovate, and inspire together as we take BulQR to new heights!
## Project Development Analytics
### timeline gant
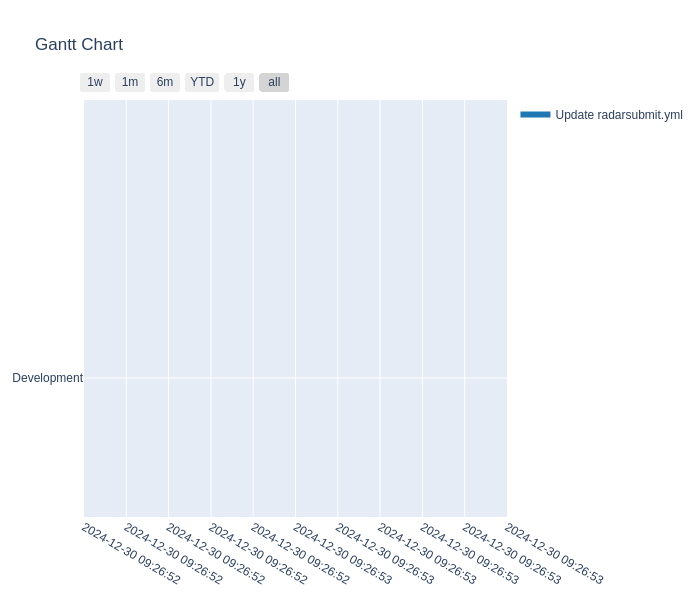
### Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
![Commit Heatmap]()
### Contributor Network
This network diagram shows how different contributors interact:
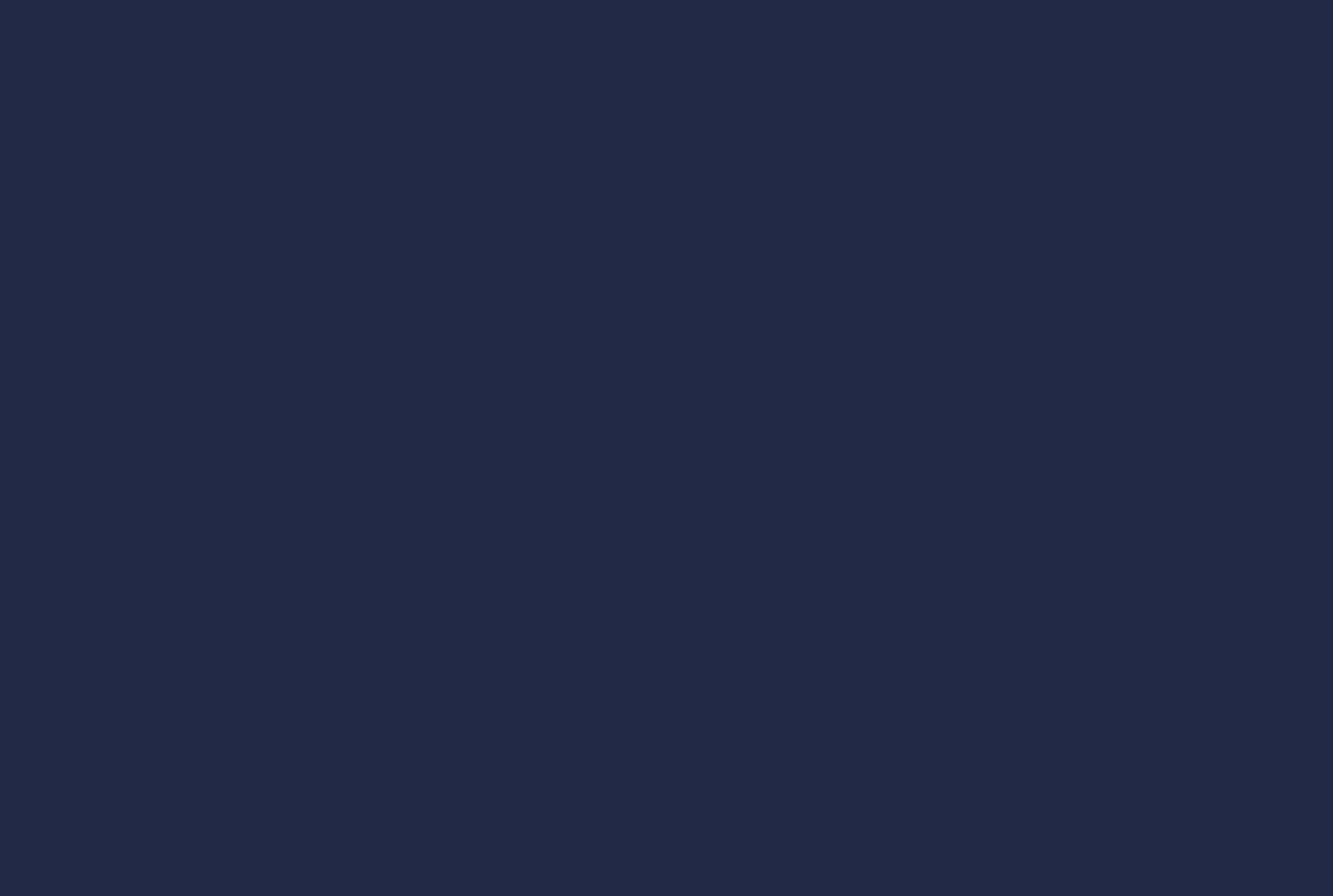
### Commit Activity Patterns
This chart shows when commits typically happen:
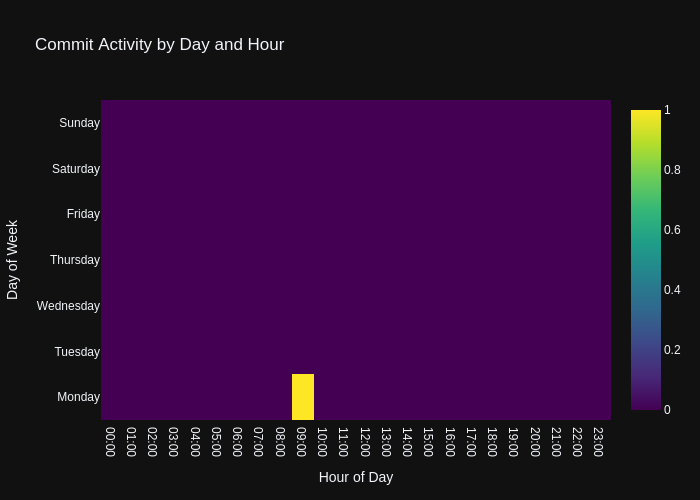
### Code Frequency
This chart shows the frequency of code changes over time:
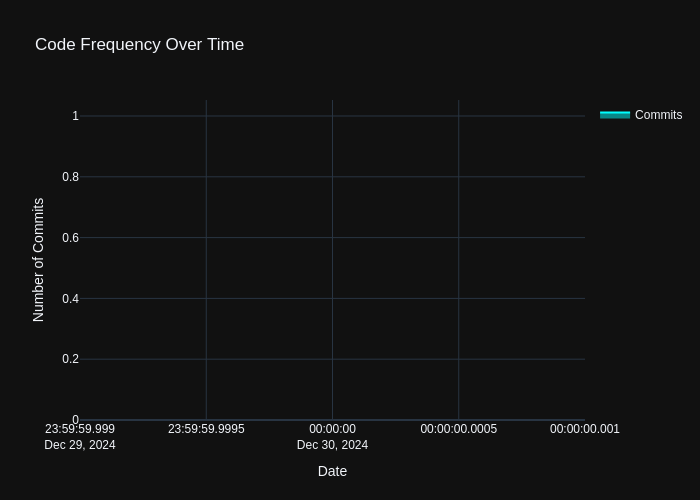
* Repository URL: [https://github.com/wanghaisheng/bulqr-generator](https://github.com/wanghaisheng/bulqr-generator)
* Stars: **0**
* Forks: **0**
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日