Building Chat-Game: Crafting an Interactive Twitch Adventure with Commands
Heisenberg
2024 年 12 月 30 日
弹幕互动游戏 A group of players are driving the Wagon from point A to point B, not knowing whether they will get there. Twitch viewers can use !commands, that trigger a lot of actions 🪓⛏️🌱🤝
Built by wanghaisheng | Last updated: 20241230
11 minutes 24 seconds read
Project Genesis
Unleashing Adventure: The Journey Behind Chat Game for Twitch
Hey there, fellow gamers and streamers! I’m thrilled to share the story behind a project that has become a passion of mine: the Chat Game for Twitch. Picture this: a procedurally generated world where you, the viewer, can dive into an adventure right from the chat box. It all started with a simple idea—what if I could create an interactive experience that not only entertained but also brought my community closer together?
As a streamer, I’ve always been fascinated by the power of engagement. I wanted to break the fourth wall and invite my viewers to be more than just spectators. The spark for this project ignited during one of my live streams when a viewer suggested a game where chat commands could influence the gameplay. That moment was a lightbulb moment for me! I envisioned a world where we could travel together in a virtual wagon, embark on quests, and even face challenges as a team—all through the magic of chat commands.
But let me tell you, the journey wasn’t without its hurdles. Initially, I faced the daunting task of figuring out how to seamlessly integrate chat commands with real-time actions in the game. The technical challenges were overwhelming at times, and there were moments when I questioned whether I could bring this vision to life. However, my passion for gaming and my desire to create a unique experience for my community kept me pushing forward.
After countless hours of coding, brainstorming, and testing, I finally found a solution that worked. The Chat Game allows viewers to interact with the game in real time, making decisions that shape the adventure. Whether it’s completing main quests or tackling side missions, every command typed in chat brings the world to life.
I can’t wait for you to join me on this incredible journey! Check out the game on our website, watch us develop and play live on Twitch, and connect with our amazing community on Discord. Together, we’ll explore uncharted territories and create unforgettable memories. Let’s embark on this adventure!
From Idea to Implementation
1. Initial Research and Planning
The journey of developing the Chat Game for Twitch began with extensive research into the gaming landscape, particularly focusing on interactive experiences that engage viewers in real-time. The team analyzed existing Twitch integrations, identifying gaps in viewer participation and interaction. This led to the concept of a procedurally generated world where viewers could influence gameplay through chat commands, creating a unique blend of gaming and streaming.
During the planning phase, the team outlined the core features that would define the game, such as real-time chat commands, quest systems, and resource gathering. User experience was a primary focus, ensuring that interactions were intuitive and engaging. The team also explored various game mechanics that would keep viewers invested, such as achievements and building systems, which would encourage ongoing participation.
2. Technical Decisions and Their Rationale
The technical stack was carefully chosen to support the game’s interactive and dynamic nature. Here are some key decisions:
- PixiJS: Selected for its ability to create rich, interactive graphics in the browser, allowing for a visually appealing game experience.
- Twurple: This library was chosen to facilitate seamless integration with Twitch APIs, enabling real-time chat interactions and user engagement.
- Vue and Nuxt: The decision to use Vue.js and Nuxt.js stemmed from their versatility and performance, making it easier to build a responsive user interface while managing state effectively.
- Prisma: This ORM was chosen for its ease of use with TypeScript, allowing for efficient database interactions and schema management.
- Howler.js: The audio library was integrated to enhance the gaming experience with sound effects and background music, making the game more immersive.
- TypeScript: The use of TypeScript was crucial for maintaining code quality and scalability, providing strong typing and better tooling support.
These decisions were made with the goal of creating a robust, maintainable codebase that could evolve as the game developed.
3. Alternative Approaches Considered
During the planning and development phases, several alternative approaches were considered:
- Game Engines: Initially, the team explored using traditional game engines like Unity or Unreal Engine. However, these options were deemed too complex for the intended web-based, interactive experience. The decision to use web technologies allowed for easier access and participation for viewers.
- Different Frameworks: Other JavaScript frameworks, such as React or Angular, were considered. Ultimately, Vue.js was chosen for its simplicity and ease of integration with the other tools in the stack.
- Server-Side Rendering vs. Client-Side Rendering: The team debated between server-side rendering and client-side rendering. The decision to use Nuxt.js for server-side rendering was made to improve performance and SEO, ensuring that the game could be easily discovered and accessed.
4. Key Insights That Shaped the Project
Several key insights emerged throughout the development process:
- Viewer Engagement: The realization that viewers want to be active participants rather than passive observers was pivotal. This insight drove the design of the game mechanics, emphasizing real-time interactions and collaborative gameplay.
- Community Building: The importance of fostering a community around the game became clear. Integrating Discord for community interaction and feedback was a strategic move to enhance player engagement and gather insights for future development.
- Iterative Development: The team adopted an iterative development approach, allowing for continuous feedback and improvement. This flexibility enabled the project to adapt to user needs and preferences, ensuring that the game remained relevant and enjoyable.
In conclusion, the journey from concept to code for the Chat Game for Twitch was marked by thorough research, thoughtful technical decisions, and a commitment to community engagement. The project reflects a blend of creativity and technology, aiming to create a unique interactive experience for Twitch viewers.
Under the Hood
Technical Deep-Dive: Chat Game for Twitch
1. Architecture Decisions
The architecture of the Chat Game for Twitch is designed to facilitate real-time interaction between streamers and their viewers. The game leverages a client-server model where the server handles game logic and state management, while the client is responsible for rendering the game and managing user interactions.
Key Architectural Components:
- Client-Side Rendering: The game is built using Vue.js and Nuxt.js, which allows for a reactive user interface that updates in real-time as users interact with the game through Twitch chat commands.
- WebSocket Communication: To enable real-time interactions, the game likely uses WebSockets for bi-directional communication between the client and server. This allows for immediate feedback when users issue commands in the chat.
- Microservices: The use of Prisma as an ORM suggests a microservices architecture where different services (e.g., user management, game state, quests) can be developed and scaled independently.
2. Key Technologies Used
The project utilizes a modern tech stack that includes:
- PixiJS: For rendering 2D graphics, enabling smooth animations and interactions within the game.
- Twurple: To interact with Twitch APIs, allowing the game to respond to chat commands and manage user sessions.
- Vue.js and Nuxt.js: For building the user interface, providing a reactive and component-based architecture.
- Prisma: As an ORM for database interactions, simplifying data management and migrations.
- TypeScript: Enhances code quality and maintainability by providing static typing.
- Howler.js: For audio management, allowing the integration of sound effects and background music.
Example of Using Twurple to Listen for Chat Commands:
import { ChatClient } from 'twurple';
const client = new ChatClient({
channels: ['your_channel_name']
});
client.connect();
client.onMessage((channel, user, message, self) => {
if (self) return; // Ignore messages from the bot
if (message.startsWith('!travel')) {
// Handle travel command
travelToLocation(user, message.split(' ')[1]);
}
});
3. Interesting Implementation Details
Procedural Generation
The game features a procedurally generated world, which means that the game environment is created algorithmically rather than being pre-designed. This allows for a unique experience for each player session.
Command Handling
The game allows viewers to interact through chat commands. Each command triggers specific actions in the game, such as traveling, completing quests, or gathering materials. This interaction model is crucial for engaging viewers and making them feel part of the game.
Example of Command Processing:
function processCommand(command, user) {
switch (command) {
case 'travel':
// Logic to move the user to a new location
break;
case 'gather':
// Logic to gather materials
break;
case 'quest':
// Logic to start a quest
break;
default:
// Handle unknown commands
break;
}
}
4. Technical Challenges Overcome
Real-Time Interaction
One of the main challenges was ensuring that the game could handle real-time interactions from multiple users simultaneously. This required efficient state management and the use of WebSockets to push updates to all connected clients.
Scalability
As the game grows in popularity, scaling the backend to handle increased traffic and user interactions is crucial. The use of microservices and a robust database management system (like Prisma) helps in managing this scalability.
Example of State Management:
import { reactive } from 'vue';
const gameState = reactive({
players: [],
resources: {},
quests: []
});
// Function to update game state
function updateGameState(newState) {
Object.assign(gameState, newState);
}
Conclusion
The Chat Game for Twitch is an innovative project that combines gaming with live streaming, creating an interactive experience for viewers. By leveraging modern web technologies and a well-thought-out architecture, the game successfully engages users and provides a unique platform for interaction. The challenges faced during development, such as real-time communication and scalability, were effectively addressed through the use of appropriate technologies and design patterns.
Lessons from the Trenches
Here are some key technical lessons learned, what worked well, what could be done differently, and advice for others based on the project history and README of the Chat Game for Twitch:
1. Key Technical Lessons Learned
- Integration of Multiple Libraries: Successfully integrating various libraries (PixiJS for graphics, Twurple for Twitch API, Vue and Nuxt for UI, Prisma for ORM) highlighted the importance of ensuring compatibility and understanding the strengths of each library. It’s crucial to read documentation thoroughly and test integrations early in the development process.
- Real-time Interaction: Implementing real-time chat commands required a solid understanding of WebSocket or similar technologies. This experience emphasized the need for efficient state management to handle user inputs and game state updates seamlessly.
- Containerized Development: Using Dev Containers streamlined the development process, allowing contributors to set up their environments quickly. This approach reduced onboarding time and ensured consistency across development setups.
2. What Worked Well
- Community Engagement: Building a community on Discord and streaming development on Twitch fostered engagement and provided valuable feedback. This interaction helped in refining game mechanics and understanding player expectations.
- Procedural Generation: The procedural generation of the game world added replayability and kept the experience fresh for users. This feature was well-received and encouraged players to explore different strategies and outcomes.
- Modular Architecture: The use of a modular architecture with Vue and Nuxt allowed for easy scalability and maintainability. This structure made it easier to add new features and manage the codebase as the project grew.
3. What You’d Do Differently
- More Comprehensive Testing: While the project has been successful, implementing a more robust testing framework (e.g., unit tests, integration tests) from the beginning would have helped catch bugs earlier and improved overall code quality.
- Documentation: Although the README provides a good overview, more detailed documentation on specific features, API usage, and contribution guidelines would benefit new developers and contributors. This could include examples and best practices.
- Performance Optimization: As the game scales, performance may become an issue. Planning for optimization strategies (e.g., lazy loading assets, optimizing rendering) earlier in the development process could prevent bottlenecks later.
4. Advice for Others
- Start Small and Iterate: Begin with a minimal viable product (MVP) and gradually add features based on user feedback. This approach helps in validating ideas and ensures that development efforts align with user interests.
- Foster Community: Engage with your audience through platforms like Discord and Twitch. Community feedback is invaluable for improving the game and keeping players invested.
- Embrace Open Source: Encourage contributions by making it easy for others to get involved. Clear contribution guidelines and a welcoming environment can attract more developers to your project.
- Stay Updated: Technology evolves rapidly. Keep an eye on updates to the libraries and frameworks you use, as well as emerging technologies that could enhance your project.
By reflecting on these aspects, the Chat Game for Twitch can continue to evolve and improve, providing an engaging experience for both developers and players.
What’s Next?
Conclusion: The Journey Ahead for Chat Game
As we stand at the current project status of the Chat Game for Twitch, we are thrilled to see the progress made thus far. Our community has embraced the concept of a procedurally generated world where players can interact in real-time through chat commands, embark on quests, gather materials, and construct their own unique environments. The integration of technologies like PixiJS, Twurple, and Vue has laid a solid foundation for an engaging and immersive experience.
Looking ahead, our future development plans are ambitious and exciting. We aim to expand the game’s universe with more intricate quests, diverse characters, and enhanced gameplay mechanics. We envision a vibrant ecosystem where players can not only participate but also influence the world around them. Additionally, we are exploring opportunities to incorporate user-generated content, allowing our community to contribute their creativity and ideas directly into the game.
We invite all contributors—whether you’re a developer, designer, or simply a passionate gamer—to join us on this journey. Your input and creativity are invaluable as we continue to refine and expand the Chat Game. Together, we can build a thriving community of Stargazers who shape this world collaboratively. Check out our Discord community to connect with fellow contributors and share your ideas!
In closing, the journey of developing the Chat Game has been nothing short of exhilarating. From the initial concept to the current playable version, we have witnessed the power of collaboration and creativity. As we move forward, we are excited to see how our community will continue to shape this project. Let’s embark on this adventure together and create something truly special for Twitch viewers and gamers alike. Thank you for being a part of this incredible journey!
Project Development Analytics
timeline gant
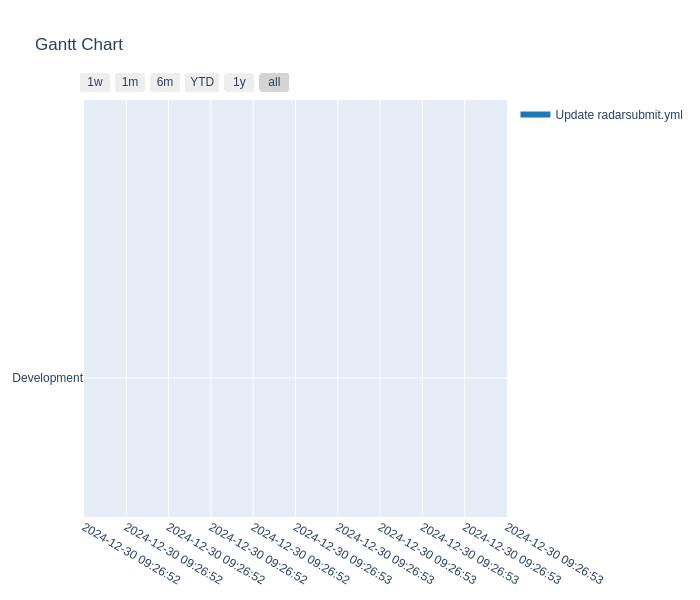
Commit timelinegant
Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
Commit Heatmap
Contributor Network
This network diagram shows how different contributors interact:
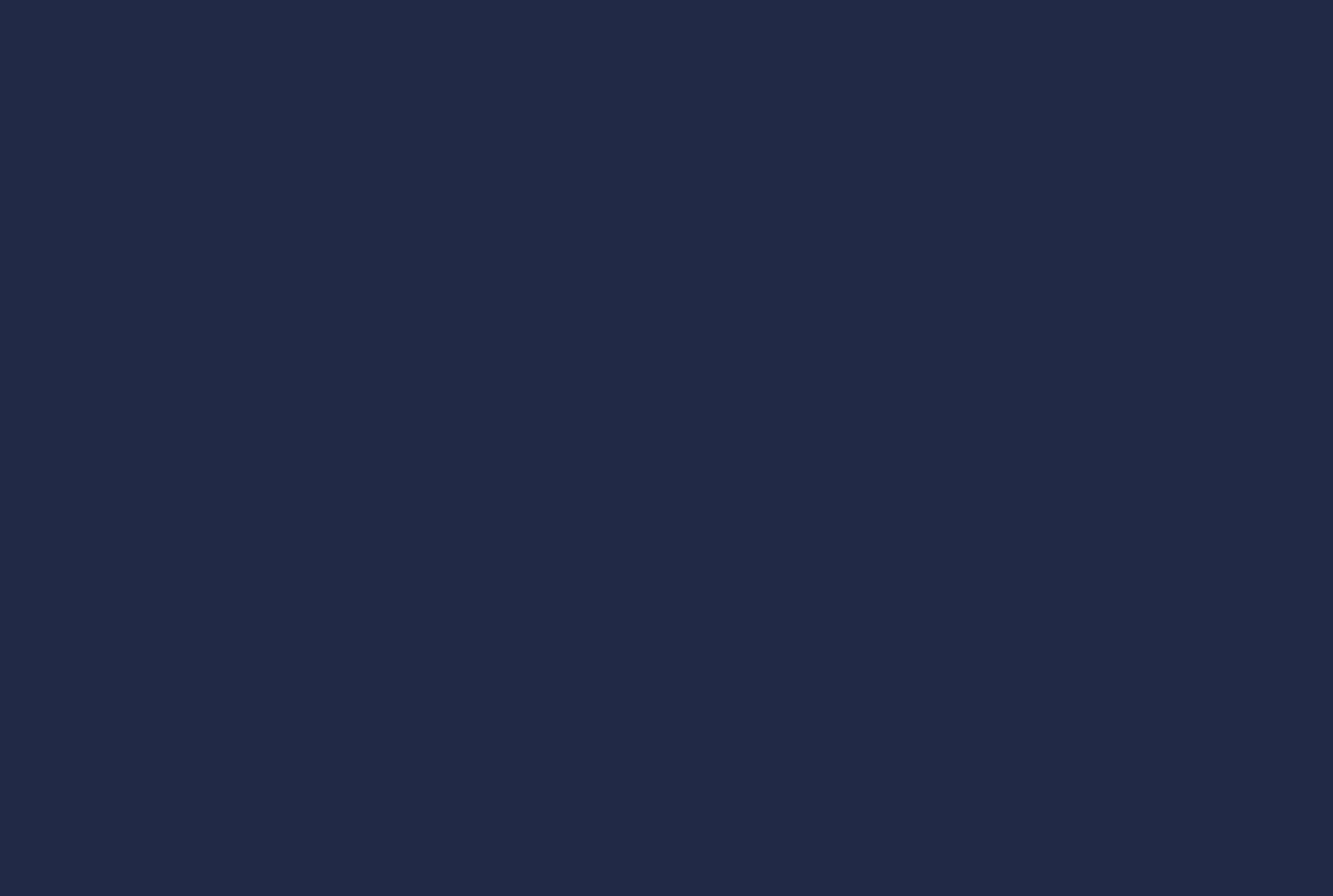
Contributor Network
Commit Activity Patterns
This chart shows when commits typically happen:
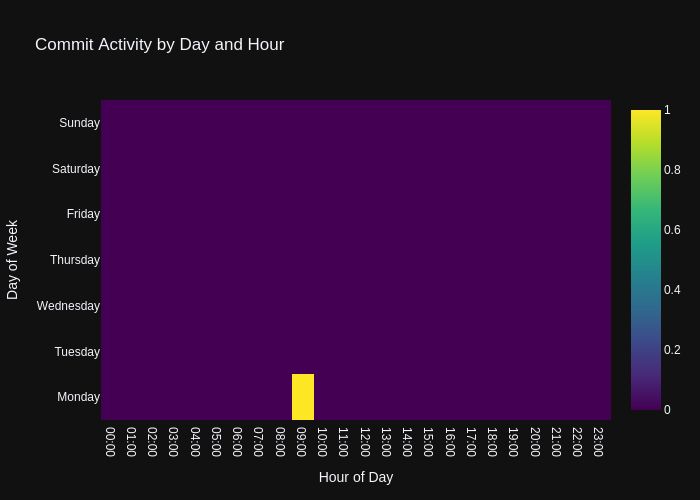
Commit Activity
Code Frequency
This chart shows the frequency of code changes over time:
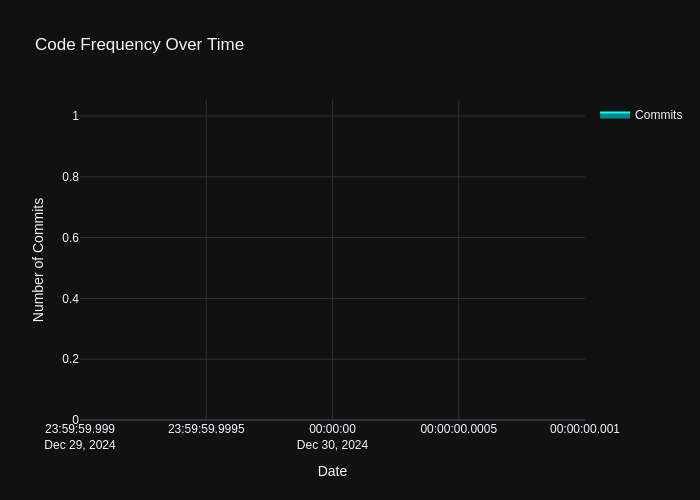
Code Frequency
- Repository URL: https://github.com/wanghaisheng/chat-game
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日