From Idea to Reality: Building the China-Made AI Blog Writer
Project Genesis
Unleashing Creativity: My Journey with the China-Made CT Blog Writer
From Idea to Implementation
文章生成工具 ai_writer 项目总结
一、初步研究与规划
二、技术决策及其理由
三、考虑的替代方案
四、塑造项目的关键见解
结论
Under the Hood
Technical Deep-Dive: 文章生成工具 ai_writer
1. Architecture Decisions
ai_writer
project is designed to optimize the generation of large volumes of text while maintaining flexibility and speed. The key architectural decisions include:-
Asynchronous Processing: The tool employs asynchronous calls to handle multiple requests simultaneously. This design choice allows the system to generate articles in parallel, significantly reducing the overall processing time. The architecture is built to ensure that the longest article generation time dictates the total time taken, leading to a time complexity of O(1).
-
Modular Prompt Management: The use of a dedicated folder (
./prompts
) for storing various prompts allows for easy modification and expansion of the tool’s capabilities. This modular approach enables users to switch between different writing styles and topics without altering the core codebase. -
Multi-Call Generation: To overcome the character limit imposed by the large language model (LLM), the architecture includes a mechanism to make multiple calls to the model for generating a single article. Each call generates a paragraph, which is then concatenated to form a complete article. This design effectively bypasses the 4096-character limit of the model.
2. Key Technologies Used
ai_writer
project leverages several key technologies:-
Large Language Models (LLMs): The core functionality relies on advanced LLMs capable of generating coherent and contextually relevant text. The choice of model can significantly impact the quality and depth of the generated content.
-
Asynchronous Programming: The implementation likely uses asynchronous programming paradigms (e.g.,
async/await
in Python) to handle multiple requests concurrently, improving performance and responsiveness. -
File I/O for Prompt Management: The project utilizes file input/output operations to manage prompts stored in the
./prompts
directory. This allows for dynamic loading and updating of prompts without requiring code changes.
3. Interesting Implementation Details
-
Prompt Variability: The ability to generate different styles and topics by simply changing prompts is a notable feature. For example, a user can create prompts for different genres, such as “technology blog,” “travel article,” or “product review,” and store them in the
./prompts
folder. This flexibility enhances the tool’s usability across various content creation scenarios. -
Batch Processing: The implementation can handle batch processing of articles. For instance, if a user requests 200 articles, the system can generate them in a single operation, leveraging the asynchronous architecture to maintain speed.
-
Error Handling and Retries: While not explicitly mentioned, robust error handling and retry mechanisms are essential in asynchronous systems. Implementing these features ensures that transient network issues do not lead to failed article generations.
4. Technical Challenges Overcome
-
Character Limit Workaround: One of the significant challenges was the character limit of the LLM. By implementing a multi-call strategy, the project successfully generates longer articles without being constrained by the model’s limitations.
-
Performance Optimization: Achieving a generation speed of less than one second per article required careful optimization of the asynchronous calls and efficient management of resources. This involved profiling the code to identify bottlenecks and optimizing the network calls to the LLM.
-
User Experience: Ensuring a seamless user experience while managing complex asynchronous operations can be challenging. The project likely includes user-friendly interfaces and clear documentation to guide users in utilizing the tool effectively.
Example Code Concepts
ai_writer
project:Asynchronous Article Generation
import asyncio
import aiohttp
async def generate_article(prompt):
async with aiohttp.ClientSession() as session:
async with session.post('https://api.llm.com/generate', json={'prompt': prompt}) as response:
return await response.json()
async def generate_articles(prompts):
tasks = [generate_article(prompt) for prompt in prompts]
return await asyncio.gather(*tasks)
# Example usage
prompts = ["Write a blog post about AI.", "Create a travel guide for Paris."]
articles = asyncio.run(generate_articles(prompts))
Managing Prompts
import os
def load_prompts(directory='./prompts'):
prompts = []
for filename in os.listdir(directory):
with open(os.path.join(directory, filename), 'r', encoding='utf-8') as file:
prompts.append(file.read())
return prompts
# Example usage
prompts = load_prompts()
Multi-Call Generation
async def generate_long_article(prompt):
paragraphs = []
for _ in range(5): # Generate 5 paragraphs
paragraph = await generate_article(prompt)
paragraphs.append(paragraph['text'])
return "\n\n".join(paragraphs)
# Example usage
long_article = asyncio.run(generate_long_article("Discuss the future of technology."))
ai_writer
Lessons from the Trenches
1. Key Technical Lessons Learned
- Asynchronous Processing: Implementing asynchronous calls significantly improved the speed of article generation. This approach allows for handling multiple requests simultaneously, which is crucial for generating large volumes of content efficiently.
- Prompt Engineering: The ability to modify prompts to generate diverse content highlights the importance of prompt engineering in AI applications. A well-structured prompt can lead to better and more relevant outputs.
- Chunking Output: By breaking down the article generation into multiple calls (3-5 times) to the model, the project effectively bypassed the character limit constraints of the AI model, allowing for the creation of longer and more in-depth articles.
2. What Worked Well
- Speed of Generation: The tool’s ability to generate 200 articles in 150 seconds is a significant achievement, demonstrating the effectiveness of the asynchronous model and the overall architecture.
- Flexibility and Customization: The dedicated folder for prompts allows users to easily switch between different styles and topics, making the tool versatile for various content needs.
- Depth of Content: The multi-call approach not only increased the length of the articles but also enhanced their depth and richness, providing users with more valuable content.
3. What You’d Do Differently
- User Interface Improvements: While the tool is powerful, enhancing the user interface could improve user experience. A more intuitive design could help users navigate the prompt customization and article generation process more easily.
- Error Handling and Logging: Implementing robust error handling and logging mechanisms would help in diagnosing issues during the generation process, especially when dealing with large batches of requests.
- Performance Monitoring: Setting up performance monitoring tools could provide insights into the system’s performance over time, helping to identify bottlenecks or areas for optimization.
4. Advice for Others
- Start with a Clear Use Case: Before diving into development, clearly define the target audience and use cases for your tool. This will guide your design decisions and feature set.
- Iterate Based on Feedback: After launching the initial version, gather user feedback and iterate on the tool. Continuous improvement based on real user experiences can lead to a more successful product.
- Invest in Documentation: Comprehensive documentation is essential for user adoption. Ensure that users have access to clear instructions on how to use the tool effectively, including examples of prompts and best practices.
- Explore Community Contributions: Encourage users to contribute their own prompts or styles to the project. This can enhance the tool’s capabilities and foster a community around it.
What’s Next?
Conclusion: Looking Ahead for the AI Writer Project
Project Development Analytics
timeline gant
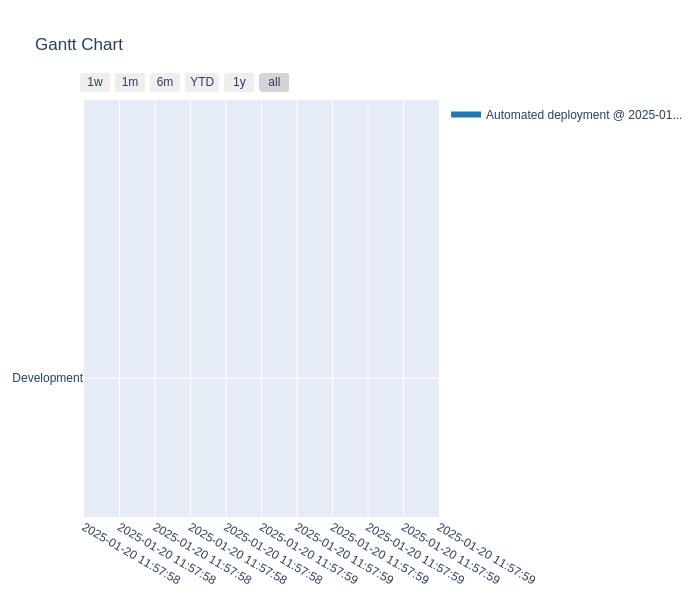
Commit Activity Heatmap
Contributor Network
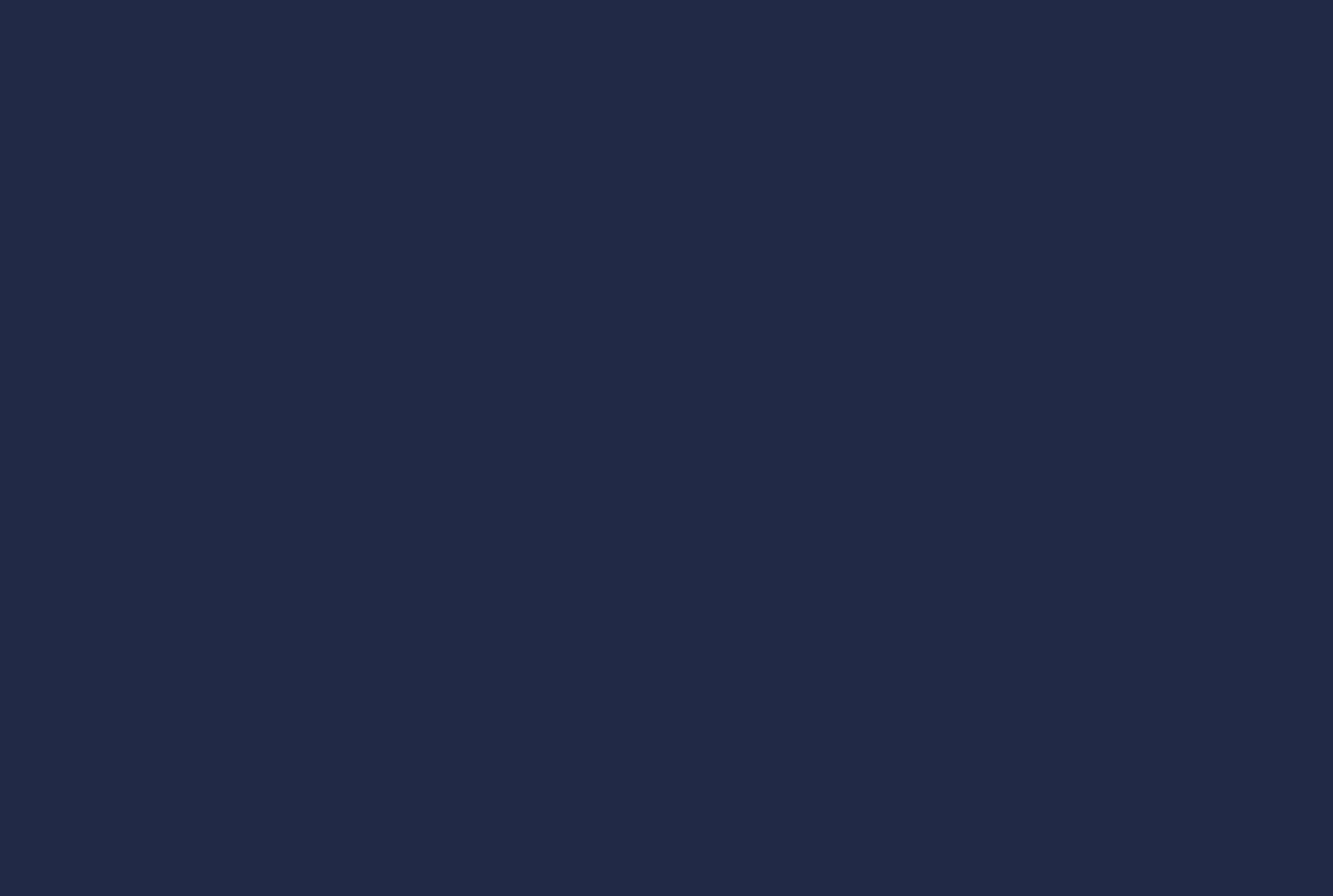
Commit Activity Patterns
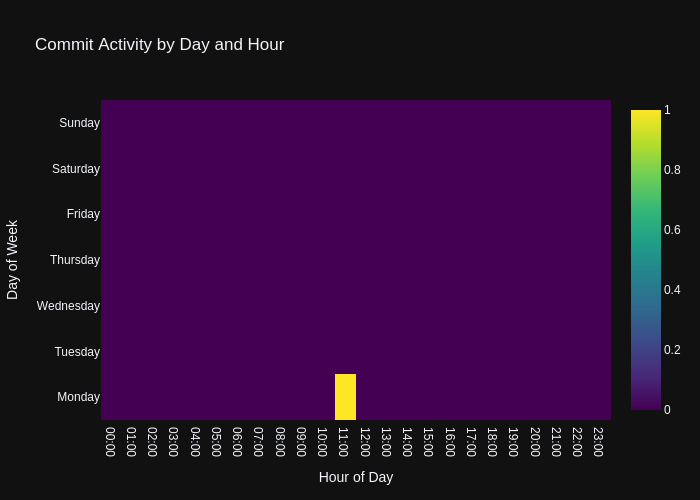
Code Frequency
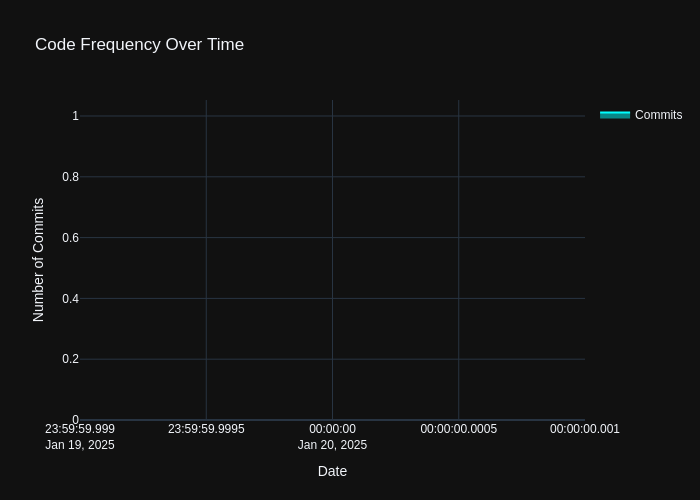
- Repository URL: https://github.com/wanghaisheng/china-made-ct-blog-writer
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 27 日