Building Cocos-Ball-Sort: A Colorful Journey in Game Development
Project Genesis
Sorting Fun: My Journey into Cocos-Ball-Sort
From Idea to Implementation
cocos-ball-sort Project Journey: From Concept to Code
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
Conclusion
Under the Hood
Technical Deep-Dive: cocos-ball-sort
1. Architecture Decisions
cocos-ball-sort
game is designed to be modular and maintainable, leveraging the capabilities of the Cocos2d-x game engine. The primary architectural decisions include:-
Component-Based Architecture: The game is structured around reusable components, allowing for easy modification and extension. Each game object (e.g., balls, tubes) is represented as a component that can be independently managed.
-
Scene Management: The game utilizes Cocos2d-x’s scene management to handle different game states (e.g., main menu, gameplay, game over). This separation of concerns helps in organizing the code and improving readability.
-
Event-Driven Programming: The game employs an event-driven approach to handle user interactions and game events. This allows for a responsive user interface and decouples the game logic from the rendering logic.
Example of Component-Based Architecture
class Ball : public cocos2d::Sprite {
public:
static Ball* create(const std::string& filename) {
Ball* ball = new Ball();
if (ball && ball->initWithFile(filename)) {
ball->autorelease();
return ball;
}
CC_SAFE_DELETE(ball);
return nullptr;
}
void setColor(const cocos2d::Color3B& color) {
this->setColor(color);
}
};
2. Key Technologies Used
cocos-ball-sort
game is built using several key technologies:-
Cocos2d-x: A popular open-source game development framework that provides a rich set of features for 2D game development, including rendering, physics, and animations.
-
C++: The primary programming language used for game logic and performance-critical components. C++ allows for fine-grained control over memory management and performance optimizations.
-
JavaScript (optional): If the game is intended to be played in a web environment, JavaScript can be used alongside Cocos2d-x for scripting game logic.
-
Physics Engine: Depending on the complexity of the game, a physics engine (like Box2D) may be integrated to handle realistic movements and collisions.
3. Interesting Implementation Details
-
Sorting Algorithm: The core gameplay mechanic involves sorting colored balls into tubes. A custom sorting algorithm is implemented to determine the valid moves and check for win conditions. This algorithm is optimized for performance to ensure smooth gameplay.
-
User Interface: The game features a simple yet effective user interface, including buttons for restarting the game and displaying the current score. The UI is built using Cocos2d-x’s built-in UI components.
Example of Sorting Logic
bool canMoveBall(Tube* fromTube, Tube* toTube) {
if (fromTube->isEmpty() || toTube->isFull()) {
return false;
}
return fromTube->topBall()->getColor() == toTube->topBall()->getColor();
}
4. Technical Challenges Overcome
-
Performance Optimization: One of the main challenges was ensuring that the game runs smoothly on various devices. This involved profiling the game and optimizing rendering and logic to minimize frame drops.
-
User Experience: Designing an intuitive user interface that guides players without overwhelming them was a challenge. Iterative testing and feedback helped refine the UI elements.
-
State Management: Managing the different states of the game (e.g., paused, playing, game over) required careful planning to ensure that transitions were smooth and that the game state was preserved correctly.
Example of State Management
class GameScene : public cocos2d::Scene {
public:
void pauseGame() {
Director::getInstance()->pause();
// Show pause menu
}
void resumeGame() {
Director::getInstance()->resume();
// Hide pause menu
}
};
cocos-ball-sort
game showcases a well-structured approach to game development using Cocos2d-x, with a focus on modularity, performance, and user experience. The challenges faced during development were addressed through careful planning and optimization, resulting in a polished final product.Lessons from the Trenches
1. Key Technical Lessons Learned
- Game Engine Proficiency: Gaining a deep understanding of the Cocos game engine was crucial. Familiarity with its rendering pipeline, scene management, and event handling significantly improved development efficiency.
- Data Structures: Implementing effective data structures for managing the state of the balls and tubes was essential. Using arrays and stacks helped in efficiently sorting and retrieving ball states.
- Performance Optimization: Learning to optimize performance, especially in rendering and memory management, was vital. Techniques such as object pooling and minimizing draw calls improved frame rates.
- User Input Handling: Implementing intuitive touch controls for sorting the balls required careful consideration of user experience. Understanding how to handle multi-touch and gestures was key.
2. What Worked Well
- Modular Design: The modular approach to coding (separating game logic, UI, and assets) made it easier to manage and update the game. This also facilitated collaboration if more developers joined the project.
- Visual Feedback: Providing immediate visual feedback for user actions (like sorting balls) enhanced user engagement. Animations and color changes helped in making the game more interactive.
- Testing and Iteration: Regular playtesting sessions allowed for quick identification of bugs and gameplay issues. Iterating based on user feedback led to a more polished final product.
3. What You’d Do Differently
- Documentation: While the README provided a basic overview, more comprehensive documentation throughout the codebase would have made it easier for new contributors to understand the project structure and logic.
- Version Control Practices: Implementing more structured version control practices (like feature branches and pull requests) could have improved collaboration and reduced merge conflicts.
- User Onboarding: Creating a more guided onboarding experience for new players could enhance user retention. A tutorial level or hints system would help new users understand game mechanics better.
4. Advice for Others
- Start Small: If you’re new to game development, start with a small project to grasp the fundamentals before tackling more complex games. This helps build confidence and skills incrementally.
- Focus on User Experience: Always prioritize user experience in game design. Playtesting with real users can provide invaluable insights that you might overlook as a developer.
- Leverage Community Resources: Engage with the Cocos community for support, tutorials, and resources. Learning from others’ experiences can save time and help avoid common pitfalls.
- Iterate and Improve: Don’t be afraid to iterate on your design based on feedback. Continuous improvement is key to creating a successful game.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
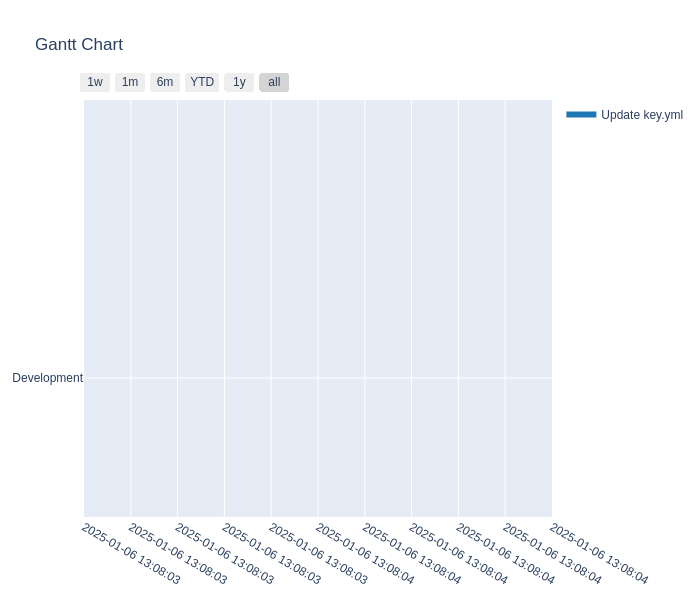
Commit Activity Heatmap
Contributor Network
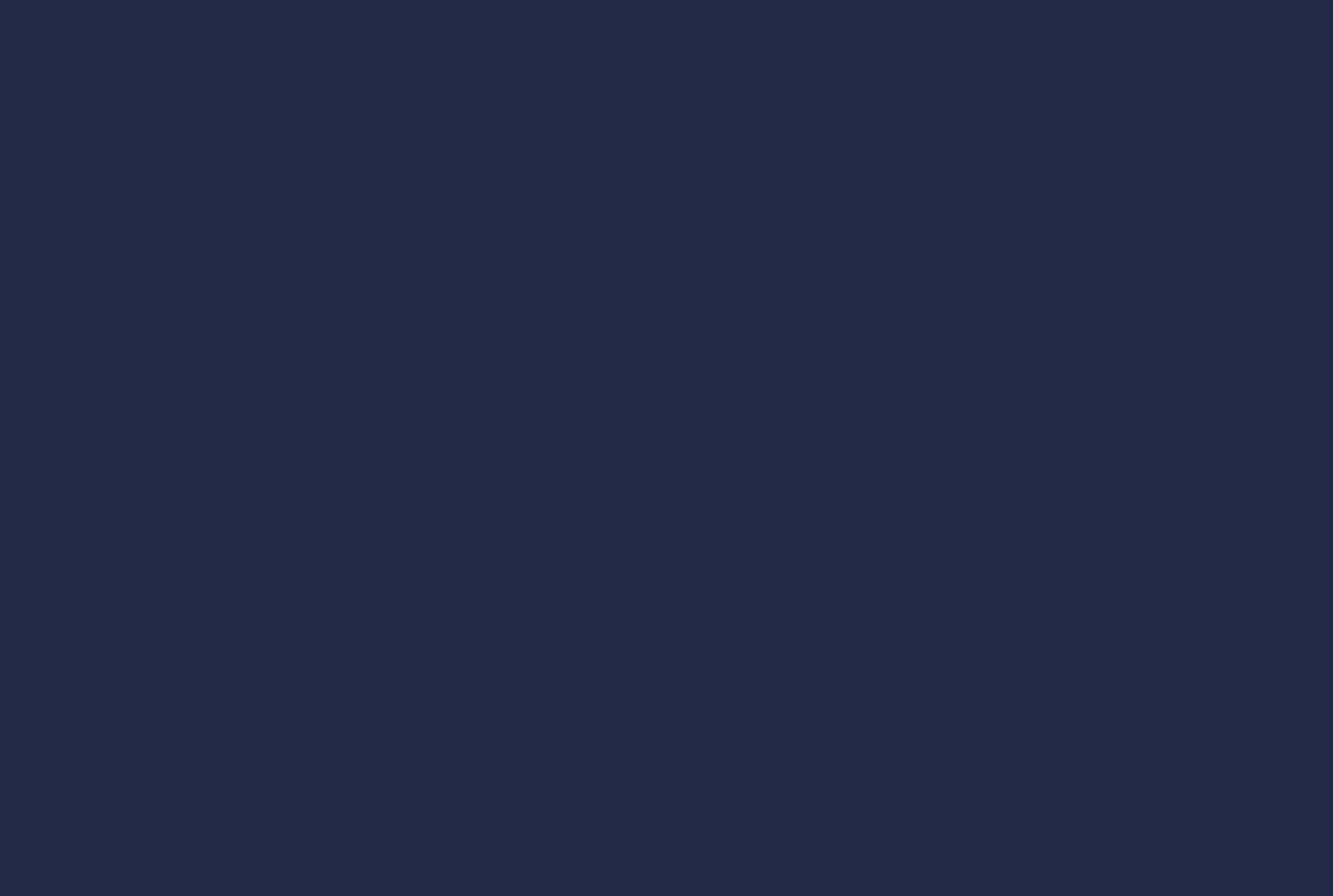
Commit Activity Patterns
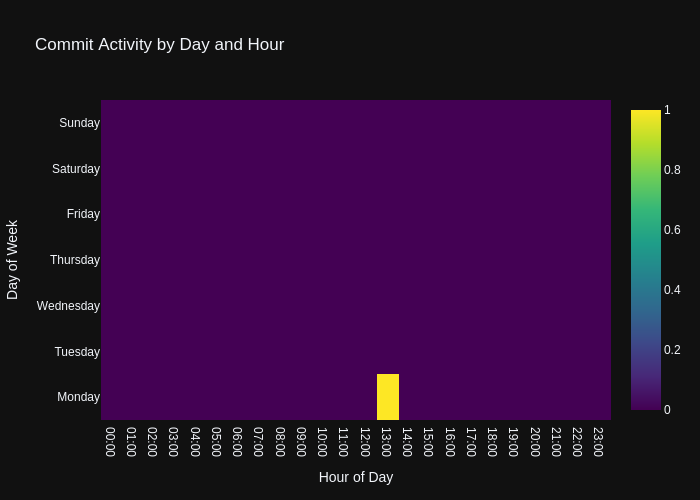
Code Frequency
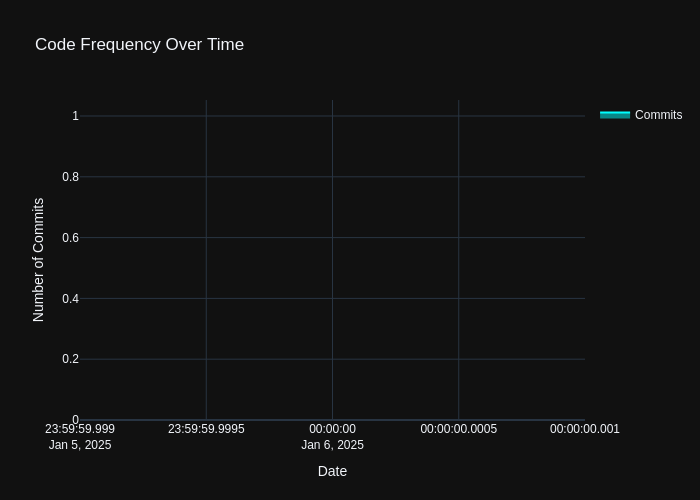
- Repository URL: https://github.com/wanghaisheng/cocos-ball-sort
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 13 日