Weekend Hack: How I Built Coffee Pal for the Perfect Brew Experience
Project Genesis
Brewing Connections: My Journey with Coffee Pal and Herb Pal
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
pnpm
as the package manager was made for its performance benefits and efficient handling of dependencies. This decision was crucial for ensuring that the development environment remained fast and responsive, especially as the project grew.3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of designing with the user in mind became evident. Continuous feedback from potential users helped refine features and prioritize functionality that truly adds value.
-
Simplicity and Usability: The realization that a clean, intuitive interface is crucial for user engagement led to a focus on simplicity in design. This insight guided the development of user flows and interactions within the application.
-
Community Engagement: Engaging with the coffee community through social media and forums provided valuable insights into trends and preferences, which influenced feature development and marketing strategies.
-
Iterative Development: Embracing an iterative development approach allowed for flexibility in responding to user feedback and making improvements. This adaptability was essential in refining the application before its launch.
Under the Hood
Technical Deep-Dive: Coffee Pal
1. Architecture Decisions
Key Architectural Choices:
- Component-Based Structure: Each utility (e.g., Journal, MyCoffees, Calculator, Drip Counter) is implemented as a separate component, promoting reusability and separation of concerns.
- State Management: The application likely uses a state management solution (e.g., Svelte stores) to manage the state across different components, ensuring that data flows seamlessly between them.
- Responsive Design: The architecture is designed to be responsive, ensuring that the application works well on various devices, from desktops to mobile phones.
2. Key Technologies Used
- Svelte: A modern JavaScript framework that compiles components into highly efficient vanilla JavaScript at build time. This results in faster performance and smaller bundle sizes.
- pnpm: A fast, disk space-efficient package manager that is used to manage dependencies. It allows for the installation of packages in a way that saves space and speeds up installations.
- Playwright: A testing framework that enables developers to write end-to-end tests for web applications. It supports multiple browsers and provides a rich API for simulating user interactions.
- ESLint and Prettier: Tools for maintaining code quality and consistency. ESLint helps identify and fix problems in JavaScript code, while Prettier formats code according to a set of rules.
3. Interesting Implementation Details
Utilities and Helpers
// Calculator.svelte
<script>
let input = '';
let result = null;
function calculate() {
try {
result = eval(input); // Caution: eval can be dangerous if not handled properly
} catch (error) {
result = 'Error';
}
}
</script>
<input bind:value={input} placeholder="Enter calculation" />
<button on:click={calculate}>Calculate</button>
<p>Result: {result}</p>
State Management
// coffeeStore.js
import { writable } from 'svelte/store';
export const coffeeEntries = writable([]);
export function addCoffee(coffee) {
coffeeEntries.update(entries => [...entries, coffee]);
}
4. Technical Challenges Overcome
Challenge: Managing State Across Components
Challenge: Testing Across Multiple Browsers
// Example Playwright test
import { test, expect } from '@playwright/test';
test('Calculator works correctly', async ({ page }) => {
await page.goto('https://coffee-pal.vercel.app');
await page.fill('input', '2 + 2');
await page.click('button');
const result = await page.textContent('p');
expect(result).toBe('Result: 4');
});
Challenge: Code Quality and Consistency
Lessons from the Trenches
1. Key Technical Lessons Learned
- Dependency Management: Using
pnpm
for dependency management proved to be efficient. It reduces disk space usage and speeds up installations compared to npm or yarn. Understanding how to leveragepnpm
effectively was crucial for maintaining a clean and efficient project. - Testing Frameworks: Integrating Playwright for end-to-end testing highlighted the importance of automated testing in ensuring application reliability. Learning how to write effective tests and set up CI/CD pipelines to run these tests automatically was invaluable.
- Code Quality Tools: Implementing tools like Prettier and ESLint helped maintain code quality and consistency across the project. It reinforced the importance of code style and linting in collaborative environments.
2. What Worked Well
- Modular Design: The project’s modular structure, with separate utilities for the journal, coffee tracking, calculator, and drip counter, made it easy to manage and extend. This separation of concerns facilitated easier debugging and feature additions.
- CI/CD Integration: The use of GitHub Actions for continuous integration and deployment streamlined the development process. Automated checks and deployments reduced manual errors and improved overall workflow efficiency.
- User-Centric Features: Focusing on user needs, such as the journal and coffee tracking features, led to positive feedback from users. Engaging with users early in the development process helped prioritize features that added real value.
3. What You’d Do Differently
- Documentation: While the README provides a good overview, more detailed documentation on each utility and its usage would enhance user experience. Including examples and use cases could help new users understand the functionalities better.
- User Testing: Conducting user testing sessions earlier in the development process could have provided insights into usability issues and feature requests. Gathering feedback from real users would help refine the application before launch.
- Performance Optimization: As the project grows, focusing on performance optimization from the start would be beneficial. Implementing performance monitoring tools early on could help identify bottlenecks and improve load times.
4. Advice for Others
- Start Small and Iterate: Begin with a minimal viable product (MVP) and iterate based on user feedback. This approach allows for flexibility and ensures that you are building features that users actually want.
- Emphasize Testing: Invest time in setting up automated tests early in the project. This will save time in the long run and help maintain code quality as the project scales.
- Engage with the Community: Don’t hesitate to seek feedback from the community or potential users. Engaging with others can provide fresh perspectives and ideas that can significantly enhance your project.
- Maintain Code Quality: Use tools like ESLint and Prettier consistently throughout the development process. Establishing coding standards early on will help maintain a clean codebase and facilitate collaboration.
What’s Next?
Conclusion: Looking Ahead for Coffee Pal
Project Development Analytics
timeline gant
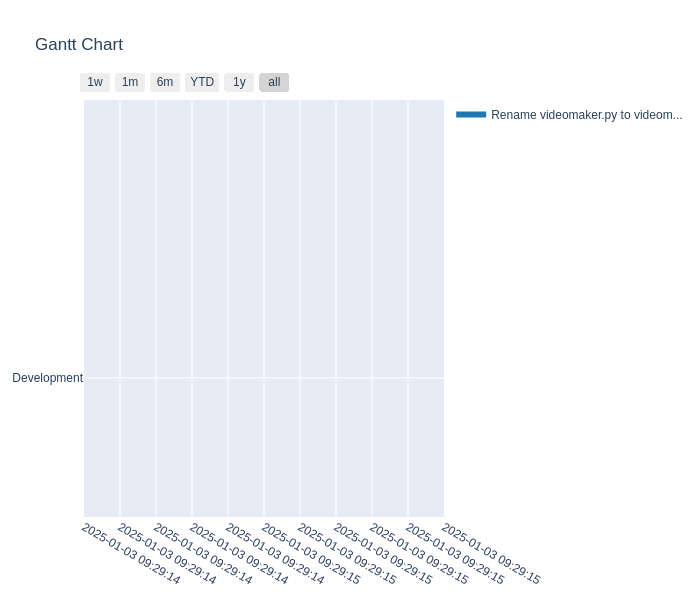
Commit Activity Heatmap
Contributor Network
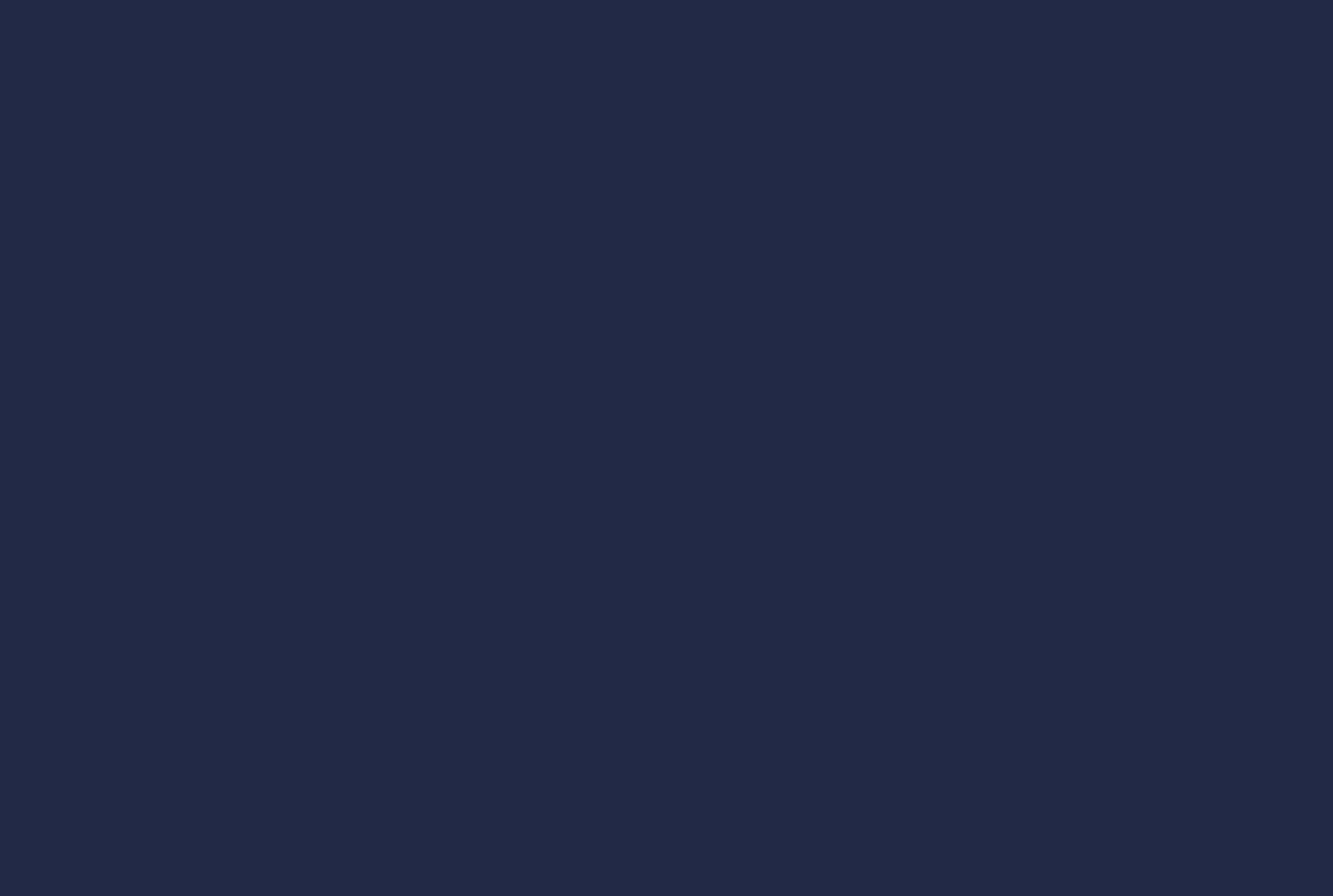
Commit Activity Patterns
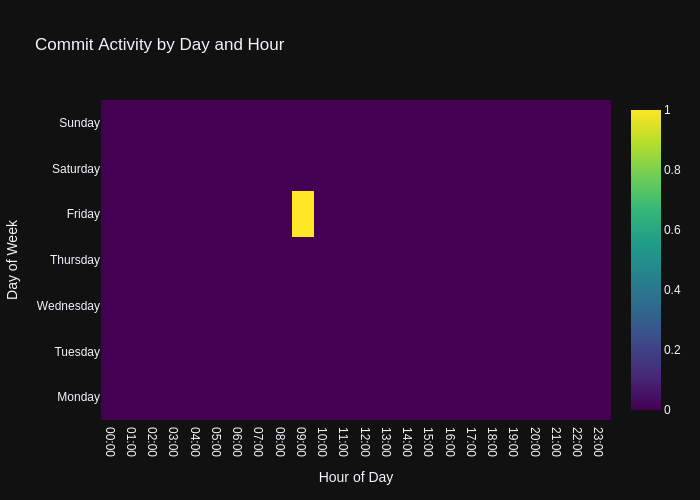
Code Frequency
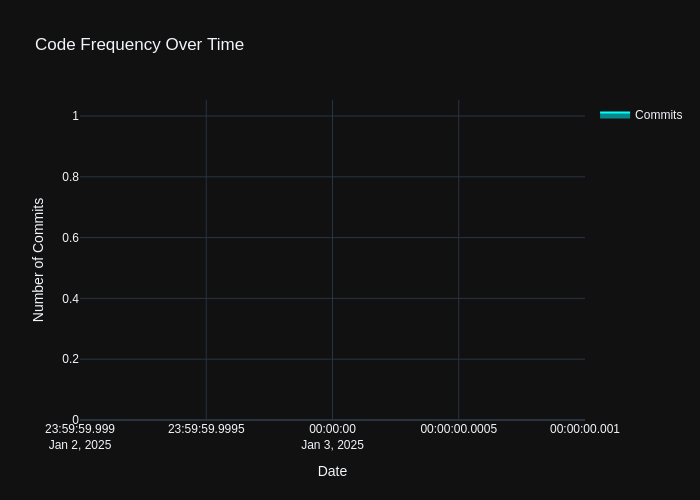
- Repository URL: https://github.com/wanghaisheng/coffee-pal-herb-pal
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日