Building Cognitive Boost Games: A Developer's Journey to SEO Success
Project Genesis
Unlocking the Mind: My Journey into Cognitive Boost Games
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Choice of Programming Language: The team opted for Python due to its rich ecosystem of libraries for web scraping, SEO analysis, and HTTP requests. Libraries like BeautifulSoup for HTML parsing and requests for making HTTP calls were chosen for their ease of use and community support.
-
Sitemap Generation: The decision to auto-generate the sitemap based on language subfolders was driven by the need for a structured approach to indexing. This would ensure that all relevant pages, including .html files, were included, improving the site’s discoverability.
-
SEO Checks: Implementing automated SEO checks was crucial to avoid common pitfalls such as Google redirection issues and non-indexable pages. The team decided to create a set of rules based on best practices in SEO, which would be integrated into the workflow.
-
IndexNow Protocol: The choice to use the IndexNow protocol for URL submission was influenced by its efficiency in notifying search engines about content updates. This decision aimed to streamline the indexing process and improve the site’s responsiveness to changes.
3. Alternative Approaches Considered
-
Manual Sitemap Creation: Initially, there was a discussion about manually creating the sitemap. However, this was quickly dismissed due to the potential for human error and the time-consuming nature of the task, especially for larger sites.
-
Using Third-Party Tools: The team explored the possibility of leveraging existing third-party tools for SEO checks and sitemap generation. While these tools offered convenience, they often lacked customization options and could incur additional costs. Ultimately, building a custom solution was deemed more beneficial for long-term maintenance and adaptability.
-
Different Submission Methods: Other methods for submitting URLs to search engines were considered, such as using the Google Search Console API. However, the IndexNow protocol was favored for its simplicity and direct integration with multiple search engines.
4. Key Insights That Shaped the Project
-
Importance of Automation: The realization that automation could save time and reduce errors was pivotal. By automating the sitemap generation and SEO checks, the team could focus on more strategic tasks, such as content creation and marketing.
-
User-Centric Design: Understanding the end-user’s perspective was crucial. The team recognized that a well-structured sitemap and adherence to SEO best practices would enhance user experience, leading to better engagement and retention.
-
Iterative Development: Embracing an iterative development approach allowed the team to test features incrementally and gather feedback. This flexibility proved invaluable in refining the project and addressing unforeseen challenges.
-
Collaboration and Communication: Regular communication among team members facilitated knowledge sharing and problem-solving. This collaborative environment fostered creativity and innovation, leading to a more robust final product.
Under the Hood
Technical Deep-Dive: WebSim Site Builder
1. Architecture Decisions
- Frontend: A user interface that allows users to interact with the site builder, configure settings, and view reports.
- Backend: A server-side application that handles the logic for sitemap generation, SEO checks, and URL submissions.
- Database: A storage solution for keeping track of site configurations, generated sitemaps, and SEO reports.
Design Principles
- Separation of Concerns: Each component of the architecture is responsible for a specific task, which simplifies debugging and enhances maintainability.
- Scalability: The architecture is designed to handle an increasing number of languages and subfolders without significant performance degradation.
- Extensibility: New features can be added with minimal disruption to existing functionality.
2. Key Technologies Used
- Node.js: The backend is built using Node.js, which allows for asynchronous processing and efficient handling of I/O operations.
- Express.js: A web framework for Node.js that simplifies the creation of server-side applications and APIs.
- Cheerio: A library for parsing and manipulating HTML, used for SEO checks and sitemap generation.
- Google Indexing API: Utilized for submitting URLs to Google for indexing via the IndexNow protocol.
- File System (fs): Node.js’s built-in file system module is used to read and write files, including the generated sitemap.
3. Interesting Implementation Details
Sitemap Generation
fs
module to read the directory structure and generate a sitemap in XML format.const fs = require('fs');
const path = require('path');
function generateSitemap(langFolder) {
const sitemapEntries = [];
const files = fs.readdirSync(langFolder);
files.forEach(file => {
if (file.endsWith('.html')) {
const url = `https://example.com/${path.join(langFolder, file)}`;
sitemapEntries.push(`<url><loc>${url}</loc></url>`);
}
});
const sitemap = `<urlset xmlns="http://www.sitemaps.org/schemas/sitemap-image/1.1">${sitemapEntries.join('')}</urlset>`;
fs.writeFileSync(path.join(langFolder, 'sitemap.xml'), sitemap);
}
SEO Checks
const cheerio = require('cheerio');
function checkSEO(filePath) {
const content = fs.readFileSync(filePath, 'utf-8');
const $ = cheerio.load(content);
const hasTitle = $('title').length > 0;
const hasMetaDescription = $('meta[name="description"]').length > 0;
return {
hasTitle,
hasMetaDescription,
};
}
URL Submission
const axios = require('axios');
async function submitUrlToGoogle(url) {
const response = await axios.post('https://indexing.googleapis.com/v3/urlNotifications:publish', {
url: url,
type: 'URL_UPDATED'
}, {
headers: {
'Authorization': `Bearer ${YOUR_ACCESS_TOKEN}`,
'Content-Type': 'application/json'
}
});
return response.data;
}
4. Technical Challenges Overcome
Handling Multiple Languages
SEO Compliance
Google Indexing API Integration
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Understanding Sitemap Generation: Implementing an auto-generated sitemap based on language subfolders highlighted the importance of structuring content for multilingual sites. It reinforced the need for a clear directory structure to facilitate easier navigation and indexing by search engines.
-
SEO Compliance: The process of checking SEO requirements taught the significance of adhering to best practices. It was crucial to understand how Google interprets redirects and indexing, which can significantly impact site visibility.
-
IndexNow Protocol: Integrating the IndexNow API for URL submission was a valuable lesson in leveraging modern tools for SEO. It emphasized the importance of staying updated with the latest technologies that can enhance site performance and visibility.
What Worked Well
-
Automation of Sitemap Generation: The automated sitemap generation functioned effectively, saving time and reducing the potential for human error. It ensured that all relevant pages were included and updated as content changed.
-
SEO Checks: The automated checks for SEO compliance were beneficial in identifying issues early in the development process. This proactive approach helped in maintaining a healthy site structure and avoiding common pitfalls.
-
Integration with IndexNow: The seamless integration with IndexNow for URL submissions was a highlight. It simplified the process of notifying search engines about new content, leading to faster indexing.
What You’d Do Differently
-
Enhanced Error Handling: While the automation worked well, implementing more robust error handling and logging would be beneficial. This would help in diagnosing issues quickly and improving the overall reliability of the system.
-
User Interface for Configuration: Creating a user-friendly interface for configuring sitemap settings and SEO checks would enhance usability. This would allow non-technical users to easily manage settings without delving into the code.
-
Testing and Validation: More extensive testing, particularly in different environments and with various content types, would help identify edge cases and ensure the system is robust across all scenarios.
Advice for Others
-
Start with Clear Requirements: Before diving into development, clearly outline the project requirements and objectives. This will guide the development process and help avoid scope creep.
-
Leverage Existing Tools: Don’t reinvent the wheel. Utilize existing libraries and tools for sitemap generation and SEO checks. This can save time and reduce complexity.
-
Iterate and Improve: Adopt an iterative approach to development. Start with a minimum viable product (MVP) and gradually add features based on user feedback and testing results.
-
Stay Updated on SEO Practices: SEO is an ever-evolving field. Regularly update your knowledge on best practices and tools to ensure your site remains competitive in search rankings.
What’s Next?
Conclusion: The Future of Cognitive Boost Games
Project Development Analytics
timeline gant
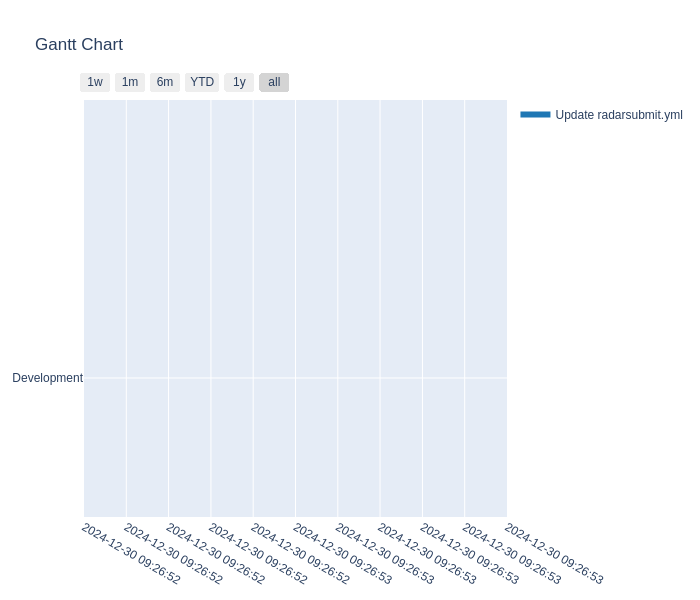
Commit Activity Heatmap
Contributor Network
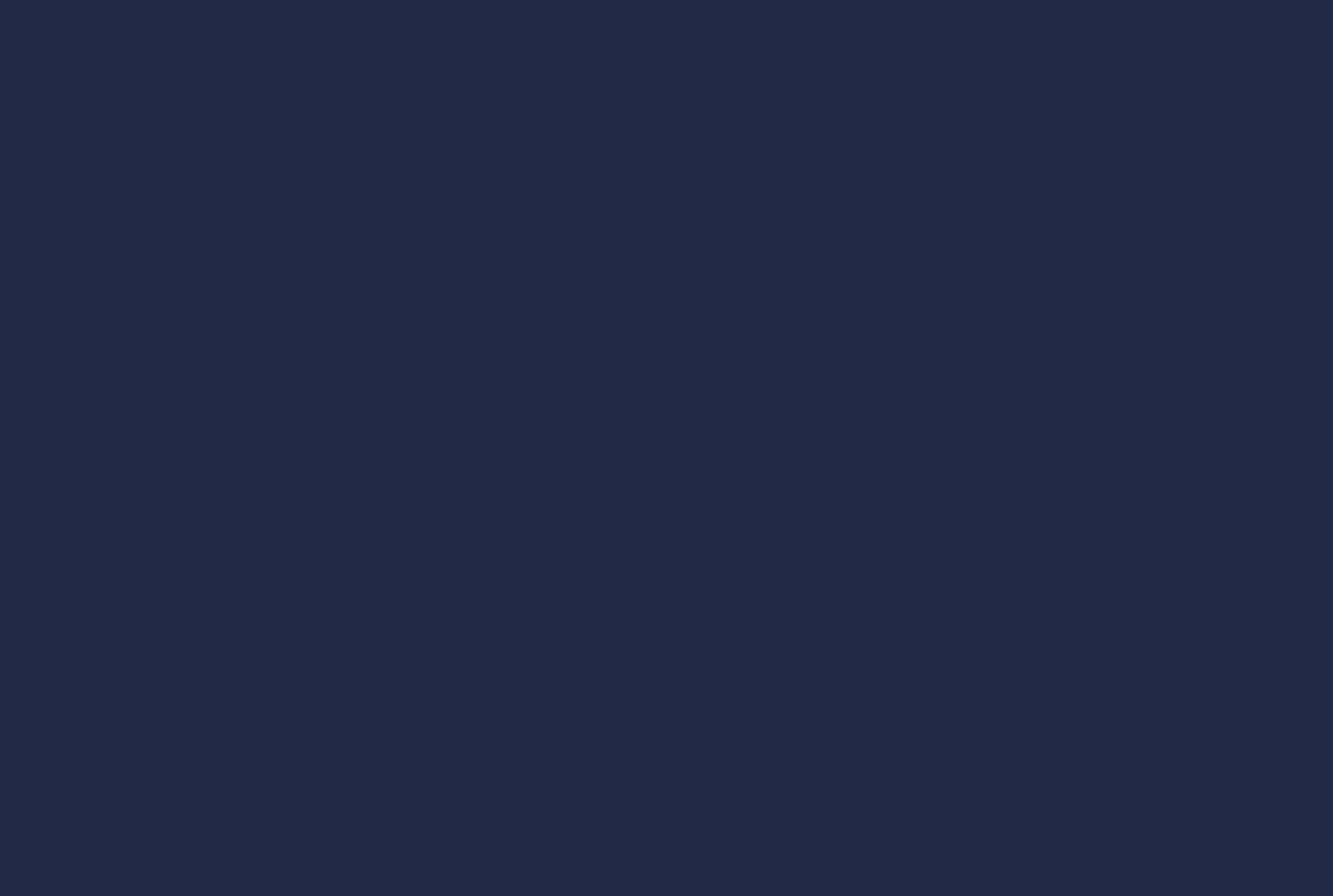
Commit Activity Patterns
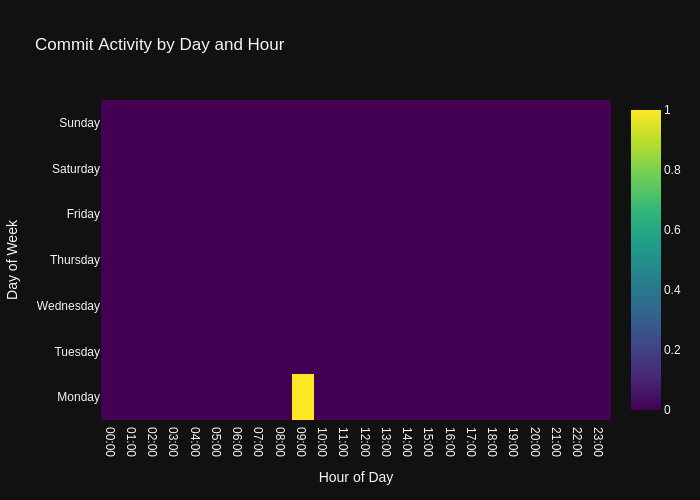
Code Frequency
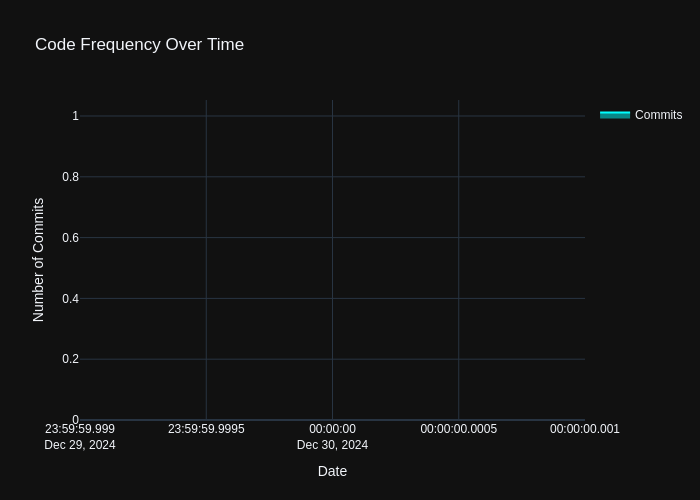
- Repository URL: https://github.com/wanghaisheng/cognitive-boost-games
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日