Building Coinbase-Top-25: A Next.js Developer's Weekend Hack Journey
Project Genesis
Exploring the Top 25 Cryptocurrencies: My Journey with Coinbase and Next.js
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Framework Choice: Next.js was chosen for its hybrid rendering capabilities, allowing us to serve both static and dynamic content efficiently. This decision was driven by the need for fast load times and improved SEO.
-
TypeScript Integration: We opted to use TypeScript for type safety and better developer experience. This decision aimed to reduce runtime errors and improve code maintainability, especially as the project scales.
-
API Routes: Utilizing Next.js API routes allowed us to create a seamless integration between the frontend and backend without the need for a separate server. This streamlined our development process and reduced deployment complexity.
-
Font Optimization: The use of
next/font
for loading custom fonts was implemented to enhance performance by reducing the font loading time, which is crucial for user experience.
3. Alternative Approaches Considered
-
Single Page Applications (SPAs): Initially, we explored building a traditional SPA using React. However, we recognized that the lack of SSR would hinder SEO and performance, especially for content-heavy pages.
-
Static Site Generators (SSGs): We also looked into using static site generators like Gatsby. While they offer excellent performance for static content, the need for dynamic features and API integration made Next.js a more suitable option.
-
Backend Frameworks: We considered using a separate backend framework (like Express) for API development. However, the built-in API routes in Next.js provided a more cohesive development experience, allowing us to keep everything within a single codebase.
4. Key Insights That Shaped the Project
-
User Experience is Paramount: Early user testing highlighted the importance of fast load times and responsive design. This insight reinforced our decision to leverage Next.js’s SSR and SSG capabilities.
-
Simplicity in Architecture: Keeping the architecture simple by using Next.js for both frontend and backend reduced the cognitive load on the development team and minimized deployment challenges.
-
Iterative Development: Adopting an iterative development approach allowed us to gather feedback early and often. This practice led to continuous improvements and adjustments based on user needs and technical challenges encountered during development.
-
Documentation and Community Support: The extensive documentation and active community surrounding Next.js provided invaluable resources and support, making it easier to troubleshoot issues and implement best practices.
Under the Hood
Technical Deep-Dive: Next.js Project
1. Architecture Decisions
Key Architectural Choices:
- File-based Routing: Next.js uses a file-based routing system where the structure of the
pages
directory directly maps to the application’s routes. For example,pages/index.tsx
corresponds to the root route/
, whilepages/api/hello.ts
corresponds to the API route/api/hello
. - API Routes: The project leverages API routes to create backend endpoints within the same codebase. This allows for seamless integration between the frontend and backend, reducing the need for a separate server.
- Static and Dynamic Content: By utilizing both static generation and server-side rendering, the application can serve static content quickly while still being able to fetch dynamic data when necessary.
2. Key Technologies Used
- Next.js: A React framework that enables server-side rendering and static site generation.
- TypeScript: The project uses TypeScript (
.tsx
files) for type safety and improved developer experience. - React: The underlying library for building user interfaces, allowing for component-based architecture.
- Vercel: The deployment platform that provides seamless integration with Next.js, enabling easy deployment and scaling.
- next/font: A feature that optimizes font loading, specifically for the Inter font, improving performance and user experience.
3. Interesting Implementation Details
Auto-Updating Pages
pages/index.tsx
file. This is achieved through Next.js’s hot reloading feature, which automatically refreshes the browser when changes are detected.API Route Example
pages/api/hello.ts
can be implemented as follows:// pages/api/hello.ts
import type { NextApiRequest, NextApiResponse } from 'next';
export default function handler(req: NextApiRequest, res: NextApiResponse) {
res.status(200).json({ message: 'Hello, World!' });
}
/api/hello
, demonstrating how easy it is to create backend functionality within a Next.js application.Font Optimization
next/font
for loading the Inter font can be implemented as follows:// pages/_app.tsx
import { Inter } from 'next/font/google';
const inter = Inter({ subsets: ['latin'] });
function MyApp({ Component, pageProps }) {
return (
<main className={inter.className}>
<Component {...pageProps} />
</main>
);
}
export default MyApp;
4. Technical Challenges Overcome
Managing State Across Pages
// context/MyContext.tsx
import { createContext, useContext, useState } from 'react';
const MyContext = createContext(null);
export const MyProvider = ({ children }) => {
const [state, setState] = useState({});
return (
<MyContext.Provider value={{ state, setState }}>
{children}
</MyContext.Provider>
);
};
export const useMyContext = () => useContext(MyContext);
Handling API Errors
// pages/api/hello.ts
export default function handler(req: NextApiRequest, res: NextApiResponse) {
try {
// Simulate some logic
res.status(200).json({ message: 'Hello, World!' });
} catch (error) {
res.status(500).json({ error: 'Internal Server Error' });
}
}
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- File-Based Routing: Next.js uses a file-based routing system, which simplifies the creation of routes. Understanding how to structure the
pages
directory effectively is crucial for maintaining a clean and organized codebase. - API Routes: The ability to create API routes within the same project is a powerful feature. It allows for seamless integration of backend functionality without needing a separate server setup. Learning how to handle requests and responses in these routes was essential.
- Automatic Code Splitting: Next.js automatically splits code for each page, which improves performance. Understanding how this works can help in optimizing the application further.
- Static Site Generation (SSG) and Server-Side Rendering (SSR): Familiarizing myself with the differences between SSG and SSR, and knowing when to use each, was important for optimizing load times and SEO.
2. What Worked Well
- Development Experience: The hot-reloading feature made development smooth and efficient. Changes reflected immediately in the browser, which sped up the development process.
- Integration with CSS and Fonts: Using
next/font
for font optimization was straightforward and improved loading times. The integration of CSS modules also helped in maintaining scoped styles. - Community and Documentation: The extensive documentation and community support for Next.js made it easy to find solutions to problems and learn best practices.
3. What You’d Do Differently
- State Management: Initially, I relied on local component state for managing data. In larger applications, I would consider using a state management library (like Redux or Zustand) from the start to better manage global state.
- TypeScript Usage: While I used TypeScript, I would invest more time in understanding its advanced features and best practices to improve type safety and reduce runtime errors.
- Testing: I would implement testing (unit and integration tests) earlier in the development process to ensure code quality and catch bugs before deployment.
4. Advice for Others
- Start Small: If you’re new to Next.js, start with a small project to familiarize yourself with its features before scaling up to more complex applications.
- Leverage the Documentation: Don’t hesitate to refer to the official Next.js documentation. It’s comprehensive and provides examples that can save you time.
- Explore Deployment Options Early: Familiarize yourself with deployment options, especially Vercel, as it integrates seamlessly with Next.js. Understanding the deployment process early can help avoid last-minute issues.
- Stay Updated: Next.js is actively developed, and new features are regularly added. Keep an eye on the changelog and community discussions to stay updated on best practices and new capabilities.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
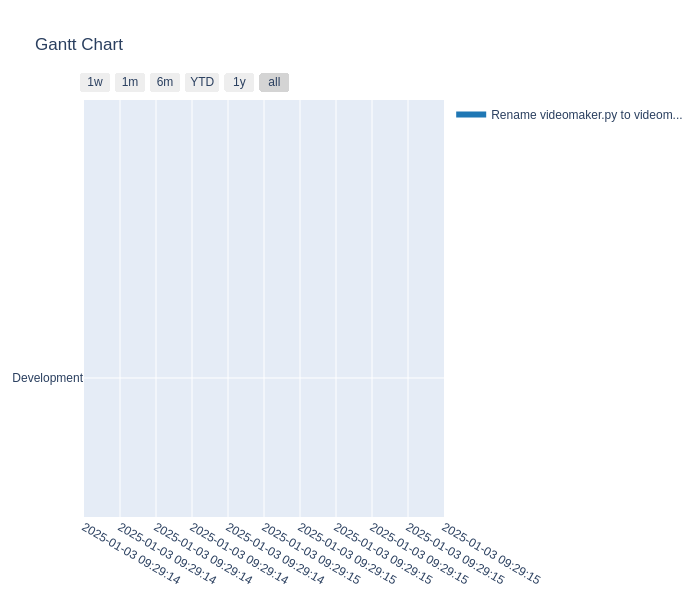
Commit Activity Heatmap
Contributor Network
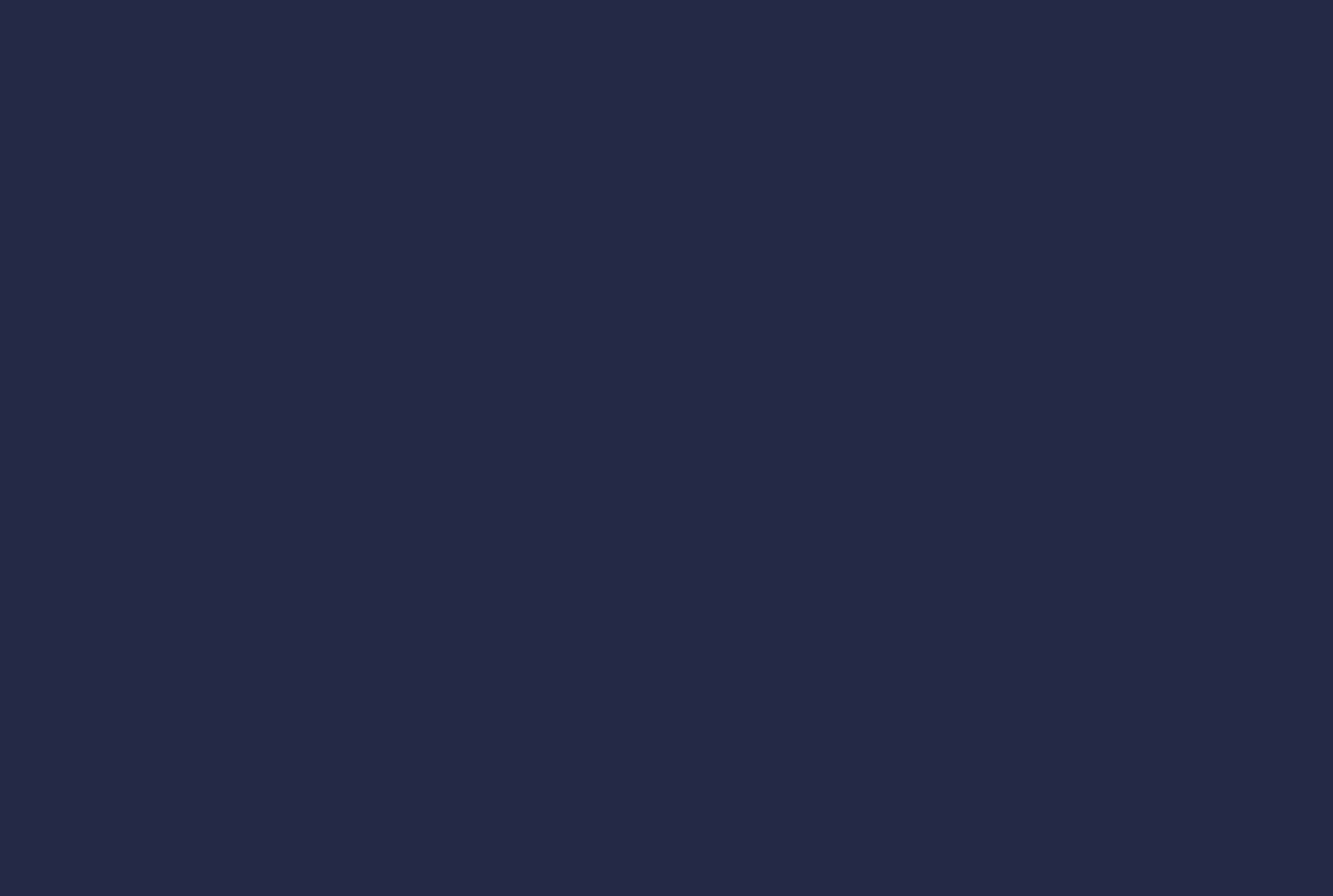
Commit Activity Patterns
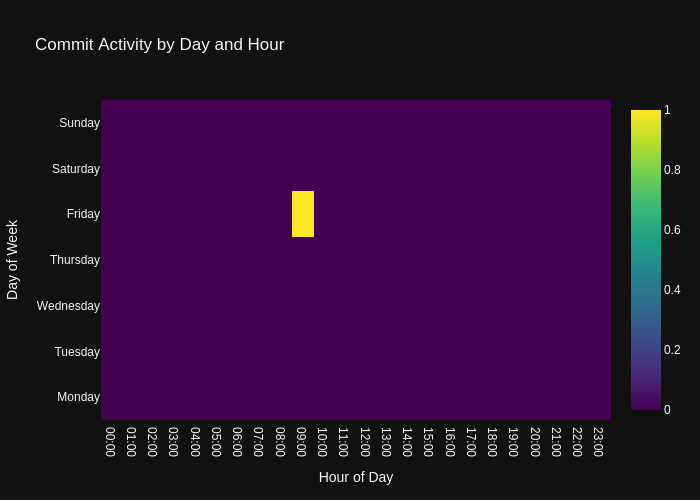
Code Frequency
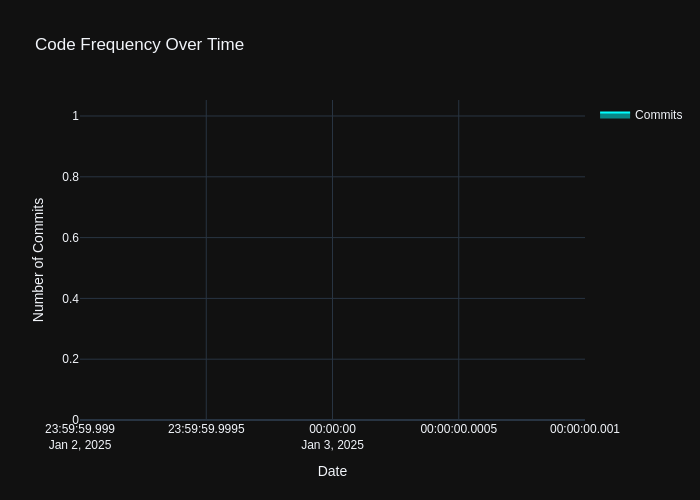
- Repository URL: https://github.com/wanghaisheng/coinbase-top-25
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日