From Idea to Reality: Building a HonoJS REST API on Cloudflare Workers
Project Genesis
Crafting a Seamless REST API with HonoJS and Cloudflare Workers
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Framework Choice: HonoJS was selected for its lightweight nature and focus on performance, making it ideal for serverless deployments on Cloudflare Workers. Its middleware capabilities allow for easy extension and customization of the API.
-
Authentication: The implementation of JWT for authentication was chosen for its stateless nature, allowing for easy scalability and reduced server load. This decision also aligned with modern best practices in API security.
-
Validation with Zod: The use of Zod for route validation was driven by the need for type-safe validation that integrates well with TypeScript. This choice enhances code reliability and reduces runtime errors.
-
Testing Framework: Jest was selected for testing due to its comprehensive feature set, including support for unit and integration testing, as well as its ease of use and extensive documentation.
-
Code Quality Tools: The integration of ESLint and Prettier was aimed at maintaining code quality and consistency throughout the project. This decision was made to facilitate collaboration and ensure that the codebase remains clean and maintainable.
3. Alternative Approaches Considered
-
Other Frameworks: While HonoJS was ultimately chosen, other frameworks such as Express.js and Fastify were evaluated. However, they were deemed too heavy for the specific requirements of a Cloudflare Workers environment.
-
Authentication Methods: Alternatives to JWT, such as OAuth2, were considered. However, the complexity of implementing OAuth2 for a simple REST API led to the decision to stick with JWT, which provided a more straightforward solution.
-
Testing Libraries: Other testing libraries like Mocha and Chai were explored, but Jest’s all-in-one approach and built-in mocking capabilities made it the preferred choice.
4. Key Insights That Shaped the Project
-
Simplicity is Key: The importance of keeping the setup minimal and straightforward became evident. This approach not only accelerates development but also makes it easier for new developers to onboard and contribute.
-
Modularity and Extensibility: Designing the API with middleware in mind allowed for greater flexibility and the ability to easily add new features or modify existing ones without disrupting the core functionality.
-
Testing as a Priority: Early emphasis on testing led to a more robust codebase. The realization that thorough testing can prevent future issues and facilitate smoother deployments was a crucial takeaway.
-
Community and Documentation: Leveraging well-documented libraries and frameworks proved invaluable. The availability of resources and community support played a significant role in overcoming challenges and accelerating development.
Under the Hood
Technical Deep-Dive: create-hono-cloudflare-workers-rest-api
1. Architecture Decisions
create-hono-cloudflare-workers-rest-api
project is designed to leverage the capabilities of Cloudflare Workers, which allows developers to run JavaScript code at the edge. This architecture is particularly beneficial for building REST APIs due to its low latency and scalability.Key Architectural Choices:
- Serverless Framework: The use of Cloudflare Workers means that the application is serverless, allowing for automatic scaling and reduced operational overhead.
- Microservices Approach: The project is structured to support a microservices architecture, where each endpoint can be developed, deployed, and scaled independently.
- Middleware Pattern: The application utilizes middleware to handle cross-cutting concerns such as authentication, logging, and error handling, promoting separation of concerns and reusability.
2. Key Technologies Used
- HonoJS: A lightweight web framework for building APIs, which provides a simple and intuitive API for defining routes and middleware.
- JWT (JSON Web Tokens): Used for secure authentication, allowing stateless user sessions.
- Zod: A TypeScript-first schema declaration and validation library, which is used for route validation to ensure that incoming requests meet expected formats.
- Bun: A modern JavaScript runtime that is fast and efficient, used for package management and running scripts.
- Jest: A testing framework that provides a robust environment for writing and running tests, including unit and integration tests.
- ESLint and Prettier: Tools for maintaining code quality and consistency, ensuring that the codebase adheres to best practices.
3. Interesting Implementation Details
Middleware Magic
import { NextFunction, Request, Response } from 'hono';
import jwt from 'jsonwebtoken';
const authenticateJWT = (req: Request, res: Response, next: NextFunction) => {
const token = req.headers['authorization']?.split(' ')[1];
if (token) {
jwt.verify(token, process.env.JWT_SECRET, (err, user) => {
if (err) {
return res.status(403).send('Forbidden');
}
req.user = user;
next();
});
} else {
res.status(401).send('Unauthorized');
}
};
Route Validation with Zod
import { z } from 'zod';
const userSchema = z.object({
name: z.string().min(1),
email: z.string().email(),
});
app.post('/users', async (req, res) => {
const result = userSchema.safeParse(req.body);
if (!result.success) {
return res.status(400).send(result.error.format());
}
// Proceed with creating the user
});
Grouping Tests
@group
annotation, which allows developers to run specific groups of tests easily. This is particularly useful for large codebases where tests can be categorized into unit and integration tests./**
* @group unit
*/
test('should return user by ID', async () => {
// Test implementation
});
/**
* @group integration
*/
test('should create a new user', async () => {
// Test implementation
});
4. Technical Challenges Overcome
Handling Asynchronous Operations
app.get('/users/:id', async (req, res) => {
try {
const user = await getUserById(req.params.id);
if (!user) {
return res.status(404).send('User not found');
}
res.json(user);
} catch (error) {
res.status(500).send('Internal Server Error');
}
});
Ensuring Security with JWT
Testing Strategy
# Running all tests
bun run test
# Running specific groups of tests
bun run unit
bun run integration
Conclusion
create-hono-cloudflare-workers-rest-api
Lessons from the Trenches
Key Technical Lessons Learned
-
Middleware Implementation: Utilizing middleware effectively can streamline request handling and enhance code modularity. Understanding how to create and apply middleware in HonoJS was crucial for managing authentication and validation.
-
JWT Authentication: Implementing JWT for authentication provided a secure way to manage user sessions. Learning how to encode and decode JWT tokens was essential for protecting routes and ensuring that only authorized users could access certain endpoints.
-
Route Validation with Zod: Using Zod for route validation helped catch errors early in the request lifecycle. This reinforced the importance of validating incoming data to prevent issues down the line.
-
Testing Strategies: The distinction between unit and integration tests clarified the testing process. Learning to use Jest for both types of tests improved code reliability and confidence in deployments.
What Worked Well
-
Minimal Setup: The initial setup process was straightforward and efficient, allowing for rapid development. The use of
npx degit
to clone the template made it easy to get started. -
Clear Documentation: The README provided clear instructions for installation, running, and testing the application. This clarity helped onboard new developers quickly.
-
Code Quality Tools: Integrating ESLint and Prettier ensured that the codebase remained clean and consistent. This practice improved collaboration among team members and reduced code review friction.
-
Testing Framework: Jest’s capabilities for grouping tests and running specific test suites made it easy to manage and execute tests, leading to better coverage and fewer bugs.
What You’d Do Differently
-
More Comprehensive Examples: While the README provided a good overview, including more detailed examples of middleware usage, JWT implementation, and Zod validation would have been beneficial for new users.
-
Error Handling: Implementing a more robust error handling strategy from the start could improve the user experience and make debugging easier. This could include standardized error responses and logging.
-
Environment Configuration: Providing a clearer guide on managing environment variables and configurations for different environments (development, testing, production) would enhance deployment practices.
-
CI/CD Integration: Setting up continuous integration and deployment (CI/CD) pipelines early in the project could streamline the deployment process and ensure that tests are run automatically on code changes.
Advice for Others
-
Start Small: When working with new frameworks or libraries, start with a small, focused project to understand the core concepts before scaling up.
-
Leverage Community Resources: Engage with the community around HonoJS and related technologies. Forums, GitHub issues, and Discord channels can provide valuable insights and support.
-
Prioritize Testing: Invest time in writing tests early in the development process. This practice pays off by reducing bugs and improving code quality.
-
Document as You Go: Keep documentation up to date as the project evolves. This practice helps onboard new team members and serves as a reference for existing developers.
-
Experiment with Features: Don’t hesitate to experiment with different features and libraries. This exploration can lead to discovering better solutions and improving your overall development skills.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
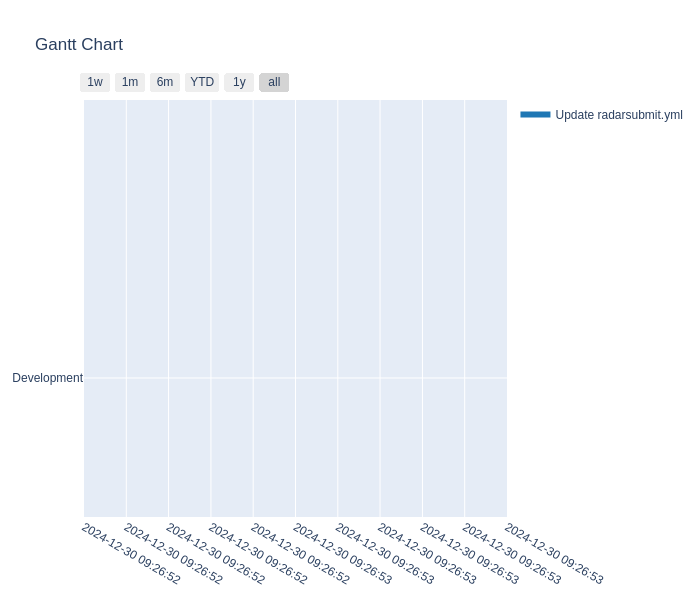
Commit Activity Heatmap
Contributor Network
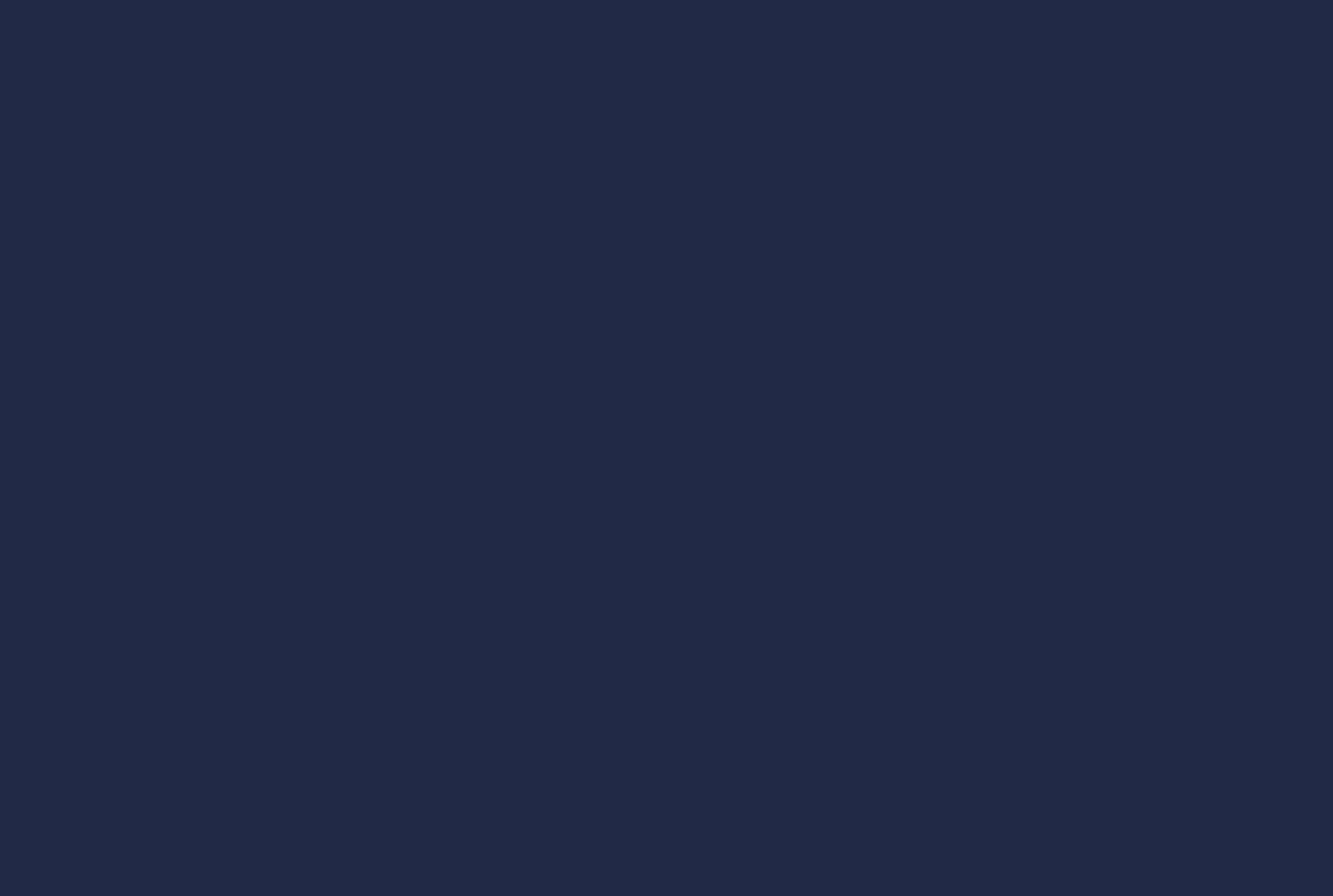
Commit Activity Patterns
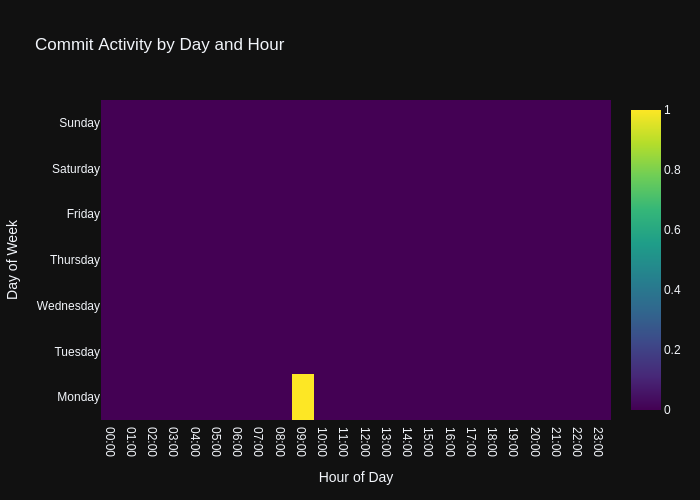
Code Frequency
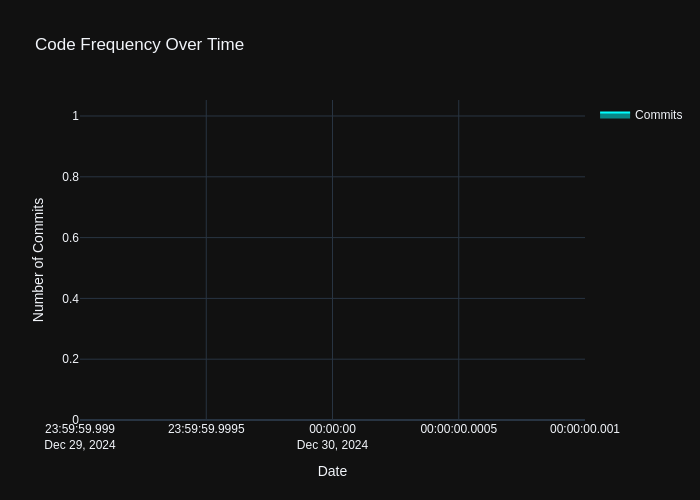
- Repository URL: https://github.com/wanghaisheng/create-hono-cloudflare-workers-rest-api
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日